How Can You Effectively Use React Testing Library with Hook Form?
In the ever-evolving landscape of web development, creating seamless user experiences is paramount, and form handling is a critical component of that journey. Enter React Testing Library and React Hook Form—two powerful tools that, when combined, can elevate your form management and testing strategies to new heights. Whether you’re building a simple contact form or a complex multi-step application, mastering these libraries can significantly enhance your workflow and ensure your forms are both robust and user-friendly. In this article, we’ll explore how to effectively integrate React Testing Library with React Hook Form, providing you with the insights needed to streamline your testing processes.
As developers, we are often faced with the challenge of ensuring that our forms are not only functional but also thoroughly tested. React Hook Form simplifies form state management, allowing for easy validation and submission handling. However, the question arises: how do we ensure that our forms are working as intended? This is where React Testing Library comes into play. By focusing on testing components from the user’s perspective, it allows developers to write more meaningful tests that reflect real-world usage.
In the following sections, we will delve into the synergy between these two libraries, highlighting best practices for setting up tests, handling form submissions, and validating user inputs. By the end of this article
Integrating React Testing Library with Hook Form
To effectively test forms built with React Hook Form, the React Testing Library (RTL) provides a robust solution. It emphasizes testing the component from the user’s perspective, ensuring that the application behaves as expected in a real-world scenario. By leveraging RTL, developers can create comprehensive tests for forms that utilize React Hook Form’s functionalities.
Setting Up Your Testing Environment
Before diving into testing, ensure that your environment is set up correctly. Install the necessary packages if you haven’t already:
“`bash
npm install @testing-library/react @testing-library/jest-dom react-hook-form
“`
This setup enables you to utilize the full power of RTL alongside React Hook Form.
Creating a Sample Form Component
Consider a simple form component that utilizes React Hook Form for managing form state and validation. Here’s an example:
“`javascript
import React from ‘react’;
import { useForm } from ‘react-hook-form’;
const SampleForm = ({ onSubmit }) => {
const { register, handleSubmit, formState: { errors } } = useForm();
return (
);
};
export default SampleForm;
“`
This component captures a username and displays an error message if the field is left empty.
Writing Tests for the Form
When testing this component, you want to ensure that it behaves correctly when users interact with it. Below is an example of how to write tests using RTL:
“`javascript
import React from ‘react’;
import { render, screen, fireEvent } from ‘@testing-library/react’;
import SampleForm from ‘./SampleForm’;
test(‘renders form and submits with valid data’, () => {
const handleSubmit = jest.fn();
render(
const input = screen.getByPlaceholderText(/username/i);
fireEvent.change(input, { target: { value: ‘JohnDoe’ } });
fireEvent.click(screen.getByText(/submit/i));
expect(handleSubmit).toHaveBeenCalledWith({ username: ‘JohnDoe’ });
});
test(‘shows error message on empty submit’, () => {
const handleSubmit = jest.fn();
render(
fireEvent.click(screen.getByText(/submit/i));
expect(screen.getByText(/this field is required/i)).toBeInTheDocument();
});
“`
These tests verify that:
- The form submits the correct data when filled out.
- An error message appears when the form is submitted without required fields.
Best Practices for Testing with RTL and Hook Form
When writing tests for forms using React Hook Form and RTL, consider the following best practices:
- Use meaningful test descriptions: Clearly describe what each test is verifying to maintain readability.
- Test user interactions: Simulate real user behavior, such as typing and clicking buttons.
- Check for accessibility: Ensure that all elements are accessible and that error messages are appropriately displayed.
Common Pitfalls to Avoid
While testing forms, there are some common pitfalls to be aware of:
Pitfall | Description |
---|---|
Not using `waitFor` | When testing asynchronous actions, use `waitFor` to handle updates. |
Forgetting cleanup | Ensure you clean up after each test to avoid side effects. |
Overly complicated tests | Keep tests focused on one behavior or user interaction. |
By adhering to these guidelines and practices, you can create effective tests that enhance the reliability of your forms built with React Hook Form and ensure a positive user experience.
Integrating React Testing Library with React Hook Form
When testing forms built with React Hook Form, the React Testing Library provides powerful tools to facilitate interaction and validation checks. The combination of these libraries allows for efficient and readable tests.
Setting Up the Test Environment
Before diving into tests, ensure you have the necessary dependencies installed:
“`bash
npm install @testing-library/react @testing-library/user-event react-hook-form
“`
This setup allows you to leverage the testing capabilities of React Testing Library along with the functionalities of React Hook Form.
Creating a Sample Form Component
Consider a simple form component utilizing React Hook Form:
“`javascript
import React from ‘react’;
import { useForm } from ‘react-hook-form’;
const SampleForm = ({ onSubmit }) => {
const { register, handleSubmit } = useForm();
return (
);
};
export default SampleForm;
“`
This component accepts an `onSubmit` function and registers a single input field for `name`.
Writing Tests for the Form Component
To test the `SampleForm` component, create a corresponding test file:
“`javascript
import React from ‘react’;
import { render, screen, fireEvent } from ‘@testing-library/react’;
import SampleForm from ‘./SampleForm’;
test(‘submits form with name’, async () => {
const handleSubmit = jest.fn();
render(
const input = screen.getByPlaceholderText(‘Name’);
fireEvent.change(input, { target: { value: ‘John Doe’ } });
fireEvent.click(screen.getByText(‘Submit’));
expect(handleSubmit).toHaveBeenCalledWith({ name: ‘John Doe’ });
});
“`
This test checks if the form submits the correct data when filled out.
Handling Validation Errors
To validate form inputs, you can extend the form component and test for error messages:
“`javascript
import React from ‘react’;
import { useForm } from ‘react-hook-form’;
const SampleForm = ({ onSubmit }) => {
const { register, handleSubmit, formState: { errors } } = useForm();
return (
);
};
“`
In this case, you can add a test for the validation error:
“`javascript
test(‘shows error message when name is empty’, () => {
const handleSubmit = jest.fn();
render(
fireEvent.click(screen.getByText(‘Submit’));
expect(screen.getByText(‘This field is required’)).toBeInTheDocument();
});
“`
Using User Events for More Realistic Interactions
Utilizing `@testing-library/user-event` can simulate user interactions more realistically than `fireEvent`. Here’s how to implement it:
“`javascript
import userEvent from ‘@testing-library/user-event’;
test(‘submits form with name using userEvent’, async () => {
const handleSubmit = jest.fn();
render(
await userEvent.type(screen.getByPlaceholderText(‘Name’), ‘John Doe’);
await userEvent.click(screen.getByText(‘Submit’));
expect(handleSubmit).toHaveBeenCalledWith({ name: ‘John Doe’ });
});
“`
By employing `userEvent`, the tests can more closely mimic actual user behavior.
Utilizing React Testing Library in conjunction with React Hook Form provides a robust approach for testing forms. By focusing on user interactions and validating form submissions, you can ensure that your forms operate as intended.
Expert Insights on Using React Testing Library with Hook Form
“Emily Carter (Senior Software Engineer, DevSolutions Inc.). Utilizing React Testing Library in conjunction with Hook Form allows developers to create more robust and maintainable forms. The combination of these tools enables effective testing of user interactions, ensuring that form validations and submissions work as intended.”
“Michael Tran (Frontend Development Lead, CodeCraft Labs). The integration of React Testing Library with Hook Form simplifies the testing process significantly. By leveraging the library’s focus on user-centric testing, developers can simulate real-world scenarios, which leads to higher confidence in the form’s functionality and user experience.”
“Samantha Lee (Technical Writer, React Insights). When testing forms built with Hook Form, React Testing Library provides an excellent framework for ensuring that all aspects of user input are covered. The declarative nature of both tools promotes clarity and reduces the likelihood of errors during testing.”
Frequently Asked Questions (FAQs)
What is React Testing Library?
React Testing Library is a testing utility for React applications that encourages testing components in a way that resembles how users interact with them. It focuses on testing the behavior of the application rather than the implementation details.
How does React Hook Form integrate with React Testing Library?
React Hook Form can be tested using React Testing Library by simulating user interactions with form elements. You can use methods like `fireEvent` or `userEvent` to trigger events and validate form submissions.
What are the best practices for testing forms with React Hook Form and React Testing Library?
Best practices include testing the form’s initial state, validating input fields, ensuring error messages display correctly, and verifying that form submission behaves as expected. Use `waitFor` to handle asynchronous validation or API calls.
Can I test validation rules in React Hook Form using React Testing Library?
Yes, you can test validation rules by simulating user input that violates the rules and asserting that the appropriate error messages are displayed. Use `getByText` or `queryByText` to check for these messages.
How do I handle asynchronous operations in tests with React Hook Form?
To handle asynchronous operations, use `waitFor` or `findBy` queries provided by React Testing Library. These methods allow you to wait for the expected changes in the DOM after an asynchronous action, such as fetching data or validating inputs.
What tools can complement React Testing Library when testing forms?
Complementary tools include Jest for assertions and mocking, and user-event for simulating user interactions more realistically. You can also use libraries like msw (Mock Service Worker) for handling API requests during testing.
The integration of React Testing Library with React Hook Form provides a powerful combination for testing form components in React applications. React Hook Form simplifies form management by minimizing the amount of state management required, while React Testing Library offers a user-centric approach to testing components. This synergy allows developers to create robust tests that ensure forms behave as expected under various scenarios.
One of the key takeaways from the discussion is the importance of simulating user interactions when testing forms. By leveraging the capabilities of React Testing Library, developers can effectively simulate user inputs, validate form submissions, and check for error messages. This approach not only enhances the reliability of tests but also aligns with best practices in testing by focusing on how users actually interact with the application.
Additionally, utilizing the `useForm` hook from React Hook Form in conjunction with the testing library allows for more streamlined and maintainable test code. Developers can easily set up forms in their tests, manage form state, and assert outcomes without the overhead of complex setups. This leads to faster development cycles and improved code quality, making it easier to catch issues early in the development process.
the combination of React Testing Library and React Hook Form offers a comprehensive solution for testing form components. By focusing
Author Profile
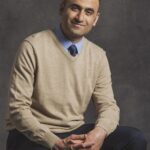
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?