How to Resolve the ‘ResizeObserver Loop Completed with Undelivered Notifications’ Error?
In the ever-evolving landscape of web development, performance and responsiveness are paramount. As applications grow in complexity, developers often turn to tools that can help manage dynamic content and layout changes. One such tool is the ResizeObserver, a powerful API that allows developers to react to changes in the size of an element. However, with great power comes great responsibility, and many developers encounter a common yet perplexing issue: the “ResizeObserver loop completed with undelivered notifications.” This warning can be a source of frustration, signaling that something is amiss in the way size changes are being handled. In this article, we will delve into the intricacies of the ResizeObserver, explore the causes of this warning, and provide insights on how to effectively manage and resolve it.
The ResizeObserver API is designed to notify developers when an observed element’s size changes, enabling responsive designs and dynamic layouts. However, the warning about undelivered notifications often arises when the callback function that processes these size changes inadvertently triggers additional size changes, creating a feedback loop. This can lead to performance issues and unexpected behavior in web applications. Understanding the mechanics behind this warning is crucial for developers aiming to create smooth and efficient user experiences.
In the following sections, we will unpack the nuances of the Resize
Understanding ResizeObserver
The `ResizeObserver` API provides a mechanism to observe changes to the dimensions of an element. This API is particularly useful for responsive designs, where elements need to adapt to changes in size dynamically. When an observed element’s size changes, the `ResizeObserver` callback is invoked, allowing developers to react to these changes accordingly.
While `ResizeObserver` is powerful, it can sometimes lead to performance issues, particularly when there are multiple resize notifications queued for processing. This can manifest as the warning: “ResizeObserver loop completed with undelivered notifications.”
Causes of the Warning
The warning occurs when the callback provided to a `ResizeObserver` results in further changes to the observed element’s size, creating a feedback loop. This means the observer keeps firing notifications for changes that are not being processed, leading to a scenario where the loop cannot complete effectively. The primary causes include:
- Direct size changes: Modifying the size of an element within the callback function.
- DOM manipulations: Adding or removing elements that affect the size of the observed element.
- Style changes: Altering CSS properties that influence layout during callback execution.
Best Practices to Avoid the Warning
To mitigate the risk of encountering this warning, developers can adhere to the following best practices:
- Debounce the callback: Implement a debounce mechanism to limit how frequently the resize callback is executed.
- Use flags: Establish flags to prevent size modifications within the observer callback.
- Separate concerns: Move size manipulation logic outside the observer callback to prevent triggering further resize events.
Handling ResizeObserver Notifications
Proper handling of notifications can help ensure that resize events are processed without performance degradation. Here’s a table outlining effective strategies for managing notifications:
Strategy | Description |
---|---|
Throttling | Limit the number of times the callback is invoked over a specified time period. |
Batch processing | Aggregate multiple resize notifications and process them in a single execution cycle. |
Conditional execution | Only execute logic if the size change meets certain criteria, reducing unnecessary updates. |
Use requestAnimationFrame | Schedule updates within the animation frame to synchronize with the browser’s rendering cycle. |
Debugging ResizeObserver Issues
When debugging issues related to `ResizeObserver`, developers can utilize several techniques:
- Console logging: Log the dimensions of the observed elements within the callback to trace resize events.
- Performance profiling: Use browser developer tools to monitor performance and identify bottlenecks associated with resize notifications.
- Isolate the observer: Temporarily disable the observer to determine if it is the source of performance issues.
By following these guidelines, developers can effectively manage `ResizeObserver` notifications and minimize the occurrence of the warning related to undelivered notifications.
Understanding ResizeObserver
ResizeObserver is a JavaScript API that allows developers to monitor changes to the size of an element. It provides a way to react to resizing events in a more efficient manner than traditional methods. When the size of an element changes, the ResizeObserver callback is triggered, allowing developers to perform necessary adjustments to the layout or styles of the page.
Common Causes of Loop Notifications
The warning “ResizeObserver loop completed with undelivered notifications” typically arises from the following scenarios:
- Frequent Resizing Events: When resizing events are triggered continuously, especially in rapid succession, it can lead to an overflow of notifications that the observer cannot process in a single cycle.
- Mutual Observations: If the size change of one element causes the size of another observed element to change, it can create a recursive loop of notifications.
- CSS Transitions and Animations: Using CSS animations or transitions can inadvertently trigger multiple resize events, leading to loop notifications.
Best Practices to Avoid Notifications
To mitigate the issue of undelivered notifications when using ResizeObserver, consider the following best practices:
– **Debouncing Resize Events**: Implement a debouncing mechanism to limit how often the resize callback is invoked. This can be done by delaying the execution of the callback until after a specified time period has passed without another resize event.
“`javascript
let resizeTimeout;
const observer = new ResizeObserver(entries => {
clearTimeout(resizeTimeout);
resizeTimeout = setTimeout(() => {
// Handle resize event
}, 100);
});
“`
- Limiting Observed Elements: Minimize the number of elements being observed. Only observe those that are crucial for your layout adjustments.
- Batch Processing: Instead of processing each resize notification immediately, batch them and handle them collectively to reduce the number of reflows and repaints.
Debugging ResizeObserver Issues
When encountering the loop notification, employ the following debugging techniques:
Technique | Description |
---|---|
Console Logging | Add console logs in the ResizeObserver callback to track how often it is being called. |
Inspect CSS Transitions | Review CSS styles and transitions that may be causing repeated resizes. |
Analyze DOM Changes | Use tools like Chrome DevTools to observe changes in the DOM that may affect element sizes. |
Example of ResizeObserver Implementation
Here is a simple example of how to use ResizeObserver effectively:
“`javascript
const element = document.querySelector(‘.resize-me’);
const observer = new ResizeObserver(entries => {
for (let entry of entries) {
console.log(‘Size changed:’, entry.contentRect.width, entry.contentRect.height);
// Additional logic to adjust layout or styles
}
});
observer.observe(element);
“`
Ensure that the observed element has clearly defined dimensions to minimize unnecessary notifications.
Conclusion on Handling ResizeObserver Notifications
By understanding the mechanics of ResizeObserver and implementing the suggested best practices, developers can efficiently manage resize events, reduce performance bottlenecks, and prevent the “loop completed with undelivered notifications” warning.
Understanding the ResizeObserver Loop Notification Issue
Dr. Emily Carter (Web Performance Specialist, Tech Innovations Group). “The ‘ResizeObserver loop completed with undelivered notifications’ warning typically arises when the layout changes during the observation process. This can lead to an infinite loop of notifications, which can significantly impact performance. Developers should ensure that their resize handling logic is optimized and does not trigger further layout changes during the observation phase.”
Michael Chen (Front-End Developer, CodeCraft Solutions). “This warning indicates that the ResizeObserver is attempting to notify changes that were not processed due to ongoing layout updates. To mitigate this issue, it is advisable to throttle the resize events or use a debouncing technique to minimize the frequency of updates, thus preventing the loop from occurring.”
Sarah Thompson (UX/UI Engineer, Digital Design Agency). “When encountering the ‘ResizeObserver loop completed with undelivered notifications’ message, it is crucial to analyze the interaction between your CSS and JavaScript. Often, excessive DOM manipulation or CSS transitions can exacerbate this issue. Implementing a more efficient rendering strategy can help in reducing unnecessary notifications and improve overall user experience.”
Frequently Asked Questions (FAQs)
What does the error “ResizeObserver loop completed with undelivered notifications” mean?
This error indicates that the ResizeObserver has detected changes in the size of an observed element, but the notifications about these changes could not be delivered due to a loop in the resize observation process. This typically occurs when the resize event triggers further changes that cause additional resize notifications.
What causes the ResizeObserver loop error?
The error is often caused by a situation where the resize event leads to modifications in the DOM that subsequently trigger another resize event. This can create a feedback loop that the ResizeObserver cannot resolve, resulting in undelivered notifications.
How can I fix the ResizeObserver loop error?
To resolve this issue, ensure that your resize handling logic does not cause further changes to the observed elements that would trigger additional resize events. You can also debounce or throttle your resize event handling to minimize the frequency of updates and prevent loops.
Is the ResizeObserver loop error a critical issue?
While the error does not typically prevent your application from functioning, it can lead to performance issues and unexpected behavior. It is advisable to address the underlying cause to ensure optimal performance and user experience.
Can I safely ignore the ResizeObserver loop error?
Ignoring the error is not recommended, as it may indicate underlying issues in your layout or event handling logic. Addressing the error can improve performance and prevent potential bugs in your application.
Are there any tools to help debug ResizeObserver issues?
Yes, you can use browser developer tools to inspect the elements being observed and monitor the resize events. Additionally, logging the resize events and their handlers can help identify the source of the loop and facilitate troubleshooting.
The issue of “ResizeObserver loop completed with undelivered notifications” typically arises in web development when using the ResizeObserver API. This warning indicates that the observer has detected changes in the size of an element, but the subsequent notifications about these changes could not be delivered due to a loop in the resize events. Such loops can occur when the resize event triggers further changes in the observed elements, creating a cycle that the browser cannot resolve efficiently.
Understanding this warning is crucial for developers as it highlights potential performance bottlenecks in applications. When the ResizeObserver detects changes, it attempts to notify all observers of those changes. However, if the handling of these notifications leads to further size changes, it can create an infinite loop, causing the browser to issue this warning. This can degrade the user experience by leading to unnecessary reflows and repaints.
To mitigate this issue, developers should ensure that any size changes triggered by the ResizeObserver are managed judiciously. This can involve debouncing resize events, using flags to prevent recursive calls, or optimizing the logic within the observer callback to avoid unnecessary modifications. By addressing these concerns, developers can enhance the performance and responsiveness of their web applications, ultimately leading to a smoother user experience.
Author Profile
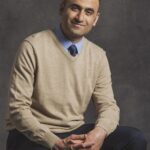
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?