Why Am I Seeing ‘SLF4J: Class Path Contains Multiple SLF4J Bindings’ and How Can I Fix It?
In the world of Java development, logging is an essential aspect that can significantly impact the performance and maintainability of applications. One of the most popular logging frameworks is SLF4J (Simple Logging Facade for Java), which provides a simple interface for various logging implementations. However, as developers integrate multiple libraries and frameworks into their projects, they often encounter a common yet perplexing issue: the warning message indicating that the class path contains multiple SLF4J bindings. This seemingly innocuous warning can lead to confusion and potential logging failures if not addressed properly. In this article, we will delve into the intricacies of SLF4J bindings, exploring their significance, the implications of having multiple bindings, and how to resolve this common pitfall in Java applications.
Understanding SLF4J bindings is crucial for any Java developer, as they dictate how log messages are handled and routed to the appropriate logging framework. When multiple bindings are present, it can create ambiguity about which logging implementation will be used, leading to unpredictable behavior in your application. This scenario not only complicates debugging but can also result in performance issues, as the logging framework may struggle to determine the correct binding to utilize.
As we navigate this topic, we will examine the reasons behind multiple SLF4J
Understanding SLF4J Bindings
When working with SLF4J (Simple Logging Facade for Java), it is crucial to understand the concept of bindings. SLF4J serves as a facade for various logging frameworks, allowing developers to plug in their preferred logging implementation without being tied to a specific framework. However, having multiple bindings in the classpath can lead to conflicts and unexpected behavior.
The SLF4J library relies on a single binding to resolve logging calls. When multiple bindings are detected, it raises a warning, indicating that the classpath contains multiple SLF4J bindings. This can happen when different libraries or dependencies utilize different logging frameworks or configurations.
Common Causes of Multiple Bindings
Several scenarios can lead to multiple SLF4J bindings being included in the classpath:
- Dependency Conflicts: Different libraries may depend on different logging implementations.
- Transitive Dependencies: Libraries might have their own dependencies that include SLF4J bindings.
- Incompatible Versions: Using multiple versions of the same logging framework can also cause this issue.
Identifying the Bindings
To identify which SLF4J bindings are present in your classpath, you can use the following command in your terminal:
“`bash
mvn dependency:tree | grep slf4j
“`
This command will list the SLF4J dependencies and their versions. You can also check the `pom.xml` file or your build configuration files for any explicit SLF4J dependencies.
Resolving Multiple Binding Issues
To resolve the issue of multiple SLF4J bindings, consider the following steps:
- Exclude Unused Dependencies: If a library includes an unwanted SLF4J binding, you can exclude it in your build configuration.
- Align Versions: Ensure all libraries use the same version of SLF4J.
- Use Dependency Management: Utilize tools like Maven’s dependency management to control which versions are included.
Step | Action |
---|---|
1 | Run dependency analysis to check for conflicting bindings. |
2 | Identify and exclude unnecessary bindings in your build file. |
3 | Test the application to ensure logging works as expected. |
By following these guidelines, you can effectively manage SLF4J bindings and maintain a clean logging setup that avoids conflicts.
Understanding SLF4J Bindings
SLF4J (Simple Logging Facade for Java) serves as a simple facade or abstraction for various logging frameworks. It allows developers to plug in any desired logging framework at deployment time. However, the presence of multiple SLF4J bindings in the class path can lead to conflicts and unexpected behavior.
Common Causes of Multiple Bindings
The “class path contains multiple SLF4J bindings” warning typically arises from one or more of the following scenarios:
- Dependency Conflicts: Different libraries in your project may depend on different SLF4J implementations.
- Incorrect Dependency Management: Manual inclusion of multiple SLF4J bindings in your project can lead to redundancy.
- Transitive Dependencies: A library you included may bring in its own SLF4J binding, leading to multiple versions.
Identifying SLF4J Bindings
To identify which bindings are present in your class path, you can use the following methods:
- Maven Dependency Plugin:
- Execute `mvn dependency:tree` to get a structured view of dependencies.
- Gradle Dependency Insight:
- Use `./gradlew dependencies` to list all dependencies and their versions.
- Check JAR Files:
- Inspect the `lib` directory of your project for JAR files containing SLF4J bindings.
Resolving Multiple Bindings
Addressing the issue of multiple SLF4J bindings involves several steps:
- Choose a Single Binding:
- Select one SLF4J binding that fits your project’s logging requirements (e.g., logback, log4j).
- Exclude Conflicting Bindings:
- Use dependency management features to exclude unwanted bindings. For Maven:
“`xml
“`
- For Gradle:
“`groovy
implementation(‘com.example:example-lib:1.0’) {
exclude group: ‘org.slf4j’, module: ‘slf4j-log4j12’
}
“`
- Clean Your Build:
- After making changes, ensure to clean and rebuild your project to avoid any stale references.
Best Practices for Managing SLF4J
To prevent issues with SLF4J bindings in the future, follow these best practices:
- Use Dependency Management Tools: Rely on tools like Maven or Gradle to handle dependencies effectively.
- Regularly Update Dependencies: Keep your libraries up to date to benefit from bug fixes and improvements.
- Monitor Transitive Dependencies: Use dependency analysis tools to track and manage transitive dependencies effectively.
Example of SLF4J Binding Conflict
Conflict Scenario | Description |
---|---|
Binding A | `slf4j-api-1.7.30.jar` |
Binding B | `slf4j-log4j12-1.7.30.jar` |
Binding C | `logback-classic-1.2.3.jar` |
In this scenario, if both Binding B and Binding C are present, SLF4J will warn about the conflict, as it cannot determine which logging framework to use.
By following the outlined steps and best practices, developers can effectively manage SLF4J bindings and avoid the pitfalls associated with multiple bindings in their Java applications.
Understanding SLF4J Binding Conflicts in Java Applications
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The warning about multiple SLF4J bindings typically indicates that your application is loading more than one logging implementation. This can lead to unpredictable behavior, as SLF4J may choose one binding over the others, which can result in missing logs or incorrect log outputs.”
Michael Tran (Lead Java Developer, CodeCraft Solutions). “Resolving the ‘multiple SLF4J bindings’ issue requires careful dependency management. Tools like Maven or Gradle can help you analyze and exclude unnecessary bindings, ensuring that only the intended logging framework is included in your classpath.”
Jessica Liu (Java Framework Specialist, OpenSource Development Group). “It is crucial to understand that while SLF4J acts as a facade for various logging frameworks, having multiple bindings can complicate the logging setup. Developers should aim to maintain a clean and consistent logging strategy by selecting a single binding that suits their project requirements.”
Frequently Asked Questions (FAQs)
What does the warning “slf4j: class path contains multiple slf4j bindings” mean?
The warning indicates that there are multiple SLF4J binding implementations present in the classpath. SLF4J allows only one binding to be active at a time, and having multiple bindings can lead to unpredictable logging behavior.
How can I resolve the “multiple slf4j bindings” warning?
To resolve this warning, identify the SLF4J bindings in your classpath and remove the unnecessary ones. Ensure that only one binding, such as slf4j-log4j12 or slf4j-simple, is included in your project dependencies.
What are common SLF4J bindings that might cause this issue?
Common SLF4J bindings include slf4j-log4j12, slf4j-simple, and slf4j-jdk14. If your project includes multiple logging frameworks or libraries, it is likely that more than one binding has been included.
Can multiple SLF4J bindings affect application performance?
Yes, multiple bindings can lead to performance issues due to potential conflicts and increased overhead in resolving which binding to use. It is best practice to maintain a single binding for optimal performance.
What tools can help identify multiple SLF4J bindings in a project?
Dependency management tools like Maven or Gradle can help identify multiple SLF4J bindings. Use commands like `mvn dependency:tree` or `gradle dependencies` to inspect your project’s dependencies.
Is it safe to ignore the “multiple slf4j bindings” warning?
Ignoring the warning is not advisable, as it may lead to inconsistent logging behavior and complicate debugging. It is best to address the issue to ensure reliable logging in your application.
The warning message regarding “class path contains multiple SLF4J bindings” indicates that the Simple Logging Facade for Java (SLF4J) framework has detected more than one binding for logging implementations in the classpath. This situation typically arises when multiple logging frameworks, such as Logback, Log4j, or java.util.logging, are included in a project, leading to potential conflicts and unpredictable logging behavior. It is essential to address this issue to ensure that the application logs correctly and consistently.
To resolve the multiple bindings warning, developers should identify all the SLF4J binding JARs present in their project dependencies. This can be achieved by inspecting the project’s dependency tree or using build tools like Maven or Gradle to analyze the dependencies. Once identified, the developer must decide which logging framework to retain and exclude the others to maintain a clean and efficient logging setup.
Moreover, it is advisable to keep the SLF4J API and the chosen binding version compatible to avoid runtime exceptions. Regularly updating dependencies and performing dependency audits can help prevent such conflicts in the future. By maintaining a single SLF4J binding, developers can ensure that their applications utilize a consistent logging strategy, which is crucial for debugging and monitoring purposes.
Author Profile
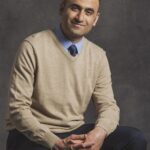
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?