How Can You Check if a Key Exists in a Node JSON Object?
In the world of web development, working with JSON (JavaScript Object Notation) is nearly unavoidable. This lightweight data interchange format is widely used for transmitting data between a server and a web application, making it a staple in modern programming. However, as developers dive deeper into the intricacies of JSON, they often encounter a common challenge: how to efficiently check if a specific key exists within a JSON object. This seemingly simple task can have significant implications for data validation, error handling, and overall application performance.
Understanding how to verify the existence of keys in JSON not only enhances your coding efficiency but also helps you maintain cleaner, more reliable code. Whether you’re parsing API responses or managing configuration files, knowing how to check for keys can prevent potential bugs and streamline your data manipulation processes. With JavaScript’s flexible syntax and powerful built-in methods, developers have a range of tools at their disposal to tackle this challenge effectively.
In this article, we will explore various techniques to check if a key exists in a JSON object using Node.js. From leveraging simple conditional statements to utilizing more advanced methods, we will provide insights that cater to both novice and experienced developers alike. By the end, you’ll be equipped with the knowledge to handle key existence checks seamlessly, ensuring your applications run smoothly and efficiently
Checking for Key Existence in JSON Objects
In JavaScript, particularly when working with Node.js, determining whether a specific key exists in a JSON object is a common task. JSON objects are essentially JavaScript objects, and therefore, they can be manipulated using standard JavaScript techniques.
To check if a key exists in a JSON object, you can use several approaches, each with its own advantages. Below are some of the most commonly used methods:
- Using the `in` operator: This operator checks if the specified property is in the object or its prototype chain.
- Using `hasOwnProperty()` method: This method checks if the object has the specified property as its own (not inherited).
- Using `Object.keys()`: This method retrieves an array of a given object’s own enumerable property names, and you can check if the key exists within that array.
Examples of Checking Key Existence
Here are examples demonstrating each of these methods:
“`javascript
const jsonObject = {
name: “Alice”,
age: 30,
city: “New York”
};
// Method 1: Using the ‘in’ operator
if (‘age’ in jsonObject) {
console.log(“Key ‘age’ exists in jsonObject.”);
}
// Method 2: Using hasOwnProperty
if (jsonObject.hasOwnProperty(‘city’)) {
console.log(“Key ‘city’ exists in jsonObject.”);
}
// Method 3: Using Object.keys()
if (Object.keys(jsonObject).includes(‘name’)) {
console.log(“Key ‘name’ exists in jsonObject.”);
}
“`
Comparison of Methods
Method | Description | Returns |
---|---|---|
`in` operator | Checks if a property exists in the object or its prototype. | Boolean |
`hasOwnProperty()` | Checks if a property is a direct property of the object. | Boolean |
`Object.keys()` | Retrieves an array of the object’s own keys, checks for existence. | Boolean |
Performance Considerations
When deciding on which method to use, consider the following performance aspects:
- `in` Operator: Fast and efficient for checking both own and inherited properties. However, it may return true for properties in the prototype chain, which could lead to unexpected results if inherited properties are not desirable.
- `hasOwnProperty()`: Generally more reliable when you want to ensure that the key is a direct property of the object. This method is slightly slower than the `in` operator but provides more accurate results for most applications.
- `Object.keys()`: Useful for when you need to work with the keys of an object, but it involves creating an array, which may introduce additional overhead, especially for large objects.
By using these methods, developers can efficiently check for the existence of keys in JSON objects while understanding the implications of each approach. The choice of method should align with the specific requirements of the application and the structure of the data being handled.
Checking for Key Existence in JSON Objects Using Node.js
In Node.js, you can easily check if a key exists within a JSON object by utilizing various methods. Here are the most common approaches:
Using the `in` Operator
The `in` operator is a straightforward way to determine if a property exists in an object. This method checks for the existence of the property in the object itself or in its prototype chain.
“`javascript
const jsonObject = {
name: “Alice”,
age: 30,
city: “New York”
};
console.log(‘age’ in jsonObject); // true
console.log(‘gender’ in jsonObject); //
“`
Using `hasOwnProperty` Method
The `hasOwnProperty` method is another effective way to check for a key’s presence. This method only returns true if the property exists directly on the object, not inherited through the prototype chain.
“`javascript
console.log(jsonObject.hasOwnProperty(‘city’)); // true
console.log(jsonObject.hasOwnProperty(‘country’)); //
“`
Using Optional Chaining and Logical Operators
For nested JSON objects, optional chaining can be particularly useful. It allows you to safely access deeply nested properties without throwing an error if a key is missing.
“`javascript
const nestedJsonObject = {
user: {
name: “Bob”,
address: {
city: “Los Angeles”
}
}
};
console.log(nestedJsonObject.user?.address?.city !== ); // true
console.log(nestedJsonObject.user?.address?.state !== ); //
“`
Using `Object.keys` Method
You can also use `Object.keys()` to obtain an array of an object’s keys and then check for the existence of the key using the `includes` method.
“`javascript
const keys = Object.keys(jsonObject);
console.log(keys.includes(‘name’)); // true
console.log(keys.includes(’email’)); //
“`
Performance Considerations
When selecting a method for checking key existence, consider the following:
Method | Performance | Inherited Properties | Safe for Nested Objects |
---|---|---|---|
`in` | Fast | Yes | No |
`hasOwnProperty` | Fast | No | No |
Optional Chaining | Moderate | No | Yes |
`Object.keys` | Moderate | No | No |
Practical Use Cases
- Validation: Ensure required fields are present before processing data.
- Conditional Logic: Execute specific code paths based on the existence of keys.
- Dynamic Property Access: Safely access properties without risking runtime errors.
Each of these methods serves specific needs in the context of working with JSON objects in Node.js, allowing for versatile and efficient coding practices.
Expert Insights on Checking Key Existence in Node JSON
Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Node.js, checking if a key exists in a JSON object can be efficiently done using the `hasOwnProperty` method. This approach ensures that you are only checking for properties that belong directly to the object and not its prototype chain, which can prevent potential bugs in your application.”
James Liu (Lead Developer, Node.js Community). “When working with JSON data in Node.js, utilizing the `in` operator provides a straightforward way to verify key existence. However, developers should be cautious as this operator checks both own properties and inherited properties, which may lead to unexpected results if not properly managed.”
Maria Gonzalez (Full Stack Developer, CodeCraft Solutions). “For a more modern approach, using optional chaining in combination with the nullish coalescing operator can simplify the process of checking for key existence in deeply nested JSON objects. This method enhances code readability and reduces the risk of runtime errors when accessing properties.”
Frequently Asked Questions (FAQs)
How can I check if a key exists in a JSON object in Node.js?
You can check if a key exists in a JSON object by using the `in` operator or the `hasOwnProperty` method. For example, `if (‘keyName’ in jsonObject)` or `if (jsonObject.hasOwnProperty(‘keyName’))` will return true if the key exists.
What is the difference between using `in` and `hasOwnProperty`?
The `in` operator checks for the existence of a key in the entire prototype chain, while `hasOwnProperty` checks only for the key in the object itself, ignoring inherited properties.
Can I check for nested keys in a JSON object?
Yes, to check for nested keys, you can use a combination of dot notation or bracket notation. For example, `if (jsonObject.parentKey && ‘childKey’ in jsonObject.parentKey)` verifies the existence of a nested key.
What happens if I check for a key that does not exist?
If you check for a key that does not exist using either the `in` operator or `hasOwnProperty`, it will return , indicating that the key is not present in the object.
Is there a method to safely check for keys in deeply nested JSON structures?
Yes, you can create a utility function that traverses the JSON object to check for keys at any depth. Libraries like Lodash provide functions such as `_.get` to safely access nested properties.
Can I use optional chaining to check for key existence?
Yes, optional chaining (e.g., `jsonObject?.keyName`) allows you to safely access properties without throwing an error if the key does not exist, returning “ instead.
In the context of working with JSON objects in Node.js, checking for the existence of a key is a fundamental operation that developers frequently encounter. JSON, which stands for JavaScript Object Notation, is a lightweight data interchange format that is easy for humans to read and write, and easy for machines to parse and generate. In Node.js, JSON objects are typically represented as JavaScript objects, allowing developers to leverage JavaScript’s inherent capabilities to interact with these data structures effectively.
To determine if a key exists within a JSON object in Node.js, developers can utilize several methods. The most common approach is to use the `in` operator, which checks for the presence of a specified key within an object. Alternatively, the `hasOwnProperty` method can be employed, which verifies if the object has the specified property as its own (not inherited). Both methods are straightforward and provide reliable results, making them essential tools in a developer’s toolkit when handling JSON data.
Understanding how to check for key existence in JSON objects is crucial for error handling and data validation. This capability allows developers to write more robust applications that can gracefully handle situations where expected data may be missing. By incorporating these practices, developers can enhance the reliability and maintainability
Author Profile
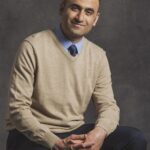
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?