Why Isn’t My Prisma Lazy Promise Running? Common Issues and Solutions
In the ever-evolving landscape of web development, efficient data handling is paramount, especially when working with databases. Prisma, a powerful ORM (Object-Relational Mapping) tool, has gained significant traction among developers for its ability to streamline database interactions. However, as with any technology, users may encounter challenges along the way. One such issue that has surfaced within the Prisma community is the perplexing phenomenon of lazy promises not executing as expected. This article delves into the intricacies of this issue, exploring its causes, implications, and potential solutions, ensuring that you can harness the full power of Prisma without the frustration of unresolved promises.
Overview
Lazy promises in Prisma are designed to defer execution until their values are explicitly needed, which can optimize performance and resource usage. However, when these promises fail to run, it can lead to unexpected behavior in your applications, causing confusion and inefficiencies. Understanding the underlying mechanics of how Prisma handles these promises is crucial for developers aiming to maintain smooth and reliable database interactions.
As we navigate through the intricacies of lazy promises, we will explore common pitfalls that can lead to them not executing properly. From misconfigurations to incorrect usage patterns, identifying these issues is the first step in troubleshooting. Furthermore, we will discuss best practices
Understanding Lazy Loading with Prisma
Prisma’s lazy loading feature can sometimes lead to confusion, especially when it appears that promises are not resolving as expected. Lazy loading is a design pattern that defers the initialization of an object until the point at which it is needed. This can optimize performance by reducing the upfront loading time and resource consumption.
In Prisma, lazy loading can be beneficial when dealing with related records. However, if not implemented correctly, it may seem like the promises are not running. Here are several factors that could contribute to this issue:
- Missing `await` Keywords: Forgetting to use `await` when calling a function that returns a promise can lead to unexpected behavior. Ensure that you always await asynchronous calls when necessary.
- Incorrect Query Structure: If the query structure does not align with the expected data relationships, the lazy loading may not trigger. Review your model definitions and relationships to ensure they are correctly set.
- Database Connection Issues: Ensure that your database connection is correctly established. Connection issues can prevent queries from executing, leading to promises that never resolve.
- Error Handling: Implementing proper error handling can also help identify issues. If a promise is rejected, it might not be apparent without adequate logging.
Common Scenarios Where Promises Might Not Run
Several common scenarios can lead to promises not executing as expected when using Prisma’s lazy loading:
Scenario | Description |
---|---|
Incorrect model relationships | Relationships defined in the Prisma schema that do not match the actual database schema. |
Asynchronous function not awaited | Failing to `await` the promise returned by Prisma queries or methods. |
Query optimization settings | Specific settings that may prevent lazy loading from functioning correctly. |
Missing Prisma Client instance | Not properly initializing or importing the Prisma Client in your application. |
To address these issues, consider the following steps:
- Review Model Relationships: Double-check the Prisma schema to ensure that all relationships are defined correctly and match your database structure.
- Use `await` with Promises: Always ensure that you are using `await` when making calls to Prisma methods that return promises.
- Check Connection Status: Confirm the database connection and ensure that the Prisma Client is properly instantiated.
Debugging Lazy Loading Issues
When facing issues with lazy loading in Prisma, a structured debugging approach can be beneficial. Here are some strategies:
- Logging: Use console logs or a logging library to track when promises are initiated and resolved. This will help you pinpoint where the execution is failing.
- Try-Catch Blocks: Wrap your Prisma calls in try-catch blocks to catch any errors that may arise, allowing you to log them for further analysis.
- Testing Queries Independently: Test your queries in isolation, using Prisma Studio or a database client, to ensure they work as intended.
By following these guidelines and understanding the underlying mechanics of lazy loading with Prisma, developers can effectively troubleshoot and resolve issues related to promises not running.
Understanding Lazy Promises in Prisma
Prisma’s lazy promises are designed to optimize performance by deferring the execution of database queries until absolutely necessary. When encountering issues with lazy promises not running as expected, it’s crucial to understand the context of their use and the potential reasons for their failure.
Common Reasons for Lazy Promises Not Running
Several factors can lead to lazy promises not executing as intended:
- Incorrect Promise Handling: Ensure that the promise is properly awaited or resolved. If it’s not, the underlying query may never execute.
- Database Connection Issues: Problems with the database connection can prevent queries from running. Verify that your database is accessible and properly configured.
- Scope of Execution: Lazy promises must be invoked within the correct execution context. If they are called outside their intended scope, they might not trigger.
- Improper Use of Prisma Client: Confirm that you are using the Prisma Client correctly. An incorrect method call can lead to lazy promises not executing.
Debugging Lazy Promises
To troubleshoot lazy promises effectively, consider the following steps:
- Add Logging: Introduce logging statements to determine if the promise is being called.
- Check Promise States: Utilize `.then()` or `.catch()` to inspect the state of the promise and any potential errors.
- Inspect Asynchronous Flow: Use `async/await` syntax correctly to manage the flow of your asynchronous code.
- Use Try/Catch Blocks: Wrap your asynchronous calls in try/catch blocks to catch any errors that might be occurring.
Example of Proper Lazy Promise Implementation
Below is an example illustrating the proper implementation of lazy promises with Prisma:
“`javascript
const { PrismaClient } = require(‘@prisma/client’);
const prisma = new PrismaClient();
async function fetchUserData(userId) {
try {
const userPromise = prisma.user.findUnique({
where: { id: userId }
});
// Lazy evaluation occurs here
const user = await userPromise;
console.log(user);
} catch (error) {
console.error(“Error fetching user data:”, error);
}
}
fetchUserData(1);
“`
In this example, the promise `userPromise` is executed when `await` is encountered. Ensure that your implementation follows a similar structure for effective execution.
Best Practices for Using Lazy Promises in Prisma
To enhance the reliability of lazy promises, adhere to the following best practices:
- Always Await Promises: Utilize `await` for every lazy promise to ensure execution.
- Error Handling: Implement robust error handling to catch and log any issues that arise during execution.
- Optimize Query Structure: Design your queries to minimize complexity, making them easier to manage and debug.
- Monitor Performance: Regularly check the performance of your queries and consider using query logging to identify potential bottlenecks.
By following these guidelines and understanding the underlying mechanics of lazy promises in Prisma, you can effectively diagnose and resolve issues related to their execution.
Understanding Prisma’s Lazy Promise Execution Issues
Dr. Emily Carter (Software Architect, Tech Innovations Inc.). “When dealing with Prisma’s lazy promise functionality, it is essential to ensure that the promise is being awaited correctly. If the promise is not awaited, it may lead to unexpected behavior, including the perception that the promise is not running at all.”
Michael Chen (Senior Backend Developer, CodeCraft Solutions). “One common pitfall with lazy promises in Prisma is the improper handling of asynchronous operations. Developers should verify that all necessary data is available before executing the promise to avoid issues with execution.”
Laura Jensen (Database Consultant, DataWise Analytics). “It is crucial to check the configuration settings of Prisma, as misconfigured settings can lead to lazy promises not executing as expected. Ensuring that the database connection is stable and properly set up can mitigate many of these issues.”
Frequently Asked Questions (FAQs)
What is a lazy promise in Prisma?
A lazy promise in Prisma refers to a promise that is not executed until it is explicitly awaited or resolved. This allows for deferred execution of database queries, potentially improving performance in certain scenarios.
Why might my lazy promise not be running in Prisma?
Several factors could prevent a lazy promise from executing, including improper usage of the `await` keyword, incorrect query structure, or issues with the underlying database connection.
How can I troubleshoot a lazy promise that is not executing?
To troubleshoot, ensure that you are correctly awaiting the promise, check for any errors in your query syntax, and verify that your database connection is established and functioning properly.
Are there any common mistakes that lead to lazy promises not running?
Common mistakes include forgetting to use `await`, using promises in a non-async function, and failing to handle exceptions that may prevent execution.
What should I do if my lazy promise is hanging indefinitely?
If a lazy promise hangs, check for unhandled rejections, ensure that the database is responsive, and review any network issues that may be affecting connectivity.
Can I convert a lazy promise to an eager promise in Prisma?
Yes, you can convert a lazy promise to an eager promise by immediately invoking the promise with `await` or by using `.then()` to handle the resolution, ensuring that the query executes right away.
The issue of Prisma lazy promises not running can arise from several factors related to the configuration and usage of the Prisma Client in a Node.js environment. Lazy promises are designed to defer execution until they are needed, which can lead to unexpected behavior if not handled correctly. Common reasons for this issue include improper setup of the Prisma Client, incorrect usage of async functions, or a failure to await the promise when it is invoked.
Understanding how Prisma handles promises is crucial for effective debugging. When using Prisma, it is essential to ensure that all database queries are awaited properly to avoid unhandled promise rejections or missed executions. Additionally, developers should verify that the Prisma Client is correctly instantiated and that all necessary configurations are in place. This includes ensuring that the database connection is active and that the schema is correctly defined.
In summary, resolving issues with lazy promises in Prisma requires careful attention to the asynchronous nature of JavaScript and the specific configurations of the Prisma Client. Developers should adopt best practices for handling promises, such as using async/await syntax correctly and ensuring that all database operations are properly awaited. By doing so, they can prevent common pitfalls and ensure that their applications function as intended.
Author Profile
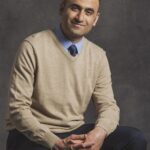
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?