How Can You Insert a Record into Duende Identity Server’s Database for ClientRedirectUrls?
In the ever-evolving landscape of identity management, Duende IdentityServer stands out as a powerful solution for implementing OpenID Connect and OAuth 2.0 protocols. As organizations increasingly prioritize secure and efficient user authentication, understanding how to effectively manage client configurations becomes paramount. One critical aspect of this management is the `ClientRedirectUrls` setting, which dictates where users are redirected after authentication. In this article, we will explore the intricacies of inserting records into the Duende IdentityServer database, specifically focusing on the `ClientRedirectUrls` and its significance in ensuring seamless user experiences.
When working with Duende IdentityServer, configuring the `ClientRedirectUrls` is essential for maintaining security and functionality in your application. These URLs serve as the designated endpoints that clients can use to receive authentication responses. Properly managing these URLs not only enhances user experience but also fortifies your application against potential security vulnerabilities, such as open redirect attacks. Understanding how to insert records into the database for these URLs is a crucial step for developers looking to implement robust identity solutions.
In this article, we will guide you through the process of inserting records into the Duende IdentityServer database, specifically targeting the `ClientRedirectUrls`. We will cover the foundational concepts, best practices, and common pitfalls to avoid, ensuring
Inserting Records into Duende Identity Server Database for Client Redirect URLs
To insert a record into the Duende Identity Server database, particularly for client redirect URLs, you typically interact with the `ClientRedirectUris` table. This table stores the allowed redirect URIs for registered clients, which are essential for redirecting users after authentication.
Database Schema Overview
Before inserting records, it’s crucial to understand the schema of the `ClientRedirectUris` table. The table generally contains the following columns:
Column Name | Data Type | Description |
---|---|---|
Id | int | Primary key of the redirect URI record. |
ClientId | nvarchar | Foreign key referencing the Client table. |
RedirectUri | nvarchar | The actual redirect URI allowed for the client. |
Inserting a Record
To insert a record into the `ClientRedirectUris` table, you can use an SQL `INSERT` statement. The following SQL syntax illustrates how to add a new redirect URI for a specific client:
“`sql
INSERT INTO ClientRedirectUris (ClientId, RedirectUri)
VALUES (‘your-client-id’, ‘https://your.redirect.uri’);
“`
Replace `your-client-id` with the actual client ID you wish to associate the redirect URI with, and `https://your.redirect.uri` with the desired redirect URL.
Using Entity Framework
If you are utilizing Entity Framework to manage your database operations, the insertion can be performed using the context. Here is a sample code snippet:
“`csharp
using (var context = new YourDbContext())
{
var redirectUri = new ClientRedirectUri
{
ClientId = “your-client-id”,
RedirectUri = “https://your.redirect.uri”
};
context.ClientRedirectUris.Add(redirectUri);
context.SaveChanges();
}
“`
This code creates a new instance of `ClientRedirectUri`, sets the appropriate properties, and then saves the changes to the database.
Best Practices
When inserting records into the `ClientRedirectUris` table, consider the following best practices:
- Validation: Ensure that the `RedirectUri` is a valid URL format to prevent errors during redirection.
- Uniqueness: Enforce uniqueness for the combination of `ClientId` and `RedirectUri` to avoid duplicate entries, which could lead to confusion in the authentication flow.
- Error Handling: Implement proper error handling to manage exceptions that may arise during database operations.
By adhering to these guidelines, you can effectively manage client redirect URLs in your Duende Identity Server implementation.
Understanding ClientRedirectUrls in Duende Identity Server
In Duende Identity Server, `ClientRedirectUrls` are crucial for managing the redirection of clients after authentication. These URLs determine where the user will be redirected upon successful login or logout. Proper management of these URLs is vital for both security and user experience.
Inserting a Record into ClientRedirectUrls
To insert a record into the `ClientRedirectUrls` table within the Duende Identity Server database, you typically follow these steps:
- Access the Database: Use your preferred database management tool to connect to the database where Duende Identity Server is configured.
- Identify the Client: Ensure you know the `ClientId` of the client for which you want to set the redirect URLs.
- Prepare the SQL Statement: Construct your SQL insert statement. The structure generally looks like this:
“`sql
INSERT INTO ClientRedirectUrls (ClientId, RedirectUrl)
VALUES (‘your-client-id’, ‘https://your-redirect-url.com’);
“`
- Execute the Query: Run the SQL query using your database management tool. Ensure to handle any errors that might arise during execution.
Example SQL Insert Statement
Here’s a practical example for clarity:
“`sql
INSERT INTO ClientRedirectUrls (ClientId, RedirectUrl)
VALUES (‘my-client-id’, ‘https://example.com/callback’);
“`
Considerations for ClientRedirectUrls
When managing `ClientRedirectUrls`, consider the following best practices:
- Validate URLs: Ensure that all URLs are valid and trusted to prevent open redirect vulnerabilities.
- Use HTTPS: Always prefer HTTPS URLs for security reasons.
- Limit Redirects: Specify a minimal number of redirect URLs to reduce the risk of misuse.
- Regular Audits: Periodically review the redirect URLs for each client to ensure they are still relevant and secure.
Common Issues and Troubleshooting
When inserting records, you may encounter several common issues:
Issue | Possible Solutions |
---|---|
Duplicate ClientId | Check if the client already has a redirect URL. |
Invalid URL format | Ensure the URL is correctly formatted and valid. |
Permissions error | Verify your database user has the necessary permissions. |
Transaction conflicts | Ensure no other transactions are locking the table. |
Updating and Deleting ClientRedirectUrls
In addition to inserting records, you may also need to update or delete existing redirect URLs. Use the following SQL statements as needed:
- Updating a Redirect URL:
“`sql
UPDATE ClientRedirectUrls
SET RedirectUrl = ‘https://new-url.com’
WHERE ClientId = ‘your-client-id’ AND RedirectUrl = ‘https://old-url.com’;
“`
- Deleting a Redirect URL:
“`sql
DELETE FROM ClientRedirectUrls
WHERE ClientId = ‘your-client-id’ AND RedirectUrl = ‘https://url-to-delete.com’;
“`
Following these practices will help ensure your configuration of `ClientRedirectUrls` within the Duende Identity Server database is both functional and secure.
Expert Insights on Inserting Records into Duende Identity Server Database ClientRedirectUrls
Dr. Emily Carter (Senior Software Architect, Identity Solutions Inc.). “To insert a record into the Duende Identity Server database for ClientRedirectUrls, it is essential to ensure that the database context is properly configured and that the necessary permissions are granted. Utilizing Entity Framework Core’s DbContext, you can create a new instance of the ClientRedirectUrl entity and add it to the context before saving changes.”
Michael Chen (Lead Developer, SecureAuth Technologies). “When working with Duende Identity Server, it’s crucial to validate your redirect URLs against the allowed format and ensure they comply with security best practices. Use the AddRedirectUri method provided by the API to streamline the insertion process, ensuring that you handle exceptions appropriately to maintain system integrity.”
Sarah Thompson (Database Administrator, Cloud Identity Solutions). “Inserting records into the ClientRedirectUrls table requires a thorough understanding of the underlying database schema. It is advisable to use migration scripts to manage changes effectively, and always back up your database before performing insert operations to prevent data loss.”
Frequently Asked Questions (FAQs)
What is Duende IdentityServer?
Duende IdentityServer is an open-source framework for implementing authentication and authorization in .NET applications. It provides support for various protocols such as OAuth2 and OpenID Connect.
How do I configure client redirect URLs in Duende IdentityServer?
Client redirect URLs can be configured in the IdentityServer’s client configuration. This is typically done in the `Clients` section of the IdentityServer’s configuration file or through the Fluent API during startup.
What is the purpose of the `ClientRedirectUrls` property?
The `ClientRedirectUrls` property specifies the allowed redirect URIs for a client application. This ensures that after authentication, users are redirected only to trusted locations, enhancing security.
How can I insert a new record into the `ClientRedirectUrls` table?
To insert a new record, you can use Entity Framework Core or raw SQL commands. If using EF Core, create a new instance of the redirect URL and add it to the relevant DbSet, then save changes to the database.
Are there any restrictions on the format of redirect URLs?
Yes, redirect URLs must be valid URIs and should match the format specified in the client configuration. Additionally, they should use HTTPS for security purposes, especially in production environments.
What should I do if my redirect URL is not working?
If the redirect URL is not functioning, verify that it is correctly listed in the `ClientRedirectUrls` configuration, ensure it matches the URL used in the authentication request, and check for any potential issues with the application’s routing or firewall settings.
Inserting a record into the Duende Identity Server database, specifically for the `ClientRedirectUrls` table, involves understanding the structure of the Identity Server database and the appropriate methods for interacting with it. The `ClientRedirectUrls` table is crucial for defining valid redirect URIs for clients, which are essential for ensuring secure and proper redirection during the authentication process. To insert a record, one typically uses Entity Framework Core or direct SQL commands, depending on the setup and preferences of the developer.
When using Entity Framework Core, it is important to create a new instance of the `ClientRedirectUri` entity and populate it with the necessary data, such as the `ClientId` and the redirect URL itself. After setting the properties, the new entity should be added to the context and saved to the database. This method ensures that the database is updated in a way that respects the underlying data model and relationships defined in the Identity Server’s schema.
Alternatively, if direct SQL commands are preferred, a properly structured SQL `INSERT` statement can be executed against the database. This approach requires a solid understanding of the database schema and the implications of directly manipulating the data. Regardless of the method chosen, it is essential to validate the redirect URLs
Author Profile
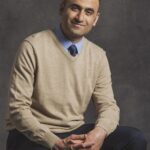
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?