How Can You Discover Layer Names in Unity Beyond Just Using LayerToName?
In the world of game development, particularly within Unity, understanding how to manage layers effectively is crucial for optimizing performance and enhancing gameplay mechanics. While many developers are familiar with the `LayerToName` function for retrieving layer names, there are various other methods and techniques to explore that can streamline your workflow. Whether you’re a seasoned developer or just starting your journey in Unity, discovering alternative approaches to find layer names can significantly impact your project’s organization and efficiency.
When working with layers in Unity, it’s essential to grasp the underlying structure and functionality that layers provide. Layers are not just a way to categorize objects; they play a vital role in collision detection, rendering, and managing visibility in your scenes. By delving deeper into the Unity API and exploring different methods for accessing layer names, developers can unlock new possibilities for customizing their game environments and interactions.
In this article, we’ll explore various strategies for finding layer names beyond the traditional `LayerToName` method. From leveraging the Unity Editor’s built-in features to utilizing scripting techniques, you’ll gain insights into how to efficiently manage your layers and enhance your overall development experience. Prepare to elevate your Unity skills as we uncover the hidden gems of layer management!
Using LayerMask in Unity
LayerMask is a powerful tool in Unity that allows you to work with layers more efficiently. Instead of relying on the layer name directly, you can use LayerMask to manipulate layers programmatically. This approach is especially useful when dealing with multiple layers or when you want to avoid hardcoding strings that can lead to errors.
To utilize LayerMask, you can create a LayerMask variable and assign layers to it using the bitmask representation. Here’s how you can do it:
- Define a LayerMask variable in your script.
- Use the `LayerMask.GetMask` method to convert layer names to their corresponding bitmask values.
Example code snippet:
“`csharp
LayerMask myLayerMask = LayerMask.GetMask(“MyLayerName”);
“`
This code will create a LayerMask that includes only the specified layer, allowing for efficient checks during collision detection or raycasting.
Accessing Layer Names Programmatically
If you need to retrieve the names of layers dynamically, you can utilize Unity’s `LayerMask.LayerToName` method. This method takes an integer representing the layer index and returns the corresponding layer name as a string.
The following example demonstrates how to iterate through all layers and get their names:
“`csharp
for (int i = 0; i < 32; i++)
{
string layerName = LayerMask.LayerToName(i);
if (!string.IsNullOrEmpty(layerName))
{
Debug.Log($"Layer {i}: {layerName}");
}
}
```
This code will log all the layers present in your Unity project, providing a quick reference for layer names without hardcoding them.
Table of Layer Access Methods
The following table summarizes various methods for accessing layer information in Unity:
Method | Description |
---|---|
LayerMask.GetMask(string layerName) | Converts a layer name to its corresponding LayerMask. |
LayerMask.LayerToName(int layer) | Retrieves the name of the layer based on the layer index. |
LayerMask.NameToLayer(string layerName) | Gets the layer index from the layer name. |
GameObject.layer | Accesses or sets the layer for a GameObject. |
By leveraging these methods, you can streamline your layer management within Unity, minimizing potential errors and improving code maintainability.
Alternative Methods to Identify Layer Names in Unity
To find layer names in Unity aside from using `LayerMask.NameToLayer()`, there are several approaches that can be utilized. Each method offers distinct advantages depending on the context of your project and the specific requirements of your game development process.
Utilizing Unity’s Editor
You can easily check and manage layer names directly within the Unity Editor. Here are the steps:
- Navigate to the top menu bar.
- Select **Edit** > **Project Settings** > Tags and Layers.
- In the Layers section, you will find a list of all defined layers.
This method allows you to visually inspect layer names and make necessary adjustments without needing to write any code.
Accessing Layer Names via Script
If you prefer a programmatic approach, you can access layer names through the `LayerMask` class in Unity. Here are a few techniques:
- Using `LayerMask`: You can retrieve the layer name associated with a specific layer index. Here’s a simple code snippet:
“`csharp
for (int i = 0; i < 32; i++)
{
string layerName = LayerMask.LayerToName(i);
if (!string.IsNullOrEmpty(layerName))
{
Debug.Log($"Layer {i}: {layerName}");
}
}
```
This loop will output all layer names currently defined in the project.
- Using SerializedObject: If you are working with custom editor scripts, you can use `SerializedObject` to access layer names dynamically:
“`csharp
SerializedObject serializedObject = new SerializedObject(AssetDatabase.LoadAllAssetsAtPath(“ProjectSettings/TagManager.asset”)[0]);
SerializedProperty layers = serializedObject.FindProperty(“layers”);
for (int i = 0; i < layers.arraySize; i++)
{
Debug.Log(layers.GetArrayElementAtIndex(i).stringValue);
}
```
This method is useful for obtaining layer names in a more controlled context, especially in editor scripts.
Layer Names in Physics and Collision
When dealing with physics and collisions in Unity, you may want to check layer names to manage interactions effectively. The following methods can be employed:
- Physics.Raycast: You can specify layers for raycasting to filter collisions based on layer names. For example:
“`csharp
int layerMask = LayerMask.GetMask(“Player”, “Enemy”);
if (Physics.Raycast(transform.position, transform.forward, out hit, 100f, layerMask))
{
Debug.Log($”Hit: {hit.collider.gameObject.name} on layer: {LayerMask.LayerToName(hit.collider.gameObject.layer)}”);
}
“`
This ensures that only objects on the specified layers are considered in the raycast.
- Collision Detection: Use the `OnCollisionEnter` method to identify the layer of the collided object:
“`csharp
void OnCollisionEnter(Collision collision)
{
string collidedLayer = LayerMask.LayerToName(collision.gameObject.layer);
Debug.Log($”Collided with an object on layer: {collidedLayer}”);
}
“`
Custom Layer Management Tools
For larger projects, consider implementing custom layer management tools or scripts. This can streamline the process of handling layers:
- Layer Management Class: Create a class that centralizes layer information and provides easy access to layer names and indices.
“`csharp
public static class LayerManager
{
public static string GetLayerName(int layerIndex)
{
return LayerMask.LayerToName(layerIndex);
}
public static int GetLayerIndex(string layerName)
{
return LayerMask.NameToLayer(layerName);
}
}
“`
This enables you to manage layers more effectively throughout your codebase.
Discovering Layer Names in Unity Beyond LayerToName
Dr. Emily Chen (Game Development Specialist, Unity Technologies). “To find layer names in Unity besides using LayerToName, developers can utilize the LayerMask class. By accessing the layer mask directly, you can iterate through the layers and retrieve their names programmatically, which offers a more flexible approach for dynamic game environments.”
Mark Thompson (Senior Software Engineer, GameDev Insights). “Another effective method to find layer names is to leverage the Unity Editor scripting capabilities. By creating custom editor scripts, you can access the internal layer settings and display them in a user-friendly format, making it easier for developers to manage layers during the design phase.”
Sarah Patel (Technical Artist, Indie Game Studio). “In addition to LayerToName, I recommend checking the Unity documentation for the LayerMask enumeration. This not only provides a comprehensive list of layers but also helps in understanding how to manipulate them through scripting, which is essential for optimizing performance in complex scenes.”
Frequently Asked Questions (FAQs)
How can I find the layer name of a GameObject in Unity?
You can find the layer name of a GameObject by accessing the `LayerMask.LayerToName` method. Pass the GameObject’s layer index using `gameObject.layer` to retrieve the corresponding layer name.
Is there a way to list all layer names in Unity?
Yes, you can list all layer names by iterating through the layers defined in Unity. Use `for (int i = 0; i < 32; i++) { string layerName = LayerMask.LayerToName(i); if (!string.IsNullOrEmpty(layerName)) { Debug.Log(layerName); } }` to log each layer name.
Can I change a GameObject’s layer programmatically?
Yes, you can change a GameObject’s layer by setting its `layer` property. For example, `gameObject.layer = LayerMask.NameToLayer(“NewLayerName”);` assigns the specified layer to the GameObject.
What is the purpose of layers in Unity?
Layers in Unity are used to categorize GameObjects for various purposes, such as rendering, physics interactions, and culling. They help optimize performance and manage visibility in complex scenes.
How do I find out which layer a specific GameObject is on?
To determine which layer a specific GameObject is on, access the `layer` property of the GameObject. You can then convert it to a layer name using `LayerMask.LayerToName(gameObject.layer)`.
Are there any limitations to the number of layers I can create in Unity?
Unity allows a maximum of 32 layers, which can be defined in the Layer Inspector. Each layer can be assigned to GameObjects, but exceeding this limit is not possible.
In Unity, finding layer names beyond the conventional LayerToName function can be approached through various methods. While LayerToName is a straightforward way to retrieve layer names based on their index, developers can also utilize the Unity Editor’s Layer settings to view and manage layers directly. Additionally, accessing layer names programmatically can be achieved through the use of predefined constants or by iterating through the layers using the `GetLayer` method in scripts.
Another effective approach is to utilize the Unity API, specifically the `Physics` class, which provides methods to interact with layers in a more dynamic manner. This includes functions like `LayerMask`, which allows developers to create masks that can filter interactions based on layer assignments. Furthermore, custom scripts can be developed to encapsulate layer management, providing a more organized way to handle layer names and their corresponding indices.
In summary, while LayerToName is a commonly used method for retrieving layer names in Unity, there are multiple alternative strategies available. By leveraging the Unity Editor, utilizing the Unity API, and creating custom scripts, developers can efficiently manage and access layer names tailored to their specific project needs. These insights can enhance workflow efficiency and improve the overall development experience in Unity.
Author Profile
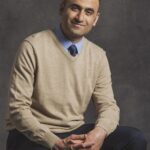
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?