How Can I Resolve the Error: ‘Cannot Convert java.lang.String to JSON Object’ in Kotlin?
In the world of software development, the seamless integration of data formats is crucial for building robust applications. One common challenge that developers face is the conversion of data types, particularly when working with JSON in Kotlin. If you’ve ever encountered the error message “cannot convert java.lang.String to JSON object,” you know how frustrating it can be to navigate the intricacies of data handling in a language that prides itself on its modernity and efficiency. This article delves into the nuances of this issue, exploring the underlying causes, potential pitfalls, and effective solutions to ensure smooth data manipulation in your Kotlin applications.
When working with JSON in Kotlin, developers often rely on libraries such as Gson or Moshi to facilitate the conversion between JSON strings and Kotlin objects. However, the error in question typically arises when the expected data type does not align with the actual data being processed. Understanding the root of this discrepancy is essential for troubleshooting and resolving the issue effectively.
Moreover, this article will guide you through best practices for handling JSON data in Kotlin, including tips on validating JSON structures and ensuring type safety. By the end, you will be equipped with the knowledge to tackle the “cannot convert java.lang.String to JSON object” error confidently and enhance your overall programming experience. Whether you’re a seasoned developer
Understanding the Error
The error message “cannot convert java.lang.String to JSONObject” typically arises when attempting to parse a JSON string in Kotlin using the `JSONObject` class from the Android SDK or similar libraries. This issue often indicates that the string being provided does not conform to the expected JSON format, or it may be improperly constructed or escaped.
When working with JSON in Kotlin, ensure that:
- The string is valid JSON.
- Any quotes or special characters are properly escaped.
- The appropriate libraries are being used for parsing.
Common Causes
Several factors can contribute to this error, including:
- Invalid JSON Format: The string might have syntax issues, such as missing brackets or misplaced commas.
- Improper String Handling: If the string is fetched from a source (like an API) and not correctly processed, it may not be in a valid JSON format.
- Character Encoding Issues: Sometimes, strings retrieved from external sources may have encoding problems that affect their structure.
Debugging the Issue
To resolve this error, follow these debugging steps:
- Check JSON Syntax: Use a JSON validator tool to verify that the string is correctly formatted.
- Log the String: Print the string before parsing to ensure you are working with the expected data.
- Handle Exceptions: Utilize try-catch blocks to catch parsing errors and log them for further analysis.
For example:
“`kotlin
try {
val jsonObject = JSONObject(jsonString)
} catch (e: JSONException) {
Log.e(“JSON Error”, “Parsing error: ${e.message}”)
}
“`
Example of Parsing JSON
Here is a simple example of how to convert a JSON string into a `JSONObject` in Kotlin:
“`kotlin
val jsonString = “””{“name”: “John”, “age”: 30}”””
try {
val jsonObject = JSONObject(jsonString)
val name = jsonObject.getString(“name”)
val age = jsonObject.getInt(“age”)
println(“Name: $name, Age: $age”)
} catch (e: JSONException) {
e.printStackTrace()
}
“`
This example correctly parses a well-formed JSON string, demonstrating how to safely handle potential exceptions.
Best Practices
To minimize the chances of encountering this error, consider the following best practices:
- Use Kotlin Serialization: Utilize Kotlin’s serialization libraries to handle JSON parsing more safely and efficiently.
- Validate Input: Always validate external input data before attempting to parse it.
- Use Data Classes: Leverage Kotlin data classes to map JSON structures directly, enhancing readability and maintainability.
Best Practice | Description |
---|---|
Kotlin Serialization | Utilize libraries like kotlinx.serialization for robust JSON handling. |
Input Validation | Ensure that incoming data is checked for validity before parsing. |
Data Classes | Map JSON data to Kotlin data classes for better structure. |
Understanding the Error
The error “cannot convert java.lang.String to JSONObject” typically occurs in Kotlin when trying to parse a JSON string into a JSONObject without proper conversion. This suggests that the string being processed is not being recognized as a valid JSON format.
Common Causes
Several factors can lead to this error:
- Invalid JSON Format: The string may not be properly formatted as JSON, which can occur if it is missing brackets, quotes, or other structural components.
- Incorrect Type: Attempting to pass a plain string that does not represent JSON to a function expecting a JSONObject.
- Library Misuse: Using a JSON library incorrectly, such as calling a method that expects a JSONObject while providing a string instead.
How to Resolve the Issue
To fix the error, you can follow these steps:
- Validate JSON String: Ensure that the string you are trying to convert is a valid JSON. You can use online JSON validators or formatters to check the string.
- Use Proper Conversion Methods: If you are using libraries like `org.json`, ensure you are using the correct constructors. For example, to convert a JSON string to a JSONObject, you should do the following:
“`kotlin
val jsonString = “{\”key\”:\”value\”}” // Example of a valid JSON string
val jsonObject = JSONObject(jsonString)
“`
- Debugging Output: Print the string before conversion to confirm its content:
“`kotlin
println(“JSON String: $jsonString”)
“`
- Error Handling: Implement error handling to catch potential exceptions during the conversion:
“`kotlin
try {
val jsonObject = JSONObject(jsonString)
} catch (e: JSONException) {
e.printStackTrace() // Handle the error
}
“`
Example Code Snippet
Here is a complete example that demonstrates the correct conversion from a JSON string to a JSONObject in Kotlin:
“`kotlin
import org.json.JSONObject
import org.json.JSONException
fun main() {
val jsonString = “{\”name\”:\”John\”, \”age\”:30}” // Example JSON string
try {
val jsonObject = JSONObject(jsonString)
println(“JSONObject: $jsonObject”)
} catch (e: JSONException) {
println(“Error converting string to JSONObject: ${e.message}”)
}
}
“`
Best Practices
To avoid similar issues in the future, consider the following best practices:
- Always Validate Input: Ensure that the input string is valid JSON before attempting conversion.
- Use JSON Libraries: Rely on robust libraries such as `Gson` or `Moshi`, which provide more straightforward handling of JSON serialization and deserialization.
- Consistent Error Handling: Implement consistent error handling across your application to manage exceptions effectively.
Alternative Libraries
If you continue to face issues with JSON parsing in Kotlin, consider using alternative libraries:
Library | Description |
---|---|
Gson | A popular library for converting Java Objects into JSON and back. |
Moshi | A modern JSON library for Android and Java that makes it easy to parse JSON. |
Klaxon | A lightweight JSON parser for Kotlin with a simple API. |
Each of these libraries has its own strengths and may suit different project requirements better than the standard JSONObject approach.
Understanding JSON Conversion Issues in Kotlin
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error ‘cannot convert java.lang.string to json object’ typically arises when a string is not properly formatted as JSON. In Kotlin, it’s crucial to ensure that the string you are trying to convert adheres to JSON syntax. Using libraries like Gson or Moshi can help streamline this process by providing robust parsing capabilities.”
Michael Chen (Lead Kotlin Developer, App Solutions Ltd.). “When encountering this error, developers should first validate the string content. Often, the issue lies in unexpected characters or incorrect structure. Utilizing tools like JSONLint can assist in verifying the string format before attempting conversion in Kotlin.”
Sarah Thompson (Mobile Application Architect, Future Tech Group). “In Kotlin, it is essential to handle JSON conversion with care, especially when dealing with external data sources. Implementing error handling and logging can provide insights into what the string contains at runtime, allowing developers to diagnose the conversion issue effectively.”
Frequently Asked Questions (FAQs)
What does the error “cannot convert java.lang.String to JSONObject” mean in Kotlin?
This error indicates that the code is attempting to convert a plain Java String into a JSON object without proper parsing. A String must be formatted as valid JSON before it can be converted.
How can I convert a String to a JSONObject in Kotlin?
To convert a String to a JSONObject, ensure the String is a valid JSON format. Use the `JSONObject` constructor: `val jsonObject = JSONObject(yourString)`.
What are common reasons for encountering this error?
Common reasons include invalid JSON format, missing quotes, or extra characters in the String that prevent it from being parsed as JSON.
How can I validate a JSON String before conversion in Kotlin?
You can validate a JSON String by using a try-catch block when creating a JSONObject. If the String is invalid, a JSONException will be thrown, indicating the issue.
Are there alternatives to using JSONObject in Kotlin?
Yes, you can use libraries like Gson or Moshi, which provide more flexibility and ease of use for JSON parsing and serialization in Kotlin.
What should I do if I need to handle nested JSON objects?
For nested JSON objects, you can create multiple JSONObject instances or use libraries that support nested structures, allowing for easier manipulation and access to data.
The error message “cannot convert java.lang.String to JSONObject” in Kotlin typically arises when attempting to parse a string that is not in the correct JSON format. This often occurs when the input string is malformed or does not conform to the expected JSON structure, leading to failures in conversion. It is crucial to ensure that the string being parsed is a valid JSON representation, which includes proper formatting, such as the use of double quotes for keys and values, and correct nesting of objects and arrays.
To resolve this issue, developers should first validate the string to confirm it is well-formed JSON. Tools such as JSON validators can be used to check the syntax of the string. Additionally, utilizing libraries like Gson or Moshi can simplify the process of converting JSON strings to Kotlin objects, as these libraries provide robust error handling and parsing capabilities. It is also beneficial to implement exception handling in the code to gracefully manage any parsing errors that may occur.
understanding the structure of JSON and ensuring that the input string adheres to its specifications are vital steps in avoiding the “cannot convert java.lang.String to JSONObject” error in Kotlin. By validating the JSON format and leveraging appropriate libraries, developers can effectively handle JSON parsing and enhance the reliability of their applications
Author Profile
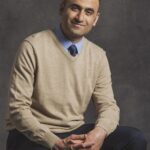
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?