How Can You Create a Pipeline in PyTorch Similar to scikit-learn?
In the rapidly evolving landscape of machine learning, the need for efficient and streamlined workflows has never been more critical. For practitioners accustomed to the elegant simplicity of scikit-learn’s pipeline functionality, transitioning to more complex frameworks like PyTorch can feel daunting. However, the flexibility and power of PyTorch offer unique advantages, enabling developers to construct sophisticated models that can be seamlessly integrated into their data processing workflows. In this article, we will explore how to create a pipeline in PyTorch that mirrors the intuitive design of scikit-learn, empowering you to harness the full potential of deep learning while maintaining the ease of use you expect from traditional machine learning libraries.
At its core, a pipeline in PyTorch serves as a structured approach to managing the various stages of a machine learning project, from data preprocessing to model training and evaluation. While scikit-learn provides a straightforward interface for chaining together transformers and estimators, PyTorch requires a more hands-on approach. This article will delve into the methods and best practices for building a cohesive pipeline that allows for modularity and reusability, ensuring that each component of your workflow can be easily modified or replaced as needed.
By leveraging PyTorch’s capabilities, you can implement custom data loaders, define complex neural network architectures, and optimize training processes—all
Building a Pipeline in PyTorch
Creating a pipeline in PyTorch can be structured similarly to the pipeline functionality in scikit-learn, although the implementation details differ due to the underlying design principles of each library. In PyTorch, you can encapsulate data preprocessing, model training, and evaluation in a cohesive manner, making the code modular and reusable.
To facilitate a pipeline structure in PyTorch, you can utilize classes to represent various components such as data loaders, models, and training routines. Here’s an outline of how to implement a basic pipeline:
- Data Preprocessing: This includes loading your dataset and applying transformations.
- Model Definition: Define your neural network architecture.
- Training Loop: Implement the logic for training the model.
- Evaluation: Assess the model’s performance on the validation/test set.
Example Implementation
Here is a simplified example of how to create a pipeline in PyTorch:
“`python
import torch
import torch.nn as nn
import torch.optim as optim
from torch.utils.data import DataLoader, Dataset
Sample Dataset
class CustomDataset(Dataset):
def __init__(self, data, targets):
self.data = data
self.targets = targets
def __len__(self):
return len(self.data)
def __getitem__(self, idx):
return self.data[idx], self.targets[idx]
Sample Model
class SimpleModel(nn.Module):
def __init__(self):
super(SimpleModel, self).__init__()
self.fc = nn.Linear(10, 1) Example for input size of 10
def forward(self, x):
return self.fc(x)
Training Function
def train_model(model, dataloader, criterion, optimizer, num_epochs):
model.train()
for epoch in range(num_epochs):
for inputs, labels in dataloader:
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
“`
In this example, we have defined a dataset, a simple neural network, and a training function. You would typically create instances of these components, pass your data through the pipeline, and execute the training loop.
Pipeline Structure
To visualize how these components fit together, consider the following table that outlines the steps involved in the pipeline:
Step | Description |
---|---|
1 | Data Loading: Load your dataset and create a DataLoader for batching. |
2 | Data Preprocessing: Apply any necessary transformations or augmentations. |
3 | Model Initialization: Instantiate the model architecture. |
4 | Define Loss Function: Choose an appropriate loss function for your task. |
5 | Optimizer Setup: Initialize an optimizer for model parameters. |
6 | Training Loop: Iterate through epochs and batches to train the model. |
7 | Evaluation: Test the model on unseen data and log metrics. |
This pipeline structure allows for flexibility and clarity in managing different stages of the machine learning workflow, similar to what is offered in scikit-learn but tailored to the dynamic and computational nature of deep learning with PyTorch.
Pipelines in PyTorch
In PyTorch, while there is no direct equivalent to the Scikit-learn pipeline, you can create similar functionality by organizing your data processing and model training steps into cohesive modules. This can be achieved using a combination of custom classes and existing PyTorch utilities.
Creating a PyTorch Pipeline
To create a pipeline in PyTorch, you generally follow these steps:
- Define a Data Preprocessing Class: This class will handle data transformations, such as normalization, augmentation, and batching.
- Create a Model Class: The model class encapsulates the architecture of your neural network.
- Combine into a Training Loop: The training loop will integrate the preprocessing and model classes, allowing for streamlined training and evaluation.
Example Implementation
Below is an example demonstrating how to implement a simple pipeline in PyTorch.
“`python
import torch
import torch.nn as nn
import torch.optim as optim
from torchvision import transforms, datasets
from torch.utils.data import DataLoader
Data Preprocessing Class
class DataPreprocessor:
def __init__(self, batch_size):
self.transform = transforms.Compose([
transforms.ToTensor(),
transforms.Normalize((0.5,), (0.5,))
])
self.batch_size = batch_size
def load_data(self, dataset_path):
dataset = datasets.MNIST(root=dataset_path, train=True, transform=self.transform, download=True)
return DataLoader(dataset, batch_size=self.batch_size, shuffle=True)
Model Class
class SimpleNN(nn.Module):
def __init__(self):
super(SimpleNN, self).__init__()
self.fc1 = nn.Linear(28 * 28, 128)
self.fc2 = nn.Linear(128, 10)
def forward(self, x):
x = x.view(-1, 28 * 28)
x = torch.relu(self.fc1(x))
return self.fc2(x)
Training Loop
def train_model(data_loader, model, criterion, optimizer, num_epochs):
model.train()
for epoch in range(num_epochs):
for images, labels in data_loader:
optimizer.zero_grad()
outputs = model(images)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
print(f’Epoch [{epoch+1}/{num_epochs}], Loss: {loss.item():.4f}’)
Usage
data_preprocessor = DataPreprocessor(batch_size=64)
data_loader = data_preprocessor.load_data(‘path/to/dataset’)
model = SimpleNN()
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
train_model(data_loader, model, criterion, optimizer, num_epochs=5)
“`
Benefits of a Custom Pipeline
By creating a custom pipeline in PyTorch, you gain several advantages:
- Flexibility: Tailor the pipeline to specific project requirements without the constraints of predefined structures.
- Modularity: Easier to modify or replace components, such as changing the model architecture or data transformations.
- Reusability: Components can be reused across different projects or experiments.
Considerations When Using Pipelines
When implementing a pipeline, keep in mind:
- Performance: Ensure that data loading and preprocessing do not become bottlenecks. Utilize PyTorch’s built-in functionalities to optimize data loading.
- Complexity: Overly complex pipelines may hinder debugging and testing. Aim for a balance between modularity and simplicity.
- Integration with Other Libraries: If you are using libraries like `torchvision` or `torchaudio`, ensure that your pipeline effectively incorporates their functionalities.
While PyTorch does not provide a built-in pipeline mechanism like Scikit-learn, by structuring your code into reusable classes and functions, you can achieve similar functionality tailored to your specific deep learning workflows.
Building a Pipeline in PyTorch Similar to Scikit-Learn
Dr. Emily Carter (Machine Learning Researcher, AI Innovations Lab). “While PyTorch does not have a built-in pipeline feature like Scikit-Learn, you can create custom pipelines by leveraging the `torch.nn.Module` class to encapsulate your model and preprocessing steps. This approach allows for modularity and reusability, similar to Scikit-Learn’s pipeline functionality.”
Mark Thompson (Data Scientist, Tech Solutions Inc.). “To implement a pipeline in PyTorch akin to Scikit-Learn, consider using the `sklearn.pipeline.Pipeline` class in conjunction with PyTorch models. This hybrid approach enables you to seamlessly integrate preprocessing steps from Scikit-Learn with the flexibility of PyTorch for model training.”
Dr. Sarah Kim (AI Engineer, Neural Networks Corp.). “Creating a pipeline in PyTorch can be achieved by defining a custom class that combines data loading, transformation, and model training. This allows for a structured approach to handling complex workflows, similar to Scikit-Learn, while taking full advantage of PyTorch’s dynamic computation graph.”
Frequently Asked Questions (FAQs)
What is a pipeline in PyTorch similar to that in scikit-learn?
A pipeline in PyTorch is a sequence of data processing and model training steps that can be streamlined to facilitate the machine learning workflow, similar to scikit-learn. It allows for the encapsulation of preprocessing, model training, and evaluation into a cohesive structure.
How can I create a pipeline in PyTorch?
To create a pipeline in PyTorch, you can use the `torch.nn.Module` class to define a custom model that includes layers for preprocessing and the neural network itself. You can also use libraries like `sklearn` for preprocessing steps and integrate them with PyTorch models.
Are there any libraries that provide pipeline functionality in PyTorch?
Yes, libraries such as `skorch` and `pytorch-ignite` offer functionalities that facilitate the creation of pipelines in PyTorch. They provide abstractions for training, validation, and testing, similar to scikit-learn’s pipeline.
Can I use scikit-learn transformers in a PyTorch pipeline?
Yes, you can use scikit-learn transformers within a PyTorch pipeline. You can preprocess your data with scikit-learn and then feed the transformed data into a PyTorch model for training and evaluation.
What are the benefits of using a pipeline in PyTorch?
Using a pipeline in PyTorch enhances code organization, improves reproducibility, and simplifies the process of hyperparameter tuning and model evaluation. It allows for easier experimentation and management of complex workflows.
Is it possible to save and load a PyTorch pipeline?
Yes, you can save and load a PyTorch pipeline by serializing the model and its components using `torch.save()` and `torch.load()`. This allows for easy restoration of the pipeline for future use or deployment.
In recent years, the demand for streamlined workflows in machine learning has led to the development of pipeline structures that facilitate model training and evaluation. While libraries like Scikit-learn have established a robust pipeline API that simplifies the process of chaining together preprocessing steps and model training, PyTorch has traditionally required more manual setup. However, with the of the `torch.utils.data` module and various utility libraries, users can now create a pipeline in PyTorch that mimics the functionality of Scikit-learn’s pipeline.
Key components of a PyTorch pipeline include data preprocessing, model definition, training, and evaluation. By leveraging classes such as `Dataset` and `DataLoader`, users can efficiently manage their data inputs. Additionally, custom transformations can be applied to data, similar to Scikit-learn’s transformers. The integration of these components allows for a modular approach, enabling users to easily swap out different preprocessing methods or models without significant code changes.
Furthermore, the use of libraries like `sklearn-pytorch` and `PyTorch Lightning` can enhance the pipeline experience in PyTorch. These libraries provide higher-level abstractions that simplify the training loop and allow for better organization of code. Consequently, users can focus more on experimentation and less
Author Profile
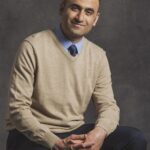
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?