Why Am I Seeing a TypeError: ‘tuple’ Object is Not Callable and How Can I Fix It?
If you’ve ever delved into the world of Python programming, chances are you’ve encountered the notorious `TypeError: ‘tuple’ object is not callable`. This seemingly cryptic error message can be a source of frustration for both novice and seasoned developers alike. As you navigate through your code, the sudden appearance of this error can halt your progress, leaving you puzzled and searching for answers. But fear not! Understanding the nuances behind this error can not only save you time but also enhance your coding skills and problem-solving abilities.
In the realm of Python, tuples are a fundamental data structure, often used to store collections of items. However, the versatility of tuples can sometimes lead to unexpected behaviors, especially when they are mistakenly treated as callable functions. This article will unravel the mystery behind the `TypeError: ‘tuple’ object is not callable`, exploring common scenarios that lead to this error and providing insights into best practices for avoiding it in your code.
By the end of this journey, you will not only grasp the underlying principles of this error but also gain a deeper appreciation for Python’s data types and their proper usage. Whether you’re debugging a complex application or honing your coding skills, understanding this error will empower you to write cleaner, more efficient code. Prepare to dive into
Understanding the TypeError
The error message `TypeError: ‘tuple’ object is not callable` typically arises in Python when an attempt is made to call a tuple as if it were a function. This situation often occurs due to a naming conflict or a misunderstanding of how tuples operate within the language.
Common scenarios that trigger this error include:
- Variable Shadowing: When a variable name is reused to redefine a built-in function or a tuple, leading to ambiguity. For example, if a tuple is assigned to a variable named `list`, Python will not be able to distinguish between the original list function and the new tuple.
- Incorrect Parentheses Usage: Using parentheses intended for function calls on a tuple can cause Python to interpret the tuple as a callable object.
To illustrate, consider the following example:
“`python
my_tuple = (1, 2, 3)
result = my_tuple() This will raise TypeError
“`
In this case, `my_tuple` is a tuple, and attempting to call it with parentheses results in an error.
Common Causes and Solutions
To effectively address the `TypeError: ‘tuple’ object is not callable`, it is essential to identify its root causes and implement appropriate solutions. Below are common causes along with their remedies:
Cause | Description | Solution |
---|---|---|
Variable Shadowing | Assigning a tuple to a variable name that shadows a built-in function. | Change the variable name to avoid conflicts. |
Misuse of Parentheses | Attempting to call a tuple with parentheses. | Remove parentheses when accessing tuple elements. |
Incorrect Function Definitions | Defining a function that returns a tuple but using the tuple incorrectly. | Ensure functions return expected types and access them correctly. |
Best Practices to Avoid TypeErrors:
- Always use descriptive variable names that do not conflict with built-in types or functions.
- Be mindful of the data types you are working with, especially in dynamic languages like Python.
- Utilize tools such as linters to catch potential conflicts before they lead to runtime errors.
Debugging Tips
When encountering the `TypeError: ‘tuple’ object is not callable`, the following debugging strategies can be helpful:
- Check Variable Names: Review the scope of your variables to ensure no naming conflicts exist.
- Print Statements: Use print statements to inspect the types of variables before the error occurs. For example:
“`python
print(type(my_variable))
“`
- Trace the Call Stack: Utilize debugging tools to trace back the function calls leading up to the error, allowing you to pinpoint the exact location of the issue.
By understanding the underlying mechanisms that lead to this error and employing systematic debugging techniques, developers can effectively resolve the `TypeError: ‘tuple’ object is not callable` and enhance their coding practices.
Understanding the Error
The `TypeError: ‘tuple’ object is not callable` typically arises in Python when you attempt to call a tuple as if it were a function. This often occurs due to variable name shadowing or incorrect usage of parentheses.
Common Causes
- Variable Shadowing: You may have assigned a tuple to a variable name that is also the name of a function. For example:
“`python
max = (1, 2, 3) Shadowing the built-in max() function
print(max(5)) Raises TypeError: ‘tuple’ object is not callable
“`
- Incorrect Parentheses: Misusing parentheses when trying to access elements of a tuple can lead to this error. For example:
“`python
my_tuple = (1, 2, 3)
result = my_tuple(0) Incorrect usage, should be my_tuple[0]
“`
- Returning a Tuple: If a function is designed to return multiple values as a tuple, and you mistakenly call it with parentheses, this can also trigger the error.
Examples and Solutions
To provide clarity, here are specific examples of this error along with their solutions.
Example 1: Shadowing Built-in Functions
“`python
Problematic code
min = (1, 2, 3)
print(min(0)) Raises TypeError
Solution
del min Remove the shadowing variable
print(min(1, 2, 3)) Correct usage
“`
Example 2: Misusing Parentheses
“`python
Problematic code
coordinates = (10, 20)
x = coordinates(0) Raises TypeError
Solution
x = coordinates[0] Correctly access the first element
“`
Example 3: Returning a Tuple
“`python
def get_values():
return (1, 2)
Problematic code
result = get_values(0) Raises TypeError
Solution
result = get_values()[0] Correctly access the first value from the returned tuple
“`
Best Practices to Avoid the Error
To mitigate the risk of encountering this error, consider the following best practices:
- Avoid Shadowing: Use unique variable names that do not conflict with built-in functions.
- Be Mindful of Parentheses: Differentiate between function calls and indexing. Always use brackets (`[]`) for accessing tuple elements.
- Code Reviews: Regularly review your code to identify potential variable shadowing issues and ensure clarity in function and variable names.
Quick Reference Table
Issue Type | Example Code | Fix |
---|---|---|
Variable Shadowing | `max = (1, 2, 3)` | Use `del max` to remove shadowing. |
Incorrect Parentheses Usage | `my_tuple(0)` | Change to `my_tuple[0]`. |
Misuse of Return Values | `get_values(0)` | Use `get_values()[0]`. |
By adhering to these practices and understanding the underlying causes of the `TypeError: ‘tuple’ object is not callable`, you can write more robust Python code and avoid common pitfalls.
Understanding the ‘Tuple’ Object Error in Python Programming
Dr. Emily Carter (Senior Software Engineer, CodeSolutions Inc.). “The ‘TypeError: ‘tuple’ object is not callable’ typically arises when a tuple is mistakenly invoked as a function. This often occurs when a variable name shadows a built-in function, leading to confusion in the code execution.”
Michael Chen (Python Developer, Tech Innovations). “To resolve this error, developers should carefully check their variable names and ensure that they are not inadvertently using tuples where function calls are expected. Debugging tools can be invaluable in tracing the source of the issue.”
Sarah Johnson (Lead Data Scientist, DataVision Analytics). “Understanding the distinction between tuples and callable objects is crucial for Python developers. This error serves as a reminder to maintain clear and consistent naming conventions to prevent such conflicts in the code.”
Frequently Asked Questions (FAQs)
What does the error ‘typeerror: ‘tuple’ object is not callable’ mean?
This error indicates that a tuple is being treated as a callable function. In Python, tuples are not callable, so attempting to invoke them like a function results in this TypeError.
What are common causes of the ‘typeerror: ‘tuple’ object is not callable’ error?
Common causes include accidentally overwriting a function name with a tuple, using parentheses incorrectly when attempting to access tuple elements, or mistakenly trying to call a tuple as if it were a function.
How can I resolve the ‘typeerror: ‘tuple’ object is not callable’ error?
To resolve this error, check your variable names to ensure no functions have been overwritten by tuples. Review your code for incorrect parentheses usage and ensure that you are accessing tuple elements properly.
Can you provide an example that triggers the ‘typeerror: ‘tuple’ object is not callable’ error?
Certainly. An example is as follows:
“`python
my_tuple = (1, 2, 3)
result = my_tuple() This line will raise the TypeError.
“`
Is it possible to prevent this error from occurring in my code?
Yes, to prevent this error, avoid using the same name for variables and functions. Additionally, be cautious with parentheses, ensuring they are used correctly for function calls and not for accessing tuple elements.
What should I do if I encounter this error in a third-party library?
If the error occurs in a third-party library, check the library’s documentation for updates or known issues. You may also consider reaching out to the library’s support or community for assistance.
The error message “TypeError: ‘tuple’ object is not callable” typically arises in Python programming when an attempt is made to call a tuple as if it were a function. This situation often occurs due to variable name conflicts or incorrect usage of parentheses, leading to confusion between tuples and callable objects like functions. Understanding the context in which this error arises is crucial for effective debugging and resolution.
One common scenario that leads to this error is when a variable that was initially intended to store a function is inadvertently reassigned to a tuple. For instance, if a developer names a variable ‘my_function’ and later assigns a tuple to it, any subsequent attempts to invoke ‘my_function’ will result in a TypeError. Identifying such variable shadowing is essential for preventing this error from occurring.
To avoid the “TypeError: ‘tuple’ object is not callable,” developers should adhere to best practices in naming conventions and variable management. It is advisable to use descriptive names that clearly indicate the variable’s purpose and to avoid reusing names for different data types. Additionally, careful attention to the use of parentheses can help distinguish between calling functions and creating tuples, thereby reducing the likelihood of this error.
Author Profile
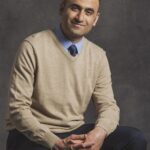
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?