How Can You Convert a String to an Integer in Python?
In the world of programming, data types are the building blocks of any application. Whether you’re developing a simple script or a complex software solution, understanding how to manipulate these data types is crucial. One common task that many developers encounter is converting strings to integers in Python. This seemingly straightforward operation can be the key to unlocking a plethora of functionalities, from performing mathematical calculations to processing user inputs effectively. If you’ve ever found yourself grappling with this conversion, you’re not alone—many programmers have faced this challenge, and mastering it can significantly enhance your coding prowess.
In Python, strings and integers serve different purposes, and knowing how to convert between them is essential for seamless data handling. The process involves understanding the nuances of data types and leveraging built-in functions that Python provides. This conversion is not just about changing the format; it’s about ensuring that your code runs smoothly and efficiently, especially when dealing with user-generated data or external inputs.
As you delve deeper into this topic, you’ll discover various methods and best practices for converting strings to integers, along with potential pitfalls to avoid. From handling exceptions to ensuring data integrity, the journey into this fundamental aspect of Python programming will equip you with the skills needed to tackle a wide range of coding scenarios. Whether you’re a beginner or looking to refresh
Using the `int()` Function
One of the most straightforward ways to convert a string to an integer in Python is by using the built-in `int()` function. This function takes a string representation of a number and converts it into an integer. If the string cannot be converted, it will raise a `ValueError`.
Example usage:
python
string_number = “42”
integer_number = int(string_number)
In this example, `integer_number` will hold the value `42` as an integer.
Handling Different Bases
The `int()` function also allows you to convert strings that represent numbers in different bases. You can specify the base as the second argument to the function, which can be useful when working with binary, octal, or hexadecimal strings.
Example:
python
binary_string = “1010”
integer_from_binary = int(binary_string, 2) # base 2
In this case, `integer_from_binary` will be `10` in decimal.
Dealing with Leading and Trailing Whitespace
When converting strings to integers, it is essential to ensure that there are no leading or trailing whitespace characters in the string. The `strip()` method can be utilized to remove any such whitespace before conversion.
Example:
python
string_with_spaces = ” 100 ”
cleaned_string = string_with_spaces.strip()
integer_number = int(cleaned_string)
This approach guarantees that the conversion will succeed without raising an error due to unexpected whitespace.
Handling Exceptions
It is good practice to handle potential exceptions when converting strings to integers. You can use a `try-except` block to catch `ValueError` exceptions gracefully.
Example:
python
try:
user_input = “not_a_number”
integer_number = int(user_input)
except ValueError:
print(“Invalid input! Please enter a valid number.”)
This ensures that your program can handle erroneous input without crashing.
Conversion Table
Below is a simple table illustrating how different string representations can be converted to integers:
String Input | Base | Integer Output |
---|---|---|
“42” | 10 | 42 |
“1010” | 2 | 10 |
“7F” | 16 | 127 |
” 56 “ | 10 | 56 |
This table demonstrates how various string formats relate to their corresponding integer values when converted using different bases. Each row provides insight into the flexibility of the `int()` function for handling various input scenarios.
Using the `int()` Function
The most straightforward way to convert a string to an integer in Python is by using the built-in `int()` function. This function takes a string argument and converts it into an integer representation.
python
string_number = “42”
integer_number = int(string_number)
print(integer_number) # Output: 42
### Important Considerations
- The string must represent a valid integer; otherwise, a `ValueError` will be raised.
- Leading and trailing whitespace in the string will be ignored.
python
string_with_spaces = ” 123 ”
integer_number = int(string_with_spaces)
print(integer_number) # Output: 123
Handling Invalid Input
When converting strings to integers, it is essential to handle cases where the string may not be a valid integer. This can be achieved using try-except blocks.
python
string_number = “abc”
try:
integer_number = int(string_number)
except ValueError:
print(“Invalid input: not a number”)
### Common Errors
- `ValueError`: Raised when the input string cannot be converted into an integer.
- `TypeError`: Raised if the input is not a string or a number.
Converting with Base Specification
The `int()` function can also convert strings representing numbers in different bases. You can specify the base as the second argument.
python
binary_string = “1010”
integer_number = int(binary_string, 2) # Base 2
print(integer_number) # Output: 10
hex_string = “1A”
integer_number = int(hex_string, 16) # Base 16
print(integer_number) # Output: 26
### Supported Bases
- Binary: Base 2
- Octal: Base 8
- Decimal: Base 10 (default)
- Hexadecimal: Base 16
Using `float()` for Decimal Strings
If the string represents a decimal number, you can convert it to a float first and then to an integer. This method allows for conversion of strings with decimal points.
python
decimal_string = “42.99”
integer_number = int(float(decimal_string))
print(integer_number) # Output: 42
### Rounding Behavior
- The conversion from float to integer truncates the decimal portion.
Using the `ast.literal_eval()` Function
For more complex scenarios, such as converting a string that represents a Python literal, you can use the `ast.literal_eval()` method from the `ast` module. This method safely evaluates a string containing a Python literal.
python
import ast
string_number = “42”
integer_number = ast.literal_eval(string_number)
print(integer_number) # Output: 42
### Advantages of `ast.literal_eval()`
- It safely evaluates strings containing Python literals without executing arbitrary code.
Converting strings to integers in Python can be accomplished using various methods, each suited to specific requirements. Whether using the `int()` function, handling errors, specifying bases, or evaluating literals, these techniques provide flexibility and safety in data conversion.
Expert Insights on Converting Strings to Integers in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Converting a string to an integer in Python is straightforward using the built-in `int()` function. However, developers should always ensure that the string is a valid representation of an integer to avoid exceptions. Implementing error handling with try-except blocks is a best practice.”
James Liu (Data Scientist, Analytics Solutions). “In data processing, converting strings to integers is a common requirement. Using the `int()` function is efficient, but one must be cautious with leading and trailing spaces in strings. Utilizing the `strip()` method before conversion can prevent unexpected errors.”
Maria Gonzalez (Software Engineer, CodeCraft Labs). “When dealing with user input, it is crucial to validate the string before conversion. Employing regular expressions can help ensure that the string contains only numeric characters, thus making the conversion process more robust and error-free.”
Frequently Asked Questions (FAQs)
How do I convert a string to an integer in Python?
You can convert a string to an integer in Python using the `int()` function. For example, `int(“123”)` will return the integer `123`.
What happens if the string cannot be converted to an integer?
If the string cannot be converted to an integer, Python will raise a `ValueError`. For example, `int(“abc”)` will result in an error.
Can I convert a string with whitespace to an integer?
Yes, the `int()` function can handle strings with leading or trailing whitespace. For instance, `int(” 456 “)` will return the integer `456`.
Is it possible to convert a string representing a floating-point number to an integer?
Yes, you can convert a string representing a floating-point number to an integer, but you must first convert it to a float. For example, `int(float(“3.14”))` will yield `3`.
What is the difference between `int()` and `float()` in Python?
The `int()` function converts a string to an integer, while the `float()` function converts a string to a floating-point number. Use `int()` for whole numbers and `float()` for decimal values.
Can I specify a base when converting a string to an integer?
Yes, the `int()` function allows you to specify a base. For example, `int(“1010”, 2)` converts the binary string “1010” to the integer `10`.
Converting a string to an integer in Python is a straightforward process that can be accomplished using the built-in `int()` function. This function takes a string as its argument and returns the corresponding integer value. It is important to ensure that the string represents a valid integer; otherwise, a `ValueError` will be raised. For example, `int(“123”)` will yield the integer `123`, while `int(“abc”)` will result in an error.
In addition to the basic conversion, Python’s `int()` function also allows for the conversion of strings representing numbers in different bases. By providing a second argument that specifies the base, users can convert strings from binary, octal, or hexadecimal formats into integers. For instance, `int(“1010”, 2)` converts the binary string “1010” to the integer `10`.
Overall, understanding how to convert strings to integers is essential for data manipulation and processing in Python. This capability enables developers to handle user input, read data from files, and perform mathematical operations effectively. By leveraging the `int()` function, users can ensure their applications handle numerical data correctly and efficiently.
Author Profile
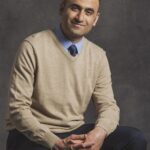
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?