Can WordPress Automatically Deactivate a Plugin if Another Plugin is Deactivated?
In the dynamic world of WordPress, plugins are the lifeblood of customization and functionality. However, managing multiple plugins can sometimes lead to conflicts or compatibility issues, especially when one plugin relies on another to function correctly. Imagine a scenario where deactivating a single plugin inadvertently disrupts the entire ecosystem of your website. This is where the concept of automatically deactivating related plugins comes into play—a feature that can save webmasters from the headaches of troubleshooting and ensure a seamless user experience.
When working with WordPress, it’s crucial to understand the intricate relationships between plugins. Some plugins are designed to complement each other, while others might create conflicts that hinder performance. The ability to automatically deactivate a plugin when another is turned off can streamline site management, preventing potential errors and maintaining optimal functionality. This feature not only enhances the user experience but also simplifies the maintenance process for site administrators.
As we delve deeper into the mechanics of this functionality, we will explore how developers can implement such a system, the benefits it offers, and best practices for ensuring that your website remains robust and reliable. Whether you’re a seasoned WordPress developer or a site owner looking to enhance your platform’s performance, understanding how to manage plugin dependencies effectively is essential for a thriving online presence.
Understanding Plugin Dependencies
When developing WordPress plugins, it’s crucial to consider dependencies between plugins. A plugin may rely on another to function correctly. If the dependent plugin is deactivated, it may lead to errors or unexpected behavior. To manage these dependencies effectively, you can programmatically deactivate a plugin if its required counterpart is turned off.
Implementing Automatic Deactivation
You can automate the deactivation of a plugin using hooks provided by WordPress. The `deactivate_plugins` function can be utilized in combination with the `plugins_loaded` action to check the status of dependencies when a plugin is deactivated. Below is an example code snippet:
“`php
add_action(‘plugins_loaded’, ‘check_plugin_dependencies’);
function check_plugin_dependencies() {
// Specify the plugin that must be active
$required_plugin = ‘example-required-plugin/example-required-plugin.php’;
// Check if the required plugin is deactivated
if (!is_plugin_active($required_plugin)) {
// Deactivate the current plugin
deactivate_plugins(plugin_basename(__FILE__));
}
}
“`
This code checks if the required plugin is active; if not, it deactivates the current plugin.
Managing Dependencies with a Table
To provide a clear overview of plugin dependencies, consider using a table to illustrate which plugins rely on others. Below is an example format you might use:
Plugin Name | Dependent On |
---|---|
My Custom Plugin | Example Required Plugin |
Another Plugin | My Custom Plugin |
This table helps users understand the relationships between plugins, making it easier to manage and troubleshoot.
Best Practices for Managing Plugin Dependencies
When handling plugin dependencies, consider the following best practices:
- Documentation: Clearly document any dependencies in your plugin’s readme file.
- User Notifications: Provide users with clear notifications when a plugin is deactivated due to missing dependencies.
- Graceful Degradation: Ensure your plugin can handle the absence of dependencies gracefully, providing fallback functionality or notifications instead of breaking completely.
- Testing: Rigorously test your plugin in environments with various combinations of active and inactive plugins to ensure smooth operation.
By adhering to these practices, you can enhance the user experience and maintain the integrity of your plugins.
Understanding Plugin Dependencies in WordPress
In WordPress, some plugins may rely on others to function correctly. When a required plugin is deactivated, it’s crucial to ensure that dependent plugins either notify the user or deactivate automatically to prevent conflicts and issues.
Implementing Automatic Deactivation
To implement automatic deactivation of a plugin when another plugin is deactivated, developers can use the `register_deactivation_hook()` function in the WordPress plugin API. This function allows developers to specify actions that should occur when a plugin is deactivated.
Steps to Automatically Deactivate a Plugin
- Identify Dependencies: Determine which plugins need to be monitored for deactivation.
- Code Implementation:
- Use `add_action()` to hook into the deactivation process of the primary plugin.
- Check if the dependent plugin is active and deactivate it if necessary.
“`php
// Example code snippet
function my_plugin_deactivation() {
if ( is_plugin_active( ‘dependent-plugin/dependent-plugin.php’ ) ) {
deactivate_plugins( ‘dependent-plugin/dependent-plugin.php’ );
}
}
register_deactivation_hook( __FILE__, ‘my_plugin_deactivation’ );
“`
- Testing: After implementing the code, thoroughly test the plugins to ensure they interact correctly and that the dependent plugin deactivates as intended.
Considerations for Plugin Development
When developing plugins that depend on others, consider the following:
- User Experience: Automatically deactivating plugins can confuse users. Consider providing notifications or instructions.
- Error Handling: Implement error handling to manage scenarios where the primary plugin fails to deactivate.
- Performance: Ensure that the checks for plugin status do not significantly impact performance.
Best Practices for Managing Plugin Dependencies
- Documentation: Clearly document any dependencies in the plugin’s description and readme files.
- Version Control: Use version checks to ensure compatibility between plugins.
- Regular Updates: Maintain and update plugins regularly to address dependency issues as WordPress evolves.
Tools and Resources
Resource | Description |
---|---|
WordPress Plugin Handbook | Comprehensive guide on plugin development practices. |
Plugin Dependencies Checker | A tool to check for plugin compatibility and dependencies. |
WP_DEBUG | Enable debug mode in WordPress to troubleshoot issues related to plugins. |
By adhering to these guidelines, WordPress developers can create robust plugins that manage dependencies effectively, enhancing the overall user experience and functionality of their sites.
Expert Insights on Automatic Plugin Deactivation in WordPress
Dr. Emily Carter (WordPress Security Analyst, WebGuard Solutions). “Automatic deactivation of plugins in WordPress upon the deactivation of another plugin can significantly enhance site security. It prevents potential conflicts and vulnerabilities that could arise from incompatible plugins, ensuring a smoother user experience and maintaining the integrity of the website.”
Mark Thompson (Senior WordPress Developer, CodeCrafters). “Implementing a system where one plugin automatically deactivates another can streamline site management. This feature can be particularly useful for developers who need to ensure that dependencies are respected, thus reducing the risk of site errors and improving overall performance.”
Lisa Wang (Digital Marketing Specialist, SEO Innovations). “From a marketing perspective, ensuring that only compatible plugins are active is crucial. Automatically deactivating plugins can help maintain site speed and functionality, which are essential for user engagement and SEO rankings.”
Frequently Asked Questions (FAQs)
Can a WordPress plugin automatically deactivate another plugin?
Yes, a WordPress plugin can be programmed to automatically deactivate another plugin if certain conditions are met, such as dependencies or conflicts.
How can I set up a plugin to deactivate another plugin in WordPress?
You can use the `deactivate_plugins()` function within your plugin’s code. This function allows you to specify the plugin you want to deactivate based on your criteria.
Are there any risks associated with automatically deactivating plugins?
Yes, automatically deactivating plugins can lead to unexpected behavior on your site, such as loss of functionality or broken features, especially if the deactivated plugin is critical for your site’s operation.
What are common scenarios for deactivating a plugin when another is deactivated?
Common scenarios include resolving plugin conflicts, managing dependencies, or ensuring compatibility with updates or changes in the WordPress environment.
Is there a way to notify users when a plugin is automatically deactivated?
Yes, you can implement a notification system using WordPress hooks and functions like `add_action()` to inform users via admin notices when a plugin is deactivated automatically.
Can I prevent automatic deactivation of plugins in WordPress?
While you cannot entirely prevent automatic deactivation from occurring due to plugin conflicts, you can manage dependencies carefully and ensure that your plugins are compatible to minimize such occurrences.
In the context of WordPress, the automatic deactivation of a plugin when another plugin is deactivated is a critical aspect of maintaining site functionality and integrity. This behavior is typically not a default feature of WordPress but can be implemented through custom coding or plugin development. It is essential for developers to understand how to manage plugin dependencies effectively to ensure that users do not encounter issues when a required plugin is disabled.
One of the primary insights from this discussion is the importance of establishing clear dependencies between plugins. Developers can use hooks and filters to create a system where the deactivation of one plugin triggers the deactivation of another. This practice not only enhances user experience by preventing potential conflicts but also ensures that the site remains stable and secure. Properly managing these dependencies can significantly reduce the risk of errors and improve overall site performance.
Additionally, it is vital for plugin developers to communicate these dependencies clearly to users. Documentation should specify which plugins are required for optimal functionality, and any automatic deactivation behavior should be well-documented. This transparency helps users make informed decisions about their plugin installations and configurations, ultimately leading to a more reliable WordPress environment.
Author Profile
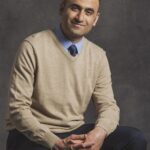
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?