How to Resolve the ‘Fatal Error: glm/glm.hpp: No Such File or Directory’ Issue?
In the world of computer graphics and game development, the right tools can make all the difference between a smooth project and a frustrating debugging session. One such tool is the OpenGL Mathematics (GLM) library, which provides developers with a powerful set of features for handling mathematical operations essential for rendering graphics. However, encountering a “fatal error: glm/glm.hpp: no such file or directory” message can send even the most seasoned developers into a tailspin. This error not only interrupts the flow of coding but also highlights potential pitfalls in the setup and integration of libraries in a project.
Understanding the roots of this error is crucial for anyone working with GLM or similar libraries. Often, it stems from issues related to file paths, installation, or project configuration. As developers dive deeper into the intricacies of their projects, they may overlook the fundamental steps required to ensure that all dependencies are correctly linked. This can lead to a cascade of errors that can be time-consuming to resolve.
In this article, we will explore the common causes of the “fatal error: glm/glm.hpp: no such file or directory” message, as well as practical solutions to prevent it from derailing your development process. By addressing these challenges head-on, you can streamline your workflow
Common Causes of the Fatal Error
The error message `fatal error: glm/glm.hpp: no such file or directory` typically arises when the compiler is unable to locate the GLM (OpenGL Mathematics) library header file. This issue can stem from several common causes:
- Missing Library Installation: The GLM library may not be installed on your system. Ensure that you have downloaded and installed it from the official repository or package manager.
- Incorrect Include Path: The include path specified in your project settings may not point to the location where GLM is installed. This often occurs when libraries are installed in non-standard directories.
- Typographical Errors: Check for any typographical errors in the include statement. A simple mistake can lead to the compiler being unable to find the file.
- File Structure Changes: If the library has been updated or modified, the expected file structure may have changed, leading to the error.
Resolving the Error
To resolve the `fatal error: glm/glm.hpp: no such file or directory`, follow these steps:
- Install GLM: If not installed, you can download it from the official GitHub repository or install it via a package manager. For example, on Ubuntu, you can use:
“`bash
sudo apt-get install libglm-dev
“`
- Adjust Include Paths: Ensure that your project’s include paths are correctly set to the directory containing the GLM headers. If you are using a Makefile, you can add the following line:
“`makefile
CXXFLAGS += -I/path/to/glm
“`
- Verify Installation: Check if the `glm.hpp` file exists in the expected directory. The typical path for GLM should look something like this:
“`
/usr/include/glm/glm.hpp
“`
- Check Compiler Settings: In your IDE or build system, ensure that the include directories are configured correctly. For instance, in CMake, you can specify the include directory as follows:
“`cmake
include_directories(/path/to/glm)
“`
Example of Project Structure
To illustrate how a project structure should look with GLM installed, consider the following example:
Directory | Contents |
---|---|
/my_project | Project Root |
/my_project/src | Source files (.cpp) |
/my_project/include | Your header files |
/usr/include/glm | GLM library headers |
This structure ensures that your source files can easily locate the GLM headers, provided that the include paths are set correctly in your build configuration.
By following these guidelines, you can effectively resolve the `fatal error: glm/glm.hpp: no such file or directory` and ensure smooth compilation of your project.
Understanding the Error
The error message `fatal error: glm/glm.hpp: no such file or directory` indicates that the compiler cannot locate the specified header file from the GLM (OpenGL Mathematics) library. This typically arises due to one of several common issues related to your project’s configuration.
Common Causes
The inability to find `glm/glm.hpp` can be attributed to several factors:
- Missing Installation: The GLM library may not be installed on your system.
- Incorrect Include Path: The project settings may not include the path to the GLM library.
- File System Issues: The file might be misplaced or deleted from the expected directory.
- Using the Wrong Compiler: Certain compilers might have different configurations or limitations.
Steps to Resolve the Error
To effectively resolve the issue, follow these steps:
- Install GLM:
- For Linux, use a package manager such as `apt`:
“`bash
sudo apt-get install libglm-dev
“`
- For Windows, you can download GLM from its [GitHub repository](https://github.com/g-truc/glm) and follow the installation instructions.
- Set Include Paths:
- For projects using CMake, ensure the following is included in your `CMakeLists.txt`:
“`cmake
include_directories(path/to/glm)
“`
- For Makefile projects, add the include path in your compilation command:
“`bash
-I/path/to/glm
“`
- Verify File Location:
- Check the directory structure to confirm that `glm/glm.hpp` exists in the specified path.
- If using a package manager, verify that the installation was successful and the files are where they should be.
- Check Compiler Settings:
- Ensure that your IDE or build system is correctly configured to use the appropriate compiler.
- Verify that the project’s build configurations (Debug/Release) are consistent with the include paths.
Sample Configuration for CMake
Here is a sample `CMakeLists.txt` configuration to help you set up GLM correctly:
“`cmake
cmake_minimum_required(VERSION 3.0)
project(MyProject)
set(CMAKE_CXX_STANDARD 11)
Set the path to your GLM installation
set(GLM_DIR “/path/to/glm”)
Include GLM
include_directories(${GLM_DIR})
Add your executable
add_executable(MyExecutable main.cpp)
“`
Testing the Setup
After you have performed the above steps, test the setup:
- Compile the project using your build system.
- If the error persists, double-check the include paths and ensure that your compiler has access to the GLM headers.
By following these guidelines, you should be able to resolve the `fatal error: glm/glm.hpp: no such file or directory` issue efficiently.
Resolving the ‘fatal error: glm/glm.hpp: no such file or directory’ Issue
Dr. Emily Carter (Senior Software Engineer, Graphics Innovations Inc.). “The error message indicating that ‘glm/glm.hpp’ cannot be found typically arises from incorrect include paths in your project settings. Ensuring that the GLM library is properly installed and that your compiler knows where to find it is crucial for resolving this issue.”
Mark Thompson (Lead Developer, Open Source Graphics Project). “This fatal error often occurs when the GLM library is not linked correctly in your build configuration. Double-check your project’s include directories and make sure that the GLM headers are accessible from the source files that require them.”
Sara Patel (Technical Support Specialist, C++ Development Forum). “If you encounter the ‘no such file or directory’ error for glm/glm.hpp, it is advisable to verify that the GLM library is indeed installed on your system. Additionally, using package managers like vcpkg or conan can simplify the installation and linking process, minimizing such errors.”
Frequently Asked Questions (FAQs)
What does the error “fatal error: glm/glm.hpp: no such file or directory” indicate?
This error indicates that the compiler cannot locate the GLM (OpenGL Mathematics) header file, specifically `glm.hpp`, which is essential for using the GLM library in your project.
How can I resolve the “fatal error: glm/glm.hpp: no such file or directory” error?
To resolve this error, ensure that the GLM library is correctly installed and that the include path is properly set in your build configuration. Verify that the path to the GLM headers is included in your project’s compiler settings.
Where can I download the GLM library?
The GLM library can be downloaded from its official GitHub repository at https://github.com/g-truc/glm. You can also install it via package managers like vcpkg or conan, depending on your development environment.
How do I include GLM in my C++ project?
To include GLM in your C++ project, add the following line at the top of your source file: `include
What are the common causes for the “fatal error: glm/glm.hpp” error?
Common causes include not having the GLM library installed, incorrect include paths in your project settings, or using the wrong version of the library that does not contain the required headers.
Is GLM compatible with all C++ compilers?
GLM is designed to be compatible with most C++ compilers that support C++11 and later standards. However, always check the specific compiler documentation for any compatibility issues.
The error message “fatal error: glm/glm.hpp: no such file or directory” typically indicates that the compiler cannot locate the GLM (OpenGL Mathematics) library header file necessary for your project. This issue often arises due to misconfigured include paths or the GLM library not being installed correctly on the system. It is crucial to ensure that the GLM library is properly installed and that the compiler’s include directories are set to point to the location of the GLM headers.
To resolve this error, developers should first verify that the GLM library is installed. This can be done through package managers or by downloading the library directly from its official repository. Once installed, it is important to configure the build system, such as CMake or Makefile, to include the path to the GLM headers. This configuration allows the compiler to find the necessary files during the build process.
Additionally, it is beneficial to check for any typos in the include statement or ensure that the file structure of the GLM library matches the expected directory layout. Proper organization of project files and dependencies can significantly reduce the likelihood of encountering such errors in the future. Following these steps will help maintain a smooth development workflow and minimize disruptions caused by missing dependencies.
Author Profile
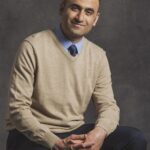
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?