How Can You Swiftly Keep Remote Files In Sync?
In today’s fast-paced digital landscape, the ability to manage and access files remotely has become a cornerstone of productivity and collaboration. As more individuals and organizations transition to remote work and cloud-based solutions, the need for effective file management strategies has never been more crucial. One such strategy involves utilizing Swift, a powerful programming language developed by Apple, to keep remote files in sync effortlessly. This article delves into the intricacies of maintaining file synchronization using Swift, exploring the tools and techniques that can streamline this process and enhance your workflow.
Keeping remote files in sync is not just about accessing data from different locations; it’s about ensuring that the information is current, reliable, and easily retrievable. Swift provides developers with robust capabilities to interact with cloud storage services, enabling seamless integration and real-time updates. By leveraging Swift’s features, you can create applications that not only manage file uploads and downloads but also handle conflicts and version control, ensuring that your data remains consistent across all platforms.
As we explore the various methods and best practices for keeping remote files in sync using Swift, we will highlight the importance of choosing the right APIs, understanding data transfer protocols, and implementing efficient error handling. Whether you’re a seasoned developer or just starting with Swift, this article aims to equip you with the knowledge and tools necessary
Understanding Remote File Management in Swift
Managing remote files in Swift involves interacting with files that are not stored locally on a device, but rather on a server or cloud storage. This process typically requires the use of APIs and networking protocols to facilitate the transfer and manipulation of files. Swift provides several libraries and frameworks that can be utilized for this purpose, enhancing the capabilities of developers working on applications that require remote file interactions.
Key Components for Remote File Access
To effectively manage remote files in Swift, several key components must be considered:
- Networking Libraries: Swift provides `URLSession`, a powerful API for making HTTP requests to fetch or upload files.
- File Formats: Understanding the types of files being handled (e.g., JSON, XML) is crucial for proper serialization and deserialization.
- Authentication: Many remote file systems require authentication (e.g., OAuth, API keys) to access their resources securely.
Using URLSession for Remote File Operations
`URLSession` is essential for performing network tasks in Swift. It allows developers to configure and send requests to remote servers. Here is a basic example of how to use `URLSession` to download a file:
“`swift
let url = URL(string: “https://example.com/file.txt”)!
let task = URLSession.shared.dataTask(with: url) { data, response, error in
if let error = error {
print(“Error downloading file: \(error)”)
return
}
if let data = data {
// Process the downloaded data
let content = String(data: data, encoding: .utf8)
print(“Downloaded content: \(content ?? “No content”)”)
}
}
task.resume()
“`
This code snippet demonstrates how to initiate a download task, handle potential errors, and process the data once received.
Handling File Uploads
Uploading files to a remote server can also be accomplished using `URLSession`. The following is a typical approach to upload data:
“`swift
let url = URL(string: “https://example.com/upload”)!
var request = URLRequest(url: url)
request.httpMethod = “POST”
let boundary = UUID().uuidString
request.setValue(“multipart/form-data; boundary=\(boundary)”, forHTTPHeaderField: “Content-Type”)
var body = Data()
let fileData = Data() // Assume this is your file data
body.append(“–\(boundary)\r\n”)
body.append(“Content-Disposition: form-data; name=\”file\”; filename=\”file.txt\”\r\n”)
body.append(“Content-Type: text/plain\r\n\r\n”)
body.append(fileData)
body.append(“\r\n–\(boundary)–\r\n”)
request.httpBody = body
let uploadTask = URLSession.shared.dataTask(with: request) { data, response, error in
if let error = error {
print(“Error uploading file: \(error)”)
return
}
// Handle server response
}
uploadTask.resume()
“`
In this example, a multipart/form-data request is created to upload a file, ensuring that the correct content type is specified.
Best Practices for Remote File Management
To ensure optimal performance and security when managing remote files in Swift, consider the following best practices:
- Error Handling: Implement comprehensive error handling to manage network issues gracefully.
- Data Caching: Use caching strategies to minimize network calls and improve performance.
- Security: Always use HTTPS to encrypt data in transit and protect sensitive information.
File Management Summary Table
Operation | Swift Component | Best Practice |
---|---|---|
Download File | URLSession | Handle errors and validate data |
Upload File | URLSession with multipart/form-data | Secure data and use proper content types |
Authentication | Custom Headers | Use OAuth or API keys securely |
Maintaining Remote File Integrity in Swift
When dealing with remote files in Swift, ensuring their integrity is crucial for the proper functioning of applications. This involves various strategies and techniques to manage file access, synchronization, and error handling.
File Access Management
Accessing remote files efficiently requires a structured approach. Consider the following methods:
- URLSession: Utilize `URLSession` for downloading and uploading files. This class provides a robust API to handle network requests, including authentication, caching, and background transfers.
- File Coordination: Use `NSFileCoordinator` for coordinating file access, especially when multiple processes might be accessing the same file. This ensures that read and write operations do not conflict.
- Error Handling: Implement thorough error handling using `do-catch` blocks to manage exceptions that may arise during file access.
Example of using `URLSession` for downloading a file:
“`swift
let url = URL(string: “https://example.com/remote-file.txt”)!
let task = URLSession.shared.dataTask(with: url) { data, response, error in
guard let data = data, error == nil else {
print(“Error: \(error?.localizedDescription ?? “Unknown error”)”)
return
}
// Process the data
}
task.resume()
“`
File Synchronization Techniques
Synchronizing remote files ensures that the latest versions are accessible across devices. Key techniques include:
- Polling: Regularly check for updates on the remote server. Use a timer or a background task to query the server at defined intervals.
- WebSocket: For real-time updates, consider using WebSocket connections. This allows immediate notification of changes on the server side.
- Change Tracking: Implement a change-tracking mechanism that logs modifications to remote files. This could involve timestamps or versioning.
Data Caching Strategies
Caching is vital to reduce latency and improve performance. Explore the following strategies:
Cache Type | Description | Use Case |
---|---|---|
In-Memory Cache | Stores frequently accessed data temporarily. | Ideal for quick access to small files. |
Disk Cache | Saves files on local storage for offline access. | Useful for larger files or infrequent access. |
Persistent Store | Utilizes databases to manage file metadata and state. | Best for applications requiring data integrity. |
Implementing a caching mechanism can help reduce the number of requests made to the server, thus optimizing performance.
Security Considerations
When managing remote files, security is paramount. Here are essential practices:
- Encryption: Use HTTPS for secure file transfers. Additionally, consider encrypting files before storage.
- Authentication: Implement OAuth or similar authentication methods to ensure that only authorized users can access sensitive files.
- Access Control: Define clear permissions for file access and modifications to prevent unauthorized changes.
By integrating these security measures, you safeguard your remote files against unauthorized access and data breaches.
Testing and Debugging
Robust testing and debugging practices help maintain file integrity. Consider the following:
- Unit Testing: Write unit tests for file handling functions to ensure reliability and correctness.
- Mocking Remote Responses: Use mocking frameworks to simulate remote server responses during testing.
- Logging: Implement logging to capture file access attempts, errors, and other relevant events for analysis.
By adhering to these practices, you can effectively manage remote file integrity in Swift applications, ensuring a seamless user experience.
Expert Insights on Swift Remote File Management
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Utilizing Swift for managing remote files is a powerful approach, especially with its robust networking capabilities. Developers should leverage URLSession for efficient data transfer, ensuring that file integrity is maintained during remote operations.”
James Liu (Lead Mobile Developer, App Solutions Group). “Incorporating Swift for remote file handling requires a solid understanding of asynchronous programming. This allows for seamless user experiences while files are being downloaded or uploaded, minimizing app latency and improving responsiveness.”
Sarah Thompson (Cloud Computing Specialist, Future Tech Labs). “When working with remote files in Swift, it is crucial to implement proper error handling and data validation. This ensures that any issues during file transfers are gracefully managed, thereby enhancing the overall reliability of the application.”
Frequently Asked Questions (FAQs)
What is Swift keeping remote files in sync?
Swift keeping remote files in sync refers to the process of ensuring that files stored on a remote server or cloud service are consistently updated and match the local copies. This is crucial for maintaining data integrity and accessibility across different devices.
How does Swift manage file synchronization?
Swift utilizes a combination of APIs and background processes to monitor changes in files. It employs techniques such as checksums and timestamps to detect modifications, ensuring that updates are propagated to all relevant locations without manual intervention.
What are the benefits of using Swift for remote file synchronization?
The benefits include real-time updates, reduced risk of data loss, improved collaboration among users, and the ability to access the latest versions of files from any device connected to the internet.
Can Swift handle large files during synchronization?
Yes, Swift is designed to efficiently manage large files. It employs chunking methods to break down files into smaller segments, allowing for faster uploads and downloads, as well as minimizing the impact on bandwidth.
Is Swift compatible with all cloud storage providers?
Swift is compatible with many cloud storage providers, but specific integrations may vary. It is advisable to check the documentation for supported services and any necessary configurations for optimal performance.
What security measures does Swift implement for remote file synchronization?
Swift employs encryption protocols for data in transit and at rest, ensuring that files are protected from unauthorized access. Additionally, it supports authentication mechanisms to verify user identities before granting access to files.
In summary, the concept of keeping remote files in sync using Swift involves leveraging various APIs and frameworks that facilitate seamless data management between local and remote storage solutions. Swift provides robust tools for developers to implement synchronization mechanisms, ensuring that any changes made to files are consistently reflected across all platforms. This capability is essential for applications that require real-time updates and collaborative features, enhancing user experience and operational efficiency.
Additionally, the implementation of remote file synchronization in Swift can be optimized through the use of background tasks and efficient data transfer protocols. Developers are encouraged to utilize libraries and frameworks that support asynchronous operations, allowing for smooth user interactions without compromising performance. By adopting best practices in error handling and conflict resolution, applications can maintain data integrity and reliability, which are crucial for user trust and satisfaction.
Key takeaways from this discussion highlight the importance of understanding the underlying principles of file synchronization, including the need for robust networking capabilities and effective data management strategies. Developers should remain informed about the latest advancements in Swift and related technologies to ensure their applications are competitive and meet the evolving needs of users. Emphasizing security and user privacy during the synchronization process is also paramount, as it builds confidence in the application and protects sensitive information.
Author Profile
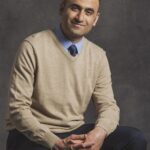
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?