How Can You Assign an Event Handler in C++ Builder?
In the world of software development, creating responsive and interactive applications is essential for enhancing user experience. One of the key elements that contribute to this interactivity is the use of event handlers. For developers working with C++ Builder, mastering the art of assigning event handlers can open the door to a more dynamic and engaging application. Whether you are a seasoned programmer or just starting your journey in C++ Builder, understanding how to effectively manage events will empower you to create applications that respond seamlessly to user actions.
Event handling in C++ Builder is a fundamental concept that allows your application to react to various user inputs, such as mouse clicks, keyboard presses, or other actions. By assigning event handlers, you can define specific behaviors that occur when these events are triggered, making your application more intuitive and user-friendly. This process involves linking specific events to functions or methods that contain the logic you want to execute, enabling a smooth flow of interaction within your application.
As you delve deeper into the mechanics of event handling in C++ Builder, you will discover the various components and techniques that facilitate this process. From understanding the event-driven programming model to exploring the different types of events available in the framework, this article will guide you through the essential steps needed to assign event handlers effectively. Get ready
Understanding Event Handlers in C++ Builder
In C++ Builder, event handlers are crucial for responding to user actions or system events. An event handler is a function that is executed in response to a specific event, such as a button click, a mouse movement, or a keyboard input. Assigning event handlers allows developers to define how the application should behave when these events occur.
Assigning Event Handlers
To assign an event handler in C++ Builder, follow these key steps:
- Identify the Event: Determine which event you want to handle (e.g., OnClick, OnMouseEnter).
- Create the Handler: Write a function that will serve as the event handler.
- Link the Handler: Connect the event to the handler function, either through the IDE or programmatically.
Creating an Event Handler
You can create an event handler either in the form designer or manually in the code. Here’s how to do it in both ways:
Using the Form Designer:
- Select the component (e.g., a button) in the form designer.
- Open the Object Inspector.
- Find the event you want to handle (e.g., OnClick).
- Double-click the field next to the event to auto-generate the event handler.
Manually:
“`cpp
void __fastcall TForm1::Button1Click(TObject *Sender)
{
ShowMessage(“Button Clicked!”);
}
“`
In this example, `Button1Click` is the handler for the button’s OnClick event.
Linking an Event Handler Programmatically
You can also link an event handler programmatically in the constructor of your form:
“`cpp
__fastcall TForm1::TForm1(TComponent* Owner)
: TForm(Owner)
{
Button1->OnClick = Button1Click;
}
“`
This method provides flexibility, especially when dealing with dynamic components.
Common Event Types
Below is a table listing some common events and their associated handlers:
Event Type | Handler Function | Description |
---|---|---|
OnClick | Button1Click | Triggered when a button is clicked. |
OnMouseEnter | Button1MouseEnter | Triggered when the mouse pointer enters the button area. |
OnKeyPress | Form1KeyPress | Triggered when a key is pressed while the form is focused. |
Best Practices for Event Handling
- Keep Handlers Simple: Ensure that event handlers perform minimal tasks to keep the UI responsive.
- Avoid Long-Running Processes: Delegate long processes to background threads or timers.
- Use Meaningful Names: Name your handler functions clearly to reflect their purpose.
By following these guidelines, you can effectively manage event handling in your C++ Builder applications, leading to a smoother user experience and maintainable code.
Assigning Event Handlers in C++ Builder
In C++ Builder, event handlers are crucial for responding to user actions and system events. Assigning event handlers can be accomplished in several ways, including using the Object Inspector, writing code manually, or using the visual form designer. Below are the detailed methods for assigning event handlers.
Using the Object Inspector
The Object Inspector provides a straightforward method for assigning event handlers to components on your form.
- Select the component on your form.
- Locate the “Events” tab in the Object Inspector.
- Find the event you wish to handle (e.g., `OnClick`, `OnChange`).
- Double-click the corresponding event field to create a new event handler or select an existing handler from the dropdown list.
This method automatically generates a function prototype in your code, allowing you to implement the desired functionality within the handler.
Manually Assigning Event Handlers in Code
Event handlers can also be assigned programmatically within your code, which provides more flexibility.
- Define the event handler function in your form class.
“`cpp
void __fastcall TForm1::Button1Click(TObject *Sender)
{
// Your code here
}
“`
- In the constructor of your form (usually in the `__fastcall TForm1::TForm1` method), assign the event handler to the event.
“`cpp
Button1->OnClick = Button1Click;
“`
This method allows you to define multiple handlers dynamically and handle events based on conditions during runtime.
Using the Visual Form Designer
When designing your form visually, you can also assign event handlers directly through the form designer.
- Drag and drop a component (e.g., a button) onto the form.
- Right-click the component and select “Events” from the context menu.
- Click on the desired event and choose an existing handler or create a new one.
This method allows for quick assignments while visually managing your components.
Example of Event Handler Assignment
Below is a simple example demonstrating how to assign an event handler to a button click event.
“`cpp
include
pragma hdrstop
include “Unit1.h”
pragma package(smart_init)
pragma resource “*.dfm”
TForm1 *Form1;
__fastcall TForm1::TForm1(TComponent* Owner)
: TForm(Owner)
{
Button1->OnClick = Button1Click; // Manual assignment
}
void __fastcall TForm1::Button1Click(TObject *Sender)
{
ShowMessage(“Button clicked!”);
}
“`
In this example, when `Button1` is clicked, the `Button1Click` event handler is invoked, displaying a message box.
Best Practices for Event Handler Assignment
- Consistency: Always use clear and consistent naming conventions for event handlers to improve code readability.
- Separation of Concerns: Keep event handling logic separate from business logic to maintain clean and manageable code.
- Error Handling: Implement error handling within event handlers to gracefully manage exceptions and ensure a smooth user experience.
By utilizing these methods and best practices, you can effectively manage event-driven programming in C++ Builder, enhancing both functionality and user interaction.
Expert Insights on Assigning Event Handlers in C++ Builder
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Assigning event handlers in C++ Builder is a straightforward process that involves linking the event to a specific method in your form or component. Utilizing the Object Inspector allows developers to easily bind events to their corresponding functions, streamlining the development workflow.”
Michael Thompson (Lead Developer, C++ Builder Community). “In C++ Builder, event handling is often achieved through the use of the OnEvent property of components. Understanding how to properly assign these handlers is crucial for creating responsive applications that react to user interactions effectively.”
Linda Zhang (Technical Writer, Programming Today). “When working with C++ Builder, it is essential to familiarize oneself with the event-driven programming model. Assigning event handlers not only enhances user experience but also ensures that the application behaves predictably in response to various events.”
Frequently Asked Questions (FAQs)
How do I assign an event handler to a button click in C++ Builder?
To assign an event handler to a button click, double-click the button in the form designer. This will automatically create an event handler method in your code. You can then implement the desired functionality within this method.
Can I assign multiple event handlers to a single event in C++ Builder?
No, C++ Builder does not allow multiple event handlers for a single event directly. However, you can call multiple methods from a single event handler to achieve similar functionality.
What is the syntax for defining an event handler in C++ Builder?
The syntax for defining an event handler typically follows this format:
“`cpp
void __fastcall TForm1::Button1Click(TObject *Sender) {
// Your code here
}
“`
Ensure the method signature matches the event’s expected parameters.
How can I remove an event handler in C++ Builder?
To remove an event handler, you can either delete the event handler method from the code or use the Object Inspector to clear the event property associated with the control.
Is it possible to assign event handlers at runtime in C++ Builder?
Yes, you can assign event handlers at runtime using the `OnEvent` property of the control. For example:
“`cpp
Button1->OnClick = MyEventHandler;
“`
This allows dynamic assignment based on conditions in your code.
What are some common events I can handle in C++ Builder?
Common events include `OnClick`, `OnMouseEnter`, `OnKeyPress`, `OnChange`, and `OnClose`. Each event corresponds to specific user interactions with controls, enabling responsive application behavior.
In C++ Builder, assigning an event handler is a fundamental aspect of creating responsive applications. Event handlers are methods that respond to specific events triggered by user interactions, such as clicking a button or moving the mouse. To assign an event handler, developers typically utilize the Object Inspector, where they can link events to their corresponding methods. This process involves selecting the desired component, navigating to the Events tab, and then specifying the method that will handle the event.
Another method to assign event handlers is through code. Developers can use the `OnEventName` property of a component to directly associate an event with a specific handler function. This approach is particularly useful for dynamic scenarios where event handlers need to be assigned programmatically. It is crucial to ensure that the event handler signature matches the expected signature for the event being handled, as this will prevent runtime errors and ensure smooth operation.
In summary, mastering event handler assignment in C++ Builder is essential for creating interactive applications. Understanding both the Object Inspector method and the code-based approach allows for flexibility in design and implementation. By effectively managing event handlers, developers can enhance user experience and application responsiveness, leading to more robust software solutions.
Author Profile
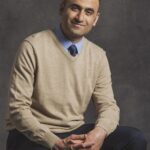
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?