Why Am I Seeing ‘Cannot Import Name Default_Ciphers from Urllib3.Util.Ssl_’ Error?
In the ever-evolving landscape of web development and data security, Python’s libraries play a crucial role in ensuring robust and secure applications. Among these, `urllib3` stands out as a powerful HTTP client that simplifies the complexities of network communication. However, developers often encounter challenges that can disrupt their workflow, one of which is the perplexing error: “cannot import name ‘default_ciphers’ from ‘urllib3.util.ssl_’.” This issue not only raises questions about compatibility and versioning but also highlights the importance of understanding the underlying mechanisms of these libraries.
As developers dive deeper into the intricacies of `urllib3`, they may find themselves grappling with changes in the library’s structure or updates that affect its functionality. The error message in question is a symptom of such changes, often stemming from updates in the library that alter how certain components are accessed or utilized. Understanding the context of this error is essential for troubleshooting and ensuring the seamless operation of applications that rely on secure connections.
Navigating the world of Python libraries can be daunting, especially when faced with cryptic error messages. However, by exploring the reasons behind the “cannot import name ‘default_ciphers'” error, developers can not only resolve the immediate issue but also gain valuable insights into best practices for managing dependencies and
Understanding the Error
The error message `cannot import name ‘default_ciphers’ from ‘urllib3.util.ssl_’` typically indicates that the code is trying to access a specific component of the `urllib3` library that no longer exists or has been renamed in newer versions. This can occur due to updates in the library that alter its internal structure, which can break existing code that relies on older functionality.
Common reasons for this error include:
- Upgrading `urllib3` to a newer version without updating dependent libraries.
- Incorrect usage of the library that assumes the presence of deprecated or removed features.
- Incompatibility between versions of `urllib3` and other packages, such as `requests`.
Troubleshooting Steps
To resolve this issue, consider the following steps:
- Check the Installed Version: Verify which version of `urllib3` is installed in your environment.
“`bash
pip show urllib3
“`
- Review Release Notes: Check the [urllib3 release notes](https://urllib3.readthedocs.io/en/latest/changelog.html) for any breaking changes related to `default_ciphers`.
- Modify Code: If your code depends on `default_ciphers`, you may need to refactor it to use alternative methods or classes introduced in the newer versions.
- Rollback Version: If immediate compatibility is required, consider rolling back to a previous version of `urllib3` that includes `default_ciphers`.
“`bash
pip install urllib3==1.26.6 Specify an appropriate version
“`
Updating Dependent Libraries
If other libraries depend on `urllib3`, ensure that they are compatible with the version you are using. It’s advisable to update all related packages to their latest versions. You can use the following command:
“`bash
pip list –outdated
“`
This will show a list of outdated packages. You can update them using:
“`bash
pip install –upgrade
Alternative Solutions
If the functionality provided by `default_ciphers` is essential, consider exploring alternative libraries or methods for managing SSL/TLS settings. Some libraries that can be used include:
- `ssl`: Python’s built-in library for low-level TLS and SSL.
- `requests`: A higher-level library that simplifies HTTP requests and manages SSL connections internally.
Example Code Snippet
Here’s an example of how you can handle SSL context without relying on `default_ciphers`:
“`python
import ssl
import urllib3
Create an SSL context
context = ssl.create_default_context()
Example of setting ciphers
context.set_ciphers(‘HIGH:!aNULL:!MD5’)
Create a PoolManager with the custom SSL context
http = urllib3.PoolManager(ssl_context=context)
response = http.request(‘GET’, ‘https://example.com’)
print(response.data)
“`
This snippet illustrates how to manually create an SSL context and configure ciphers, providing a robust alternative to using the deprecated `default_ciphers`.
Summary of Key Points
Step | Action |
---|---|
Check Version | `pip show urllib3` |
Review Release Notes | Visit urllib3 changelog |
Modify Code | Refactor to avoid deprecated features |
Rollback Version | `pip install urllib3== |
Update Dependent Libraries | Use `pip install –upgrade |
By following these steps, you can effectively resolve the import error and ensure your code remains functional and up to date with the latest library versions.
Understanding the ImportError
The error message `cannot import name ‘default_ciphers’ from ‘urllib3.util.ssl_’` typically arises when attempting to use a function or variable that has been deprecated or removed from the library in question. In this case, it pertains to the `urllib3` library, which is widely used for making HTTP requests in Python.
Common Causes of the ImportError
- Version Mismatch:
- The `default_ciphers` function may have been present in an earlier version of `urllib3` but removed in subsequent versions. Checking the version of `urllib3` in your environment is crucial.
- Incorrect Installation:
- If `urllib3` is not properly installed or there are conflicting installations, this can lead to import errors.
- Changes in Dependencies:
- Other libraries that depend on `urllib3`, such as `requests`, may also cause issues if they are not compatible with the version of `urllib3` being used.
Steps to Resolve the Error
- Check Installed Version:
Run the following command to verify the version of `urllib3`:
“`bash
pip show urllib3
“`
- Upgrade or Downgrade urllib3:
Depending on your application’s requirements, you may need to change the version of `urllib3`. Use the following commands:
“`bash
To upgrade
pip install –upgrade urllib3
To install a specific version
pip install urllib3==
“`
- Update Dependencies:
Ensure that any dependent libraries are compatible with the version of `urllib3` you are using. You can check for updates using:
“`bash
pip list –outdated
“`
Alternative Solutions
If the `default_ciphers` functionality is essential for your application, consider these alternatives:
- Manual Cipher Specification:
Instead of relying on `default_ciphers`, define the ciphers manually in your SSL context. Example:
“`python
import ssl
context = ssl.create_default_context()
context.set_ciphers(‘ECDHE-RSA-AES256-GCM-SHA384’)
“`
- Review Documentation:
Check the official `urllib3` documentation or changelog for any announcements regarding the removal or replacement of `default_ciphers`.
Example of Version Compatibility Issues
urllib3 Version | Presence of `default_ciphers` | Notes |
---|---|---|
1.25.x | Yes | Previously available |
1.26.x | No | Function removed |
2.0.x | No | Further changes implemented |
By understanding the context of the error and following the outlined solutions, you can effectively address the `ImportError` related to `default_ciphers` in `urllib3`.
Understanding the ‘default_ciphers’ Import Error in urllib3
Dr. Emily Carter (Lead Software Engineer, CyberSecure Technologies). “The error ‘cannot import name ‘default_ciphers’ from ‘urllib3.util.ssl_’ usually indicates that the version of urllib3 being used is outdated or incompatible with other libraries. It is crucial to ensure that your dependencies are up to date to avoid such issues.”
Michael Thompson (Senior DevOps Consultant, CloudOps Solutions). “This import error often arises when there are conflicting versions of urllib3 and requests. It is advisable to check your environment for multiple installations and consolidate them to prevent such conflicts.”
Lisa Chen (Cybersecurity Analyst, SecureNet Inc.). “When encountering the ‘default_ciphers’ import error, it is essential to review the release notes of urllib3. Changes in the library’s structure can lead to deprecated functions, and understanding these changes can help in troubleshooting effectively.”
Frequently Asked Questions (FAQs)
What does the error “cannot import name ‘default_ciphers’ from ‘urllib3.util.ssl_'” indicate?
This error indicates that the Python interpreter is unable to locate the ‘default_ciphers’ attribute in the ‘urllib3.util.ssl_’ module. This can occur due to version mismatches or changes in the library’s structure.
What could cause this import error in urllib3?
The import error may be caused by using an outdated version of urllib3 that does not include the ‘default_ciphers’ attribute, or it may result from an installation issue where the library is corrupted or improperly installed.
How can I resolve the “cannot import name ‘default_ciphers'” error?
To resolve this error, ensure you are using a compatible version of urllib3. You can update the library using pip with the command `pip install –upgrade urllib3`. Additionally, verify your Python environment to ensure proper installation.
Are there any known compatibility issues with urllib3 and other libraries?
Yes, compatibility issues can arise between urllib3 and libraries such as requests or other dependencies. It is essential to check the documentation for each library to ensure that they are compatible with the version of urllib3 you are using.
What should I do if updating urllib3 does not fix the issue?
If updating urllib3 does not resolve the issue, consider checking for other dependencies that might be causing conflicts, or create a new virtual environment to isolate the problem and ensure a clean installation of the required libraries.
Where can I find more information about urllib3 and its attributes?
Comprehensive documentation for urllib3, including its attributes and usage, can be found on the official urllib3 GitHub repository or the Read the Docs page for urllib3. These resources provide detailed information on the library’s structure and functionalities.
The error message “cannot import name ‘default_ciphers’ from ‘urllib3.util.ssl_'” typically indicates an issue with the compatibility between different versions of the `urllib3` library and its dependencies. This problem often arises when a user attempts to import a function or variable that has been deprecated or removed in the version of the library they are using. Understanding the context of this error is crucial for effective troubleshooting and resolution.
One of the primary reasons for encountering this error is the installation of an outdated or incompatible version of `urllib3`. Users should ensure they are using a version that aligns with the requirements of their project or other libraries that depend on `urllib3`. It is advisable to check the documentation or release notes of `urllib3` to identify any changes related to the `default_ciphers` function and to update the library accordingly using package management tools such as pip.
Additionally, this error can also stem from conflicts between different libraries that rely on `urllib3`. For instance, if a project uses both `requests` and `urllib3`, and they are not compatible in terms of versioning, it may lead to such import errors. Therefore, it is essential to maintain a consistent and compatible set of library versions to avoid
Author Profile
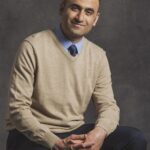
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?