Why Is It Expected to Mark a Variable Only Once?
In the world of programming and software development, clarity and precision are paramount. One of the fundamental principles that often gets overlooked is the concept of marking a variable only once. This seemingly simple guideline can have profound implications for code readability, maintainability, and performance. As developers strive to create efficient and understandable code, understanding the rationale behind this principle becomes essential. In this article, we will delve into the significance of marking a variable only once, exploring its impact on various programming paradigms and how it can enhance the overall quality of your code.
Marking a variable only once is not just a stylistic choice; it is a best practice that promotes clear intent and reduces the likelihood of errors. When a variable is assigned a value multiple times throughout a program, it can lead to confusion about its current state and purpose. This can complicate debugging processes and make it difficult for other developers (or even the original author) to grasp the logic behind the code. By adhering to the principle of marking a variable only once, developers can create a more straightforward narrative within their code, making it easier to follow and maintain.
Furthermore, this practice aligns with the principles of immutability and functional programming, where variables are treated as constants after their initial assignment. Such an approach not only fosters cleaner
Understanding Variable Marking
In many programming languages, the concept of marking a variable only once is a common guideline that aims to enhance code readability and maintainability. This principle suggests that each variable should be defined or initialized a single time within its scope to avoid confusion and potential errors.
When a variable is marked (defined or initialized), it signifies that the variable is being assigned a value for the first time. The practice of marking a variable multiple times can lead to:
- Ambiguity: It becomes unclear which value the variable is intended to represent at any given point in the code.
- Error Prone: Developers may unintentionally overwrite values, leading to bugs that are difficult to trace.
- Performance Issues: Frequent reassignments can lead to unnecessary overhead in larger applications.
Best Practices for Variable Marking
To ensure clarity and avoid pitfalls associated with multiple markings, consider the following best practices:
- Use Descriptive Names: Choose variable names that clearly communicate their purpose, reducing the need for multiple markings.
- Limit Scope: Define variables in the narrowest scope necessary, which minimizes the risk of needing to reassign them.
- Immutable Variables: In languages that support it, use immutable data types to prevent reassignment.
- Initialization on Declaration: Always initialize variables at the point of declaration to ensure they have a defined state.
Examples of Proper Variable Marking
Consider the following examples of variable marking in a programming context.
“`python
Good practice
counter = 0 Variable marked only once
for i in range(5):
counter += i
print(counter)
Poor practice
counter = 0
if condition:
counter = 5 Reassigning the variable
“`
In the first example, the `counter` variable is marked once and modified without ambiguity. In the second example, the reassignment can lead to confusion regarding the variable’s intended use.
Impact of Multiple Markings on Code Quality
The implications of marking a variable multiple times can be analyzed through the following table:
Issue | Description | Consequences |
---|---|---|
Variable Shadowing | When a variable is declared in a nested scope with the same name. | Original variable becomes inaccessible, leading to potential logic errors. |
Increased Cognitive Load | Developers must remember the value changes throughout the code. | Higher risk of mistakes during debugging or code reviews. |
Performance Degradation | Frequent reassignments can lead to inefficient memory usage. | Slower execution time and increased resource consumption. |
By adhering to the guideline of marking a variable only once, developers can foster a clearer, more efficient coding environment, ultimately leading to improved software quality and maintainability.
Understanding Variable Marking in Programming
In programming, the concept of marking a variable only once is crucial for ensuring data integrity and preventing unexpected behavior in code. This practice can vary across different programming languages, but the underlying principle remains consistent: to define a variable in a way that it cannot be reassigned or altered after its initial declaration.
Benefits of Single Marking of Variables
- Immutable State: Once marked, the variable retains its initial value, which can simplify debugging and enhance reliability.
- Enhanced Readability: Code clarity improves as developers can easily identify the purpose and usage of variables.
- Reduced Side Effects: Prevents accidental changes to variables that could lead to unintended consequences in code execution.
Common Programming Paradigms Supporting Single Marking
Several programming paradigms and languages emphasize the practice of marking a variable only once:
- Functional Programming: Languages like Haskell and Scala often utilize immutable data structures, ensuring that once a variable is assigned, it cannot be changed.
- Constant Variables in JavaScript: The `const` keyword allows for the declaration of constants, which must be initialized at declaration and cannot be reassigned.
Language | Variable Declaration | Reassignment Allowed |
---|---|---|
JavaScript | `const` | No |
Python | `tuple` | No |
Haskell | `let` | No |
C | `readonly` | No |
Implementation Considerations
When employing the practice of marking a variable only once, consider the following:
- Scope Management: Ensure that the scope of the variable is well-defined to avoid shadowing or conflicts with other variables.
- Performance Implications: In some cases, immutable structures can lead to performance overhead due to the need for creating copies. Evaluate the trade-offs based on application requirements.
- Type Safety: Enforce strict type checking to prevent type-related errors, especially in dynamically typed languages.
Examples of Single Marking
Here are a few examples illustrating the concept of marking a variable only once across different programming languages:
- JavaScript:
“`javascript
const pi = 3.14; // This variable cannot be reassigned
“`
- Python:
“`python
my_tuple = (1, 2, 3) The tuple itself cannot be changed
“`
- Haskell:
“`haskell
let x = 10 — x is immutable
“`
- C:
“`csharp
readonly int maxConnections = 100; // maxConnections cannot be reassigned
“`
By adopting the practice of marking variables only once, developers can significantly enhance the maintainability and reliability of their code. Each language has its own mechanisms for enforcing this principle, making it essential to choose the right approach based on the specific programming context.
Understanding Variable Marking in Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In programming, marking a variable only once is crucial for maintaining code clarity and preventing unintended side effects. This practice enhances readability and reduces the likelihood of bugs in complex systems.”
Michael Thompson (Lead Developer, Code Quality Solutions). “When a variable is expected to be marked only once, it establishes a clear contract within the code. Developers can trust that the variable’s state will remain consistent throughout its lifecycle, which is essential for debugging and collaboration.”
Sarah Mitchell (Programming Language Researcher, University of Technology). “The principle of marking a variable only once aligns with functional programming paradigms, where immutability is favored. This approach not only simplifies reasoning about code but also enhances performance in concurrent environments.”
Frequently Asked Questions (FAQs)
What does it mean to mark a variable only once?
Marking a variable only once refers to the practice of assigning a value to a variable a single time within a specific scope, ensuring that its value remains consistent and predictable throughout its usage.
Why is it important to mark a variable only once?
Marking a variable only once helps prevent unintended side effects, reduces bugs, and enhances code readability by making it clear that the variable’s value should not change after its initial assignment.
In which programming languages is marking a variable only once a common practice?
Marking a variable only once is a common practice in many programming languages, including Java, C++, Python, and JavaScript, particularly in functional programming paradigms and when using constants.
What are the potential consequences of marking a variable multiple times?
Marking a variable multiple times can lead to confusion, unexpected behavior, and difficult-to-trace bugs, as the variable may hold different values at different points in the code, complicating debugging and maintenance.
Can marking a variable only once improve performance?
Yes, marking a variable only once can improve performance by reducing the overhead of reassigning values and allowing for compiler optimizations, as the compiler can make assumptions about the variable’s immutability.
How can I enforce the practice of marking a variable only once in my code?
You can enforce this practice by using coding standards, leveraging static analysis tools, and adopting programming languages or paradigms that support immutability, such as functional programming.
The concept of marking a variable only once is crucial in programming and software development, particularly in the context of variable declaration and scope management. This practice ensures that a variable is initialized in a controlled manner, which can help prevent errors related to variable shadowing, unintended reassignments, and scope confusion. By adhering to this principle, developers can create more predictable and maintainable code, thus enhancing the overall quality of their software projects.
One of the primary insights derived from this discussion is the importance of clarity in code. When a variable is marked only once, it becomes easier for other developers to understand its purpose and lifecycle within the codebase. This clarity reduces cognitive load and facilitates collaboration, as team members can quickly grasp the intended use of the variable without wading through multiple declarations or modifications. Additionally, this practice aligns with best coding standards that advocate for simplicity and readability.
Another key takeaway is the potential for improved debugging and testing outcomes. By limiting the instances where a variable can be marked, developers can more easily track its value and behavior throughout the execution of the program. This predictability aids in identifying bugs and ensures that tests are more reliable. Overall, marking a variable only once is a best practice that contributes to the robustness and reliability
Author Profile
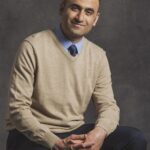
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?