How Can You Implement Reinforcement Learning in Python?
In the rapidly evolving landscape of artificial intelligence, reinforcement learning (RL) stands out as a powerful paradigm that mimics the way humans and animals learn through interaction with their environment. Imagine teaching a computer to play chess or navigate a maze, rewarding it for making the right moves while discouraging mistakes. This fascinating process not only enhances the machine’s decision-making capabilities but also opens the door to solving complex problems across various domains, from robotics to finance. If you’re eager to dive into the world of reinforcement learning using Python, you’re in for an exciting journey filled with challenges and discoveries.
Reinforcement learning is fundamentally about learning from experience. Unlike supervised learning, where a model is trained on labeled data, RL involves an agent that learns to make decisions by receiving feedback in the form of rewards or penalties. This dynamic learning process allows the agent to explore its environment, develop strategies, and ultimately optimize its performance over time. Python, with its rich ecosystem of libraries and frameworks, provides an ideal platform for implementing RL algorithms, making it accessible for both beginners and seasoned developers.
As you embark on your reinforcement learning adventure, you’ll encounter key concepts such as agents, environments, states, and actions. Understanding these components is crucial for building effective RL models. Additionally, popular libraries like
Setting Up Your Environment
To begin working with reinforcement learning in Python, you need to set up your development environment appropriately. The following steps will help you prepare:
- Install Python: Ensure you have Python 3.6 or higher installed. You can download it from the [official Python website](https://www.python.org/downloads/).
- Create a Virtual Environment: It is good practice to create a virtual environment for your projects to manage dependencies. You can do this using `venv`:
“`bash
python -m venv rl-env
source rl-env/bin/activate On Windows use `rl-env\Scripts\activate`
“`
- Install Required Libraries: Common libraries for reinforcement learning include NumPy, OpenAI Gym, and TensorFlow or PyTorch. Install them using pip:
“`bash
pip install numpy gym tensorflow or pip install torch for PyTorch
“`
Understanding the Basics of Reinforcement Learning
Reinforcement learning (RL) involves training an agent to make decisions by maximizing cumulative rewards in an environment. Key components include:
- Agent: The learner or decision maker.
- Environment: The external system the agent interacts with.
- State: A representation of the current situation of the agent.
- Action: The choices available to the agent.
- Reward: Feedback from the environment based on the action taken.
The agent learns by exploring actions and receiving feedback in the form of rewards or penalties, which helps it improve its decision-making over time.
Implementing a Simple Reinforcement Learning Algorithm
A popular algorithm to start with is Q-learning, which is model-free and works well in discrete action spaces. Here’s a simple implementation:
- Initialize Q-Table: Create a table with states and actions initialized to zero.
- Choose Action: Use an epsilon-greedy policy to balance exploration and exploitation.
- Update Q-Values: Apply the Q-learning formula:
\[
Q(s, a) \leftarrow Q(s, a) + \alpha \left( r + \gamma \max_a Q(s’, a) – Q(s, a) \right)
\]
- Iterate: Repeat the process for a number of episodes.
Here’s a basic structure of the code:
“`python
import numpy as np
import gym
Create the environment
env = gym.make(‘Taxi-v3’)
Initialize Q-table
Q = np.zeros([env.observation_space.n, env.action_space.n])
Parameters
alpha = 0.1 Learning rate
gamma = 0.6 Discount factor
epsilon = 0.1 Exploration rate
Training
for episode in range(1000):
state = env.reset()
done =
while not done:
if np.random.rand() < epsilon:
action = env.action_space.sample() Explore
else:
action = np.argmax(Q[state]) Exploit
next_state, reward, done, _ = env.step(action)
Update Q-table
Q[state, action] += alpha * (reward + gamma * np.max(Q[next_state]) - Q[state, action])
state = next_state
```
Evaluating the Agent
After training your reinforcement learning agent, it is essential to evaluate its performance. Common metrics include:
- Total Reward: The sum of rewards received during the episode.
- Success Rate: The percentage of episodes where the goal was achieved.
You can create a simple evaluation loop:
“`python
total_rewards = 0
for episode in range(100):
state = env.reset()
done =
while not done:
action = np.argmax(Q[state]) Select the best action
state, reward, done, _ = env.step(action)
total_rewards += reward
print(f’Average Reward over 100 episodes: {total_rewards / 100}’)
“`
Advanced Techniques and Libraries
For more complex problems, consider using advanced algorithms like Deep Q-Networks (DQN) or Proximal Policy Optimization (PPO). Popular libraries that facilitate reinforcement learning include:
Library | Description |
---|---|
Stable Baselines3 | A set of reliable implementations of RL algorithms in PyTorch. |
Ray RLLib | A scalable reinforcement learning library built on Ray. |
OpenAI Baselines | High-quality implementations of RL algorithms in TensorFlow. |
These libraries can significantly reduce the complexity of building RL agents and allow you to focus on experimentation and fine-tuning.
Understanding the Basics of Reinforcement Learning
Reinforcement Learning (RL) is a type of machine learning where an agent learns to make decisions by taking actions in an environment to maximize cumulative reward. The key components of RL include:
- Agent: The learner or decision-maker.
- Environment: The external system the agent interacts with.
- Action: Choices made by the agent that affect the state of the environment.
- State: A representation of the current situation of the agent within the environment.
- Reward: Feedback from the environment based on the action taken.
Setting Up Your Python Environment
To start implementing reinforcement learning in Python, ensure you have the following libraries installed:
- NumPy: For numerical operations.
- Matplotlib: For plotting results.
- OpenAI Gym: A toolkit for developing and comparing RL algorithms.
- TensorFlow or PyTorch: For building neural networks.
You can install these libraries using pip:
“`bash
pip install numpy matplotlib gym tensorflow
“`
Implementing a Simple Reinforcement Learning Algorithm
A common starting point in RL is the Q-learning algorithm. Below is a basic outline of how to implement Q-learning in Python.
- Initialize the Q-table: A table of state-action values.
- Define the parameters: Learning rate, discount factor, and exploration rate.
- Choose an action: Based on the exploration-exploitation trade-off.
- Update the Q-values: Using the Bellman equation.
Here’s a simplified code snippet demonstrating Q-learning in a grid environment:
“`python
import numpy as np
import gym
Create the environment
env = gym.make(‘Taxi-v3’)
Initialize Q-table
Q = np.zeros([env.observation_space.n, env.action_space.n])
Define parameters
learning_rate = 0.8
discount_factor = 0.95
exploration_rate = 1.0
exploration_decay = 0.995
episodes = 1000
for episode in range(episodes):
state = env.reset()
done =
while not done:
if np.random.rand() < exploration_rate:
action = env.action_space.sample() Explore
else:
action = np.argmax(Q[state]) Exploit
next_state, reward, done, _ = env.step(action)
Update Q-values
Q[state, action] = Q[state, action] + learning_rate * (reward + discount_factor * np.max(Q[next_state]) - Q[state, action])
state = next_state
exploration_rate *= exploration_decay
```
Utilizing Deep Reinforcement Learning
For more complex environments, Deep Reinforcement Learning (DRL) combines neural networks with RL. Popular algorithms include Deep Q-Networks (DQN) and Proximal Policy Optimization (PPO). Key steps to implement DRL:
- Define the neural network architecture: This will approximate the Q-values or policy.
- Use experience replay: Store past experiences to break correlation in training data.
- Implement target networks: Stabilize training by periodically updating the target network.
A simple DQN implementation structure:
- Input Layer: State representation.
- Hidden Layers: Several fully connected layers.
- Output Layer: Q-values for each action.
“`python
import tensorflow as tf
model = tf.keras.Sequential([
tf.keras.layers.Dense(24, activation=’relu’, input_shape=(state_size,)),
tf.keras.layers.Dense(24, activation=’relu’),
tf.keras.layers.Dense(action_size, activation=’linear’)
])
model.compile(optimizer=’adam’, loss=’mse’)
“`
Evaluating and Tuning Your Model
To evaluate and improve your reinforcement learning model, consider the following metrics:
Metric | Description |
---|---|
Cumulative Reward | Total rewards received during an episode. |
Win Rate | Percentage of episodes where the agent successfully completes a task. |
Learning Curve | Graph of cumulative rewards or other metrics over episodes. |
Tuning hyperparameters such as learning rate, discount factor, and exploration rate can significantly impact performance. Employ techniques like grid search or random search for effective tuning.
Resources for Further Learning
- Books: “Reinforcement Learning: An ” by Sutton and Barto.
- Online Courses: Coursera, Udacity offer specialized courses in reinforcement learning.
- GitHub Repositories: Explore open-source implementations for practical insights.
With this framework, you can begin developing reinforcement learning models tailored to specific challenges in various domains.
Expert Insights on Implementing Reinforcement Learning in Python
Dr. Emily Chen (Lead Data Scientist, AI Innovations Lab). “To effectively implement reinforcement learning in Python, it is crucial to familiarize oneself with libraries such as TensorFlow and PyTorch. These frameworks provide robust tools for building and training reinforcement learning models, allowing practitioners to focus on algorithm design rather than low-level implementation details.”
James O’Connor (Senior Machine Learning Engineer, Tech Solutions Inc.). “Understanding the Markov Decision Process (MDP) is fundamental when working with reinforcement learning. It is essential to grasp how states, actions, and rewards interact, as this knowledge will guide the development of effective policies and value functions in your Python projects.”
Dr. Sarah Patel (Professor of Computer Science, University of Technology). “I recommend starting with simple environments, such as those provided by OpenAI’s Gym, to practice reinforcement learning algorithms. This hands-on approach will enable you to experiment with different strategies and gain practical experience before tackling more complex scenarios in Python.”
Frequently Asked Questions (FAQs)
What is reinforcement learning?
Reinforcement learning is a type of machine learning where an agent learns to make decisions by taking actions in an environment to maximize cumulative rewards over time. It involves exploration and exploitation strategies to improve performance.
What libraries are commonly used for reinforcement learning in Python?
Popular libraries for reinforcement learning in Python include TensorFlow, PyTorch, OpenAI Gym, Stable Baselines3, and Ray Rllib. These libraries provide tools and environments to facilitate the development and training of reinforcement learning models.
How do I set up a reinforcement learning environment in Python?
To set up a reinforcement learning environment, you can use OpenAI Gym. Install the library using pip, create an environment using `gym.make()`, and then define the agent’s interaction with the environment through actions, observations, and rewards.
What are the key components of a reinforcement learning algorithm?
The key components of a reinforcement learning algorithm include the agent, environment, state, action, reward, policy, and value function. The agent interacts with the environment, receiving states and rewards based on its actions, which it uses to learn an optimal policy.
How can I evaluate the performance of a reinforcement learning model?
Performance evaluation of a reinforcement learning model can be done through metrics such as average reward, success rate, and convergence speed. Additionally, visualizing the learning curve and testing the agent in various scenarios can provide insights into its effectiveness.
Are there any recommended tutorials or resources for learning reinforcement learning in Python?
Yes, several resources are available, including online courses on platforms like Coursera and Udacity, as well as books such as “Reinforcement Learning: An ” by Sutton and Barto. Additionally, numerous tutorials and projects can be found on GitHub and Medium.
Reinforcement learning (RL) in Python involves a systematic approach to developing algorithms that enable agents to learn optimal behaviors through interactions with their environment. The foundational concepts of RL include agents, environments, states, actions, and rewards. By leveraging libraries such as TensorFlow, PyTorch, and OpenAI Gym, practitioners can efficiently implement and experiment with various RL algorithms, including Q-learning, Deep Q-Networks (DQN), and Policy Gradient methods.
To successfully implement reinforcement learning in Python, one must first understand the theoretical underpinnings of the algorithms. This includes grasping concepts like exploration vs. exploitation, value functions, and the Bellman equation. Additionally, setting up a proper environment for training, which can be done using simulation tools like OpenAI Gym, is crucial for testing the agent’s performance in a controlled setting.
Moreover, practitioners should focus on hyperparameter tuning, as it significantly impacts the learning process and the eventual performance of the RL agent. Techniques such as grid search, random search, or more advanced methods like Bayesian optimization can be employed to identify optimal hyperparameters. Finally, continuous evaluation and iteration on the model are essential for improving the agent’s learning efficiency and effectiveness in real-world applications.
Author Profile
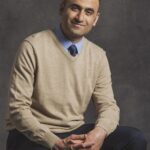
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?