How Can I Convert a Timestamp to Date in SQL?
In the world of data management and analysis, SQL (Structured Query Language) serves as the backbone for interacting with relational databases. One common task that developers and data analysts frequently encounter is the need to convert timestamps to dates. While timestamps provide precise information down to the second, there are times when only the date is required for reporting, analysis, or data presentation. Understanding how to effectively perform this conversion is essential for anyone working with SQL, as it not only streamlines data processing but also enhances the clarity and usability of the information at hand.
Converting timestamps to dates in SQL may seem straightforward, but it involves various functions and syntax that can vary across different database systems. Whether you’re using MySQL, PostgreSQL, SQL Server, or Oracle, each platform has its unique methods and functions for handling date and time data. This article will delve into the nuances of these conversions, providing you with the knowledge to manipulate and format your data accurately.
As you navigate through the intricacies of SQL date handling, you’ll discover the importance of understanding time zones, formatting options, and potential pitfalls that can arise during conversions. By mastering these techniques, you will not only enhance your SQL skills but also improve the quality of your data analysis, making your insights more impactful and actionable. Prepare to unlock
Converting Timestamps to Dates in SQL
To convert a timestamp to a date in SQL, various functions can be utilized depending on the SQL database being used. The method can differ between systems such as MySQL, PostgreSQL, Oracle, and SQL Server.
MySQL
In MySQL, you can convert a timestamp to a date using the `DATE()` function. This function extracts the date part from a datetime or timestamp expression.
“`sql
SELECT DATE(your_timestamp_column) AS date_only
FROM your_table;
“`
Alternatively, you can use the `CAST()` function:
“`sql
SELECT CAST(your_timestamp_column AS DATE) AS date_only
FROM your_table;
“`
PostgreSQL
In PostgreSQL, the `::date` cast or the `DATE()` function can be employed to achieve similar results.
“`sql
SELECT your_timestamp_column::date AS date_only
FROM your_table;
“`
Or using the `DATE()` function:
“`sql
SELECT DATE(your_timestamp_column) AS date_only
FROM your_table;
“`
SQL Server
For SQL Server, the `CONVERT()` function is typically used to convert a timestamp to a date. You can specify the style as part of the conversion.
“`sql
SELECT CONVERT(DATE, your_timestamp_column) AS date_only
FROM your_table;
“`
You can also use the `CAST()` function:
“`sql
SELECT CAST(your_timestamp_column AS DATE) AS date_only
FROM your_table;
“`
Oracle
In Oracle, use the `TRUNC()` function to remove the time component from a timestamp.
“`sql
SELECT TRUNC(your_timestamp_column) AS date_only
FROM your_table;
“`
Alternatively, you can convert the timestamp to a date explicitly using the `CAST()` function.
“`sql
SELECT CAST(your_timestamp_column AS DATE) AS date_only
FROM your_table;
“`
Comparison Table of Conversion Functions
Database | Function | Example |
---|---|---|
MySQL | DATE(), CAST() | DATE(your_timestamp_column) |
PostgreSQL | ::date, DATE() | your_timestamp_column::date |
SQL Server | CONVERT(), CAST() | CONVERT(DATE, your_timestamp_column) |
Oracle | TRUNC(), CAST() | TRUNC(your_timestamp_column) |
By utilizing these functions appropriately, you can seamlessly convert timestamps to date formats in your SQL queries, ensuring data is presented in the desired format for further analysis or reporting.
Converting Timestamp to Date in SQL
To convert a timestamp to a date in SQL, various functions can be utilized depending on the SQL dialect being used. Below are methods for some of the most common databases:
MySQL
In MySQL, you can use the `DATE()` function to extract the date from a timestamp.
“`sql
SELECT DATE(your_timestamp_column) AS converted_date
FROM your_table;
“`
- `your_timestamp_column`: This is the column containing the timestamp.
- `your_table`: This is the name of your table.
PostgreSQL
PostgreSQL provides the `::date` type cast or the `DATE()` function.
“`sql
SELECT your_timestamp_column::date AS converted_date
FROM your_table;
“`
Alternatively:
“`sql
SELECT DATE(your_timestamp_column) AS converted_date
FROM your_table;
“`
SQL Server
In SQL Server, you can use the `CAST()` or `CONVERT()` functions.
“`sql
SELECT CAST(your_timestamp_column AS DATE) AS converted_date
FROM your_table;
“`
Or:
“`sql
SELECT CONVERT(DATE, your_timestamp_column) AS converted_date
FROM your_table;
“`
- The `CAST()` function is ANSI SQL compliant, while `CONVERT()` allows for style options.
Oracle
In Oracle, you can use the `TRUNC()` function to convert a timestamp to a date, truncating the time portion.
“`sql
SELECT TRUNC(your_timestamp_column) AS converted_date
FROM your_table;
“`
- This will retain only the date part, removing the time.
SQLite
SQLite employs the `date()` function to convert timestamps.
“`sql
SELECT date(your_timestamp_column) AS converted_date
FROM your_table;
“`
- This function effectively strips the time part, returning only the date.
Example Comparison Table
Database | Conversion Function | Example Query |
---|---|---|
MySQL | DATE() | `SELECT DATE(your_timestamp_column) FROM your_table;` |
PostgreSQL | `::date` or DATE() | `SELECT your_timestamp_column::date FROM your_table;` |
SQL Server | CAST() or CONVERT() | `SELECT CAST(your_timestamp_column AS DATE) FROM your_table;` |
Oracle | TRUNC() | `SELECT TRUNC(your_timestamp_column) FROM your_table;` |
SQLite | date() | `SELECT date(your_timestamp_column) FROM your_table;` |
These methods allow for effective conversion of timestamps to dates across different SQL databases. It is essential to choose the appropriate function based on your specific database management system for optimal results.
Expert Insights on Converting Timestamps to Dates in SQL
Dr. Emily Carter (Database Architect, Tech Solutions Inc.). “Converting timestamps to dates in SQL is essential for data analysis, especially when dealing with time-series data. Utilizing the `CAST` or `CONVERT` functions allows for precise formatting, ensuring that only the date component is extracted without time information.”
Michael Chen (Data Analyst, Analytics Hub). “In SQL, the method of converting timestamps to dates can vary depending on the database system in use. For instance, in PostgreSQL, one can simply use the `::date` syntax, while in MySQL, the `DATE()` function is preferred. Understanding these nuances is crucial for effective data manipulation.”
Sarah Johnson (Senior SQL Developer, Data Insights Corp.). “When converting timestamps to dates, it’s important to consider the implications on data integrity and reporting. Using the correct functions not only streamlines queries but also enhances performance by reducing unnecessary data processing.”
Frequently Asked Questions (FAQs)
What is the SQL command to convert a timestamp to a date?
To convert a timestamp to a date in SQL, you can use the `CAST()` or `CONVERT()` functions. For example: `SELECT CAST(your_timestamp_column AS DATE)` or `SELECT CONVERT(DATE, your_timestamp_column)`.
Are there different SQL functions for converting timestamp to date in various databases?
Yes, different SQL databases have specific functions. For instance, MySQL uses `DATE(your_timestamp_column)`, while PostgreSQL uses `your_timestamp_column::DATE` or `DATE(your_timestamp_column)`.
Can I format the date when converting from a timestamp?
Yes, you can format the date during conversion. In SQL Server, you can use `FORMAT(your_timestamp_column, ‘yyyy-MM-dd’)`. In MySQL, you can use `DATE_FORMAT(your_timestamp_column, ‘%Y-%m-%d’)`.
What is the difference between a timestamp and a date in SQL?
A timestamp includes both date and time components, whereas a date only includes the date without any time information. This distinction is crucial for data precision and storage.
Is it possible to convert a timestamp to a date in a specific timezone?
Yes, you can convert a timestamp to a date in a specific timezone using functions like `AT TIME ZONE` in SQL Server or `CONVERT_TZ()` in MySQL. This allows for accurate date representation based on the desired timezone.
What happens if I convert a timestamp to a date without considering time zones?
If you convert a timestamp to a date without considering time zones, you may lose time information and potentially misrepresent the date if the timestamp is in a different timezone than expected. Always ensure timezone consistency when performing conversions.
Converting a timestamp to a date in SQL is a common requirement for database management and data analysis. SQL provides various functions to facilitate this conversion, allowing users to extract date components from timestamps effectively. The specific function used can depend on the SQL dialect being employed, such as MySQL, PostgreSQL, or SQL Server. For instance, in MySQL, the `DATE()` function can be utilized, while in PostgreSQL, the `::date` cast or the `DATE_TRUNC()` function can be applied. Understanding these functions is essential for ensuring accurate data manipulation and reporting.
Moreover, it is important to recognize that timestamps and dates serve different purposes in data management. Timestamps include both date and time information, which can be crucial for time-sensitive applications. In contrast, dates focus solely on the calendar aspect, which may be more appropriate for certain analyses. Therefore, the choice to convert a timestamp to a date should be guided by the specific requirements of the task at hand, ensuring that the data retains its intended meaning and utility.
In summary, mastering the conversion of timestamps to dates in SQL is vital for effective database operations. By leveraging the appropriate functions and understanding the implications of such conversions, users can enhance their data handling capabilities
Author Profile
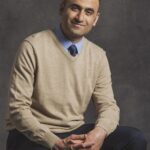
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?