What is ‘i’ in Python? Understanding Its Role and Usage
In the world of Python programming, the letter “i” often emerges as a seemingly simple yet profoundly significant character. For beginners and seasoned developers alike, understanding what “i” represents can unlock a deeper comprehension of coding practices and conventions within the language. Whether it’s used in loops, as a variable, or even as a placeholder, “i” serves as a cornerstone in Python’s syntax and functionality, embodying the elegance and efficiency that the language is celebrated for. As we delve into the nuances of this ubiquitous character, we will uncover its various roles and the context in which it thrives, enriching your Python programming journey.
At its core, “i” is frequently employed as a loop counter in iterative constructs, particularly in for-loops and while-loops. This common usage stems from its historical roots in mathematics and computer science, where “i” often denotes an index or an integer. However, its application extends beyond mere iteration; “i” can also be a versatile variable name in functions, comprehensions, and even in complex data structures. Understanding how and when to use “i” effectively can enhance code readability and maintainability, making it a valuable tool in any programmer’s toolkit.
Moreover, the simplicity of “i” belies its importance in the broader context
Understanding the Role of ‘i’ in Python
In Python, the variable `i` is commonly used as a loop index in various types of loops, particularly in `for` and `while` loops. While `i` is not a keyword or a reserved word in Python, it has become a convention among programmers to denote an integer counter or index. This tradition stems from its use in mathematics and programming languages like C, C++, and Java.
The use of `i` is not limited to loops; it can also be employed in functions, list comprehensions, and other constructs. Its flexibility as a variable name allows for concise and readable code, particularly in iterations.
Common Usage of ‘i’ in Loops
When employed in loops, `i` typically serves as an iterator that traverses through a sequence such as a list, tuple, or string. Below are some examples illustrating its use:
- For Loop Example:
python
for i in range(5):
print(i)
In this example, `i` takes on the values from 0 to 4, iterating five times.
- While Loop Example:
python
i = 0
while i < 5:
print(i)
i += 1
Here, `i` is initialized before the loop and incremented during each iteration.
Best Practices for Using ‘i’
While using `i` as a loop variable is conventional, it is essential to consider best practices for readability and maintainability of code:
- Descriptive Naming: In cases where the loop variable’s purpose is not clear, consider using more descriptive names. For instance, `index` or `count` can enhance understanding.
- Scoped Usage: Limit the scope of `i` to the block where it is needed to avoid unintended side effects in larger functions.
Examples of ‘i’ in Different Contexts
The variable `i` can serve multiple purposes beyond iteration. Below are various contexts where `i` might be used:
Context | Example |
---|---|
Loop Iteration |
for i in range(10): print(i)
|
List Comprehension |
squared = [i**2 for i in range(10)]
|
Function Argument |
def process(i): return i * 2
|
while `i` is often used as a simple loop index, its application in Python can be diverse. It is important to maintain clarity in code through appropriate naming conventions and context, ensuring that the purpose of `i` is easily understood by anyone reading the code.
Understanding the Variable `i` in Python
In Python, `i` is often used as a conventional variable name, particularly in loops and iterations. Its usage can vary based on context, but it typically serves as a counter or index.
### Common Usage of `i`
- Looping Constructs: In loops, `i` is frequently employed as the loop index.
python
for i in range(5):
print(i)
- List Comprehensions: It is also prevalent in list comprehensions, where `i` represents each element in an iterable.
python
squares = [i ** 2 for i in range(10)]
### Contextual Meaning
While `i` itself does not have a specific meaning in Python, its significance lies in its role as a placeholder for values. The choice of `i` is largely based on tradition, especially in mathematical contexts where it is commonly used as an index variable.
### Best Practices for Using `i`
- Clarity: Although `i` is a widely accepted convention, it is advisable to use more descriptive variable names in more complex code blocks for better readability.
- Scope: Ensure that the use of `i` does not conflict with other variables in the same scope, particularly in nested loops.
### Example Scenarios
Scenario | Example Code | Description |
---|---|---|
Basic Loop | `for i in range(10): print(i)` | Iterates from 0 to 9, printing each value. |
Nested Loop | `for i in range(3): for j in range(2): print(i, j)` | Nested iteration, printing pairs of indices. |
List Comprehension | `evens = [i for i in range(20) if i % 2 == 0]` | Generates a list of even numbers from 0 to 19. |
### Alternative Variable Names
In scenarios where more clarity is needed, consider using alternative variable names:
- `index` for a clearer indication of its role as an index.
- `counter` when counting iterations.
- `item` when iterating through collections.
### Conclusion
The variable `i` serves as a versatile tool in Python programming, primarily as a loop counter or index. Its simplicity and convention make it a popular choice, but developers should prioritize clarity and context when naming variables in their code.
Understanding the Role of ‘i’ in Python Programming
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, ‘i’ is commonly used as a loop variable in iterative constructs such as for loops. It serves as a convention that enhances code readability, particularly in contexts where the loop’s purpose is clear, such as iterating through a range of numbers.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “The variable ‘i’ is not inherently special in Python; it is simply a name that developers often use to represent an index or iterator. Its widespread usage in programming stems from its historical roots in mathematics and computer science, where it frequently denotes an integer variable.”
Sarah Thompson (Educational Content Creator, Python Programming Academy). “When teaching Python, I emphasize that while ‘i’ is a traditional choice for loop counters, developers should prioritize meaningful variable names that convey the purpose of the data they represent. This practice leads to more maintainable and understandable code, especially in larger projects.”
Frequently Asked Questions (FAQs)
What is the purpose of the variable ‘i’ in Python?
The variable ‘i’ is commonly used as a loop counter in Python, particularly in for and while loops. It serves as an index to iterate over sequences or to count iterations.
Is ‘i’ a special keyword in Python?
No, ‘i’ is not a special keyword in Python. It is simply a conventional name used by programmers, especially in contexts involving loops and iterations.
Can I use a different variable name instead of ‘i’?
Yes, you can use any valid variable name instead of ‘i’. However, ‘i’ is widely recognized, making code easier to understand for those familiar with common programming practices.
What type of data can ‘i’ hold in Python?
The variable ‘i’ can hold any data type, including integers, floats, strings, or even objects, depending on how it is defined and used within the code.
How does the use of ‘i’ in loops affect performance?
The use of ‘i’ as a loop counter does not inherently affect performance. The performance is more dependent on the complexity of the loop’s body and the operations performed within it.
Are there any conventions for naming loop counters in Python?
While there are no strict conventions, it is common to use single-letter variable names like ‘i’, ‘j’, and ‘k’ for loop counters, especially in nested loops, to maintain clarity and brevity in code.
In Python, the keyword ‘i’ is commonly used as a variable name, particularly in loops and iterations. It is often employed as an index variable in for loops, especially when iterating over a range of numbers. The choice of ‘i’ is largely conventional, stemming from its historical use in mathematics and programming, where it typically represents an integer. However, it is important to note that ‘i’ is not a reserved keyword in Python, meaning that developers can use it freely as a variable name without any special significance beyond its role as a placeholder for values.
Moreover, the use of ‘i’ can enhance code readability when used in contexts where its meaning is clear, such as counting iterations or indexing elements in a list. However, developers should also be mindful of the scope and context in which they use ‘i’ to avoid confusion, especially in larger codebases where multiple indices may be present. The clarity of variable names is essential for maintaining code quality and comprehensibility.
In summary, while ‘i’ is a widely accepted convention in Python programming, its effectiveness hinges on the programmer’s ability to use it appropriately within the context of the code. Choosing meaningful variable names that convey their purpose can significantly improve the maintain
Author Profile
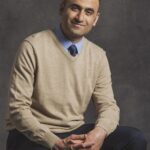
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?