How Can You Easily Transpose a Matrix in Python?
Transposing a matrix is a fundamental operation in mathematics and computer science, often essential for data manipulation, linear algebra, and machine learning applications. Whether you’re a student grappling with your first linear algebra course or a seasoned programmer looking to streamline your data processing tasks, understanding how to transpose a matrix in Python can significantly enhance your coding toolkit. With its rich ecosystem of libraries and straightforward syntax, Python offers a variety of methods to achieve this operation efficiently, making it accessible for both beginners and experts alike.
At its core, transposing a matrix involves flipping it over its diagonal, transforming rows into columns and vice versa. This simple yet powerful operation can be performed using built-in functions, as well as through popular libraries like NumPy and pandas, each offering unique advantages depending on your specific needs. For instance, while NumPy provides optimized performance for large datasets, pandas excels in handling data frames, making it easier to work with labeled data.
As we delve deeper into the various techniques available for transposing matrices in Python, we will explore practical examples and best practices that will empower you to manipulate matrices with confidence. From understanding the underlying concepts to implementing them in real-world scenarios, this guide will equip you with the knowledge you need to transpose matrices effectively and efficiently in your Python projects.
Using Nested Loops for Transposition
Transposing a matrix using nested loops involves iterating through the rows and columns of the original matrix and placing the elements in their new positions in a resulting matrix. This method is straightforward and provides a clear understanding of how transposition works.
Here is an example code snippet that demonstrates this method:
python
def transpose_matrix(matrix):
rows = len(matrix)
cols = len(matrix[0])
transposed = [[0] * rows for _ in range(cols)]
for i in range(rows):
for j in range(cols):
transposed[j][i] = matrix[i][j]
return transposed
In this code:
- A new matrix, `transposed`, is created with dimensions swapped.
- Two nested loops iterate over each element, placing them in their new transposed positions.
Using NumPy for Efficient Transposition
NumPy is a powerful library for numerical computations in Python that simplifies the process of matrix manipulation, including transposition. Using NumPy, the transposition of a matrix can be done in a single line of code.
Here’s how you can transpose a matrix using NumPy:
python
import numpy as np
matrix = np.array([[1, 2, 3], [4, 5, 6]])
transposed = matrix.T
In this code:
- The `.T` attribute of a NumPy array directly provides the transposed matrix.
- This approach is not only concise but also optimized for performance, especially with large datasets.
Transposing with List Comprehensions
List comprehensions offer a Pythonic way to transpose a matrix in a single expression. This method is both elegant and efficient, leveraging the power of Python’s list syntax.
Example:
python
def transpose_with_comprehension(matrix):
return [[row[i] for row in matrix] for i in range(len(matrix[0]))]
In this implementation:
- A list comprehension is used to iterate over the columns of the original matrix and create rows for the transposed matrix.
- This method is concise and often preferred for its readability.
Comparison of Different Methods
The choice of method for transposing a matrix can depend on factors such as readability, performance, and the size of the matrix. The following table summarizes the advantages and disadvantages of each method discussed:
Method | Advantages | Disadvantages |
---|---|---|
Nested Loops | Simple and intuitive | Less efficient for large matrices |
NumPy | Highly optimized for performance | Requires NumPy installation |
List Comprehensions | Concise and readable | Can be less intuitive for beginners |
Each method has its use case, and understanding these can help you choose the right approach for your specific needs.
Using NumPy for Matrix Transposition
NumPy is a powerful library in Python that provides support for large, multi-dimensional arrays and matrices. Transposing a matrix using NumPy is straightforward and efficient.
To transpose a matrix with NumPy, follow these steps:
- Import NumPy: Ensure the NumPy library is imported.
- Create a Matrix: Define the matrix you wish to transpose.
- Transpose the Matrix: Use the `.T` attribute or the `numpy.transpose()` function.
Here is a code example demonstrating these steps:
python
import numpy as np
# Create a 2D array (matrix)
matrix = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
# Transpose the matrix using the .T attribute
transposed_matrix = matrix.T
# Alternatively, use numpy.transpose()
transposed_matrix_alternative = np.transpose(matrix)
print(transposed_matrix)
The output will be:
[[1 4 7]
[2 5 8]
[3 6 9]]
Using List Comprehension
If you prefer not to use an external library, you can transpose a matrix with pure Python using list comprehension. This method is suitable for smaller matrices.
Here is how to do it:
- Define the Matrix: Use a list of lists to represent your matrix.
- Transpose Using List Comprehension: Generate the transposed matrix by iterating over the indices.
Example code:
python
# Define a matrix as a list of lists
matrix = [[1, 2, 3],
[4, 5, 6],
[7, 8, 9]]
# Transpose the matrix using list comprehension
transposed_matrix = [[matrix[j][i] for j in range(len(matrix))] for i in range(len(matrix[0]))]
print(transposed_matrix)
This will output:
[[1, 4, 7],
[2, 5, 8],
[3, 6, 9]]
Using the zip Function
Another Pythonic way to transpose a matrix is by utilizing the built-in `zip()` function. This method is elegant and effective for transposing matrices.
To transpose using `zip()`:
- Prepare the Matrix: Define your matrix as a list of lists.
- Use zip() with Unpacking: Call `zip()` with the unpacked matrix.
Example:
python
# Define the matrix
matrix = [[1, 2, 3],
[4, 5, 6],
[7, 8, 9]]
# Transpose using zip
transposed_matrix = list(zip(*matrix))
print(transposed_matrix)
The output will be:
[(1, 4, 7),
(2, 5, 8),
(3, 6, 9)]
Note that the output from `zip()` is a list of tuples. If you require a list of lists, you can wrap the output in `list()` as shown.
Performance Considerations
When choosing a method for transposing matrices in Python, consider the following factors:
- Matrix Size: For large matrices, using NumPy is more efficient due to optimized performance.
- Dependencies: If minimizing dependencies is a priority, use list comprehension or `zip()`.
- Readability: Choose a method that aligns with your coding style and enhances code readability.
Method | Performance | Dependencies | Code Readability |
---|---|---|---|
NumPy | Fast | Requires NumPy | High |
List Comprehension | Moderate | None | High |
zip() Function | Moderate | None | Very High |
Expert Insights on Transposing Matrices in Python
Dr. Emily Chen (Data Scientist, Tech Innovations Inc.). “Transposing a matrix in Python can be efficiently accomplished using the NumPy library, which provides a straightforward method to manipulate arrays. The `numpy.transpose()` function is particularly useful for this purpose, allowing for both 2D and higher-dimensional arrays to be transposed seamlessly.”
Professor James Patel (Computer Science Professor, University of Technology). “Understanding how to transpose a matrix is fundamental in linear algebra and data manipulation. In Python, aside from NumPy, one can also utilize list comprehensions for smaller matrices, which offers a clear and readable approach for educational purposes.”
Linda Gomez (Software Engineer, Data Solutions Corp.). “When working with large datasets, performance is key. Using NumPy not only simplifies the syntax for transposing matrices but also optimizes performance due to its underlying implementation in C, making it a preferred choice for data-intensive applications.”
Frequently Asked Questions (FAQs)
How do you transpose a matrix in Python using NumPy?
You can transpose a matrix in Python using the NumPy library by utilizing the `.T` attribute or the `numpy.transpose()` function. For example:
python
import numpy as np
matrix = np.array([[1, 2], [3, 4]])
transposed_matrix = matrix.T
# or
transposed_matrix = np.transpose(matrix)
Can you transpose a matrix without using libraries in Python?
Yes, you can transpose a matrix without libraries by using list comprehensions. For example:
python
matrix = [[1, 2], [3, 4]]
transposed_matrix = [[row[i] for row in matrix] for i in range(len(matrix[0]))]
What is the time complexity of transposing a matrix in Python?
The time complexity of transposing a matrix is O(n*m), where n is the number of rows and m is the number of columns in the matrix. This is because each element must be accessed and rearranged.
Is there a difference between in-place transposition and creating a new matrix?
Yes, in-place transposition modifies the original matrix, while creating a new matrix allocates additional memory for the transposed version. In-place transposition is not always feasible, especially for non-square matrices.
Can you transpose a matrix of different data types in Python?
Yes, Python allows you to transpose matrices containing different data types, especially when using libraries like NumPy, which can handle heterogeneous data types within an array.
What are some common applications of matrix transposition in data science?
Matrix transposition is commonly used in data science for operations such as data reshaping, preparing datasets for machine learning algorithms, and performing matrix multiplications where dimensions need to align correctly.
Transposing a matrix in Python can be accomplished using various methods, each suited to different use cases and preferences. The most common approaches include using nested loops, list comprehensions, and built-in libraries such as NumPy. Each method has its advantages, with NumPy providing the most efficient and straightforward solution for larger datasets due to its optimized performance and ease of use.
For those who prefer a more manual approach, nested loops and list comprehensions offer a clear understanding of the transposition process. However, these methods may not be as efficient as using NumPy, especially when dealing with large matrices. The choice of method often depends on the specific requirements of the task at hand, including performance considerations and the complexity of the matrix structure.
In summary, understanding how to transpose a matrix in Python is essential for data manipulation and mathematical computations. Utilizing libraries like NumPy can significantly enhance productivity and efficiency, while basic Python techniques provide foundational knowledge. Ultimately, selecting the appropriate method will depend on the context and performance needs of the application.
Author Profile
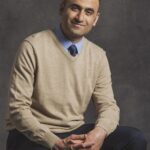
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?