How Can You Create a Database Using Python?
Creating a database in Python is an essential skill for developers and data enthusiasts alike, as it opens the door to efficient data management and retrieval. Whether you’re building a web application, analyzing data, or simply organizing information, understanding how to create a database can significantly enhance your programming toolkit. With Python’s versatility and the plethora of libraries available, the process is not only straightforward but also highly customizable to suit your specific needs.
In the world of programming, databases serve as the backbone for storing and managing data. Python offers several libraries, such as SQLite, SQLAlchemy, and PostgreSQL, that simplify the creation and manipulation of databases. These tools allow you to define the structure of your data, execute queries, and perform transactions seamlessly. As you delve deeper into the topic, you’ll discover how to set up a database, create tables, and interact with your data using Python’s powerful syntax and commands.
Moreover, understanding database design principles and best practices is crucial for building efficient applications. From choosing the right database type to implementing relationships between tables, the foundational knowledge you gain will empower you to develop robust systems that can scale with your needs. So, whether you’re a beginner eager to learn or an experienced programmer looking to refine your skills, this guide will walk you through the essential steps to create
Choosing a Database System
When creating a database in Python, the first step is to choose an appropriate database system based on your project requirements. Python supports various database systems, each with its unique features. Common options include:
- SQLite: A lightweight, file-based database that is easy to set up and use.
- PostgreSQL: An advanced, open-source object-relational database known for its robustness and scalability.
- MySQL: A widely-used relational database management system, ideal for web applications.
- MongoDB: A NoSQL database that stores data in flexible, JSON-like documents.
Consider the following factors when choosing a database system:
- Complexity of data
- Scalability requirements
- Development speed
- Community and support resources
Setting Up SQLite Database
For many applications, especially smaller projects, SQLite is an excellent choice due to its simplicity. To set up an SQLite database in Python, follow these steps:
- Import the SQLite module:
“`python
import sqlite3
“`
- Create a connection to the database file:
“`python
connection = sqlite3.connect(‘example.db’)
“`
- Create a cursor object to execute SQL commands:
“`python
cursor = connection.cursor()
“`
- Define a table structure using SQL commands:
“`python
cursor.execute(”’
CREATE TABLE IF NOT EXISTS users (
id INTEGER PRIMARY KEY,
name TEXT NOT NULL,
age INTEGER
)
”’)
“`
- Insert data into the table:
“`python
cursor.execute(”’
INSERT INTO users (name, age) VALUES (?, ?)
”’, (‘Alice’, 30))
“`
- Commit the changes and close the connection:
“`python
connection.commit()
connection.close()
“`
Using PostgreSQL with Psycopg2
If your project demands a more robust solution, PostgreSQL is a great option. To interact with a PostgreSQL database using Python, you will typically use the Psycopg2 library. Here’s how to set it up:
- Install Psycopg2 using pip:
“`bash
pip install psycopg2
“`
- Import the library in your Python script:
“`python
import psycopg2
“`
- Establish a connection to your PostgreSQL database:
“`python
connection = psycopg2.connect(
dbname=’your_database’,
user=’your_username’,
password=’your_password’,
host=’localhost’
)
“`
- Create a cursor to execute SQL commands:
“`python
cursor = connection.cursor()
“`
- Create a table and perform operations:
“`python
cursor.execute(”’
CREATE TABLE IF NOT EXISTS products (
product_id SERIAL PRIMARY KEY,
product_name VARCHAR(100),
price DECIMAL
)
”’)
“`
- Insert data into the table:
“`python
cursor.execute(”’
INSERT INTO products (product_name, price) VALUES (%s, %s)
”’, (‘Laptop’, 999.99))
“`
- Commit the changes and close the connection:
“`python
connection.commit()
cursor.close()
connection.close()
“`
Database Type | Use Case | Library |
---|---|---|
SQLite | Small applications, local development | sqlite3 |
PostgreSQL | Enterprise applications, complex queries | psycopg2 |
MySQL | Web applications, high-read scenarios | mysql-connector-python |
MongoDB | Flexible data structures, large datasets | pymongo |
Choosing a Database Type
When creating a database in Python, the first step is to determine the type of database that best suits your application’s needs. The main categories include:
- Relational Databases: Use structured query language (SQL) for defining and manipulating data. Examples include:
- PostgreSQL
- MySQL
- SQLite
- NoSQL Databases: Designed for unstructured data and can handle various data formats. Examples include:
- MongoDB
- Cassandra
- Redis
Setting Up a Relational Database with SQLite
SQLite is a popular choice for beginners due to its simplicity and lightweight nature. It requires no server setup and is included with Python’s standard library.
- Install SQLite (if not already installed):
“`bash
pip install sqlite3
“`
- Create a Database Connection:
“`python
import sqlite3
Connect to the database (or create it if it doesn’t exist)
connection = sqlite3.connect(‘my_database.db’)
cursor = connection.cursor()
“`
- Create a Table:
“`python
cursor.execute(”’
CREATE TABLE users (
id INTEGER PRIMARY KEY,
name TEXT NOT NULL,
age INTEGER NOT NULL
)
”’)
“`
- Insert Data:
“`python
cursor.execute(”’
INSERT INTO users (name, age) VALUES (?, ?)
”’, (‘Alice’, 30))
connection.commit()
“`
- Querying Data:
“`python
cursor.execute(‘SELECT * FROM users’)
rows = cursor.fetchall()
for row in rows:
print(row)
“`
- Close the Connection:
“`python
connection.close()
“`
Using a NoSQL Database with MongoDB
MongoDB is a widely-used NoSQL database that stores data in JSON-like format. To work with MongoDB in Python, you will need the `pymongo` library.
- Install PyMongo:
“`bash
pip install pymongo
“`
- Connect to MongoDB:
“`python
from pymongo import MongoClient
Connect to the MongoDB server
client = MongoClient(‘localhost’, 27017)
db = client[‘my_database’]
collection = db[‘users’]
“`
- Insert Data:
“`python
user_data = {
‘name’: ‘Bob’,
‘age’: 25
}
collection.insert_one(user_data)
“`
- Querying Data:
“`python
for user in collection.find():
print(user)
“`
- Updating Data:
“`python
collection.update_one({‘name’: ‘Bob’}, {‘$set’: {‘age’: 26}})
“`
- Close the Connection:
“`python
client.close()
“`
Choosing the Right Library
When working with databases in Python, several libraries can facilitate database operations. Here’s a comparison of popular libraries for different database types:
Database Type | Library | Features |
---|---|---|
SQLite | sqlite3 | Built-in, lightweight |
MySQL | mysql-connector-python | Supports various data types |
PostgreSQL | psycopg2 | Robust support for advanced SQL |
MongoDB | pymongo | Flexible schema, document-oriented |
Consider your project’s requirements, such as scalability, data structure, and complexity when choosing the appropriate database and library.
Expert Insights on Creating a Database in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “When creating a database in Python, it is essential to choose the right database management system (DBMS) that aligns with your project requirements. Libraries such as SQLite, SQLAlchemy, and Django ORM provide robust frameworks for database interaction, making it easier to manage data effectively.”
Michael Thompson (Software Engineer, CodeCraft Solutions). “Utilizing Python’s built-in libraries like sqlite3 allows developers to create lightweight databases quickly. It is crucial to understand the structure of your data and define your tables and relationships properly to ensure efficient data retrieval and manipulation.”
Sarah Kim (Database Administrator, DataSecure Corp.). “Security should always be a priority when creating a database in Python. Implementing proper authentication and access controls, along with regular backups, can safeguard your data against potential threats and ensure data integrity.”
Frequently Asked Questions (FAQs)
How do I start creating a database in Python?
To start creating a database in Python, you need to choose a database management system (DBMS) such as SQLite, MySQL, or PostgreSQL. Install the appropriate Python library, such as `sqlite3` for SQLite, and then establish a connection to the database.
What libraries are commonly used for database creation in Python?
Common libraries for database creation in Python include `sqlite3` for SQLite databases, `SQLAlchemy` for ORM capabilities, `pymysql` for MySQL, and `psycopg2` for PostgreSQL. Each library provides different functionalities suited for specific DBMS.
Can I create a database without using an external library?
Creating a database without an external library is possible only with SQLite, as it is built into Python’s standard library. Other databases require external libraries to facilitate connections and operations.
What is the process to create a table in a Python database?
To create a table in a Python database, you first establish a connection to the database. Then, use a cursor object to execute a SQL `CREATE TABLE` statement, specifying the table name and columns with their data types.
How do I insert data into a database using Python?
To insert data into a database using Python, you must create an SQL `INSERT INTO` statement. Use a cursor object to execute this statement, passing the necessary values as parameters to ensure data integrity and prevent SQL injection.
Is it possible to connect to a remote database using Python?
Yes, it is possible to connect to a remote database using Python. Ensure that the database server allows remote connections, and use the appropriate library to specify the server’s IP address, port, and authentication credentials in the connection string.
Creating a database in Python involves several steps that can vary based on the type of database you choose to use, such as SQLite, MySQL, or PostgreSQL. Python’s built-in libraries and third-party modules facilitate the interaction with these databases. For instance, SQLite is often favored for its simplicity and ease of use, making it ideal for small to medium-sized applications. To create a database, one typically establishes a connection to the database server, executes SQL commands to create tables, and defines the structure of the data to be stored.
Furthermore, utilizing Object-Relational Mapping (ORM) tools like SQLAlchemy can streamline the process of database management in Python. ORMs allow developers to interact with the database using Python objects instead of writing raw SQL queries. This abstraction not only enhances productivity but also improves code readability and maintainability. Additionally, understanding how to handle exceptions and manage transactions is crucial for ensuring data integrity and reliability in applications.
In summary, creating a database in Python is a straightforward process that can be accomplished using various libraries and frameworks. By leveraging tools like SQLite for simple projects or ORMs for more complex applications, developers can efficiently manage data storage and retrieval. As you embark on your database creation journey, it is essential
Author Profile
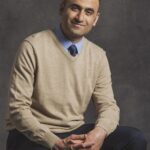
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?