Welcome to Araqev – Your Practical Guide to Programming
Technology moves fast, and whether you’re a seasoned developer or just getting started, keeping up with programming challenges can feel overwhelming. That’s why Araqev was created, to be a practical, no-nonsense guide to solving real-world coding issues.
Here, we don’t just throw definitions at you. We break down troubleshooting tips, best practices, and hands-on coding solutions in a way that makes sense. From CSS quirks to Kubernetes management, from debugging TypeScript to navigating Linux, we tackle everyday programming hurdles so you can focus on writing better code and building smarter applications.
No fluff, no unnecessary theory, just straightforward explanations and step-by-step solutions. Whether you’re fixing a stubborn Docker issue, optimizing your Go backend, or exploring hardware recommendations, you’ll find practical insights to help you code smarter, not harder.
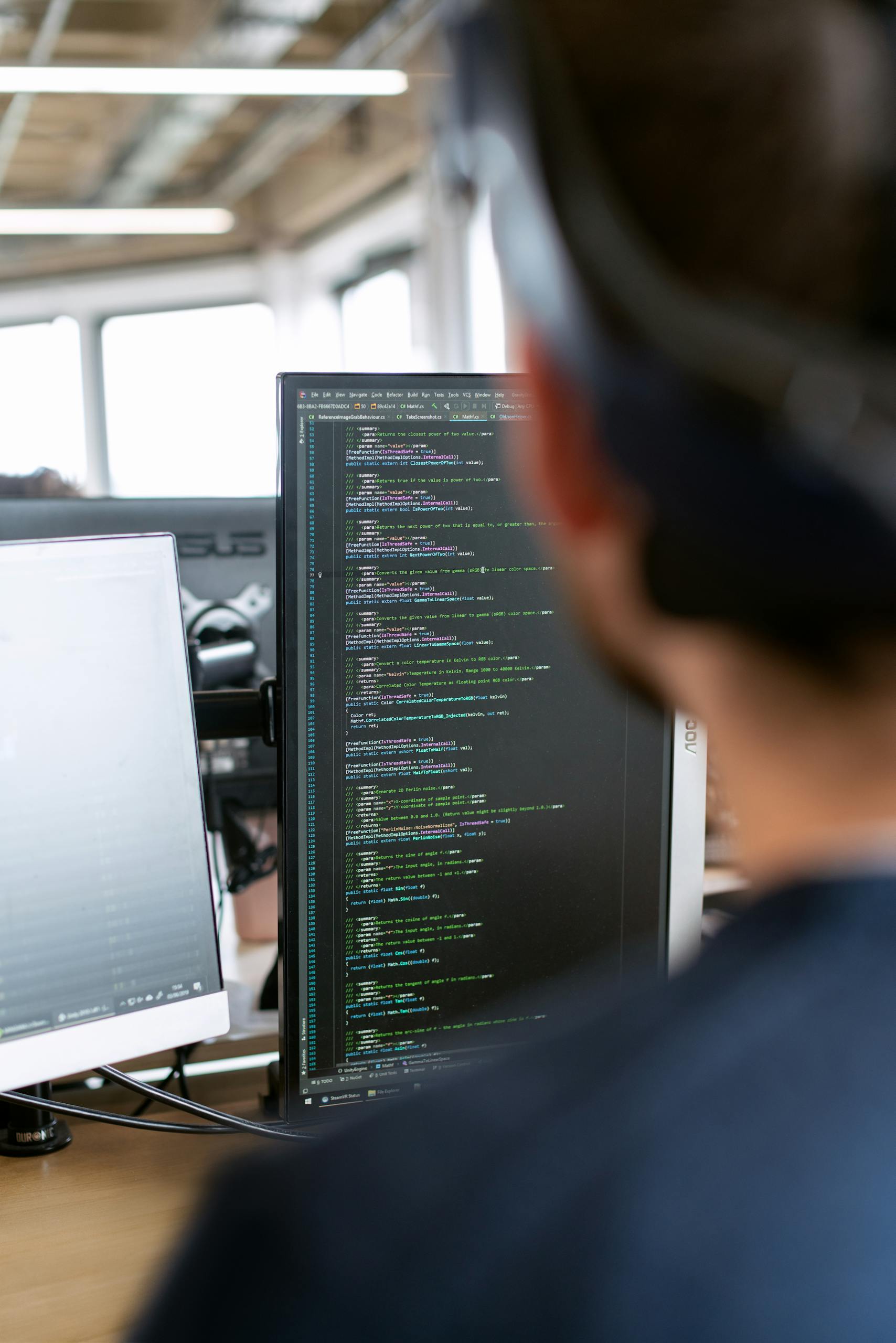
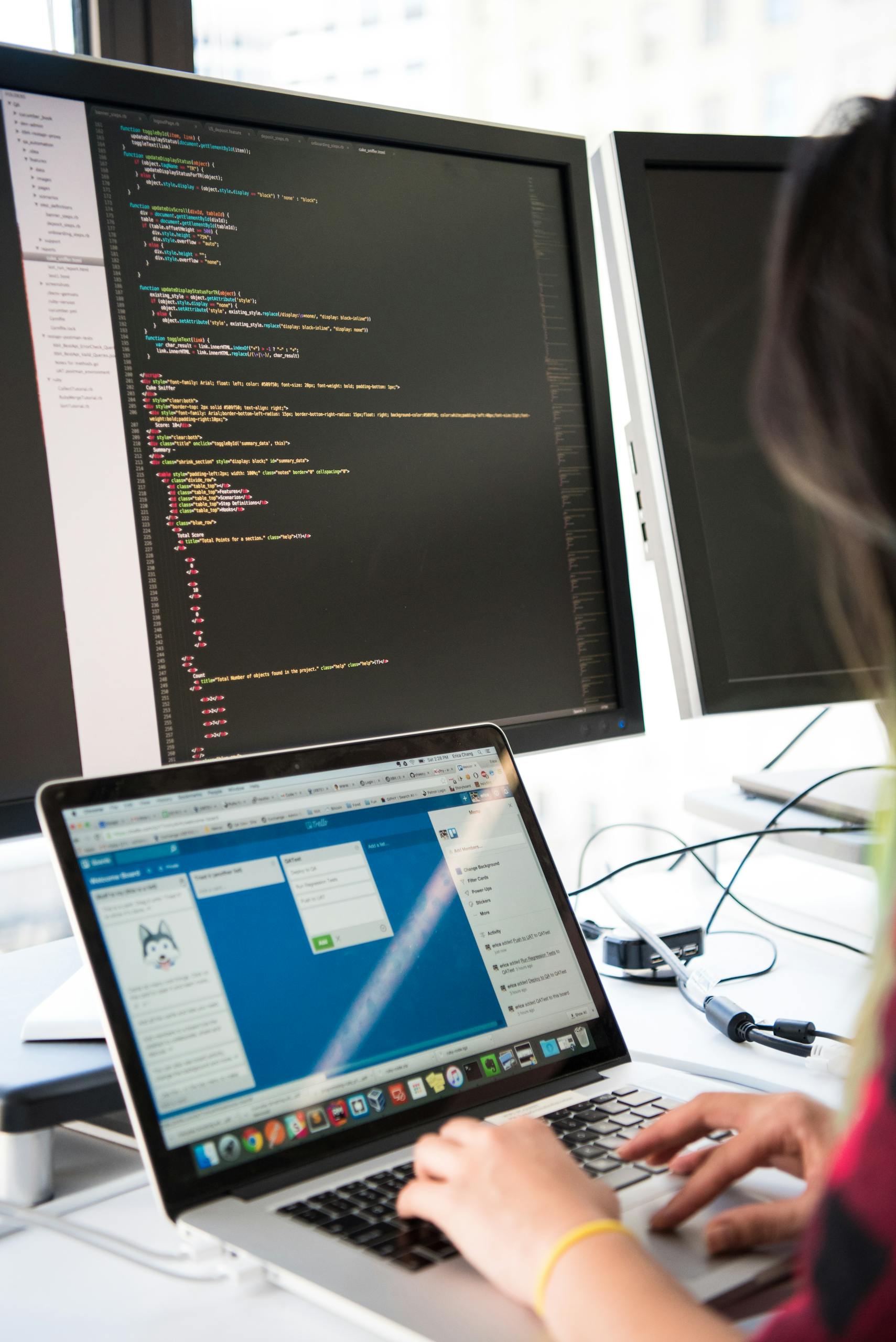
There’s More to Araqev Than Just Coding
At Araqev, we don’t stop at coding—we dive deep into the technical ecosystem that surrounds it. Writing clean CSS or debugging a tricky JavaScript function is just part of the picture. But what about setting up Docker containers, managing Kubernetes clusters, or optimizing your Ubuntu OS for peak performance? We’ve got that covered too.
Whether you’re troubleshooting hardware issues, configuring a Linux environment, or fine-tuning your WordPress site, we provide practical insights and hands-on solutions to keep your workflow smooth. From Go and Python programming to solving Stack Overflow’s toughest queries, Araqev is a space where developers, system admins, and tech enthusiasts can find real solutions for real-world problems.
Because in the world of technology, it’s never just about the code—it’s about everything that makes it work. And we’re here to help with all of it.
About the Author – Dr. Arman Sabbaghi
Dr. Arman Sabbaghi is a data scientist, programmer, and problem-solver with a background in machine learning, statistical modeling, and software optimization. With a Ph.D. in Statistics from Harvard University and years of research at Purdue University, he has worked extensively in AI-driven quality control, automation, and computational efficiency.
At Araqev, Dr. Sabbaghi brings his expertise to help developers, system admins, and tech enthusiasts navigate the ever-evolving world of programming, infrastructure, and troubleshooting. His goal is to simplify complex topics—whether it’s optimizing Python scripts, debugging Docker containers, or fine-tuning Linux setups.
Through this platform, he shares practical insights, step-by-step solutions, and expert advice to help readers solve problems faster and work smarter. Whether you’re coding, configuring, or troubleshooting, his mission is simple, to make technical challenges easier, one solution at a time.
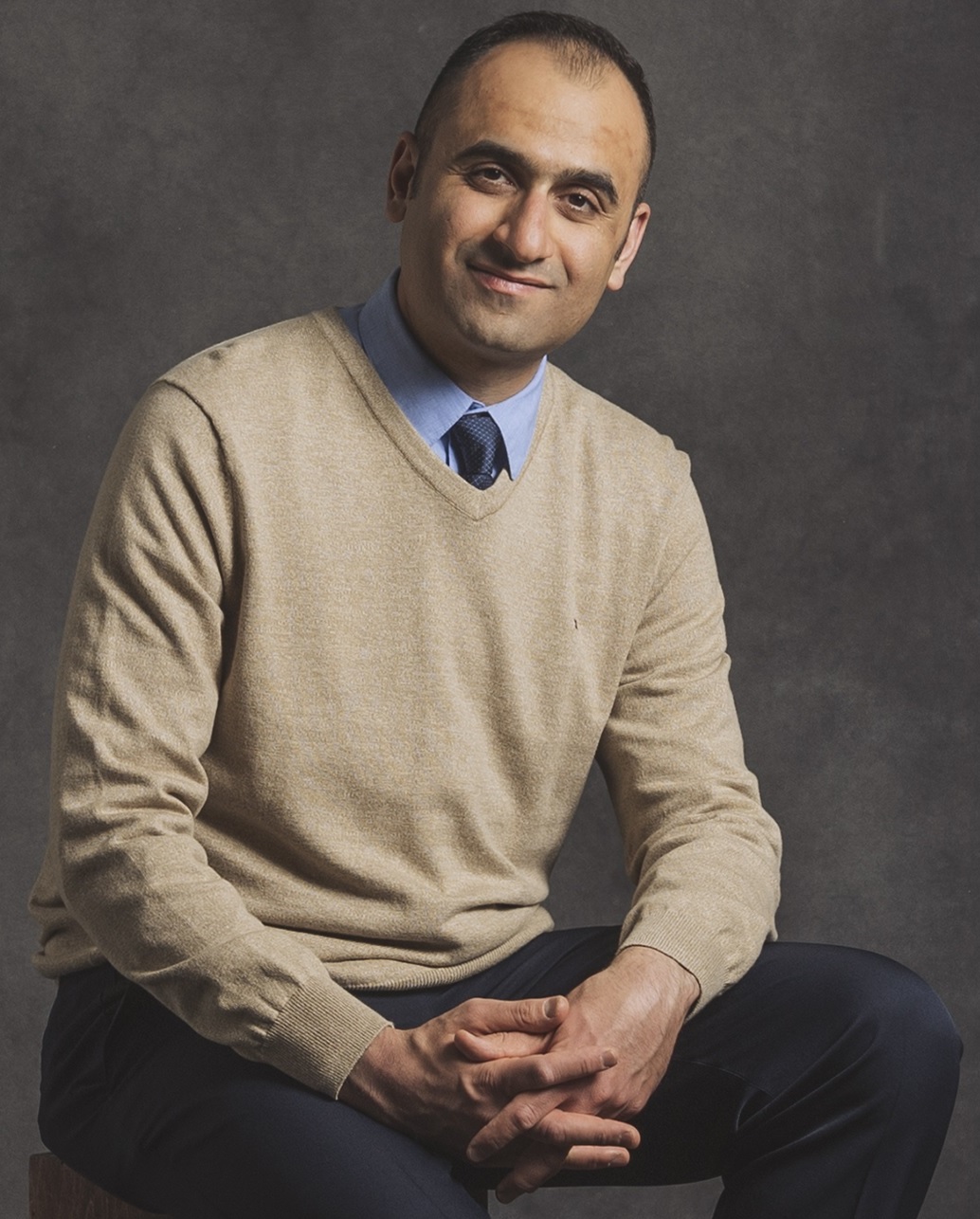
What Have We Covered In This Blog?
Mastering the Fundamentals
At Araqev, we focus on building a strong foundation in programming and system management. From understanding CSS for better styling to exploring the Go programming language, we break down key concepts into easy-to-follow, practical guides. We also cover essential scripting in JavaScript, Python, and TypeScript, helping developers write efficient, maintainable code that performs well across different platforms.
Solving Real-World Issues
Coding isn’t just about writing scripts—it’s about troubleshooting and optimizing. We tackle Stack Overflow’s trickiest questions, provide hardware recommendations, and guide you through fixing common Linux, Ubuntu, and WordPress issues. Whether it’s debugging a Node.js application or optimizing your server environment, we ensure you have the right tools and knowledge to overcome challenges with confidence.
Mastering DevOps and Containerization
For those managing servers and cloud environments, we dive deep into Docker, Kubernetes, and system administration. From handling containerization issues to improving Kubernetes cluster performance, our tutorials focus on real-world scenarios and best practices. If you’re looking to deploy, scale, and manage applications more efficiently, our guides help simplify even the most complex DevOps challenges.
Expanding Beyond Code
We believe that technology is more than just writing programs—it’s about understanding the systems that power them. That’s why we also explore hardware configurations, infrastructure management, and workflow optimizations. Whether you’re setting up a development environment, troubleshooting a server crash, or configuring JSON data handling, we provide step-by-step insights to make your tech experience seamless and productive.
What Makes Araqev Different?
In a world full of technical blogs and tutorials, Araqev stands out by focusing on real-world problem-solving rather than just theory. Many sites offer explanations, but we go further, we provide practical, step-by-step solutions that help you fix issues, optimize workflows, and improve efficiency.
What sets us apart is our holistic approach. We don’t just cover coding, we tackle everything that comes with it. From debugging tricky errors in JavaScript and Python to resolving Kubernetes and Docker issues, from hardware recommendations to fixing WordPress problems, we help you connect the dots between development, infrastructure, and troubleshooting.
This site is built by someone who understands real technical challenges, ensuring that every guide is clear, actionable, and actually useful. Whether you’re a developer, system admin, or tech enthusiast, Araqev is here to make your work smarter, faster, and less frustrating, one solution at a time.
Categories
Learn how to style web pages with CSS effectively. From layout techniques to responsive design, we cover everything you need to create visually appealing, fast-loading websites while avoiding common styling pitfalls and browser compatibility issues.
Master Docker and containerization with our practical guides. Whether you’re troubleshooting deployment errors or optimizing container performance, we provide step-by-step solutions to help you manage containerized applications efficiently across different environments.
Explore the Go programming language with hands-on tutorials and best practices. Learn about concurrency, performance optimization, and error handling while building scalable, high-performance applications suited for modern development needs.
Understand JSON for seamless data exchange and configuration. Learn how to parse, validate, optimize, and troubleshoot JSON structures, ensuring efficient data handling across APIs, databases, web applications, cloud-based systems with performance and security.
With this segment, You can enhance your JavaScript skills with in-depth tutorials on DOM manipulation, event handling, and modern frameworks. Learn how to debug errors, optimize performance, and build efficient, dynamic web applications using best practices.
Find expert advice on hardware troubleshooting and system optimization. Whether you’re selecting the best components for development setups or fixing performance issues, our guides help you make informed decisions for your hardware needs.
Streamline your Kubernetes workflow with expert guidance. We cover cluster deployment, scaling, security, and troubleshooting, ensuring efficient container orchestration for modern applications.
Master Linux administration with practical solutions for file management, security, server configuration, and system troubleshooting to optimize performance.
Develop fast, scalable applications with Node.js. In this category, we cover asynchronous server side programming, API development, error handling, and performance improvements.
Learn how TypeScript enhances JavaScript with type safety, better tooling, and structured development, improving code maintainability.
Solve real developer challenges with answers to common Stack Overflow queries, debugging strategies, and optimization techniques. No fluff, to the point answers.
Learn the most popular programming language. Hands-on guides covering automation, data processing, scripting, and performance optimization techniques.
Optimize your Ubuntu OS experience with expert tips on configuration, system management, security, and performance tuning.
Master WordPress with troubleshooting guides, plugin recommendations, security best practices, and performance tuning for better site management.
Fix common technical problems across multiple domains, from code errors to system failures, with expert-backed solutions.