Does Laravel Automatically Migrate the Database During Installation?
When embarking on a new web development project, the choice of a framework can significantly influence both the development process and the final product. Laravel, a popular PHP framework, stands out for its elegant syntax, robust features, and active community support. However, as developers dive into the installation process, they often encounter the question: “Will Laravel create a database for me?” This inquiry is not just about convenience; it touches on the broader themes of configuration, setup efficiency, and the developer’s control over their environment. In this article, we will explore the intricacies of Laravel’s installation process, particularly focusing on its interaction with databases and what developers can expect when setting up their applications.
As you begin your journey with Laravel, understanding its database management capabilities is crucial. Laravel does not automatically create a database upon installation; instead, it requires a pre-existing database to connect to. This means that developers must set up their database environment before they can fully leverage Laravel’s powerful features. The framework supports a variety of database systems, including MySQL, PostgreSQL, and SQLite, allowing for flexibility based on project requirements.
Moreover, Laravel’s configuration files play a pivotal role in establishing database connections. By customizing these settings, developers can ensure that their applications interact seamlessly with the database
Understanding Laravel’s Database Configuration
When installing Laravel, the framework requires a proper database setup to facilitate its operations. Laravel supports various database management systems, including MySQL, PostgreSQL, SQLite, and SQL Server. During installation, it is crucial to ensure that the database is configured correctly to prevent issues during application development.
The configuration file for database settings is located in the `.env` file at the root of your Laravel project. Here, you can specify the connection details for your database, including the database type, host, username, password, and database name.
Common Database Connection Parameters
Here are the common parameters you will need to set in your `.env` file for a MySQL database:
- DB_CONNECTION: Specifies the database driver (e.g., `mysql`, `pgsql`, `sqlite`, etc.)
- DB_HOST: The hostname of the database server (e.g., `127.0.0.1` or `localhost`)
- DB_PORT: The port number to connect to the database (default for MySQL is `3306`)
- DB_DATABASE: The name of the database you want to use
- DB_USERNAME: The username for connecting to the database
- DB_PASSWORD: The password for the database user
Here’s an example of how these parameters might look in your `.env` file:
“`plaintext
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=laravel_db
DB_USERNAME=root
DB_PASSWORD=secret
“`
Setting Up the Database
Before running your Laravel application, ensure that the specified database exists. You can create a new database using MySQL command line or a database management tool like phpMyAdmin. To create a database using the MySQL command line, you can execute the following command:
“`sql
CREATE DATABASE laravel_db;
“`
After creating the database, verify the connection settings in your `.env` file. If you encounter issues, check:
- If the database server is running
- If the credentials are correct
- Whether the user has appropriate permissions on the database
Running Migrations
Laravel uses a feature called migrations to manage database schema changes. After setting up your database, you can run migrations to create the necessary tables by executing the following artisan command:
“`bash
php artisan migrate
“`
This command will read the migration files in the `database/migrations` directory and create the corresponding tables in the database.
Common Installation Issues
While installing Laravel and setting up the database, you may face some common issues:
Issue | Possible Solution |
---|---|
Database connection error | Check your `.env` configuration |
Migration errors | Ensure migrations are up to date |
User permission issues | Grant the necessary permissions to the user |
Missing database driver | Install the required PHP extension (e.g., `pdo_mysql`) |
Ensuring that you have addressed these issues will streamline your development process and minimize disruptions as you work with Laravel.
Potential Database Issues During Laravel Installation
When installing Laravel, there are several database-related challenges you might encounter. Understanding these potential issues can help in troubleshooting effectively.
Common Database Configuration Errors
Incorrect database configuration can lead to various errors during installation. The following are common mistakes:
- Wrong Database Credentials: Ensure that the username, password, database name, and host are correct in the `.env` file.
- Database Driver Mismatch: The specified database driver in the `.env` file must match the installed database system (e.g., MySQL, PostgreSQL).
- Missing Database: The specified database should exist before running migrations; otherwise, an error will occur.
Database Connection Troubleshooting Steps
If you encounter connection issues, follow these troubleshooting steps:
- Check .env File: Review the following entries in your `.env` file:
- `DB_CONNECTION`
- `DB_HOST`
- `DB_PORT`
- `DB_DATABASE`
- `DB_USERNAME`
- `DB_PASSWORD`
- Test Database Connection: Use a database client (e.g., MySQL Workbench, pgAdmin) to verify that you can connect using the same credentials.
- Check Firewall Settings: Ensure that your database server is accessible from your application server. Configure firewall settings if necessary.
- Install Required Extensions: Ensure that the PHP extensions for your database (like `pdo_mysql` for MySQL) are installed and enabled.
Common Database Migration Issues
Migrations are essential for setting up your database structure in Laravel. However, issues can arise:
- Migration File Errors: Check for syntax errors in migration files. Use `php artisan migrate` for error messages.
- Table Already Exists: If you try to migrate and receive a “table already exists” error, you may need to roll back migrations using `php artisan migrate:rollback`.
- Foreign Key Constraints: Ensure that foreign keys are set correctly and that related tables are migrated in the proper order.
Database Seeding Challenges
Database seeding can also present problems:
- Seed Class Not Found: Ensure that the seed class exists and is correctly referenced in your `DatabaseSeeder.php`.
- Data Integrity Issues: When seeding, data must comply with validation rules defined in your models.
Best Practices for Database Management in Laravel
To avoid potential issues during installation and database management, consider the following best practices:
- Version Control: Use version control for your migration files to track changes effectively.
- Backup Databases: Regularly back up your databases to prevent data loss.
- Use Environment Variables: Keep sensitive information like database credentials out of your codebase by using environment variables.
Best Practice | Description |
---|---|
Version Control | Track changes in migration files |
Backup Databases | Prevent data loss through regular backups |
Environment Variables | Secure sensitive data in `.env` files |
Consistent Naming Conventions | Use clear and consistent naming for migrations and seeds |
By adhering to these practices, you can streamline the installation process and mitigate potential database issues in your Laravel applications.
Expert Insights on Laravel Database Installation Challenges
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When installing Laravel, it is essential to ensure that the database configuration is set up correctly. Many developers overlook this step, leading to potential issues during the installation process. A misconfigured database can result in connection errors that hinder application functionality.”
Michael Chen (Lead Developer, CodeCraft Solutions). “Laravel’s installation process is designed to be straightforward, but it can be complicated by environmental factors such as PHP version compatibility and database server settings. It’s crucial to verify that your local or server environment meets all requirements to avoid installation hiccups.”
Lisa Thompson (Database Administrator, DataWise Technologies). “One common issue during Laravel installation is the database migration process. If the database is not properly set up beforehand, migrations can fail, leading to incomplete installations. Developers should always double-check their database settings and permissions prior to starting the Laravel installation.”
Frequently Asked Questions (FAQs)
What database does Laravel use by default?
Laravel uses MySQL as its default database management system. However, it also supports other databases such as PostgreSQL, SQLite, and SQL Server.
Can I change the database configuration during Laravel installation?
Yes, you can change the database configuration during installation by modifying the `.env` file. This file allows you to specify your preferred database connection settings.
Does Laravel automatically create a database upon installation?
No, Laravel does not automatically create a database upon installation. You must manually create the database in your chosen database management system before running migrations.
What command do I use to set up the database in Laravel?
To set up the database, you can use the command `php artisan migrate`. This command runs the migrations defined in your application, creating the necessary tables in the database.
Is it necessary to have a database for Laravel to function?
While Laravel can function without a database for certain applications, many features, such as Eloquent ORM and migrations, require a database to store and manage data effectively.
How can I check if the database connection is successful in Laravel?
You can check the database connection by running the command `php artisan migrate` or by using the `DB::connection()->getPdo()` method in your application. If the connection is successful, no errors will be thrown.
In summary, when installing Laravel, it is essential to consider the potential database configurations that may arise during the setup process. Laravel supports various database systems, including MySQL, PostgreSQL, SQLite, and SQL Server. The framework’s flexibility allows developers to choose the most suitable database for their application needs, which can significantly influence the overall performance and scalability of the project.
Additionally, Laravel provides a robust migration system that facilitates the management of database schemas. This feature allows developers to easily create, modify, and share database structures, ensuring that the application remains consistent across different environments. It is advisable to configure the database connection settings in the .env file during installation to streamline the setup process and avoid potential connectivity issues.
Moreover, leveraging Laravel’s built-in Eloquent ORM can enhance database interactions by providing an elegant and expressive syntax for querying and manipulating data. This abstraction layer simplifies the process of working with databases, making it easier for developers to focus on building features rather than dealing with complex SQL queries.
understanding the database implications during the installation of Laravel is crucial for setting up a successful application. By carefully selecting the appropriate database system and utilizing Laravel’s powerful features, developers can create efficient and maintainable applications that meet
Author Profile
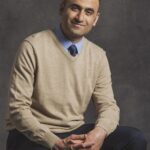
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?