Can You Use Double for Int? Exploring the Compatibility of Data Types in Programming
When it comes to programming, understanding data types is crucial for effective coding and application performance. One common question that arises among both novice and experienced programmers is, “Can you use double for int?” This inquiry delves into the nuances of type conversion, precision, and the implications of using floating-point numbers in place of integers. As we explore this topic, we will uncover the intricacies of data types and how they interact within various programming languages, ultimately guiding you toward making informed decisions in your coding practices.
At its core, the distinction between integers and doubles lies in their representation of numerical values. Integers are whole numbers without any decimal points, while doubles are capable of representing fractional values, allowing for a broader range of numerical expression. However, substituting a double for an int can lead to unexpected behaviors, particularly concerning precision and memory usage. Understanding the underlying mechanics of these data types is essential for optimizing your code and avoiding potential pitfalls.
Moreover, the implications of using doubles in place of integers extend beyond mere syntax. Depending on the programming language, this choice can affect performance, lead to increased memory consumption, and introduce subtle bugs in calculations. As we delve deeper into this topic, we will examine the scenarios where such conversions might be appropriate, the potential risks involved, and best
Understanding Data Types
When working in programming languages, understanding data types is crucial. The `int` type typically represents integer values, while `double` is used for floating-point numbers, allowing for the representation of decimal values. Using a `double` for an `int` can be done, but it comes with certain implications.
Conversion Between Types
In many programming languages, it is possible to convert a `double` to an `int`. This process is known as type casting. However, this conversion can lead to the loss of precision. Here are key points to consider:
- Precision Loss: When converting a `double` to an `int`, the decimal portion is truncated. For example, converting `5.9` to an `int` results in `5`.
- Range Limitations: The range of values that an `int` can hold is narrower than that of a `double`. Therefore, if a `double` value exceeds the range of an `int`, converting it will lead to overflow or an erroneous value.
Practical Implications
In practical applications, using `double` for `int` values can be beneficial in scenarios where precision is essential during calculations but should ultimately be converted to an `int` for final storage or output. Here are some situations to consider:
- Mathematical Calculations: Use `double` for intermediate calculations to retain precision.
- Final Output: Convert to `int` when the result needs to be an integer for display or further processing.
Example of Conversion
Consider the following example in pseudocode:
“`
double num = 10.75;
int result = (int)num; // result will be 10
“`
In this example, the `double` value `10.75` is cast to an `int`, resulting in `10`.
Comparison of Data Types
To better understand the differences between these two data types, the following table summarizes their characteristics:
Data Type | Size (in bytes) | Range | Precision |
---|---|---|---|
int | 4 | -2,147,483,648 to 2,147,483,647 | Exact |
double | 8 | Approximately ±1.7 × 10^308 | 15-17 decimal digits |
Best Practices
When deciding whether to use `double` for values that will ultimately be represented as `int`, consider the following best practices:
- Use `double` for Calculations: Retain `double` during calculations to manage precision.
- Convert When Necessary: Only convert to `int` when you are certain that the value will not exceed the `int` range or require decimal precision.
- Error Handling: Implement error checking to manage cases where conversion could lead to unexpected results.
By adhering to these guidelines, developers can effectively manage data types and avoid common pitfalls associated with type conversion.
Understanding Data Types
In programming, data types define the kind of data that can be stored and manipulated within a program. Two commonly used numeric types are `int` (integer) and `double` (floating-point).
- Int:
- Represents whole numbers (e.g., -1, 0, 1, 2).
- Typically occupies 4 bytes in memory, but this can vary based on the programming language and platform.
- Double:
- Represents floating-point numbers, allowing for decimals (e.g., 3.14, -0.001).
- Usually occupies 8 bytes in memory, providing a larger range and precision than an `int`.
Type Compatibility and Conversion
When considering whether you can use a `double` for an `int`, it is essential to understand how type compatibility works in programming languages.
- Implicit Conversion: Some languages allow automatic conversion from `int` to `double` without explicit instruction. This process is known as widening conversion.
- Explicit Conversion: Converting from `double` to `int` typically requires an explicit cast, as this is a narrowing conversion and may lead to data loss (e.g., truncating decimal values).
Conversion Type | Description | Example |
---|---|---|
Implicit (Widening) | `int` to `double` (safe, no data loss) | `double x = 5;` |
Explicit (Narrowing) | `double` to `int` (risk of data loss) | `int y = (int)3.14;` |
Examples in Different Programming Languages
The behavior of using `double` as an `int` varies by programming language. Below are examples in popular languages.
- Java:
“`java
double a = 5.5;
int b = (int) a; // Explicit conversion, b will be 5
“`
- C:
“`csharp
double x = 7.8;
int y = (int)x; // Explicit conversion, y will be 7
“`
- Python:
“`python
x = 9.9
y = int(x) Explicit conversion, y will be 9
“`
Potential Issues
When using `double` in contexts where `int` is expected, several issues may arise:
- Precision Loss: Converting `double` to `int` can lead to loss of the fractional part.
- Overflow: Using a `double` to represent large integers can cause overflow if the value exceeds the maximum range of `int`.
- Performance: Operations with `double` may be less efficient compared to `int`, particularly in performance-critical applications.
Best Practices
To ensure effective use of `double` and `int`, consider the following practices:
- Use the Appropriate Type: Choose `int` for whole numbers and `double` for numbers requiring decimals.
- Be Explicit About Conversions: Always perform explicit conversions to maintain clarity and avoid unintentional data loss.
- Validate Data: Implement checks to ensure that values falling within the acceptable range for the target data type.
By adhering to these guidelines, you can effectively manage the use of `double` and `int` in your programming endeavors.
Understanding Type Conversion: Can You Use Double for Int?
Dr. Emily Carter (Computer Science Professor, Tech University). “In programming, using a double to represent an integer can lead to precision issues. While it is technically possible to assign a double value to an int variable, the fractional part will be truncated, potentially resulting in data loss. Therefore, it is advisable to use the appropriate data type for the intended purpose to avoid unexpected behavior.”
Michael Chen (Lead Software Engineer, Code Solutions Inc.). “From a practical standpoint, utilizing a double for integer operations can complicate calculations and introduce unnecessary overhead. It is essential to maintain clarity in code by using the correct data types, which enhances readability and reduces the risk of runtime errors.”
Sarah Johnson (Data Analyst, Analytics Group). “When working with numerical data, it’s crucial to choose the right type to ensure accuracy. While you can technically assign a double to an int, doing so may not reflect the true intent of the data. Always consider the implications of type conversion on the integrity of your data and the performance of your application.”
Frequently Asked Questions (FAQs)
Can you use double for int in programming?
Yes, you can use a double to store an integer value, as a double can represent both whole numbers and decimal values. However, this may lead to precision issues for very large integers.
What happens when you assign an int to a double?
When you assign an int to a double, the int is implicitly converted to a double type, which allows for decimal representation. The value remains the same, but the data type changes.
Is it safe to cast a double to an int?
Casting a double to an int can lead to data loss, as the fractional part of the double will be truncated. It is essential to ensure that the double value is within the range of the int type to avoid overflow.
What are the performance implications of using double instead of int?
Using double instead of int can lead to increased memory usage and slower performance due to the more complex calculations involved with floating-point arithmetic. Ints are generally faster and more efficient for whole number operations.
When should you prefer int over double?
You should prefer int over double when dealing with whole numbers where precision is critical, such as in counting, indexing, or when performing arithmetic that does not require decimal values.
Can you perform arithmetic operations between int and double?
Yes, you can perform arithmetic operations between int and double. The int will be implicitly converted to double during the operation, resulting in a double output.
In programming, the distinction between data types is crucial for effective code execution and memory management. The question of whether a double can be used in place of an int often arises, particularly in languages like C, C++, and Java. A double is a floating-point data type that can represent a wider range of values, including decimals, while an int is a whole number type. While it is technically possible to assign a double value to an int variable through type casting, this process can lead to loss of precision and data truncation.
When a double is assigned to an int, the fractional part of the double is discarded, resulting in an integer that may not accurately represent the original value. This can lead to unexpected behavior in calculations and logic if not properly managed. Therefore, while a double can be used in contexts where an int is expected, developers must be cautious about the implications of such conversions and ensure that they are handling data types appropriately to avoid errors.
In summary, while it is feasible to use a double in place of an int through casting, it is essential to understand the potential consequences. Developers should consider the context of their applications and the importance of precision in their calculations. Ultimately, adhering to best practices in data type usage
Author Profile
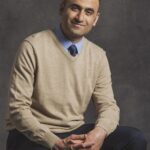
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?