What Is an Attribute Error in Python and How Can You Fix It?
### Understanding Attribute Errors in Python: A Guide for Developers
As you delve into the world of Python programming, you may encounter a variety of challenges that can stump even the most seasoned developers. One such hurdle is the notorious “AttributeError.” This common exception can arise unexpectedly, leaving you puzzled and searching for answers. Understanding what an AttributeError signifies and how to troubleshoot it is crucial for anyone looking to write efficient and error-free code. In this article, we will explore the nuances of this error, helping you to not only identify its causes but also to implement effective strategies for resolution.
An AttributeError occurs when you attempt to access an attribute or method of an object that does not exist. This can happen for several reasons, such as a typo in the attribute name, trying to access an attribute from an object of the wrong type, or simply using an object that has not been properly initialized. The error message generated by Python provides insights into the nature of the problem, but deciphering it can be daunting for beginners.
By gaining a deeper understanding of AttributeErrors, you will enhance your debugging skills and improve the robustness of your code. Whether you are a novice programmer or a seasoned coder, recognizing the common pitfalls that lead to this error will empower you to write cleaner, more
Understanding AttributeError in Python
An `AttributeError` in Python is raised when an invalid attribute reference is made, typically when an object does not have the attribute being accessed. This error indicates that the code is attempting to access or call an attribute that is not defined for that particular object or class.
The following are common scenarios that lead to an `AttributeError`:
- Trying to access a method or attribute that does not exist on an object.
- Attempting to call a method on an object that is of a different type than expected.
- Misspelling the attribute name.
- Using a variable that has not been initialized or defined properly.
Common Causes of AttributeError
To understand how `AttributeError` can occur, consider the following examples:
- Accessing Non-existent Attributes: If you try to access an attribute that hasn’t been defined in a class instance, Python raises an `AttributeError`.
python
class MyClass:
def __init__(self):
self.name = “Example”
obj = MyClass()
print(obj.age) # Raises AttributeError
- Misspelling Attributes: A simple typo can lead to this error.
python
class MyClass:
def __init__(self):
self.value = 10
obj = MyClass()
print(obj.vlaue) # Raises AttributeError due to a typo
- Incorrect Object Types: Calling a method or attribute on an object of the wrong type can lead to an `AttributeError`.
python
my_list = [1, 2, 3]
my_list.append(4)
print(my_list.size()) # Raises AttributeError; ‘list’ object has no attribute ‘size’
How to Handle AttributeError
Handling `AttributeError` can be done through several approaches:
- Use Try-Except Blocks: Wrap the code in a try-except block to gracefully handle the error.
python
try:
print(obj.age)
except AttributeError:
print(“Attribute does not exist.”)
- Check for Attribute Existence: Before accessing an attribute, verify if it exists using the built-in `hasattr()` function.
python
if hasattr(obj, ‘age’):
print(obj.age)
else:
print(“Attribute ‘age’ not found.”)
- Debugging Techniques: When encountering an `AttributeError`, utilize debugging techniques such as printing the object’s `__dict__` or using the `dir()` function to inspect its available attributes.
python
print(dir(obj)) # Lists all attributes and methods of the object
Scenario | Example Code | Result |
---|---|---|
Accessing Non-existent Attribute | print(obj.age) |
AttributeError |
Misspelled Attribute | print(obj.vlaue) |
AttributeError |
Incorrect Method Call | my_list.size() |
AttributeError |
By understanding the nature of `AttributeError`, developers can write more robust and error-resistant code. This knowledge allows for effective debugging and enhances overall code quality.
Understanding AttributeError in Python
AttributeError is a common exception in Python that occurs when an invalid attribute reference is made. This typically happens when you try to access an attribute or method of an object that doesn’t exist.
Common Causes of AttributeError
Several scenarios can lead to an AttributeError:
- Misspelled Attribute Name: A simple typographical error can cause the error.
- Nonexistent Method or Property: Attempting to call a method or access a property that is not defined in the class.
- Object Type Mismatch: Trying to access an attribute from an object that is of a different type than expected.
- Scope Issues: Accessing an attribute that has not been defined in the current scope or context.
Examples of AttributeError
Below are examples illustrating how AttributeError can arise:
python
class Dog:
def bark(self):
return “Woof!”
my_dog = Dog()
# Example 1: Misspelled method name
print(my_dog.brk()) # Raises AttributeError: ‘Dog’ object has no attribute ‘brk’
# Example 2: Accessing a nonexistent attribute
print(my_dog.age) # Raises AttributeError: ‘Dog’ object has no attribute ‘age’
How to Handle AttributeError
Handling AttributeError can be done effectively using try-except blocks. Here’s how you can implement this:
python
try:
print(my_dog.bark())
except AttributeError as e:
print(f”An error occurred: {e}”)
Additionally, you can use the `hasattr()` function to check for an attribute’s existence before accessing it:
python
if hasattr(my_dog, ‘bark’):
print(my_dog.bark())
else:
print(“The method ‘bark’ does not exist.”)
Best Practices to Avoid AttributeError
To minimize the occurrence of AttributeError, consider the following best practices:
- Use IDE Features: Leverage integrated development environments (IDEs) that provide code completion and suggestions.
- Follow Naming Conventions: Stick to consistent naming conventions to avoid typos.
- Implement Unit Tests: Regularly write unit tests to catch attribute-related errors early in the development process.
- Consult Documentation: Always refer to official documentation when using third-party libraries or frameworks to understand available attributes and methods.
Debugging AttributeError
When you encounter an AttributeError, debugging can be approached systematically:
- Read the Error Message: The message usually includes the object type and the attribute that was attempted to be accessed.
- Check Object Type: Use the `type()` function to verify the object’s type.
- Inspect Available Attributes: Utilize the `dir()` function to list all attributes of the object.
python
print(type(my_dog)) # Check the object’s type
print(dir(my_dog)) # List all attributes and methods of the object
By following these practices, you can effectively manage and troubleshoot AttributeError in your Python applications.
Understanding Attribute Errors in Python Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “An attribute error in Python occurs when you try to access an attribute or method that does not exist for a particular object. This can often be attributed to typos, incorrect object types, or attempting to access attributes before they have been defined.”
Michael Chen (Python Developer and Educator, Code Academy). “Attribute errors are common pitfalls for both novice and experienced Python developers. It is essential to understand the structure of your objects and ensure that the attributes you are trying to access are indeed present. Utilizing debugging tools can greatly assist in identifying the source of these errors.”
Sarah Thompson (Data Scientist, Analytics Solutions). “In data-driven applications, attribute errors can lead to significant disruptions. It is advisable to implement error handling mechanisms, such as try-except blocks, to gracefully manage these exceptions and maintain the robustness of your code.”
Frequently Asked Questions (FAQs)
What is an AttributeError in Python?
An AttributeError in Python occurs when an invalid attribute reference is made to an object. This means that the object does not possess the attribute or method being accessed.
What causes an AttributeError?
An AttributeError can be caused by several factors, including typos in attribute names, attempting to access attributes on objects that do not support them, or using the wrong object type.
How can I troubleshoot an AttributeError?
To troubleshoot an AttributeError, check the spelling of the attribute, ensure that the object is of the correct type, and verify that the attribute exists in the object’s class definition.
Can I handle an AttributeError in Python?
Yes, you can handle an AttributeError using a try-except block. This allows your program to continue running even if an AttributeError occurs, enabling graceful error handling.
Is an AttributeError the same as a NameError?
No, an AttributeError is specific to accessing attributes or methods of an object, while a NameError occurs when a variable or name is not defined in the current scope.
How can I prevent AttributeErrors in my code?
To prevent AttributeErrors, ensure that you are accessing attributes only on objects that are guaranteed to have them, use the `hasattr()` function to check for attributes, and maintain consistent naming conventions in your code.
An AttributeError in Python occurs when an attempt is made to access an attribute or method of an object that does not exist. This error typically arises when the attribute name is misspelled, the object is of a different type than expected, or the attribute has not been defined in the object’s class. Understanding the context in which this error occurs is crucial for effective debugging and ensuring smooth program execution.
When encountering an AttributeError, it is essential to carefully check the object and the attribute being accessed. Utilizing Python’s built-in functions, such as `dir()`, can help list all available attributes and methods of an object, aiding in identifying potential issues. Additionally, reviewing the class definitions and ensuring that the correct object types are being used can prevent such errors from occurring in the first place.
In summary, an AttributeError serves as a reminder of the importance of proper object-oriented programming practices in Python. By maintaining clear and accurate attribute definitions, and by being meticulous with object types and names, developers can minimize the occurrence of this error. Ultimately, understanding and addressing AttributeErrors contributes to the overall robustness and reliability of Python applications.
Author Profile
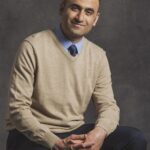
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?