How Can You Write a Matrix in Python? A Step-by-Step Guide
In the realm of programming, matrices are fundamental structures that play a pivotal role in various fields, from data science and machine learning to computer graphics and scientific computing. For those venturing into the world of Python, understanding how to write and manipulate matrices is not just beneficial but essential. Whether you’re working on complex algorithms, performing linear algebra operations, or simply organizing data, mastering matrix representation in Python can elevate your coding skills and broaden your analytical capabilities.
Python offers several ways to create and manage matrices, with libraries like NumPy providing powerful tools for efficient computation and manipulation. At its core, a matrix is simply a two-dimensional array of numbers, and Python’s flexibility allows you to implement matrices in various forms, whether through native lists or specialized data structures. This versatility makes it accessible for beginners while also catering to the needs of seasoned developers looking for optimized performance in their applications.
As we delve deeper into the topic, we will explore the different methods to write a matrix in Python, highlighting the advantages and use cases of each approach. From basic list comprehensions to leveraging advanced libraries, you’ll gain insights that will empower you to handle matrices with confidence and precision. So, whether you’re looking to enhance your programming toolkit or tackle specific mathematical problems, this guide will illuminate the
Creating a Matrix Using Lists
In Python, one of the simplest ways to create a matrix is by using nested lists. A matrix can be represented as a list of lists, where each inner list corresponds to a row in the matrix. This method is straightforward and allows for dynamic resizing.
Example of a 3×3 matrix:
“`python
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
“`
You can access individual elements using indexing:
“`python
element = matrix[1][2] This will return 6
“`
Using NumPy for Matrix Operations
For more advanced operations, the NumPy library is a powerful tool. It provides support for multi-dimensional arrays and matrices, along with a variety of mathematical functions to operate on these arrays.
To create a matrix using NumPy, follow these steps:
- Install NumPy if you haven’t already:
“`bash
pip install numpy
“`
- Import the NumPy library and create a matrix:
“`python
import numpy as np
matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
“`
With NumPy, you can perform various matrix operations efficiently, such as addition, subtraction, and multiplication.
Matrix Operations with NumPy
Here are some common operations you can perform on matrices using NumPy:
- Addition: Adding two matrices of the same shape.
- Subtraction: Subtracting one matrix from another.
- Multiplication: Matrix multiplication (dot product).
- Transpose: Flipping a matrix over its diagonal.
Here’s an example illustrating these operations:
“`python
matrix_a = np.array([[1, 2], [3, 4]])
matrix_b = np.array([[5, 6], [7, 8]])
Addition
add_result = matrix_a + matrix_b
Subtraction
sub_result = matrix_a – matrix_b
Multiplication
mul_result = np.dot(matrix_a, matrix_b)
Transpose
transpose_result = np.transpose(matrix_a)
“`
Matrix Representation in a Table
When displaying a matrix, it can be useful to represent it in a structured format. Here is an example of how a matrix can be represented in a table format:
Column 1 | Column 2 | Column 3 |
---|---|---|
1 | 2 | 3 |
4 | 5 | 6 |
7 | 8 | 9 |
This table visually represents the same matrix created earlier and can be useful for documentation or reporting purposes.
Utilizing these approaches, you can effectively create and manipulate matrices in Python, catering to both simple and complex needs.
Creating a Matrix Using Lists
In Python, one straightforward way to represent a matrix is by using nested lists. Each inner list can represent a row of the matrix. Here is an example of how to create a 2×3 matrix:
“`python
matrix = [
[1, 2, 3],
[4, 5, 6]
]
“`
This matrix can be accessed using indices. For instance, `matrix[0][1]` will return `2`, while `matrix[1][2]` will return `6`.
Creating a Matrix Using NumPy
For more advanced matrix operations, the NumPy library is widely used. It provides a more efficient way to handle matrices and perform mathematical operations. To create a matrix using NumPy, follow these steps:
- Install NumPy if it is not already installed:
“`bash
pip install numpy
“`
- Import NumPy in your script:
“`python
import numpy as np
“`
- Create a matrix:
“`python
matrix = np.array([[1, 2, 3], [4, 5, 6]])
“`
This will create a NumPy array that behaves like a matrix. You can perform operations such as addition, multiplication, and transposition easily.
Creating a Sparse Matrix
In scenarios where the matrix contains a significant number of zeros, using a sparse matrix can be more memory-efficient. The SciPy library provides functionality to create sparse matrices. Here’s how to do it:
- Install SciPy if it is not already installed:
“`bash
pip install scipy
“`
- Import the necessary module:
“`python
from scipy.sparse import csr_matrix
“`
- Create a sparse matrix:
“`python
dense_matrix = [[0, 0, 3], [4, 0, 0], [0, 0, 0]]
sparse_matrix = csr_matrix(dense_matrix)
“`
This representation is efficient for storing large matrices with many zero elements.
Accessing Elements in a Matrix
Accessing elements in both nested lists and NumPy matrices follows similar indexing principles. Here’s a comparison:
Method | Syntax | Example Output |
---|---|---|
Nested Lists | `matrix[row][col]` | `matrix[0][2]` -> `3` |
NumPy Arrays | `matrix[row, col]` | `matrix[0, 2]` -> `3` |
Matrix Operations
Using NumPy, you can easily perform various operations on matrices:
- Addition:
“`python
matrix1 + matrix2
“`
- Multiplication:
“`python
matrix1.dot(matrix2)
“`
- Transposition:
“`python
matrix.T
“`
Each operation is optimized for performance and can handle large datasets effectively.
Expert Insights on Writing Matrices in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “To effectively write a matrix in Python, leveraging libraries like NumPy is essential. NumPy provides a powerful array object that allows for efficient storage and manipulation of matrix data, making it the preferred choice for data scientists.”
Michael Thompson (Software Engineer, CodeCraft Solutions). “When writing matrices in Python, it is crucial to understand the difference between lists and arrays. While lists can be used for basic matrix representation, using NumPy arrays enhances performance and functionality, especially for mathematical operations.”
Lisa Chen (Educator and Python Programming Instructor). “For beginners, it is advisable to start with simple nested lists to understand matrix structure. However, as one progresses, transitioning to NumPy arrays will provide more advanced capabilities such as broadcasting and vectorized operations, which are vital for scientific computing.”
Frequently Asked Questions (FAQs)
How do I create a matrix using lists in Python?
You can create a matrix using nested lists in Python. For example, a 2×2 matrix can be defined as `matrix = [[1, 2], [3, 4]]`.
What libraries can I use to work with matrices in Python?
You can use libraries such as NumPy and SciPy, which provide extensive functionalities for matrix operations and manipulations.
How do I convert a list of lists into a NumPy matrix?
You can convert a list of lists into a NumPy matrix by using `numpy.array()`. For example, `import numpy as np; matrix = np.array([[1, 2], [3, 4]])`.
Can I perform matrix operations using Python’s built-in functions?
While Python’s built-in functions can handle basic operations, using libraries like NumPy is recommended for efficient and comprehensive matrix operations such as addition, multiplication, and inversion.
How do I access elements in a matrix in Python?
You can access elements in a matrix (list of lists) using indexing. For example, `matrix[0][1]` retrieves the element in the first row and second column.
What is the difference between a list of lists and a NumPy array?
A list of lists is a standard Python data structure, while a NumPy array is a specialized object that allows for efficient numerical computations and provides additional functionalities such as broadcasting and vectorization.
In Python, writing a matrix can be accomplished using various methods, each suited for different use cases. The most common approaches involve using nested lists, which are simple and intuitive, or leveraging libraries such as NumPy, which provide more advanced functionalities and efficiency for numerical computations. By utilizing these methods, users can create, manipulate, and perform operations on matrices effectively.
When using nested lists, a matrix can be represented as a list of lists, where each inner list corresponds to a row of the matrix. This approach is straightforward and requires no additional libraries, making it accessible for beginners. However, for more complex operations, such as matrix multiplication or inversion, employing a library like NumPy is recommended. NumPy not only simplifies these operations but also enhances performance due to its optimized implementations.
Furthermore, understanding the dimensionality of matrices is crucial when working with them in Python. Users should be aware of how to access elements, modify rows and columns, and utilize built-in functions for efficient matrix manipulation. Overall, whether one opts for basic nested lists or advanced libraries, Python provides flexible and powerful tools for matrix representation and operations.
Author Profile
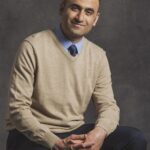
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?