How Can You Use Sed to Replace a String in a File?
In the world of text processing and command-line utilities, few tools are as powerful and versatile as `sed`. This stream editor, often referred to as the “sed” command, is a staple for anyone who regularly works with text files in Unix-like operating systems. Whether you’re a seasoned programmer or a casual user looking to automate mundane tasks, understanding how to replace strings in files with `sed` can significantly enhance your productivity. Imagine being able to modify large files with just a single command, saving you hours of manual editing.
At its core, `sed` is designed for parsing and transforming text, making it an invaluable asset for tasks ranging from simple string replacements to complex text manipulations. The ability to replace strings not only streamlines your workflow but also minimizes the risk of human error that often accompanies manual edits. With its straightforward syntax, `sed` allows users to perform batch updates, ensuring consistency across multiple files or within a single document.
As we delve deeper into the intricacies of using `sed` for string replacement, we will explore its syntax, various options, and practical examples that illustrate its capabilities. Whether you’re looking to replace a single occurrence or multiple instances of a string, mastering `sed` can empower you to tackle text processing tasks with confidence and efficiency
Using sed for String Replacement
The `sed` command, short for Stream Editor, is a powerful tool commonly used for parsing and transforming text in files. One of its most prevalent uses is to replace strings within files. Understanding how to effectively use `sed` for string replacements can significantly enhance your text processing capabilities in Unix-like operating systems.
To replace a string in a file using `sed`, the general syntax is as follows:
“`
sed ‘s/old_string/new_string/g’ filename
“`
In this command:
- `s` denotes the substitute command.
- `old_string` is the text you want to replace.
- `new_string` is the replacement text.
- `g` is a flag that indicates global replacement, meaning all occurrences in the line will be replaced.
For example, to replace “apple” with “orange” in a file named `fruits.txt`, you would execute:
“`
sed ‘s/apple/orange/g’ fruits.txt
“`
In-Place Editing with sed
When you want to apply the changes directly to the file without creating a new output file, you can use the `-i` option. This allows you to edit the file in place. The syntax is:
“`
sed -i ‘s/old_string/new_string/g’ filename
“`
Using the previous example, to replace “apple” with “orange” directly in `fruits.txt`, you would run:
“`
sed -i ‘s/apple/orange/g’ fruits.txt
“`
It’s important to note that the `-i` option can create a backup of the original file. To do this, specify a suffix for the backup file:
“`
sed -i.bak ‘s/apple/orange/g’ fruits.txt
“`
This command will create a backup of `fruits.txt` named `fruits.txt.bak`.
Handling Special Characters
When dealing with strings that contain special characters, such as slashes (`/`), it is advisable to choose an alternative delimiter. For instance, you can use the pipe symbol (`|`) instead:
“`
sed ‘s|/path/to/old|/path/to/new|g’ filename
“`
This approach avoids confusion with the standard delimiter.
Examples of sed String Replacement
Here are several practical examples illustrating the use of `sed` for various string replacement tasks:
Command | Description |
---|---|
sed ‘s/hello/world/g’ greetings.txt | Replace all occurrences of “hello” with “world” in greetings.txt. |
sed -i ‘s/foo/bar/g’ example.txt | Replace “foo” with “bar” in example.txt, editing the file in place. |
sed ‘s|http://|https://|g’ links.txt | Change all “http://” links to “https://” in links.txt. |
By mastering these commands, users can efficiently manipulate text files, ensuring that their data processing tasks are both effective and streamlined.
Using `sed` for String Replacement in Files
The `sed` (stream editor) command is a powerful utility for parsing and transforming text in Unix-like operating systems. It excels in performing basic text transformations on an input stream (file or input from a pipeline). Below are the methods to replace strings in a file using `sed`.
Basic Syntax of `sed`
The general syntax for replacing a string in a file with `sed` is as follows:
“`bash
sed ‘s/pattern/replacement/’ file.txt
“`
- `s` indicates the substitution command.
- `pattern` is the string you want to replace.
- `replacement` is the new string that will replace the `pattern`.
- `file.txt` is the name of the file to be processed.
Common Options
When using `sed` for string replacement, several options can enhance functionality:
- `-i`: Edits the file in place, modifying the original file.
- `-e`: Allows multiple expressions to be executed.
- `-n`: Suppresses automatic printing of pattern space, useful when combined with `p` for selective output.
Examples of String Replacement
Here are a few practical examples demonstrating how to use `sed` for string replacement.
Simple Replacement
To replace the first occurrence of “foo” with “bar” in `file.txt`:
“`bash
sed ‘s/foo/bar/’ file.txt
“`
Global Replacement
To replace all occurrences of “foo” with “bar”:
“`bash
sed ‘s/foo/bar/g’ file.txt
“`
- The `g` flag indicates a global replacement within each line.
In-Place Replacement
To replace “foo” with “bar” directly in the file without creating a backup:
“`bash
sed -i ‘s/foo/bar/g’ file.txt
“`
To create a backup with a specific extension (e.g., `.bak`):
“`bash
sed -i.bak ‘s/foo/bar/g’ file.txt
“`
Case-Insensitive Replacement
For a case-insensitive replacement, use the `I` flag:
“`bash
sed ‘s/foo/bar/gI’ file.txt
“`
Using Regular Expressions
`sed` also supports regular expressions, allowing for more complex pattern matching. For example, to replace either “foo” or “baz” with “bar”:
“`bash
sed ‘s/foo\|baz/bar/g’ file.txt
“`
Multiple Replacements in One Command
To perform multiple replacements in a single `sed` command, use the `-e` option:
“`bash
sed -e ‘s/foo/bar/g’ -e ‘s/baz/qux/g’ file.txt
“`
Replacing Strings in Multiple Files
To replace strings in multiple files simultaneously, you can use a wildcard:
“`bash
sed -i ‘s/foo/bar/g’ *.txt
“`
This command replaces “foo” with “bar” in all `.txt` files in the current directory.
Verifying Changes
To verify the changes made without modifying the file, redirect the output to another file:
“`bash
sed ‘s/foo/bar/g’ file.txt > new_file.txt
“`
This command writes the modified content to `new_file.txt`, preserving the original file.
Utilizing `sed` for string replacement is an efficient method for text manipulation in files. By mastering its syntax and options, users can effectively perform complex text transformations with minimal effort.
Expert Insights on Using `sed` for String Replacement in Files
Dr. Emily Carter (Senior Software Engineer, Open Source Solutions). “Utilizing `sed` for string replacement in files is not only efficient but also essential for automating text processing tasks. It allows developers to manipulate large datasets quickly without the overhead of loading them into a programming environment.”
Mark Thompson (DevOps Specialist, Tech Innovators Inc.). “The power of `sed` lies in its ability to execute complex patterns and substitutions in a single command. This makes it invaluable for system administrators who need to make bulk changes across configuration files with minimal risk of error.”
Linda Chen (Data Scientist, Analytics Hub). “When dealing with text data, `sed` serves as a robust tool for preprocessing. Its stream editing capabilities ensure that data cleaning can be performed efficiently, which is crucial for maintaining the integrity of data analysis workflows.”
Frequently Asked Questions (FAQs)
What is the basic syntax for using sed to replace a string in a file?
The basic syntax for using sed to replace a string in a file is: `sed -i ‘s/old_string/new_string/g’ filename`. The `-i` flag edits the file in place, `s` indicates substitution, and `g` means to replace all occurrences in each line.
Can I use sed to replace multiple strings in a single command?
Yes, you can replace multiple strings in a single command by chaining the substitutions. For example: `sed -i -e ‘s/old1/new1/g’ -e ‘s/old2/new2/g’ filename`.
How do I replace a string in a file without modifying the original file?
To replace a string without modifying the original file, omit the `-i` flag and redirect the output to a new file. For example: `sed ‘s/old_string/new_string/g’ original_file > new_file`.
What does the ‘g’ flag do in the sed replace command?
The ‘g’ flag in the sed replace command stands for “global.” It indicates that all occurrences of the specified string in each line should be replaced, rather than just the first occurrence.
Can I use regular expressions with sed for string replacement?
Yes, sed supports basic regular expressions, allowing for complex pattern matching and replacement. For example, you can use wildcards or character classes to match patterns more dynamically.
Is it possible to replace a string in a specific line using sed?
Yes, you can target a specific line by specifying the line number before the substitution command. For example: `sed ‘3s/old_string/new_string/’ filename` will replace `old_string` with `new_string` only on line 3.
In summary, the `sed` command is a powerful stream editor widely used in Unix and Linux environments for manipulating text files. Its primary function includes searching for specific patterns in a file and replacing them with designated strings. This capability is particularly useful for batch processing of text, allowing users to efficiently update multiple occurrences of a string within a single command. The basic syntax for replacing a string in a file using `sed` is straightforward, typically following the format `sed ‘s/old_string/new_string/g’ filename`, where the ‘g’ flag indicates a global replacement throughout the entire file.
One of the key takeaways is the flexibility that `sed` offers through various options and flags. Users can perform case-insensitive replacements, limit replacements to specific lines, or even use regular expressions for more complex pattern matching. Additionally, `sed` can operate directly on files or stream input from other commands, making it a versatile tool in scripting and automation tasks. Understanding these features enhances a user’s ability to effectively manage and edit text data.
Moreover, it is essential to exercise caution when using `sed`, especially with the `-i` option, which modifies files in place. It is advisable to create backups before making changes to prevent accidental
Author Profile
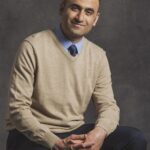
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?