How Do You Perform an Update with Inner Join in SQL?
Update Inner Join in SQL: A Powerful Tool for Data Management
In the world of database management, the ability to efficiently update records is paramount for maintaining data integrity and relevance. One of the most effective techniques for executing updates across multiple tables is the use of the `UPDATE INNER JOIN` statement in SQL. This powerful command allows developers and database administrators to synchronize information seamlessly, ensuring that related data remains consistent and accurate. Whether you’re managing customer records, inventory systems, or complex relational databases, mastering this SQL functionality can significantly enhance your data manipulation capabilities.
The `UPDATE INNER JOIN` operation combines the strengths of both the `UPDATE` and `INNER JOIN` commands, enabling users to modify records in one table based on matching criteria from another. This approach not only streamlines the update process but also minimizes the risk of errors that can arise from handling multiple queries. By understanding how to leverage this technique, you can perform bulk updates efficiently, saving time and resources while ensuring that your database reflects the most current information.
As we delve deeper into the mechanics of `UPDATE INNER JOIN`, we will explore its syntax, practical applications, and best practices. This knowledge will empower you to execute complex updates with confidence, transforming the way you interact with your data and enhancing your overall database management strategy
Understanding INNER JOIN in SQL
An INNER JOIN in SQL is a method of combining rows from two or more tables based on a related column between them. It effectively filters out records that do not match the specified criteria. This is particularly useful when you want to update records in one table using values from another table.
When performing an update with an INNER JOIN, you can specify which records in the target table should be updated based on matching values in the joined table. This approach ensures that only relevant records are modified, maintaining data integrity.
Syntax for Update with INNER JOIN
The basic syntax for updating records using an INNER JOIN is as follows:
“`sql
UPDATE target_table
SET target_table.column_name = new_value
FROM target_table
INNER JOIN source_table ON target_table.common_column = source_table.common_column
WHERE condition;
“`
- target_table: The table whose records you want to update.
- source_table: The table from which you want to pull data.
- common_column: The column used to join both tables.
- new_value: The value you want to set.
- condition: Additional conditions to filter the records.
Example of Update with INNER JOIN
Consider two tables: `employees` and `departments`. The `employees` table contains employee details, while the `departments` table holds department information. Assume you want to update the department name of employees based on their department ID.
Here’s how you might write that SQL statement:
“`sql
UPDATE employees
SET employees.department_name = departments.department_name
FROM employees
INNER JOIN departments ON employees.department_id = departments.id
WHERE departments.location = ‘New York’;
“`
In this example:
- The department name in the `employees` table is updated to match the corresponding name in the `departments` table for those employees whose department is located in New York.
Important Considerations
When using INNER JOIN in an update statement, there are several important points to consider:
- Ensure that the JOIN condition is specific enough to avoid unintended updates.
- Be cautious with the WHERE clause to limit the scope of the update.
- Test your JOIN and update logic using a SELECT statement before executing the UPDATE to verify the records that will be affected.
Common Use Cases
The INNER JOIN in update operations can be applied in various scenarios, including:
- Updating employee salaries based on performance reviews stored in another table.
- Modifying product prices based on supplier information.
- Changing customer statuses based on recent transaction data.
Table of Example Data
employees | department_id | department_name |
---|---|---|
John Doe | 1 | Sales |
Jane Smith | 2 | Marketing |
departments | id | department_name | location |
---|---|---|---|
Sales | 1 | Sales | New York |
Marketing | 2 | Marketing | Los Angeles |
By employing INNER JOIN in your UPDATE statements, you can streamline the process of maintaining and updating data across related tables.
Understanding the Update Inner Join Syntax
The `UPDATE` statement in SQL allows you to modify existing records in a table. When combined with an `INNER JOIN`, it enables updates across multiple tables based on a specified relationship. The general syntax for performing an `UPDATE` with an `INNER JOIN` is as follows:
“`sql
UPDATE table1
SET table1.column1 = value1,
table1.column2 = value2
FROM table1
INNER JOIN table2 ON table1.common_column = table2.common_column
WHERE table2.condition_column = condition_value;
“`
Key Components of the Syntax
- UPDATE table1: Specifies the primary table where the updates will occur.
- SET: Defines which columns in `table1` to update and their new values.
- FROM: Indicates the tables involved in the join operation.
- INNER JOIN: Joins `table1` with `table2` based on the specified condition.
- WHERE: Filters the records that meet certain criteria.
Example of Update Inner Join
Consider two tables: `employees` and `departments`. The `employees` table contains employee details, while the `departments` table has department information. Suppose you want to update the salary of employees based on their department’s budget.
“`sql
UPDATE employees
SET employees.salary = employees.salary * 1.10
FROM employees
INNER JOIN departments ON employees.department_id = departments.id
WHERE departments.budget > 50000;
“`
In this example:
- Employees in departments with a budget greater than 50,000 receive a 10% salary increase.
Important Considerations
- Ensure that the `INNER JOIN` condition accurately reflects the relationship between the tables to avoid unintended updates.
- Always back up your data before performing mass updates.
Common Use Cases
Using `UPDATE` with `INNER JOIN` can be particularly useful in several scenarios:
- Data Synchronization: Keeping related data consistent across multiple tables.
- Bulk Updates: Efficiently updating records based on related table criteria.
- Conditional Updates: Applying updates only when certain conditions in joined tables are met.
Example Use Cases
Use Case | Description |
---|---|
Salary Adjustment | Increase salaries based on department performance. |
Status Updates | Change order statuses based on customer feedback. |
Inventory Management | Update stock levels based on supplier deliveries. |
Best Practices
When using `UPDATE` with `INNER JOIN`, adhere to the following best practices:
- Test on a Subset: Always test your query on a small dataset to ensure it works as expected before applying it to the entire database.
- Transaction Management: Use transactions to allow rollback in case of errors during the update process.
- Logging Changes: Maintain a log of changes for auditing purposes, especially in production environments.
By following these guidelines, you can effectively and safely perform updates across related tables using `INNER JOIN` in SQL.
Expert Insights on Updating with Inner Join in SQL
Dr. Emily Chen (Database Architect, Tech Innovations Inc.). “When performing an update with an inner join in SQL, it is crucial to ensure that the join condition accurately reflects the relationship between the tables. This prevents unintended data modifications and maintains data integrity.”
Mark Thompson (Senior SQL Developer, Data Solutions Group). “Utilizing inner joins in update statements can significantly enhance performance by limiting the rows affected. However, developers must be cautious with the logic used to avoid updating incorrect records.”
Linda Garcia (Data Analyst, Insights Analytics). “Incorporating inner joins in update queries allows for more complex data manipulation. It is essential to test these queries in a safe environment before executing them in production to prevent data loss.”
Frequently Asked Questions (FAQs)
What is an inner join in SQL?
An inner join in SQL is a type of join that returns only the rows from both tables that satisfy the join condition. It combines records from two or more tables based on a related column between them.
How do you perform an update with an inner join in SQL?
To perform an update with an inner join, you use the UPDATE statement combined with the INNER JOIN clause. This allows you to update records in one table based on matching records in another table.
Can you provide an example of an update inner join in SQL?
Certainly. An example would be:
“`sql
UPDATE table1
SET table1.column_name = new_value
FROM table1
INNER JOIN table2 ON table1.id = table2.id
WHERE table2.condition = some_value;
“`
What are the benefits of using inner join in an update statement?
Using an inner join in an update statement allows for precise updates by ensuring that only the records that meet specific criteria across multiple tables are modified, enhancing data integrity and accuracy.
Are there any limitations when using update inner join in SQL?
Yes, limitations may include restrictions on the number of tables that can be joined, potential performance issues with large datasets, and the requirement for the join condition to be properly defined to avoid unintended updates.
Is it possible to update multiple tables using inner join in SQL?
No, SQL does not allow updating multiple tables in a single update statement. However, you can execute multiple update statements sequentially using inner joins to achieve the desired outcome across different tables.
In SQL, an inner join is a powerful operation that allows users to combine rows from two or more tables based on a related column between them. When performing an update operation using an inner join, the goal is typically to modify records in one table based on criteria that involve matching records in another table. This process requires a clear understanding of both the syntax and the logic behind the join to ensure that the correct records are updated.
To execute an update with an inner join, the SQL syntax generally involves specifying the target table to update, followed by the inner join clause that links the target table with the source table. The update statement will also include a set clause to define the new values for the columns being updated. This approach is efficient as it allows for precise modifications based on the relationships defined in the joined tables.
Key takeaways from the discussion on updating with inner joins include the importance of ensuring that the join conditions are correctly defined to avoid unintended updates. Additionally, it is crucial to test the update query in a safe environment before executing it on production data. Understanding the implications of the inner join will aid in maintaining data integrity and achieving the desired outcomes in database management.
Author Profile
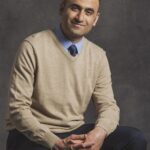
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?