How Can You Use SQL LIKE Wildcards in VBA for Access?
When working with databases in Microsoft Access, the ability to perform dynamic searches is crucial for efficient data management and analysis. One of the most powerful tools at your disposal is the SQL LIKE operator, which allows you to filter records based on partial matches. However, when integrating SQL queries into Visual Basic for Applications (VBA), many users find themselves puzzled about how to effectively implement wildcards to enhance their search capabilities. This article will unravel the intricacies of using SQL LIKE wildcards in VBA, providing you with the knowledge to streamline your database queries and unlock the full potential of your data.
In the realm of Access SQL, the LIKE operator is a game-changer, enabling users to search for patterns within text fields. By utilizing wildcards such as the asterisk (*) and the question mark (?), you can create flexible queries that return results based on varying criteria. However, when transitioning from SQL to VBA, it’s essential to understand how to properly integrate these wildcards within your code to ensure accurate and efficient data retrieval.
As we delve deeper into this topic, we will explore the syntax and practical applications of SQL LIKE wildcards in VBA. Whether you’re a seasoned developer or a novice exploring the capabilities of Access, this guide will equip you with the tools needed to enhance your database interactions and
Understanding Wildcards in SQL
Wildcards are essential tools when working with SQL queries, particularly for pattern matching in the WHERE clause. In Microsoft Access SQL, two primary wildcards are commonly used:
- Asterisk (*): Represents zero or more characters.
- Question Mark (?): Represents a single character.
These wildcards can greatly enhance the flexibility of your SQL queries when searching for specific patterns in text fields.
Using Wildcards in VBA with SQL
When using VBA (Visual Basic for Applications) to execute SQL queries that include wildcards, it is important to ensure that the SQL syntax is properly constructed. Here is a general approach to incorporating wildcards within a SQL string in VBA:
“`vba
Dim sqlQuery As String
Dim searchTerm As String
searchTerm = “example”
sqlQuery = “SELECT * FROM YourTable WHERE YourField LIKE ‘*” & searchTerm & “*’;”
“`
In this example, the query searches for any records in `YourTable` where `YourField` contains the term “example”, regardless of what characters may precede or follow it.
Examples of Wildcard Usage
Here are several examples demonstrating how wildcards can be utilized in SQL statements within VBA:
- Finding any records that start with a specific string:
“`vba
sqlQuery = “SELECT * FROM YourTable WHERE YourField LIKE ‘” & searchTerm & “*’;”
“`
- Finding records that end with a specific string:
“`vba
sqlQuery = “SELECT * FROM YourTable WHERE YourField LIKE ‘*” & searchTerm & “‘;”
“`
- Finding records that contain a specific character at a specific position:
“`vba
sqlQuery = “SELECT * FROM YourTable WHERE YourField LIKE ‘A?B’;”
“`
This will return any records where `YourField` has an “A” followed by any single character and then a “B”.
Practical Considerations
When using wildcards in SQL queries with VBA, keep the following considerations in mind:
- Performance: Wildcard searches, especially those using leading wildcards (e.g., `*example*`), can be slower since they require a full table scan.
- SQL Injection: Be cautious about incorporating user input directly into your SQL queries to avoid SQL injection risks. Always validate and sanitize user input.
- Database Compatibility: While Access SQL supports these wildcards, other SQL databases may have different syntax or wildcard characters.
Table of Wildcard Usage
Wildcard | Description | Example Usage |
---|---|---|
* | Represents zero or more characters | LIKE ‘*abc*’ |
? | Represents a single character | LIKE ‘a?c’ |
Utilizing wildcards effectively can significantly improve your ability to search and filter data in Access databases using VBA.
Using Wildcards in SQL Queries with VBA
When working with SQL queries in Microsoft Access through VBA, wildcards can be an essential tool for performing flexible string matching. In Access SQL, the common wildcards are:
- `*` (asterisk): Represents zero or more characters.
- `?` (question mark): Represents a single character.
These wildcards can be particularly useful in `LIKE` clauses when you need to filter results based on partial matches.
Implementing Wildcards in VBA
To incorporate wildcards in your SQL queries using VBA, you typically define your SQL string with the `LIKE` operator. Here’s a basic example:
“`vba
Dim db As Database
Dim rs As Recordset
Dim sqlQuery As String
Dim searchTerm As String
searchTerm = “Smith”
sqlQuery = “SELECT * FROM Employees WHERE LastName LIKE ‘*” & searchTerm & “*'”
Set db = CurrentDb
Set rs = db.OpenRecordset(sqlQuery)
“`
In this example, `*` is used to find any `LastName` containing “Smith” anywhere in the string.
Examples of Wildcard Usage
Here are some practical examples of how to use wildcards in Access SQL queries through VBA:
- Find names starting with a specific letter:
“`vba
sqlQuery = “SELECT * FROM Employees WHERE FirstName LIKE ‘A*'”
“`
- Find names ending with a specific letter:
“`vba
sqlQuery = “SELECT * FROM Employees WHERE LastName LIKE ‘*son'”
“`
- Find names with a specific pattern:
“`vba
sqlQuery = “SELECT * FROM Employees WHERE FirstName LIKE ‘J??n'”
“`
In the last example, `J??n` will match names like “John” or “Jaan” but not “Jason”.
Dynamic Search with User Input
You can also allow user input to determine search criteria. Here’s an example of how to do this:
“`vba
Dim userInput As String
userInput = InputBox(“Enter last name to search:”)
sqlQuery = “SELECT * FROM Employees WHERE LastName LIKE ‘*” & userInput & “*'”
“`
This code prompts the user for a last name and retrieves records that match the input, regardless of the position of the substring.
Considerations for Using Wildcards
When working with wildcards in SQL queries, consider the following:
- Performance: Queries using wildcards, especially leading wildcards (e.g., `*term`), can be slower because they require scanning all records.
- Data Integrity: Ensure your queries return meaningful results by validating user input to avoid unexpected results.
- Case Sensitivity: SQL Server is often case-insensitive, while Access can behave differently depending on settings. Be mindful of this when structuring queries.
Using wildcards in Access SQL queries via VBA allows for flexible and powerful searching capabilities. By effectively implementing `LIKE` with wildcards, you can enhance the functionality of your database applications.
Utilizing SQL Wildcards in VBA for Enhanced Data Queries
Dr. Emily Carter (Database Solutions Architect, Tech Innovations Inc.). “Incorporating SQL wildcards within VBA can significantly enhance your data retrieval capabilities. By using the ‘LIKE’ operator, you can efficiently filter records based on patterns, which is particularly useful for handling large datasets in Access.”
Michael Thompson (Senior VBA Developer, Data Dynamics). “When using SQL with VBA, understanding how to implement wildcards such as ‘%’ and ‘_’ is crucial. These wildcards allow for flexible querying, enabling developers to create more dynamic and responsive applications that meet user needs.”
Jessica Lin (Business Intelligence Analyst, Insight Analytics). “The integration of SQL wildcards in VBA not only streamlines data access but also improves the overall efficiency of data manipulation. By leveraging these tools effectively, analysts can derive insights faster and with greater accuracy.”
Frequently Asked Questions (FAQs)
What are the wildcard characters used in Access SQL?
Access SQL primarily uses the asterisk (*) as a wildcard for multiple characters and the question mark (?) for a single character.
How can I use the LIKE operator with wildcards in VBA?
In VBA, you can use the LIKE operator in conjunction with wildcard characters to filter records. For example, `If fieldName Like “A*” Then` checks if the field starts with “A”.
Can I use wildcards in SQL queries executed from VBA?
Yes, you can incorporate wildcards into SQL queries executed from VBA by including them in the SQL string. For instance, `SELECT * FROM TableName WHERE FieldName LIKE ‘*value*’`.
What is the difference between the LIKE operator and the = operator in Access SQL?
The LIKE operator allows for pattern matching using wildcards, while the = operator checks for an exact match without any flexibility.
Are there any limitations when using wildcards in Access SQL?
Yes, wildcards may not work as expected with certain data types, such as numeric fields. Additionally, performance may be impacted when using wildcards in large datasets.
How can I combine multiple LIKE conditions in a VBA SQL query?
You can combine multiple LIKE conditions using the AND or OR operators. For example, `WHERE Field1 LIKE ‘*value1*’ OR Field2 LIKE ‘*value2*’`.
In the context of using SQL with Microsoft Access through VBA, the LIKE operator is an essential tool for pattern matching within queries. It allows developers to search for specific string patterns in a database, making it particularly useful for filtering records based on partial matches. The use of wildcards, such as the asterisk (*) and the question mark (?), enhances the flexibility of these queries, enabling users to specify various search criteria effectively.
Key takeaways include the understanding of how to implement the LIKE operator in SQL statements within VBA. The asterisk serves as a wildcard for multiple characters, while the question mark represents a single character. This functionality allows for dynamic querying, which is crucial when dealing with user inputs or variable data. Additionally, proper syntax and careful handling of string concatenation in VBA are necessary to ensure that SQL queries execute correctly without errors.
Overall, mastering the use of the LIKE operator and wildcards in SQL queries through VBA can significantly improve data retrieval processes in Microsoft Access applications. By leveraging these features, developers can create more robust and user-friendly database applications that cater to diverse querying needs. As a result, understanding these concepts is vital for anyone looking to enhance their skills in database management and programming within the Access environment.
Author Profile
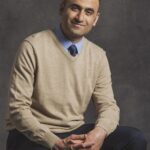
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?