Is the Unsafe Assignment of Any Value a Hidden Risk in Your Code?
In the ever-evolving landscape of programming, the concept of type safety has emerged as a cornerstone of robust software development. As developers strive to create more reliable and maintainable code, the notion of “unsafe assignment of an any value” has garnered significant attention. This phrase encapsulates a critical challenge faced by many programmers, particularly those working with languages that support dynamic typing or flexible type systems. Understanding the implications of such assignments is essential for anyone looking to elevate their coding practices and safeguard their applications against potential pitfalls.
At its core, the unsafe assignment of an any value refers to the practice of assigning a variable of a more general type—often denoted as “any”—to a more specific type without adequate checks. This can lead to unexpected behavior, runtime errors, and a host of debugging headaches down the line. As developers navigate through the complexities of type systems, the temptation to leverage the flexibility of “any” can be alluring, but it often comes at the cost of clarity and safety in the codebase.
In this article, we will delve into the nuances of type safety, exploring the risks associated with unsafe assignments and the best practices to mitigate these dangers. By examining real-world examples and drawing on industry insights, we aim to equip developers with the knowledge they need
Understanding Unsafe Assignments in TypeScript
In TypeScript, the concept of “unsafe assignment of an any value” arises from the language’s type system, which aims to provide strong typing to enhance code quality and maintainability. When a variable is assigned a value of type `any`, it bypasses the type-checking mechanisms that TypeScript provides. This can lead to unexpected behaviors and bugs in the application, especially in larger codebases.
The `any` type is a powerful feature that allows developers to opt-out of type checking for specific variables. However, excessive use of `any` can undermine the benefits of TypeScript’s type safety. Here are some key points to consider regarding `any` assignments:
- Loss of Type Safety: Assigning an `any` value to a variable means that the compiler will not enforce type constraints on that variable.
- Potential for Runtime Errors: Since type checks are bypassed, errors that could have been caught at compile time may only surface at runtime, leading to more complex debugging.
- Maintainability Challenges: Code that heavily relies on `any` becomes harder to understand and maintain, as it obscures the intended types of variables.
Examples of Unsafe Assignments
Consider the following example, which illustrates unsafe assignments using the `any` type:
“`typescript
let value: any;
value = 42; // Assigning a number
value = “Hello”; // Now it’s a string
value = { name: “Alice” }; // Now it’s an object
“`
In this scenario, `value` can hold any type without restrictions, making it difficult to predict its type throughout the code.
Best Practices for Avoiding Unsafe Assignments
To minimize unsafe assignments, developers should follow several best practices:
- Use Specific Types: Instead of `any`, define more specific types or use union types when multiple types are acceptable.
- Leverage Type Assertions: When dealing with dynamic data, use type assertions to inform the compiler of the expected type.
- Enable Strict Mode: Utilizing TypeScript’s strict mode helps catch potential issues early by enforcing stricter type checks.
Practice | Description | Example |
---|---|---|
Specific Types | Define clear types for variables. | let username: string; |
Union Types | Allow a variable to be one of several types. | let id: string | number; |
Type Assertions | Specify the type when necessary. | let value = |
By adopting these practices, developers can significantly reduce the risks associated with unsafe assignments and enhance the robustness of their TypeScript applications.
Understanding Unsafe Assignment of ‘any’ Values
In TypeScript, the ‘any’ type is a powerful feature that allows developers to bypass the strict typing system. However, using ‘any’ can lead to unsafe assignments, which may result in runtime errors that are difficult to trace and fix. It is essential to recognize the implications of assigning ‘any’ values in your applications.
Potential Risks of Using ‘any’
Assigning ‘any’ can introduce several risks, including:
- Loss of Type Safety: The primary benefit of TypeScript is its ability to enforce type safety. Using ‘any’ undermines this advantage, allowing any value to be assigned without compile-time checks.
- Runtime Errors: Since type checks are bypassed, accessing properties or methods on ‘any’ types may lead to behavior at runtime.
- Code Maintainability: Codebases that heavily rely on ‘any’ become challenging to understand and maintain, making it harder for new developers to grasp the intended data structures.
Best Practices to Avoid Unsafe Assignments
To maintain type safety and avoid unsafe assignments, consider the following best practices:
- Use Specific Types: Instead of using ‘any’, define specific types or interfaces that accurately represent the data structure.
“`typescript
interface User {
id: number;
name: string;
}
const user: User = { id: 1, name: ‘Alice’ };
“`
- Utilize Type Assertions: When you must use ‘any’, leverage type assertions to specify what type you expect.
“`typescript
const value: any = “hello”;
const strValue: string = value as string;
“`
- Use Unknown Instead of Any: The ‘unknown’ type forces you to perform some form of type checking before use, providing a safer alternative.
“`typescript
let value: unknown;
value = 42; // Assigning a number
if (typeof value === ‘number’) {
console.log(value + 2); // Safe to use as number
}
“`
Common Scenarios Leading to Unsafe Assignments
Certain coding practices can lead to unsafe assignments of ‘any’. Here are common scenarios:
Scenario | Description | Recommended Action |
---|---|---|
Using JSON.parse | Parsing JSON can return ‘any’ type, leading to unsafe assignments. | Define a type for parsed data and validate structure. |
External Libraries | Libraries without TypeScript definitions may return ‘any’. | Create type definitions or use community types. |
Dynamic Object Properties | Adding properties dynamically can lead to ‘any’ assignments. | Define a consistent interface for objects. |
Tools and Techniques for Mitigating Risks
To help mitigate the risks associated with ‘any’, developers can use various tools and techniques:
- TypeScript Compiler Options: Enable strict mode in `tsconfig.json` to catch ‘any’ usages.
“`json
{
“compilerOptions”: {
“strict”: true
}
}
“`
- Linters: Use linters like ESLint with TypeScript plugins to enforce type safety rules.
“`json
{
“rules”: {
“@typescript-eslint/no-explicit-any”: “warn”
}
}
“`
- Code Reviews: Implement a code review process that emphasizes the importance of type safety, discouraging the use of ‘any’.
By following these guidelines, developers can effectively manage the risks associated with the unsafe assignment of ‘any’ values, ensuring a more robust and maintainable codebase.
Understanding the Risks of Unsafe Assignment in Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The unsafe assignment of an any value can lead to significant runtime errors that are difficult to trace. Developers must be cautious when using ‘any’ in TypeScript, as it bypasses type safety, potentially introducing bugs that compromise application stability.”
Michael Tran (Lead Developer, CodeGuard Solutions). “Using ‘any’ in TypeScript is akin to driving without a seatbelt. While it may provide short-term flexibility, it ultimately increases the risk of unexpected behavior in your code. Adopting stricter type definitions can mitigate these risks and enhance code quality.”
Sarah Kim (Software Quality Assurance Specialist, DevSecure). “The implications of unsafe assignment are not just technical; they can affect user experience and security. Teams should prioritize type safety and consider implementing code reviews and static analysis tools to identify and rectify instances of unsafe ‘any’ usage.”
Frequently Asked Questions (FAQs)
What does “unsafe assignment of an any value” mean?
The phrase refers to a situation in TypeScript where a variable of type `any` is assigned to a variable of a more specific type without proper type checking, which can lead to runtime errors.
Why is using `any` considered unsafe in TypeScript?
Using `any` bypasses TypeScript’s type system, eliminating the benefits of type safety. This can result in unexpected behavior and bugs that are harder to detect during development.
How can I avoid unsafe assignments in TypeScript?
To avoid unsafe assignments, use explicit types or generics instead of `any`. This ensures that the TypeScript compiler can enforce type checks and catch potential errors.
What are the risks of using `any` in a TypeScript project?
The risks include increased likelihood of runtime errors, reduced code maintainability, and challenges in understanding code behavior, as type information is lost when using `any`.
Can I configure TypeScript to prevent the use of `any`?
Yes, you can configure TypeScript by setting the `noImplicitAny` option to `true` in the `tsconfig.json` file. This setting will raise errors when variables are implicitly assigned the type `any`.
What alternatives exist for using `any` in TypeScript?
Alternatives include using specific types, union types, or generics. These options provide more control over the data types and enhance type safety in your code.
The concept of “unsafe assignment of an any value” primarily pertains to programming languages that utilize type systems, particularly TypeScript. In such languages, the ‘any’ type acts as a wildcard, allowing developers to bypass strict type checks. While this flexibility can be beneficial in certain scenarios, it also introduces significant risks, such as runtime errors and unexpected behavior, which can undermine the reliability and maintainability of the codebase.
One of the main concerns with using ‘any’ is that it effectively disables type safety. When variables are assigned an ‘any’ type, the compiler cannot enforce type checks, leading to potential issues where incorrect data types are used in operations. This can result in bugs that are difficult to trace, as they may not surface until the code is executed. Therefore, relying on ‘any’ can lead to a sense of security, where developers assume their code is functioning correctly without adequate validation.
To mitigate the risks associated with ‘any’, best practices recommend using more specific types whenever possible. Developers should aim to define clear interfaces and utilize union types to maintain type safety while still allowing for flexibility. By doing so, they can ensure that their code is both robust and easier to understand, ultimately leading to higher quality software that
Author Profile
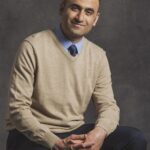
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?