Why Am I Seeing ‘Cannot Convert Float NaN to Integer’ and How Can I Fix It?
In the world of programming and data analysis, encountering errors is as common as the code itself. One such perplexing error that often leaves developers scratching their heads is the infamous “cannot convert float nan to integer.” This message serves as a reminder of the intricacies involved in managing data types, particularly when dealing with numerical computations. As data scientists and programmers strive to make sense of vast datasets, understanding the nuances of floating-point numbers and their behavior becomes crucial.
At its core, the issue arises from the presence of NaN, or “Not a Number,” a special floating-point value that signifies or unrepresentable numerical results. This can occur for various reasons, such as division by zero, invalid operations, or missing data points. When an attempt is made to convert a NaN value into an integer, the programming language throws an error, highlighting the importance of data integrity and type compatibility in coding practices.
Navigating this error requires a blend of technical knowledge and problem-solving skills. Developers must learn to identify the sources of NaN values within their datasets and implement strategies to handle them effectively. Whether through data cleaning, imputation, or conditional checks, addressing the root causes of NaN occurrences is essential for smooth program execution and accurate data analysis. In the following sections
Understanding NaN in Python
NaN, which stands for “Not a Number,” is a floating-point value that represents or unrepresentable numerical results. It is a standard in computing to indicate missing or invalid data, particularly in numerical computations. In Python, NaN is often encountered in libraries such as NumPy or Pandas when dealing with datasets that may contain missing values.
- NaN can arise from:
- Division by zero
- Operations with null values
- Invalid mathematical operations
When attempting to convert a NaN value to an integer type, Python raises a `ValueError`, indicating that it cannot perform this conversion. This limitation arises because integers cannot represent or missing values.
Common Scenarios Leading to NaN
NaN values can emerge in various scenarios, particularly when processing datasets:
- Data Import: When importing datasets from CSV or Excel files, missing entries may be represented as NaN.
- Calculations: Operations that yield results (like `0/0`) will result in NaN.
- Filtering and Transformations: Data manipulations that exclude valid data points can inadvertently lead to NaN.
Here is a table summarizing some common sources of NaN values:
Source of NaN | Example | Resulting Action |
---|---|---|
Division by Zero | 0/0 | Returns NaN |
Missing Data in Datasets | CSV with empty fields | Imported as NaN |
Invalid Mathematical Operations | sqrt(-1) | Returns NaN |
Handling NaN Values
To manage NaN values effectively, various strategies can be employed in Python:
- Removing NaN Values: Use the `dropna()` method in Pandas to eliminate rows with NaN.
- Replacing NaN Values: Utilize the `fillna()` method to substitute NaN with a specific value, such as the mean or median of the dataset.
- Checking for NaN: Use `isna()` or `isnull()` to identify NaN values within a DataFrame or array.
For example, the following code illustrates how to replace NaN values with the mean of a column in a Pandas DataFrame:
“`python
import pandas as pd
data = {‘numbers’: [1, 2, float(‘nan’), 4]}
df = pd.DataFrame(data)
df[‘numbers’].fillna(df[‘numbers’].mean(), inplace=True)
“`
These techniques can help maintain data integrity and prevent conversion errors when handling datasets with NaN values.
Best Practices for Data Validation
To avoid encountering NaN-related conversion errors, consider the following best practices:
- Data Cleaning: Always clean your data before performing numerical operations. This includes checking for NaN values and handling them appropriately.
- Type Checking: Verify the data types of your variables before conversion. Use `isinstance()` to ensure that the data is numeric and does not contain NaN.
- Error Handling: Implement try-except blocks to gracefully handle exceptions that may arise from invalid conversions.
By adhering to these practices, you can effectively manage NaN values and avoid conversion issues in your numerical computations.
Understanding NaN in Floating-Point Arithmetic
In the context of floating-point arithmetic, NaN stands for “Not a Number.” It represents an or unrepresentable value, particularly in calculations. Common sources of NaN include:
- Division by zero
- Invalid operations, such as taking the square root of a negative number
- Missing or uninitialized data
When NaN is present in a dataset, it can complicate data processing and analysis, especially when attempting to convert these values into an integer format.
Causes of the Error: “cannot convert float NaN to integer”
This error occurs in programming environments when a function attempts to convert a float that is NaN into an integer. Key scenarios leading to this error include:
- Data Processing: Handling datasets with missing or null values.
- Mathematical Operations: Performing calculations that result in NaN.
- Type Casting: Using functions like `int()` or `astype(int)` in Python with NaN values.
Handling NaN Values
To avoid the “cannot convert float NaN to integer” error, it is essential to handle NaN values appropriately. Here are several strategies:
- Removing NaN Values: Use functions to drop or filter out NaN entries from the dataset.
- In Python with Pandas:
“`python
df.dropna(inplace=True)
“`
- Filling NaN Values: Replace NaN values with a specific number or statistic, such as the mean or median of the column.
- Example in Pandas:
“`python
df.fillna(value=0, inplace=True)
“`
- Checking for NaN Before Conversion: Before converting, check if any NaN values exist.
- Example code snippet:
“`python
if df[‘column’].isnull().any():
Handle NaN values
“`
Practical Examples
Consider the following scenarios in Python to illustrate how to manage NaN values:
Example 1: Converting without Handling NaN
“`python
import numpy as np
array = np.array([1.0, 2.0, np.nan])
int_array = array.astype(int) Raises an error
“`
Example 2: Handling NaN by Filling
“`python
import numpy as np
array = np.array([1.0, 2.0, np.nan])
filled_array = np.nan_to_num(array) Replaces NaN with 0.0
int_array = filled_array.astype(int) Converts successfully
“`
Best Practices
To effectively manage NaN values and prevent conversion errors, consider the following best practices:
- Data Validation: Regularly check and clean your data for NaN values before processing.
- Use Libraries: Utilize libraries like NumPy and Pandas, which provide built-in methods for handling NaN values efficiently.
- Error Handling: Implement try-except blocks to gracefully handle exceptions related to NaN conversions.
Conclusion on NaN Handling
Mastering the management of NaN values is crucial for data integrity and processing efficiency. By following established methods and practices, you can effectively mitigate the risks associated with converting NaN values to integers, ensuring smooth data workflows and analysis.
Understanding the Error: Cannot Convert Float NaN to Integer
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “The error ‘cannot convert float nan to integer’ typically arises when a dataset contains NaN (Not a Number) values. These NaN values can occur due to missing data or invalid computations. It is crucial to preprocess the data to handle these NaNs, either by removing them or imputing appropriate values before attempting any conversions.”
Michael Chen (Software Engineer, Data Solutions Corp.). “When dealing with numerical data in programming, particularly with languages like Python, encountering NaN values can lead to unexpected errors during type conversion. It is advisable to implement checks that identify and address NaN values prior to any integer conversion to ensure data integrity and prevent runtime errors.”
Lisa Patel (Machine Learning Researcher, AI Analytics Group). “In machine learning workflows, NaN values can significantly hinder model training and evaluation. To avoid the ‘cannot convert float nan to integer’ error, practitioners should incorporate robust data validation steps that detect and handle NaN values, thereby ensuring that the data fed into algorithms is clean and usable.”
Frequently Asked Questions (FAQs)
What does “cannot convert float nan to integer” mean?
This error indicates that a program is attempting to convert a floating-point number that is ‘NaN’ (Not a Number) into an integer. Since ‘NaN’ is not a valid numeric value, the conversion fails.
What causes a float to be NaN?
A float can be NaN due to various reasons, including mathematical operations (like 0/0), invalid calculations, or when data is missing or corrupted in datasets.
How can I check for NaN values in my data?
You can use functions such as `isna()` or `isnull()` in libraries like Pandas to identify NaN values in your datasets. These functions return a boolean array indicating the presence of NaN values.
What should I do if I encounter this error in my code?
To resolve this error, you should first identify and handle the NaN values in your data. You can either remove these entries, replace them with a default value, or use imputation techniques to fill in the missing data.
Are there any specific libraries that handle NaN values effectively?
Yes, libraries like NumPy and Pandas in Python provide robust methods for dealing with NaN values, including functions for filtering, filling, or dropping NaN entries from datasets.
Can NaN values affect the performance of my machine learning model?
Yes, NaN values can significantly impact the performance of machine learning models. They can lead to errors during training and evaluation, and may also skew the results if not handled appropriately.
The error message “cannot convert float nan to integer” typically arises in programming contexts, particularly when working with data types in languages such as Python. This error indicates that an operation is attempting to convert a floating-point number that is ‘NaN’ (Not a Number) into an integer type, which is not permissible. The presence of NaN usually signifies that the data being processed is either missing, , or the result of an invalid mathematical operation, such as division by zero.
To effectively address this issue, it is essential to first identify the source of the NaN values within the dataset. Common strategies include checking for missing data, validating input values, and implementing data cleaning techniques. Additionally, utilizing functions that can handle NaN values, such as `numpy.nan_to_num()` or `pandas.fillna()`, can help mitigate the occurrence of this error by replacing NaN values with a specified number or removing them entirely from the dataset.
Furthermore, incorporating error handling mechanisms, such as try-except blocks in Python, can provide a more robust solution by allowing the program to manage exceptions gracefully. This approach not only prevents abrupt termination of the program but also facilitates better debugging and data integrity checks. Overall, understanding the nature of
Author Profile
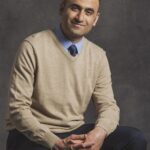
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?