How Can I Retrieve All Products from the Cart in Magento 2 Using JavaScript?
In the ever-evolving world of eCommerce, Magento 2 stands out as a powerful platform that empowers businesses to create dynamic online stores. One of the essential functionalities that every online retailer must master is the ability to manage the shopping cart effectively. Understanding how to retrieve all products from the cart using JavaScript is crucial for developers and store owners alike. This capability not only enhances user experience but also facilitates better inventory management and personalized marketing strategies. In this article, we will delve into the intricacies of accessing cart data in Magento 2, providing you with the insights needed to optimize your online store’s performance.
When working with Magento 2, developers often need to interact with the shopping cart to enhance functionality or improve user engagement. JavaScript serves as a vital tool in this process, allowing for seamless integration and real-time updates. By leveraging Magento’s built-in APIs and JavaScript capabilities, developers can efficiently retrieve all products currently in the cart. This knowledge is particularly valuable for implementing features such as dynamic pricing updates, personalized recommendations, or even cart abandonment strategies.
Moreover, understanding how to access cart data can significantly streamline the checkout process, making it smoother for customers. As online shopping becomes increasingly competitive, ensuring that your store can effectively manage cart contents is essential for driving conversions and fostering
Accessing Cart Data in Magento 2 Using JavaScript
To retrieve all products from the cart in Magento 2 using JavaScript, the most efficient way is to utilize the built-in Magento 2 API. This allows you to interact with the cart data seamlessly. Here’s how to achieve this through JavaScript:
- Use the `Magento_Customer/js/customer-data` Module: This module provides a way to access the customer data, including the cart contents. By leveraging this, you can retrieve all products currently in the user’s cart.
- Fetch Cart Items: The following code snippet demonstrates how to get the cart items using the `customer-data` module.
“`javascript
require([‘Magento_Customer/js/customer-data’], function (customerData) {
var sections = [‘cart’];
var data = customerData.get(sections);
var cartItems = data.cart.items;
console.log(cartItems); // Output the cart items
});
“`
This code utilizes the `customer-data` module to fetch the cart section, which includes an array of items. Each item in the `cartItems` array will contain details such as product ID, name, price, and quantity.
Understanding the Cart Item Structure
When you retrieve the cart items, each item will be structured as follows:
Field | Description |
---|---|
item_id | The unique identifier for the cart item. |
product_id | The ID of the product that has been added to the cart. |
name | The name of the product. |
price | The price of the product. |
qty | The quantity of the product in the cart. |
options | An array of options selected for the product, such as size or color. |
This structured data allows you to easily manipulate and display the cart contents as needed on your frontend.
Implementing Additional Features
To enhance the user experience, you can implement additional features using the cart data. Here are some ideas:
- Dynamic Cart Updates: Automatically update the cart display in real-time as items are added or removed.
- Cart Summary Display: Show a summary of items, including total price and quantity, on the shopping cart page or in a sidebar.
- Product Removal: Create a functionality that allows users to remove items directly from the cart using JavaScript.
To achieve these functionalities, you can listen for events on your cart UI and re-fetch the cart data when changes occur. This ensures that the displayed data is always up-to-date.
By using the Magento 2 JavaScript API effectively, you can create a dynamic and interactive shopping experience that keeps customers engaged and informed about their cart contents.
Accessing Cart Data in Magento 2 Using JavaScript
To retrieve all products from the cart in Magento 2 using JavaScript, you can leverage the built-in REST APIs provided by Magento. This method allows you to fetch cart items dynamically without reloading the page.
Using Magento 2 REST API to Get Cart Items
Magento 2 offers a REST API endpoint specifically for retrieving cart details. To access the cart items, follow these steps:
- Set Up API Access: Ensure you have the necessary API credentials (consumer key, consumer secret, access token) for authentication.
- Fetch Cart ID: If you are using the customer’s cart, you can obtain the cart ID by calling the relevant API endpoint.
- Retrieve Cart Items: Use the following endpoint to get the cart items:
“`
GET
“`
- **JavaScript Implementation**: Below is a sample code snippet to illustrate how to implement this in JavaScript.
“`javascript
const cartId = ‘your_cart_id’; // Replace with dynamic cart ID retrieval
const url = `https://your_magento_url/rest/V1/carts/${cartId}/items`;
fetch(url, {
method: ‘GET’,
headers: {
‘Authorization’: ‘Bearer ‘ + accessToken, // Replace with your access token
‘Content-Type’: ‘application/json’
}
})
.then(response => response.json())
.then(data => {
console.log(‘Cart Items:’, data);
})
.catch(error => {
console.error(‘Error fetching cart items:’, error);
});
“`
Handling Cart Data in JavaScript
Once you have retrieved the cart items, you can process the data accordingly. The response will typically include the following fields for each product:
- `item_id`: Unique identifier for the item.
- `sku`: Stock Keeping Unit for the product.
- `name`: Product name.
- `qty`: Quantity of the product in the cart.
- `price`: Price per unit.
- `product_type`: Type of product (e.g., simple, configurable).
You can loop through the data and manipulate it as needed:
“`javascript
data.forEach(item => {
console.log(`Product Name: ${item.name}`);
console.log(`Quantity: ${item.qty}`);
console.log(`Price: ${item.price}`);
});
“`
Considerations for Using JavaScript with Magento 2
When working with JavaScript in Magento 2, keep in mind the following:
- CORS Issues: Ensure that your Magento server is configured to allow CORS if you are making requests from a different domain.
- Session Management: If you are working with customer sessions, make sure that the authentication token is valid and securely stored.
- Error Handling: Implement robust error handling to manage potential issues with API calls, such as network errors or unauthorized access.
By following these guidelines, you can effectively access and manipulate cart data in Magento 2 using JavaScript, enhancing the user experience on your eCommerce site.
Expert Insights on Retrieving Cart Products in Magento 2 Using JavaScript
Emily Chen (E-commerce Developer, Magento Solutions Inc.). “To effectively retrieve all products from the cart in Magento 2 using JavaScript, developers can leverage the built-in REST API. This approach allows for seamless integration and ensures that you can access the cart data dynamically, providing a better user experience.”
Michael Torres (Senior Magento Architect, Digital Commerce Agency). “Utilizing the `window.checkoutConfig` object in Magento 2 can be an efficient way to get cart items in JavaScript. This method allows developers to access the cart data directly from the frontend, making it easier to manipulate and display product information as needed.”
Sarah Patel (Lead Frontend Engineer, E-commerce Innovations). “When working with Magento 2, it’s crucial to understand the asynchronous nature of JavaScript. Implementing AJAX calls to the cart endpoint will enable you to fetch all products without reloading the page, enhancing the overall performance of your e-commerce site.”
Frequently Asked Questions (FAQs)
How can I retrieve all products from the cart in Magento 2 using JavaScript?
You can retrieve all products from the cart in Magento 2 by using the `quote` API endpoint. You can make an AJAX call to `/rest/V1/carts/mine` to get the cart details, which includes the list of products.
What JavaScript libraries are commonly used to interact with Magento 2 APIs?
Common JavaScript libraries include jQuery for AJAX requests and Magento’s own Knockout.js for data binding. These libraries facilitate the interaction with Magento 2’s REST APIs.
Is it necessary to authenticate when accessing the cart data in Magento 2?
Yes, authentication is required to access cart data. You must obtain a customer token for authenticated requests or use a guest token for guest users.
What data structure is returned when fetching products from the cart?
The data structure returned is typically a JSON object containing an array of items. Each item includes details such as product ID, name, quantity, price, and other attributes.
Can I modify the cart items using JavaScript in Magento 2?
Yes, you can modify cart items using JavaScript by sending PUT requests to the appropriate API endpoints, such as `/rest/V1/carts/mine/items/{itemId}` to update the quantity or remove items.
Are there any performance considerations when fetching cart data in Magento 2?
Yes, fetching cart data can impact performance, especially with large carts. It’s advisable to implement caching strategies and optimize API calls to minimize the load on the server.
In Magento 2, retrieving all products from the cart using JavaScript can be accomplished through the use of the built-in APIs. The most common approach involves utilizing the `quote` API, which allows developers to access the cart’s contents programmatically. By making an AJAX call to the appropriate endpoint, developers can fetch the cart items, including details such as product IDs, quantities, and other relevant attributes.
Implementing this functionality typically requires familiarity with Magento’s JavaScript framework, as well as an understanding of how to handle asynchronous requests. The response from the API can be processed to extract the necessary information about each product in the cart. This enables developers to create dynamic and interactive user experiences, such as displaying cart contents in real-time or updating the UI based on user interactions.
Moreover, leveraging Magento’s built-in capabilities for managing cart data ensures that developers adhere to best practices, promoting maintainability and compatibility with future updates. It is essential to handle potential errors gracefully, ensuring that users receive appropriate feedback in case of issues during the data retrieval process. Overall, understanding how to effectively get all products from the cart in Magento 2 using JavaScript is crucial for enhancing eCommerce functionality and improving user engagement.
Author Profile
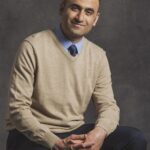
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?