How Can I Use PowerShell to Create a Directory Only If It Doesn’t Exist?
Creating directories is a fundamental task in file management, yet it can often lead to errors and inefficiencies, especially when working with scripts or automation tools. In the realm of Windows scripting, PowerShell stands out as a powerful tool that simplifies this process. One of the most common challenges users face is ensuring that a directory exists before attempting to use it. Fortunately, PowerShell provides intuitive commands that allow users to create a directory only if it does not already exist, streamlining workflows and preventing unnecessary errors.
In this article, we will explore the straightforward yet essential task of creating directories in PowerShell. Understanding how to check for the existence of a directory and create it if it is absent can save time and enhance the reliability of your scripts. We will delve into the syntax and commands that make this process seamless, ensuring that even beginners can grasp the concept without feeling overwhelmed.
By mastering the ability to create directories conditionally, you can elevate your PowerShell scripting skills and improve your overall efficiency in managing files and folders. Whether you’re automating tasks for personal projects or professional environments, this knowledge will empower you to write cleaner, more effective scripts that handle directory management with ease. Prepare to unlock the full potential of PowerShell as we guide you through this essential skill.
Creating a Directory in PowerShell
To create a directory in PowerShell, you can utilize the `New-Item` cmdlet. This cmdlet allows you to specify the type of item you want to create, including directories. However, before creating a directory, it is essential to verify whether it already exists to avoid unnecessary errors.
Checking for Directory Existence
To check if a directory exists, you can use the `Test-Path` cmdlet. This cmdlet returns a Boolean value indicating whether the specified path exists. By combining `Test-Path` with `New-Item`, you can create a directory only if it does not already exist.
Creating a Directory If It Does Not Exist
Here is a simple script to create a directory if it does not exist:
“`powershell
$directoryPath = “C:\ExampleDirectory”
if (-Not (Test-Path $directoryPath)) {
New-Item -Path $directoryPath -ItemType Directory
}
“`
In this script:
- `$directoryPath`: Specifies the path where the new directory will be created.
- `Test-Path`: Checks if the directory already exists.
- `New-Item`: Creates the directory if it does not exist.
Using a Function for Reusability
To enhance reusability, you can encapsulate the logic into a function:
“`powershell
function Create-DirectoryIfNotExists {
param (
[string]$Path
)
if (-Not (Test-Path $Path)) {
New-Item -Path $Path -ItemType Directory
Write-Host “Directory created: $Path”
} else {
Write-Host “Directory already exists: $Path”
}
}
Usage
Create-DirectoryIfNotExists -Path “C:\ExampleDirectory”
“`
This function accepts a path as a parameter and performs the same check and creation logic.
Alternative Method: Using the `mkdir` Command
PowerShell also supports the `mkdir` (or `md`) command, which is a shorthand for creating directories. However, it will not check for the existence of the directory by default. To use `mkdir` along with a check, you can do:
“`powershell
$directoryPath = “C:\ExampleDirectory”
if (-Not (Test-Path $directoryPath)) {
mkdir $directoryPath
}
“`
Best Practices
When creating directories in PowerShell, consider the following best practices:
- Use Absolute Paths: Always use absolute paths to avoid ambiguity.
- Error Handling: Implement error handling to manage exceptions that may arise during directory creation.
- Logging: Maintain logs of directory creation actions for auditing purposes.
Common Use Cases
The following table outlines common scenarios for creating directories using PowerShell:
Use Case | Description |
---|---|
Organizing Files | Create directories to organize project files or documents systematically. |
Backup Processes | Generate directories for storing backup files, ensuring that they don’t overwrite existing backups. |
Log Management | Establish directories for application logs, creating new directories as needed for different logging periods. |
Creating a Directory in PowerShell If It Does Not Exist
When working with PowerShell, ensuring that a directory exists before attempting to use it is a common requirement. This can be efficiently handled using the `Test-Path` cmdlet in combination with `New-Item`. The following sections outline how to implement this.
Using Test-Path and New-Item
To create a directory only if it does not already exist, you can use the following approach:
“`powershell
$directoryPath = “C:\Path\To\Your\Directory”
if (-not (Test-Path -Path $directoryPath)) {
New-Item -ItemType Directory -Path $directoryPath
}
“`
In this script:
- `$directoryPath` specifies the path of the directory you wish to create.
- `Test-Path` checks if the directory exists.
- `New-Item` creates the directory if it does not exist.
Alternative Method: Using the Combine Path Approach
Another method involves using `Join-Path` for constructing the directory path. This can be particularly useful when dealing with dynamic paths:
“`powershell
$basePath = “C:\Path\To”
$folderName = “NewFolder”
$fullPath = Join-Path -Path $basePath -ChildPath $folderName
if (-not (Test-Path -Path $fullPath)) {
New-Item -ItemType Directory -Path $fullPath
}
“`
This script:
- Defines a base path and a folder name.
- Combines them to create a full path.
- Checks for existence and creates the directory if necessary.
Using PowerShell’s Built-in Cmdlets
PowerShell provides built-in functionality that can simplify the process further. You can also use `mkdir` (an alias for `New-Item`) with the `-Force` parameter:
“`powershell
$directoryPath = “C:\Path\To\Your\Directory”
mkdir $directoryPath -Force
“`
The `-Force` parameter ensures that if the directory already exists, no error is thrown, effectively creating a no-fail scenario.
Checking Multiple Directories
If you need to check and create multiple directories, you can loop through an array of paths:
“`powershell
$directories = @(“C:\Path\To\FirstDirectory”, “C:\Path\To\SecondDirectory”)
foreach ($directory in $directories) {
if (-not (Test-Path -Path $directory)) {
New-Item -ItemType Directory -Path $directory
}
}
“`
This script iterates through each directory in the `$directories` array, checking for existence and creating it if it does not exist.
Best Practices
When creating directories in PowerShell, consider the following best practices:
- Always define clear and meaningful paths.
- Use `-Force` judiciously to avoid unintended overwrites.
- Handle potential errors gracefully, especially in scripts run in automated environments.
- Use comments within your scripts to clarify the purpose of each section for future reference.
By applying these methods and best practices, you can manage directory creation in PowerShell effectively and efficiently.
Expert Insights on Creating Directories in PowerShell
Jessica Lin (Senior Systems Administrator, Tech Solutions Corp). “Using PowerShell to create a directory only if it does not exist is an efficient approach for system administrators. The command ‘New-Item -ItemType Directory -Path “C:\Example\Path”‘ can be combined with ‘Test-Path’ to ensure that the directory is created only when necessary, thus optimizing script performance.”
Michael Tran (PowerShell Automation Expert, DevOps Insights). “Incorporating conditional checks in your PowerShell scripts is crucial for error prevention. I recommend using a simple if-statement: ‘if (-not (Test-Path “C:\Example\Path”)) { New-Item -ItemType Directory -Path “C:\Example\Path” }’. This method not only keeps your scripts clean but also enhances their reliability.”
Rachel Adams (IT Consultant, CloudTech Advisors). “Leveraging PowerShell for directory management is a best practice in modern IT environments. A well-structured script that checks for the existence of a directory before creation can significantly reduce the risk of conflicts and improve overall system organization. Always ensure to log actions for better tracking.”
Frequently Asked Questions (FAQs)
How can I create a directory in PowerShell if it does not exist?
To create a directory in PowerShell only if it does not exist, use the command `New-Item -Path “C:\Path\To\Directory” -ItemType Directory -Force`. The `-Force` parameter ensures that no error is thrown if the directory already exists.
What command checks if a directory exists in PowerShell?
You can check if a directory exists using the command `Test-Path “C:\Path\To\Directory”`. This command returns `True` if the directory exists and “ otherwise.
Can I create multiple directories at once in PowerShell?
Yes, you can create multiple directories at once by using a loop or by specifying multiple paths in a single command. For example, `New-Item -Path “C:\Path\To\Dir1”, “C:\Path\To\Dir2” -ItemType Directory` creates both directories if they do not exist.
Is there a one-liner to create a directory if it does not exist?
Yes, a one-liner to create a directory if it does not exist is: `if (-not (Test-Path “C:\Path\To\Directory”)) { New-Item -Path “C:\Path\To\Directory” -ItemType Directory }`. This checks for existence and creates the directory if necessary.
What happens if I use New-Item without checking if the directory exists?
If you use `New-Item` without checking for existence and the directory already exists, PowerShell will throw an error indicating that the item already exists. Using the `-Force` parameter prevents this error.
Can I create a directory with a timestamp in its name using PowerShell?
Yes, you can create a directory with a timestamp by incorporating the current date and time into the directory name. For example: `New-Item -Path “C:\Path\To\Directory_$((Get-Date).ToString(‘yyyyMMdd_HHmmss’))” -ItemType Directory`. This command creates a uniquely named directory based on the current timestamp.
In summary, creating a directory in PowerShell only if it does not already exist is a straightforward task that can be accomplished using the `New-Item` cmdlet combined with the `-Force` parameter or the `Test-Path` cmdlet. This approach not only prevents errors that may arise from attempting to create a directory that is already present but also streamlines script execution by ensuring that the desired directory structure is established without unnecessary duplication.
Utilizing the `Test-Path` cmdlet allows users to check for the existence of a directory before attempting to create it. This method enhances script efficiency and clarity, making it easier to manage file systems programmatically. Additionally, leveraging conditional statements in PowerShell can further refine the process, allowing for customized actions based on whether the directory exists or not.
Overall, the ability to create directories conditionally in PowerShell is an essential skill for system administrators and developers. It contributes to more robust scripts that can handle various file management tasks seamlessly. Understanding these techniques can significantly improve workflow automation and reduce the likelihood of errors in directory management.
Author Profile
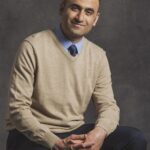
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?