Why Am I Seeing ‘Cannot Set Headers After They Are Sent to the Client’ Error?
In the world of web development, few errors can be as perplexing and frustrating as the infamous “Cannot set headers after they are sent to the client” message. This seemingly cryptic warning often leaves developers scratching their heads, trying to decipher its implications on their applications. As the backbone of modern web interactions, understanding how HTTP headers work is crucial for anyone looking to create seamless and efficient web experiences. Whether you’re a seasoned developer or just starting your journey, unraveling this error can significantly enhance your coding skills and improve your application’s performance.
At its core, the “Cannot set headers after they are sent to the client” error is a symptom of a deeper issue within the request-response cycle of a web server. When a server processes a request, it sends back a response, which includes headers that dictate how the client should handle the data. However, if a developer attempts to modify these headers after the response has already begun transmitting, the server raises this error, halting the process and leaving users with an incomplete experience. Understanding the timing and flow of data in web applications is essential to avoid this pitfall.
As we delve deeper into this topic, we will explore the common causes of this error, practical strategies for troubleshooting, and best practices for managing HTTP headers effectively
Understanding the Error
The error message “Cannot set headers after they are sent to the client” typically occurs in Node.js applications, particularly when using frameworks like Express. This situation arises when your code attempts to modify the HTTP response (such as setting headers or sending data) after the response has already been sent to the client.
When a response is sent, the HTTP protocol dictates that the headers are finalized. Any subsequent attempts to modify the headers will lead to this error. Understanding the flow of your code and the lifecycle of a request-response cycle is crucial in avoiding this error.
Common Scenarios Leading to the Error
Several scenarios can lead to this error, including:
- Multiple `res.send()` calls: If your code contains multiple calls to `res.send()`, the first call finalizes the response, causing any subsequent calls to fail.
- Asynchronous code: Using callbacks, promises, or async/await incorrectly can result in attempts to send a response after the initial response has been sent.
- Error handling: If an error occurs after a response has been sent, and an attempt is made to send an error response, this can trigger the error.
Best Practices to Prevent the Error
To avoid encountering the “Cannot set headers after they are sent to the client” error, consider the following best practices:
- Single Response Guarantee: Ensure that your route handlers send only one response. Use return statements to exit the function after sending a response.
- Control Flow Management: Carefully manage the flow of your asynchronous code. Consider using async/await to handle asynchronous operations more elegantly.
- Error Handling: Implement proper error handling to ensure that no response is sent after the initial response. Use middleware for error handling.
Example Code
Below is an illustrative example that demonstrates how to handle asynchronous operations and avoid the error:
“`javascript
app.get(‘/example’, async (req, res) => {
try {
const data = await someAsyncFunction();
if (!data) {
return res.status(404).send(‘Data not found’);
}
res.send(data);
} catch (error) {
console.error(‘Error occurred:’, error);
if (!res.headersSent) {
res.status(500).send(‘Internal Server Error’);
}
}
});
“`
In this example:
- The response is sent only once, and any return statements ensure no further code is executed after sending a response.
- The error handling checks if headers have already been sent before attempting to send an error response.
Table of Common Mistakes
Mistake | Description | Solution |
---|---|---|
Multiple res.send() | Calling res.send() more than once in the same request. | Use return statements to exit after the first res.send(). |
Asynchronous Mismanagement | Incorrectly handling asynchronous code, leading to multiple responses. | Use async/await or proper callbacks to manage flow. |
Ignoring res.headersSent | Trying to send a response after headers are already sent. | Check res.headersSent before sending an error response. |
By adhering to these practices, developers can effectively mitigate the occurrence of the “Cannot set headers after they are sent to the client” error, leading to more robust and maintainable applications.
Understanding the Error
The error message “Cannot set headers after they are sent to the client” typically occurs in server-side applications, particularly those built with Node.js and Express. This error arises when an attempt is made to modify the HTTP response after it has already been finalized and sent to the client.
Key Causes
- Multiple Response Calls: Invoking `res.send()`, `res.json()`, or similar methods more than once within the same request handler.
- Asynchronous Code: Using callbacks, promises, or async/await improperly can lead to attempts to send multiple responses.
- Middleware Mismanagement: Failing to call `next()` in middleware can lead to unexpected flow of control.
Common Scenarios
Several scenarios can lead to this error. Understanding these can help in debugging and preventing issues in your applications.
Scenario 1: Duplicate Response
“`javascript
app.get(‘/example’, (req, res) => {
res.send(‘First response’);
res.send(‘Second response’); // Error occurs here
});
“`
Scenario 2: Asynchronous Logic
“`javascript
app.get(‘/async-example’, async (req, res) => {
const result = await someAsyncFunction();
res.send(result);
// If someAsyncFunction triggers an error,
// additional res.send() in the catch block may cause the error
});
“`
Scenario 3: Conditional Responses
“`javascript
app.get(‘/conditional’, (req, res) => {
if (someCondition) {
res.send(‘Condition met’);
}
// If someCondition is true, but another part of the code tries to send a response
res.send(‘This will cause an error’); // Error occurs if the condition is met
});
“`
Best Practices to Avoid the Error
Implementing best practices can help minimize the occurrence of this error:
– **Single Response**: Ensure that each request handler sends a response only once.
– **Return After Sending**: Use `return` statements after sending responses to prevent further execution.
“`javascript
app.get(‘/single-response’, (req, res) => {
if (conditionMet) {
return res.send(‘Response sent’);
}
res.send(‘Another response’); // This will not execute if conditionMet is true
});
“`
- Error Handling: Utilize proper error handling techniques to manage asynchronous code effectively.
Debugging Strategies
If the error occurs, consider the following debugging strategies:
Strategy | Description |
---|---|
Console Logging | Log responses and flow control to trace where multiple sends may occur. |
Code Review | Review code for paths where a response could be sent multiple times. |
Use Middleware | Employ middleware for centralized error handling and response control. |
Testing | Write unit tests to cover edge cases where responses could be duplicated. |
Conclusion of Key Concepts
Addressing the “Cannot set headers after they are sent to the client” error requires careful attention to response flow and control in your application. By understanding the underlying causes and applying best practices, developers can create more robust and error-free server-side applications.
Understanding the “Cannot Set Headers After They Are Sent to the Client” Error
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error ‘cannot set headers after they are sent to the client’ typically arises when a server attempts to modify HTTP response headers after the response has already begun transmitting to the client. This is a common pitfall in asynchronous programming, where timing issues can lead to attempts to set headers post-response.”
James Lin (Lead Backend Developer, Cloud Solutions Group). “To avoid this error, developers should ensure that all header manipulations occur before any data is sent to the client. Utilizing middleware effectively can help to centralize header management and prevent premature response sending.”
Sarah Thompson (Full-Stack Developer, WebApp Masters). “Debugging this issue often involves tracing the flow of request handling in the application. Implementing proper error handling and logging can significantly aid in identifying where the headers are being incorrectly set after the response has started.”
Frequently Asked Questions (FAQs)
What does “cannot set headers after they are sent to the client” mean?
This error occurs in Node.js applications when an attempt is made to modify HTTP response headers after the response has already been sent to the client. Once the response is finalized and sent, headers cannot be changed.
What typically causes this error in a web application?
This error is often caused by asynchronous code execution, where a response is sent before all operations are completed. Common scenarios include multiple calls to `res.send()` or `res.end()`, or attempting to set headers after a response has already been sent.
How can I resolve the “cannot set headers after they are sent to the client” error?
To resolve this error, ensure that your response handling logic is structured correctly. Avoid sending multiple responses for a single request, and use return statements to prevent further execution after a response is sent.
Are there any best practices to avoid this error?
Best practices include using middleware to handle responses, ensuring that all asynchronous operations are completed before sending a response, and employing error handling to catch issues before they lead to sending headers after the response.
Can this error occur in frameworks other than Node.js?
While this specific error message is common in Node.js, similar issues can occur in other frameworks when attempting to modify response headers after the response has been sent. The underlying principle remains the same across different technologies.
What debugging techniques can help identify the source of this error?
Debugging techniques include logging the flow of your request handling, using breakpoints to trace execution, and checking for multiple response calls in your code. Additionally, reviewing asynchronous code paths can help identify where the response might be prematurely sent.
The error message “cannot set headers after they are sent to the client” is a common issue encountered in web development, particularly when working with Node.js and Express applications. This error typically arises when a developer attempts to modify the HTTP response headers after the response has already been sent to the client. Understanding the lifecycle of a request and response in web applications is crucial to preventing this error.
One of the primary causes of this error is the improper handling of asynchronous operations. When using callbacks, promises, or async/await, developers must ensure that they do not attempt to send multiple responses for a single request. This can happen if there are multiple paths of execution that lead to a response being sent, such as in conditional statements or error handling scenarios. It is essential to carefully manage the flow of the application to avoid such conflicts.
To mitigate this error, developers should implement best practices such as returning early from functions after sending a response, using middleware effectively, and ensuring that error handling is centralized. Additionally, logging and debugging can provide insight into where the response is being sent prematurely, allowing for more efficient troubleshooting. By adhering to these strategies, developers can enhance the reliability and robustness of their web applications.
Author Profile
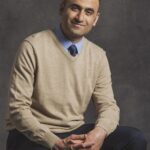
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?