How Can You Check if a Number is Prime Using Python?
### Introduction
In the world of mathematics, prime numbers hold a special place, often regarded as the building blocks of the number system. These enigmatic integers, greater than one and divisible only by themselves and one, have fascinated mathematicians and enthusiasts alike for centuries. With the rise of programming and computational mathematics, the ability to check if a number is prime has become an essential skill for developers and data scientists. Whether you’re working on cryptography, algorithms, or simply exploring the beauty of numbers, knowing how to determine the primality of a number in Python can open up a realm of possibilities.
This article delves into the various methods and techniques to check if a number is prime using Python, a versatile and widely-used programming language. From simple loops to more advanced algorithms, we will explore the different approaches you can take to efficiently determine the primality of a given integer. By understanding these methods, you will not only enhance your coding skills but also gain a deeper appreciation for the intricacies of prime numbers and their significance in various fields.
As we navigate through the landscape of prime checking in Python, you will discover practical examples and tips that will empower you to implement these techniques in your own projects. Whether you’re a beginner eager to learn or an experienced coder looking to refine your skills,
Methods to Check for Prime Numbers
To determine if a number is prime in Python, several methods can be employed. Below are some of the most common approaches:
Basic Method
The simplest way to check if a number \( n \) is prime is to check for divisibility from 2 up to \( n-1 \). If \( n \) is divisible by any of these numbers, it is not prime.
python
def is_prime_basic(n):
if n <= 1:
return
for i in range(2, n):
if n % i == 0:
return
return True
While this method is straightforward, it is inefficient for larger numbers due to its \( O(n) \) time complexity.
Optimized Method
An optimized approach involves checking for divisibility only up to the square root of \( n \). This significantly reduces the number of iterations.
python
import math
def is_prime_optimized(n):
if n <= 1:
return
for i in range(2, int(math.sqrt(n)) + 1):
if n % i == 0:
return
return True
This method operates in \( O(\sqrt{n}) \) time, making it more suitable for larger numbers.
Using the Sieve of Eratosthenes
For checking multiple numbers for primality or generating a list of prime numbers up to a certain limit, the Sieve of Eratosthenes is an efficient algorithm.
python
def sieve_of_eratosthenes(limit):
primes = [True] * (limit + 1)
p = 2
while (p * p <= limit):
if primes[p]:
for i in range(p * p, limit + 1, p):
primes[i] =
p += 1
return [p for p in range(2, limit + 1) if primes[p]]
This algorithm has a time complexity of \( O(n \log \log n) \) and is particularly effective for generating all prime numbers up to a specified limit.
Comparison of Methods
The following table summarizes the key features of the discussed methods:
Method | Time Complexity | Use Case |
---|---|---|
Basic Method | O(n) | Small numbers, simple checks |
Optimized Method | O(√n) | Larger individual checks |
Sieve of Eratosthenes | O(n log log n) | Generating primes up to a limit |
These methods provide a range of options for checking the primality of numbers, allowing for flexibility based on the context and requirements of the problem at hand.
Methods to Check for Prime Numbers in Python
To determine if a number is prime in Python, several methods can be employed. Below are some of the most effective techniques, along with sample code snippets for each.
Basic Algorithm
The simplest method to check for primality involves testing divisibility from 2 up to the square root of the number. If the number is divisible by any of these values, it is not prime.
python
def is_prime_basic(n):
if n <= 1:
return
for i in range(2, int(n**0.5) + 1):
if n % i == 0:
return
return True
Optimized Algorithm
An optimized version reduces the number of iterations by checking divisibility only by 2 and odd numbers. This method skips even numbers after checking for 2.
python
def is_prime_optimized(n):
if n <= 1:
return
if n == 2:
return True
if n % 2 == 0:
return
for i in range(3, int(n**0.5) + 1, 2):
if n % i == 0:
return
return True
Using the Sieve of Eratosthenes
For checking multiple numbers or generating a list of primes, the Sieve of Eratosthenes is efficient. This algorithm marks non-prime numbers in a boolean list.
python
def sieve_of_eratosthenes(limit):
primes = [True] * (limit + 1)
p = 2
while (p * p <= limit):
if primes[p]:
for i in range(p * p, limit + 1, p):
primes[i] =
p += 1
return [p for p in range(2, limit + 1) if primes[p]]
Using the SymPy Library
The SymPy library in Python provides a built-in function for checking prime status, which is optimized and easy to use.
python
from sympy import isprime
def check_prime_with_sympy(n):
return isprime(n)
Comparison of Methods
Method | Time Complexity | Use Case |
---|---|---|
Basic Algorithm | O(√n) | Small numbers, quick checks |
Optimized Algorithm | O(√n) | Small to medium numbers |
Sieve of Eratosthenes | O(n log log n) | Generating a list of primes |
SymPy Library | O(log n) | Quick checks with external library |
These methods provide a range of options depending on the specific needs of your application. For single checks, basic or optimized algorithms suffice, while the Sieve of Eratosthenes is ideal for generating lists of primes. Using external libraries like SymPy can simplify implementation with built-in functionality.
Expert Insights on Checking Prime Numbers in Python
Dr. Emily Carter (Computer Scientist, Python Institute). “To check if a number is prime in Python, one can implement a simple algorithm that iterates through possible divisors. This method is efficient for smaller numbers, but for larger values, consider using more advanced techniques like the Sieve of Eratosthenes or probabilistic tests.”
Michael Thompson (Software Engineer, CodeReview Magazine). “Using Python’s built-in functions can streamline the process of checking for prime numbers. A straightforward approach involves defining a function that returns for even numbers greater than 2 and checks divisibility for odd numbers up to the square root of the target number.”
Sarah Kim (Data Analyst, Tech Insights). “For those looking to optimize their prime-checking function in Python, leveraging memoization can significantly enhance performance by storing previously computed results. This is particularly useful in applications requiring frequent prime checks.”
Frequently Asked Questions (FAQs)
How can I check if a number is prime in Python?
You can check if a number is prime in Python by creating a function that tests for divisibility from 2 up to the square root of the number. If the number is divisible by any of these, it is not prime.
What is the time complexity of checking for a prime number in Python?
The time complexity of checking for a prime number using the method of trial division is O(√n), where n is the number being tested. This is efficient for small to moderately large numbers.
Is there a built-in function in Python to check for prime numbers?
Python does not have a built-in function specifically for checking prime numbers. However, you can use libraries like `sympy` which provide a function called `isprime()`.
Can I optimize the prime-checking function further?
Yes, you can optimize the function by skipping even numbers after checking for 2, thus reducing the number of iterations needed for odd numbers only.
What is the difference between a prime number and a composite number?
A prime number has exactly two distinct positive divisors: 1 and itself, while a composite number has more than two positive divisors.
Are there any libraries in Python that can help with prime number calculations?
Yes, libraries such as `sympy`, `numpy`, and `math` can assist with prime number calculations, including generating lists of primes and performing primality tests.
In Python, checking if a number is prime involves determining whether the number has any divisors other than 1 and itself. A prime number is defined as a natural number greater than 1 that cannot be formed by multiplying two smaller natural numbers. The most straightforward method to check for primality is to iterate through all integers up to the square root of the number in question, testing for divisibility. This approach significantly reduces the number of checks needed compared to testing all integers up to the number itself.
There are several efficient algorithms to check for primality, including trial division and the Sieve of Eratosthenes for generating lists of prime numbers. For individual checks, one can implement a simple function that returns True if a number is prime and otherwise. Additionally, using built-in libraries such as `sympy` can simplify the process, as they provide optimized functions for primality testing.
Key takeaways include the importance of optimizing the algorithm to reduce unnecessary computations, such as only checking for factors up to the square root of the number and skipping even numbers after checking for 2. Furthermore, understanding the mathematical properties of prime numbers can enhance one’s ability to implement more sophisticated algorithms for larger numbers. Overall, Python provides flexible
Author Profile
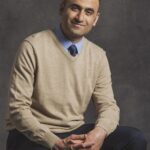
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?