Why Is My Set Object Not Subscriptable? Understanding the Error and How to Fix It
In the world of programming, particularly when working with Python, encountering errors is an inevitable part of the journey. One such error that often perplexes both beginners and seasoned developers alike is the “set object is not subscriptable” message. This seemingly cryptic phrase can halt your code in its tracks, leaving you scratching your head and searching for answers. Understanding this error is crucial not only for debugging your code but also for grasping the fundamental principles of data types and their behaviors in Python.
At its core, the “set object is not subscriptable” error arises from a fundamental misunderstanding of how sets operate in Python. Unlike lists or dictionaries, sets are unordered collections of unique elements, which means they do not support indexing or slicing. When you attempt to access a set using square brackets, as you would with a list, Python throws this error, signaling that you’re trying to treat a set like a subscriptable data type.
Delving deeper into this topic reveals not only the nature of sets but also the importance of choosing the right data structure for your programming needs. By exploring the characteristics of sets, their intended use cases, and the common pitfalls that lead to this error, you will gain a clearer understanding of how to effectively harness the power of
Understanding the Error
When you encounter the error message “set object is not subscriptable,” it indicates that you are trying to access an element of a set using indexing or subscript notation, which is not supported in Python. Sets are unordered collections of unique items, and they do not support indexing like lists or tuples.
In Python, attempting to access a set with an index will raise a `TypeError`. This is because sets do not maintain a specific order of elements, making it impossible to reference an item by its position.
Common Scenarios Leading to the Error
Several scenarios may lead to the “set object is not subscriptable” error:
- Direct Indexing: Trying to access an element directly using square brackets.
“`python
my_set = {1, 2, 3}
print(my_set[0]) Raises TypeError
“`
- Looping with Index: Using a loop to iterate over a set and mistakenly trying to access elements by their index.
“`python
my_set = {10, 20, 30}
for i in range(len(my_set)):
print(my_set[i]) Raises TypeError
“`
- Misunderstanding Set Behavior: Confusing sets with lists or tuples due to their similar usage in some contexts.
Correct Ways to Access Set Elements
To work with sets without encountering this error, you should use methods that are designed for set operations. Here are the appropriate techniques:
- Iteration: Use a `for` loop to iterate through the elements of the set.
“`python
my_set = {1, 2, 3}
for element in my_set:
print(element) Correct usage
“`
- Conversion: If you need indexed access, convert the set to a list or tuple.
“`python
my_set = {4, 5, 6}
my_list = list(my_set)
print(my_list[0]) Correctly accesses the first element
“`
- Using Functions: Functions such as `next()` can help retrieve elements in a controlled manner.
“`python
my_set = {7, 8, 9}
element = next(iter(my_set)) Retrieves one element from the set
print(element)
“`
Best Practices
To avoid the “set object is not subscriptable” error, consider the following best practices:
- Know Your Data Types: Be mindful of the data structures you are using and their properties.
- Use Appropriate Functions: Utilize built-in functions and methods designed for set operations.
- Convert When Necessary: If you need to access elements by index, convert your set to a list or tuple after ensuring that the order of elements is not crucial to your application logic.
Data Type | Subscriptable | Example |
---|---|---|
List | Yes | my_list[0] |
Tuple | Yes | my_tuple[1] |
Set | No | my_set[0] – Raises TypeError |
Understanding the Error: “Set Object is Not Subscriptable”
The error message “set object is not subscriptable” typically arises in Python when attempting to access elements of a set using indexing or slicing. Unlike lists or tuples, sets are unordered collections and do not support indexing, which leads to this error.
Why Sets Are Not Subscriptable
Sets in Python are designed to store unique elements in an unordered fashion. Key characteristics include:
- Unordered: Elements do not maintain a specific order.
- Unique Elements: Sets automatically handle duplicate entries, ensuring all items are distinct.
- Mutable: While the set itself can change (elements can be added or removed), the order of elements remains unpredictable.
Due to these properties, attempting to access a set item by an index (e.g., `my_set[0]`) is not possible, resulting in the error.
Common Scenarios Leading to the Error
Several coding scenarios can trigger this error. Here are some examples:
- Direct Indexing:
“`python
my_set = {1, 2, 3}
print(my_set[0]) Raises TypeError
“`
- Slicing Attempts:
“`python
my_set = {1, 2, 3}
print(my_set[0:2]) Raises TypeError
“`
- Using Set in List Comprehensions:
“`python
my_set = {1, 2, 3}
new_list = [my_set[i] for i in range(len(my_set))] Raises TypeError
“`
How to Access Elements in a Set
To access elements from a set without triggering the subscriptable error, consider the following approaches:
- Using Iteration:
“`python
my_set = {1, 2, 3}
for item in my_set:
print(item) Prints each item
“`
- Converting to a List:
If you need indexed access, convert the set to a list first:
“`python
my_set = {1, 2, 3}
my_list = list(my_set)
print(my_list[0]) Accesses the first element
“`
- Membership Testing:
To check if an element exists in the set:
“`python
my_set = {1, 2, 3}
if 2 in my_set:
print(“2 is in the set”)
“`
Best Practices for Working with Sets
To avoid errors and make the most of sets, consider the following best practices:
- Use sets when you need to maintain a collection of unique items.
- Avoid relying on the order of elements in a set; if order matters, consider using a list or an `OrderedDict`.
- Utilize set operations such as union, intersection, and difference to manage collections effectively.
Operation | Description | Example | |
---|---|---|---|
Union | Combines two sets | `set1 | set2` |
Intersection | Returns common elements | `set1 & set2` | |
Difference | Returns elements in one set but not the other | `set1 – set2` |
By understanding these principles and practices, you can effectively manage sets in Python without encountering the “set object is not subscriptable” error.
Understanding the “Set Object is Not Subscriptable” Error in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘set object is not subscriptable’ error occurs because sets in Python are unordered collections that do not support indexing. This design choice is intentional, as sets are meant to provide unique elements without a specific order, making them ideal for membership tests rather than sequential access.”
Mark Thompson (Lead Python Developer, CodeCraft Solutions). “When developers encounter the ‘set object is not subscriptable’ error, it often indicates a misunderstanding of data types. To access elements in a set, one should iterate over the set or convert it to a list if indexing is required. This highlights the importance of understanding the properties of different data structures in Python.”
Lisa Chen (Data Scientist, Analytics Hub). “This error serves as a reminder to carefully choose the right data structure for the task at hand. Sets are excellent for ensuring uniqueness and fast membership tests, but when order or indexing is needed, alternatives like lists or tuples should be utilized to avoid such errors.”
Frequently Asked Questions (FAQs)
What does “set object is not subscriptable” mean in Python?
The error “set object is not subscriptable” occurs when you attempt to access elements of a set using indexing or slicing, which is not allowed since sets are unordered collections.
How can I access elements in a set if I cannot use indexing?
To access elements in a set, you can use iteration with a loop or convert the set to a list or tuple, which are subscriptable data types.
What data types in Python are subscriptable?
Subscriptable data types in Python include lists, tuples, strings, and dictionaries. These types allow element access via indexing.
Can I convert a set to a list to avoid the “set object is not subscriptable” error?
Yes, you can convert a set to a list using the `list()` function, allowing you to access elements by index after the conversion.
What are some common scenarios that lead to this error?
Common scenarios include mistakenly trying to access a set element using square brackets or attempting to slice a set, both of which are invalid operations.
How can I avoid the “set object is not subscriptable” error in my code?
To avoid this error, ensure you use appropriate data structures for your needs. If you require ordered access, consider using a list or tuple instead of a set.
The error message “set object is not subscriptable” typically arises in Python programming when a developer attempts to access elements of a set using indexing or slicing. Sets in Python are unordered collections of unique elements, which means they do not support indexing like lists or tuples. This fundamental characteristic of sets is crucial for developers to understand to avoid runtime errors in their code.
When working with sets, it is essential to use methods such as `add()`, `remove()`, or `in` to manipulate or check for the presence of elements. Developers should familiarize themselves with the appropriate operations that can be performed on sets to leverage their unique properties effectively. Understanding these distinctions helps in writing more efficient and error-free code.
In summary, the “set object is not subscriptable” error serves as a reminder of the differences between data structures in Python. By recognizing that sets do not support indexing, developers can adjust their approach to data manipulation and avoid common pitfalls. This knowledge is vital for anyone looking to master Python programming and utilize its data structures effectively.
Author Profile
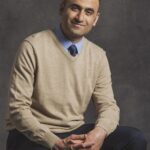
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?