Why Does System.IO.Ports.SerialPort Fail to Open with a USB Virtual COM Port?
In an era where seamless communication between devices is paramount, the integration of USB virtual COM ports with the .NET framework’s `System.IO.Ports.SerialPort` class has become a common necessity for developers. However, many encounter frustrating obstacles when attempting to open these ports, leading to delays in project timelines and increased troubleshooting efforts. Understanding the nuances of this interaction is crucial for anyone working with serial communications in a modern software environment. This article delves into the common pitfalls and solutions associated with using `System.IO.Ports.SerialPort` in conjunction with USB virtual COM ports, providing you with the insights needed to overcome these challenges.
When working with serial communication, the `System.IO.Ports.SerialPort` class serves as a vital bridge between your application and the hardware it interfaces with. Yet, the process of opening a USB virtual COM port can be fraught with complications. Factors such as driver compatibility, port configuration, and access permissions can all play a significant role in whether or not the port opens successfully. Developers often find themselves navigating a maze of potential issues, from misconfigured settings to conflicts with other applications that may be using the same port.
To effectively troubleshoot and resolve these issues, it’s essential to understand the underlying principles of how `System.IO.Ports.SerialPort` interacts with
Common Issues When Opening Serial Ports
When working with `System.IO.Ports.SerialPort` in conjunction with a USB virtual COM port, several issues may prevent successful connection. Understanding these common problems can help diagnose and resolve issues effectively.
- Driver Issues: Ensure that the correct drivers for the USB virtual COM port are installed. Faulty or outdated drivers can prevent the port from being recognized.
- Port Availability: The specified COM port may already be in use by another application or process. Check active connections to ensure that the port is free.
- Permissions: Insufficient permissions to access the COM port can lead to failures. Ensure that the application has the necessary rights to access the serial port.
- Incorrect Port Name: Verify that the port name specified in the code matches the actual name of the virtual COM port. Common names include “COM1”, “COM2”, etc.
Troubleshooting Steps
To troubleshoot the inability to open a serial port, follow these steps:
- Check Device Manager:
- Open Device Manager and locate the virtual COM port under “Ports (COM & LPT)”.
- Verify that it is listed without any warning icons.
- Use the Correct API Calls:
- Ensure the correct initialization and configuration of the `SerialPort` instance:
“`csharp
SerialPort serialPort = new SerialPort(“COM3”, 9600);
“`
- Testing with a Basic Application:
- Create a simple application that only opens the port to isolate the issue:
“`csharp
try {
serialPort.Open();
// Additional code for reading/writing
} catch (Exception ex) {
Console.WriteLine($”Error: {ex.Message}”);
}
“`
- Check for Exceptions:
- Implement exception handling to capture detailed error messages when attempting to open the port.
Configuration Parameters
The configuration of the `SerialPort` object is crucial for successful communication. Important parameters include:
Parameter | Description |
---|---|
BaudRate | The speed of data transmission (e.g., 9600) |
Parity | The parity bit setting (e.g., None, Odd, Even) |
DataBits | Number of data bits (usually 8) |
StopBits | Number of stop bits (1 or 2) |
Handshake | Flow control method (e.g., None, XOnXOff) |
Ensure that these parameters match the specifications of the connected device.
Additional Considerations
- Virtual COM Port Software: Sometimes, the software that creates the virtual COM port can introduce bugs. Ensure that it is up to date.
- Operating System Compatibility: Ensure compatibility with the operating system, as certain versions may have specific requirements or limitations regarding COM port usage.
- Testing on Another Machine: If issues persist, test the setup on a different computer to rule out hardware or OS-specific problems.
By carefully following these guidelines and troubleshooting steps, most issues related to opening a USB virtual COM port with `System.IO.Ports.SerialPort` can be effectively resolved.
Troubleshooting SerialPort Opening Issues
When working with the `System.IO.Ports.SerialPort` class in .NET, opening a connection to a USB virtual COM port can sometimes fail due to various reasons. The following troubleshooting steps can help identify and resolve these issues.
Common Reasons for Failure
- Port In Use: The specified COM port may already be open by another process. Ensure that no other application is using the port.
- Incorrect Port Name: Verify that the port name provided matches the actual COM port configured on the system (e.g., “COM3”).
- Driver Issues: Ensure that the USB virtual COM port drivers are correctly installed and up-to-date.
- Permissions: Lack of necessary permissions can prevent opening the port. Run the application with administrative privileges.
- Faulty Cable or Device: Physical hardware issues, such as a faulty USB cable or malfunctioning device, can also prevent successful connections.
Checking Available COM Ports
To confirm which COM ports are available on the system, you can use the following code snippet in C:
“`csharp
string[] ports = SerialPort.GetPortNames();
foreach (string port in ports)
{
Console.WriteLine(port);
}
“`
This will list all the currently available COM ports, allowing you to verify that the intended port is indeed accessible.
Example of Opening a Serial Port
Ensure that you are following best practices when attempting to open a serial port:
“`csharp
using System;
using System.IO.Ports;
class Program
{
static void Main()
{
try
{
using (SerialPort serialPort = new SerialPort(“COM3”, 9600))
{
serialPort.Open();
Console.WriteLine(“Port opened successfully.”);
// Additional logic here
}
}
catch (UnauthorizedAccessException ex)
{
Console.WriteLine(“Access denied: ” + ex.Message);
}
catch (IOException ex)
{
Console.WriteLine(“I/O error: ” + ex.Message);
}
catch (Exception ex)
{
Console.WriteLine(“Error: ” + ex.Message);
}
}
}
“`
This example includes exception handling for common issues that can arise when opening the port.
Using Device Manager for Verification
To verify that the USB virtual COM port is recognized by the operating system, follow these steps:
- Open Device Manager.
- Expand the Ports (COM & LPT) section.
- Identify the USB virtual COM port in the list (e.g., “USB Serial Port (COM3)”).
- Check for any warning icons that indicate driver issues.
Device Connection Steps
When setting up a USB virtual COM port, ensure the following steps are followed:
- Install Drivers: Ensure that the appropriate drivers for the USB device are installed.
- Connect the Device: Plug the USB device into the computer and confirm that it is recognized in Device Manager.
- Select the Correct COM Port: Make sure to use the correct COM port number in your application.
Testing with Other Software
If the connection still fails, consider testing the USB virtual COM port with terminal software such as:
- PuTTY
- Tera Term
- HyperTerminal
These tools can help isolate whether the issue lies with the hardware or the code implementation.
Logging and Debugging
Implement logging to capture detailed information about the port opening process. This can include timestamps, error messages, and stack traces to facilitate debugging:
“`csharp
// Example logging
catch (Exception ex)
{
LogError(“Error opening port: ” + ex.Message);
}
“`
Utilizing comprehensive logging allows for better diagnosis of issues as they arise during development and testing.
Challenges and Solutions for Serial Port Communication with USB Virtual COM Ports
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Corp). “When encountering issues with the `system.io.ports.serialport` failing to open a USB virtual COM port, it is essential to verify that the drivers for the virtual COM port are correctly installed and up to date. Many failures stem from outdated or incompatible drivers that prevent proper communication between the software and the hardware.”
Michael Zhang (Embedded Systems Specialist, Circuit Solutions LLC). “Another common reason for the failure to open a USB virtual COM port is the port being already in use by another application. It is advisable to check for any background processes that may be accessing the port, as this can lead to conflicts that result in the serial port not opening.”
Jessica Lee (IT Support Manager, ConnectTech Services). “In some cases, the issue may arise from improper configuration settings within the application trying to access the serial port. Ensuring that the baud rate, parity, and other communication settings match those expected by the device can resolve many connectivity problems.”
Frequently Asked Questions (FAQs)
Why does my USB virtual COM port fail to open using system.io.ports.serialport?
The failure to open a USB virtual COM port typically results from incorrect port settings, the port being already in use, or driver issues. Ensure that the correct COM port number is specified and that no other application is accessing it.
What steps can I take to troubleshoot the issue with system.io.ports.serialport?
Begin by checking the device manager for the correct COM port assignment. Verify that the drivers for the USB virtual COM port are up to date. Additionally, ensure that the port is not being utilized by another application.
Are there specific permissions required to access a USB virtual COM port?
Yes, accessing a USB virtual COM port may require administrative privileges, especially on Windows systems. Ensure that your application runs with the necessary permissions to interact with the hardware.
Can I use system.io.ports.serialport with multiple USB virtual COM ports simultaneously?
Yes, you can use system.io.ports.serialport with multiple USB virtual COM ports. However, each port must be managed in separate instances of the SerialPort class to avoid conflicts.
What exceptions should I expect when opening a USB virtual COM port?
Common exceptions include UnauthorizedAccessException, which indicates permission issues, and IOException, which may occur if the port is already in use or if there are hardware communication problems.
How can I ensure that my application properly closes the USB virtual COM port?
Always call the Close() method on the SerialPort instance when finished. Implementing a try-finally block ensures that the port closes even if an exception occurs during communication.
The issue of the System.IO.Ports.SerialPort class failing to open a USB virtual COM port can stem from several factors. First and foremost, it is essential to ensure that the correct port name is being used within the application. The port name must match the one assigned by the operating system, which can sometimes differ from what is expected. Additionally, users should verify that the USB virtual COM port is properly installed and recognized by the system, as driver issues can lead to failure in establishing a connection.
Another critical aspect to consider is the permissions associated with accessing the serial port. On certain operating systems, particularly those with stricter security settings, administrative privileges may be required to open a COM port. Users must ensure that their application has the necessary permissions to access the hardware resources. Furthermore, it is advisable to check if the port is already in use by another application, as this can prevent new connections from being established.
Lastly, troubleshooting steps such as testing the USB virtual COM port with a different serial communication tool can help isolate the problem. If the port works with other software but not with the System.IO.Ports.SerialPort class, it may indicate a specific configuration issue within the application itself. Ensuring that the application is correctly handling exceptions
Author Profile
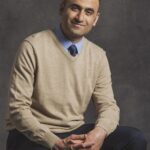
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?