How Can You Undo Actions in Python? A Comprehensive Guide
In the dynamic world of programming, mistakes are an inevitable part of the journey. Whether you’re a seasoned developer or a novice coder, encountering errors and needing to revert changes is a common experience. In Python, the ability to “undo” actions or changes can significantly enhance your coding efficiency and help maintain the integrity of your projects. But how exactly can you implement undo functionality in your Python applications? This article will explore various strategies and tools that can help you manage changes effectively, ensuring that you can navigate through your coding challenges with confidence.
When working with Python, the concept of undoing actions often revolves around the management of state and data. Unlike traditional text editors that have built-in undo features, programming requires a more deliberate approach to track changes and revert them when necessary. This can involve using data structures that maintain a history of actions or implementing specific libraries designed for this purpose. Understanding these mechanisms is crucial for developing robust applications that can gracefully handle user input and errors.
Moreover, the ability to undo actions extends beyond just correcting mistakes; it can also enhance user experience in applications by allowing users to experiment without fear of making irreversible changes. By leveraging Python’s capabilities, you can create intuitive interfaces that support undo functionality, making your applications more user-friendly and resilient. As we delve
Using Undo Functionality in Python
In Python, the concept of “undo” can be implemented in various ways, depending on the context and the application. Unlike some programming languages that have built-in support for undo operations, Python requires a more manual approach. The following techniques are commonly used to facilitate undo functionality:
Implementing Undo with Stacks
One of the most straightforward methods to implement an undo feature is by utilizing a stack data structure. A stack operates on a Last In, First Out (LIFO) principle, making it ideal for undo operations where the most recent action must be reverted first.
To implement this, you can maintain two stacks: one for actions that have been performed and another for actions that have been undone. Here’s a simple implementation:
“`python
class UndoManager:
def __init__(self):
self.actions = []
self.redo_stack = []
def perform_action(self, action):
self.actions.append(action)
self.redo_stack.clear() Clear redo stack on new action
def undo(self):
if self.actions:
action = self.actions.pop()
self.redo_stack.append(action)
return action
return None
def redo(self):
if self.redo_stack:
action = self.redo_stack.pop()
self.actions.append(action)
return action
return None
“`
This `UndoManager` class allows you to perform actions, undo them, and redo previously undone actions.
Using a Command Pattern
Another robust approach to implement undo functionality is through the Command design pattern. This pattern encapsulates a request as an object, allowing for parameterization of clients with queues, requests, and operations. This is particularly useful in applications with complex operations.
- Define a Command Interface: Create an interface for commands that includes an `execute` and an `undo` method.
- Concrete Command Classes: Implement concrete classes for each command that implement the command interface.
- Invoker: The invoker class will maintain a history of commands executed and will handle undo operations.
Here’s a brief example:
“`python
class Command:
def execute(self):
pass
def undo(self):
pass
class ConcreteCommand(Command):
def __init__(self, receiver):
self.receiver = receiver
def execute(self):
self.receiver.action()
def undo(self):
self.receiver.undo_action()
class Invoker:
def __init__(self):
self.history = []
def invoke(self, command):
command.execute()
self.history.append(command)
def undo(self):
if self.history:
command = self.history.pop()
command.undo()
“`
This setup allows for flexible command management and easy integration of undo functionality.
Example Use Cases
The following table summarizes different use cases for implementing undo functionality in Python applications:
Use Case | Preferred Method | Details |
---|---|---|
Text Editor | Stack | Store text changes to allow reverting to previous versions. |
Game Actions | Command Pattern | Encapsulate game actions and their reversals. |
Data Manipulation | Stack or Command Pattern | Maintain history of data changes for undoing. |
By choosing the appropriate method for your specific use case, you can effectively implement undo functionality in your Python applications, enhancing the user experience and maintaining data integrity.
Understanding Undo Functionality in Python
In Python, there is no built-in “undo” function akin to those found in text editors or graphical user interfaces. However, developers can implement undo functionality through various methods, depending on the context of their application. Below are some common techniques to achieve this.
Implementing Undo with a Stack
One effective way to implement undo functionality is by using a stack data structure. This approach involves maintaining a history of actions performed, allowing users to revert to previous states.
- Action Stack: Store each action as it occurs.
- Undo Action: Pop the most recent action from the stack and reverse it.
Example implementation:
“`python
class UndoManager:
def __init__(self):
self.actions = []
def perform_action(self, action):
self.actions.append(action)
Execute the action here
def undo(self):
if self.actions:
last_action = self.actions.pop()
Reverse the last action here
“`
Utilizing Command Pattern
The Command Pattern is another design pattern well-suited for undo functionality. Each action is encapsulated as an object, making it easy to execute and revert actions.
- Command Interface: Define a common interface for all commands.
- Concrete Commands: Implement specific actions that can be executed or undone.
- Invoker: Maintain a history of commands and facilitate undoing.
Example structure:
“`python
class Command:
def execute(self):
pass
def undo(self):
pass
class ConcreteCommand(Command):
def execute(self):
Perform specific action
pass
def undo(self):
Reverse specific action
pass
class CommandManager:
def __init__(self):
self.history = []
def execute_command(self, command):
command.execute()
self.history.append(command)
def undo(self):
if self.history:
command = self.history.pop()
command.undo()
“`
Using Lists for Action History
In simpler applications, a list can be used to keep track of actions. This approach is straightforward and suitable for scenarios with limited actions.
- History List: Append each action to a list.
- Reverting Actions: Iterate through the list in reverse to undo actions.
Example code snippet:
“`python
history = []
def perform_action(action):
history.append(action)
Execute action
def undo():
while history:
last_action = history.pop()
Reverse last action
“`
Considerations and Best Practices
When implementing undo functionality, consider the following best practices:
- State Management: Be mindful of how state is managed and the implications of undoing actions.
- Memory Usage: Limit the history size to prevent excessive memory usage.
- Complex Actions: For compound actions, ensure that all parts can be undone in a consistent manner.
Consideration | Description |
---|---|
State Management | Ensure consistent state across different undo levels. |
Memory Usage | Implement a maximum history length or use a circular buffer. |
Complex Actions | Handle multi-step actions carefully to maintain integrity. |
Implementing undo functionality in Python requires a structured approach, often utilizing stacks, command patterns, or simple lists. By following best practices and considering state management, developers can create robust applications that allow users to reverse actions effectively.
Expert Insights on Undoing Actions in Python
Dr. Emily Carter (Senior Software Engineer, Python Innovations Inc.). “In Python, the concept of ‘undo’ is not inherently built into the language’s core functionality. However, developers can implement undo features by maintaining a history stack that records changes. This allows for reverting to previous states effectively.”
Michael Chen (Lead Developer, CodeCraft Solutions). “To achieve an undo mechanism in Python, one can utilize the Command Pattern. By encapsulating actions as objects, it becomes straightforward to track and revert actions as needed. This approach is particularly useful in applications with complex user interactions.”
Sarah Thompson (Data Scientist, AI Research Labs). “When working with data manipulation in Python, implementing an undo feature can be crucial for data integrity. Utilizing libraries such as `pandas` along with version control techniques, such as saving snapshots of data frames, can provide a reliable method for undoing changes.”
Frequently Asked Questions (FAQs)
How can I implement an undo feature in a Python application?
To implement an undo feature, you can use a stack data structure to keep track of actions performed. Each action is pushed onto the stack, and when an undo operation is requested, the last action is popped from the stack and reversed.
Is there a built-in undo function in Python?
Python does not have a built-in undo function. However, you can create your own undo mechanism by managing the state of your application and storing previous states or actions.
What libraries can assist with implementing undo functionality in Python?
Libraries such as `tkinter` for GUI applications and `pygame` for game development provide ways to manage events and states, which can be leveraged to implement undo functionality.
Can I use version control systems for undoing changes in Python projects?
Yes, version control systems like Git allow you to revert changes in your codebase. You can use commands like `git checkout` or `git revert` to undo changes in your Python projects effectively.
How do I handle multiple levels of undo in Python?
To handle multiple levels of undo, maintain a stack for actions and a separate stack for undone actions. When an action is undone, push it onto the undone stack, allowing for redoing actions as needed.
What are some common use cases for undo functionality in Python applications?
Common use cases include text editors, graphic design software, and any application where user actions need to be reversible, enhancing user experience and error recovery.
In Python, the concept of “undo” is not natively supported as it is in some other programming languages or applications. However, developers can implement undo functionality through various methods, depending on the specific requirements of their application. Common approaches include using data structures like stacks to keep track of changes, creating a history log of actions, or utilizing design patterns such as Command or Memento to encapsulate state changes and facilitate rollback capabilities.
One of the most effective strategies for implementing undo functionality is to maintain a history of actions performed by the user. By storing snapshots of the application’s state or the commands executed, developers can easily revert to a previous state when needed. This approach not only enhances user experience but also allows for greater flexibility in managing application state changes.
Another important takeaway is the significance of careful planning and design when incorporating undo functionality. It is crucial to determine the scope of what should be undoable, manage memory effectively, and ensure that the implementation does not introduce significant performance overhead. By considering these factors, developers can create robust undo mechanisms that improve usability without compromising application performance.
Author Profile
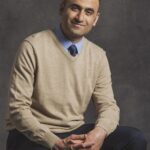
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?