How Can I Resolve the ‘Unexpected Token CJS Folder’ Error with Jest’s ModuleNameMapper?
In the world of JavaScript testing, Jest has emerged as a powerful tool that simplifies the process of writing and running tests. However, as developers dive deeper into their projects, they can encounter some perplexing challenges, particularly when it comes to module resolution and configuration. One such challenge is the error message that states, “jest encountered an unexpected token” when dealing with CommonJS (CJS) modules, often linked to the `moduleNameMapper` configuration. This issue can be frustrating, especially when it disrupts the testing workflow. In this article, we will explore the intricacies of this problem, providing clarity and solutions to help you navigate these murky waters.
When working with Jest, the `moduleNameMapper` feature plays a crucial role in directing how modules are resolved during testing. It allows developers to create aliases for modules, making it easier to manage dependencies and improve code readability. However, misconfigurations or incompatibilities with CJS modules can lead to unexpected token errors, which can halt your testing process. Understanding the underlying causes of these errors is essential for maintaining a smooth development experience.
As we delve deeper into this topic, we will unpack the common scenarios that lead to the “unexpected token” error, especially in relation to CJS folders and
Understanding Module Name Mapping in Jest
When using Jest for testing JavaScript applications, you may encounter issues related to module name mapping, particularly when dealing with CommonJS modules. The `moduleNameMapper` configuration in Jest allows you to specify how modules should be resolved during testing. This is particularly useful for handling paths or aliases that may differ between your development environment and the testing environment.
Common issues arise when Jest encounters unexpected tokens, often due to misconfigured mappings or incompatibilities in module formats. The errors can manifest when attempting to import modules defined in a CommonJS format, especially if they are located in a folder that Jest does not properly resolve.
To configure `moduleNameMapper`, you typically add an entry to your Jest configuration file (like `jest.config.js`). A basic example is shown below:
“`javascript
module.exports = {
moduleNameMapper: {
‘^@components/(.*)$’: ‘
},
};
“`
In this example, `@components` is mapped to the actual path where the components reside. This enables you to use cleaner import statements in your tests.
Common Causes of Unexpected Token Errors
Unexpected token errors can arise from several common scenarios:
- Incompatible Module Formats: If your project contains a mix of ES modules and CommonJS modules, Jest may struggle to parse them correctly.
- Incorrect Configuration: Errors in the `moduleNameMapper` configuration can lead to modules not being found or loaded improperly.
- Transpilation Issues: If using Babel or TypeScript, improper configurations can result in Jest not correctly interpreting the syntax.
To identify the specific cause, consider using the following troubleshooting steps:
- Check Jest Configuration: Ensure that your `jest.config.js` is properly set up and that any paths in `moduleNameMapper` are correct.
- Review Transpilation Settings: Make sure that your Babel or TypeScript configurations support the module formats being used.
- Inspect the Module Imports: Verify that the imports in your test files are correctly pointing to the intended modules.
Example of Resolving Errors with Module Name Mapping
Suppose you have the following directory structure:
“`
/project
/src
/components
MyComponent.cjs
/utils
helper.js
/tests
MyComponent.test.js
“`
If you encounter an unexpected token error when trying to test `MyComponent`, you may need to adjust your Jest configuration.
Here’s how you might configure `moduleNameMapper`:
“`javascript
module.exports = {
moduleNameMapper: {
‘^@utils/(.*)$’: ‘
‘^@components/(.*)$’: ‘
},
};
“`
This setup allows you to import components and utilities using:
“`javascript
import MyComponent from ‘@components/MyComponent.cjs’;
import { helperFunction } from ‘@utils/helper’;
“`
Handling Different Module Formats
When working with various module formats, it is critical to ensure that Jest can understand both CommonJS and ES module syntax. Here is a concise comparison of handling these formats:
Module Type | Syntax | Jest Handling |
---|---|---|
CommonJS | const module = require(‘module’); | Ensure Babel is configured for CommonJS |
ES Modules | import module from ‘module’; | Use Babel or set `transform` in Jest config |
By ensuring that your module paths are correctly mapped and that your Jest configuration supports the necessary syntax, you can effectively resolve unexpected token errors and streamline your testing process.
Understanding the Jest Configuration
When encountering the error message related to `jest modulenamemapper` and the unexpected token in the CommonJS (CJS) folder, it is essential to examine your Jest configuration. This typically arises when Jest attempts to handle module imports that are not appropriately configured.
- Check the Jest Configuration File: Ensure that your `jest.config.js` (or `jest.config.ts`) file is correctly set up to handle module resolution. The `moduleNameMapper` property can be adjusted to map module paths appropriately.
“`javascript
module.exports = {
moduleNameMapper: {
‘^@/(.*)$’: ‘
},
};
“`
- Transpilation Settings: If you are using TypeScript or Babel, ensure that your transpilation settings are correctly applied. This may involve using `ts-jest` or `babel-jest` depending on your setup.
Troubleshooting the Unexpected Token Error
The unexpected token error often indicates an issue with how Jest is interpreting the files in your project. Here are steps to troubleshoot:
- Verify File Extensions: Ensure that the files you are testing have the correct extensions (`.js`, `.ts`, etc.). If you’re using `.cjs` files, they might not be processed correctly depending on your configuration.
- Update Dependencies: Ensure that Jest and related packages are up to date. Mismatched versions can lead to unexpected behavior.
- Check Babel and TypeScript Configuration: Ensure that your Babel (`.babelrc` or `babel.config.js`) and TypeScript (`tsconfig.json`) configurations are set up to compile the necessary file types.
- Use `transform` Property: If you are working with non-standard JavaScript files, ensure you are using the `transform` property to specify how Jest should handle these files.
“`javascript
transform: {
‘^.+\\.jsx?$’: ‘babel-jest’,
‘^.+\\.tsx?$’: ‘ts-jest’,
},
“`
Common Causes of the Error
The following are common scenarios that may lead to the encountered error:
Issue | Description |
---|---|
Misconfigured `moduleNameMapper` | Incorrect paths can cause Jest to fail when trying to resolve modules. |
Unsupported file types | Jest may not recognize `.cjs` files if not configured to handle them explicitly. |
Incorrect Jest version | Using an outdated version of Jest that does not support certain features or configurations. |
Missing dependencies | Failing to install necessary dependencies like `babel-jest` or `ts-jest` can lead to errors. |
Best Practices for Jest Configuration
To avoid similar issues in the future, consider the following best practices:
- Consistent Module Resolution: Use a consistent way to resolve modules across your application to minimize conflicts.
- Documentation Review: Regularly check Jest’s official documentation for updates on configuration options and breaking changes.
- Testing Environment: Maintain a clean testing environment by utilizing tools like `npm ci` to ensure that the installed packages are consistent with the `package-lock.json`.
- Clear Cache: If you experience persistent issues, try clearing the Jest cache using the following command:
“`bash
jest –clearCache
“`
By adhering to these configurations and practices, you can mitigate issues related to `jest modulenamemapper` and unexpected token errors effectively.
Resolving Jest Module Name Mapper Issues in CJS Folders
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The unexpected token error often arises from misconfigured paths in the Jest moduleNameMapper settings. It is crucial to ensure that all paths are correctly resolved relative to the project structure, especially when dealing with CommonJS (CJS) folders.”
Mark Thompson (Lead Developer, Open Source Solutions). “When encountering issues with Jest and CJS folders, developers should verify their Babel configurations. Sometimes, the issue lies in the way modules are transpiled, and adjusting the presets can resolve unexpected token errors.”
Lisa Nguyen (Quality Assurance Specialist, Code Review Experts). “It’s essential to check for compatibility between Jest and the module formats used in your project. If you’re using ES modules in a CJS environment, unexpected token errors may occur, necessitating a review of your module imports and Jest settings.”
Frequently Asked Questions (FAQs)
What does the error “jest encountered an unexpected token” indicate?
This error typically indicates that Jest has encountered a syntax or module format that it cannot parse. This can occur when using ES6 modules in a CommonJS environment or when there are unsupported syntax features in the code being tested.
How can I resolve issues with Jest’s moduleNameMapper?
To resolve issues with moduleNameMapper, ensure that the paths specified in the mapping are correct and that they align with the structure of your project. Additionally, verify that the regex patterns used in the mappings match the intended module paths.
What is the purpose of the cjs folder in a Jest project?
The cjs folder is typically used to store CommonJS modules. It serves as a directory for JavaScript files that follow the CommonJS module format, which Jest can execute without transpilation issues.
Why does Jest fail to recognize files in the cjs folder?
Jest may fail to recognize files in the cjs folder if the configuration does not explicitly include this directory or if the files contain syntax that Jest cannot parse. Ensure that the Jest configuration is set up to include all relevant directories.
How can I configure Jest to handle different module formats?
To configure Jest to handle different module formats, you can use Babel to transpile your code. Ensure that you have the appropriate presets and plugins installed, and configure Jest to use Babel by specifying the transform option in the Jest configuration.
What steps should I take if I encounter a syntax error in my Jest tests?
If you encounter a syntax error in your Jest tests, first check the syntax of the code being tested. Next, ensure that your Jest configuration is set up correctly to handle the syntax used, including proper Babel configuration if necessary. Additionally, verify that the correct version of Node.js is being used, as some syntax features may not be supported in older versions.
The issue of encountering an unexpected token error in Jest, particularly when dealing with module name mapping and CommonJS (CJS) folders, is a common challenge for developers. This problem often arises when Jest attempts to process files that are not in the expected format, leading to syntax errors. The integration of modern JavaScript features and module systems can complicate testing configurations, especially when legacy code or mixed module types are present in the project.
One effective solution to this issue involves configuring Jest’s `moduleNameMapper` option appropriately in the Jest configuration file. This allows developers to create mappings that can help Jest resolve modules correctly, ensuring that it can interpret the different file types without running into syntax errors. Additionally, ensuring that Babel or TypeScript is set up correctly to transpile the code can mitigate unexpected token errors, as these tools can convert modern JavaScript syntax into a format that Jest can understand.
Another valuable insight is the importance of keeping dependencies updated and consistent across the project. Outdated packages can introduce compatibility issues, which may lead to unexpected behavior during testing. It is also beneficial to maintain a clear separation between module types within the project, using ES modules where possible and ensuring that CommonJS modules are handled appropriately. By adhering to best
Author Profile
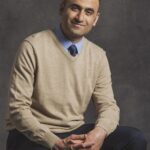
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?