How Can You Access HashiCorp Vault Values in Your TypeScript Application?
In today’s rapidly evolving digital landscape, securing sensitive information is more critical than ever. As applications become increasingly complex, developers are tasked with not only building robust functionalities but also ensuring that sensitive data, such as API keys, passwords, and certificates, are managed securely. This is where HashiCorp Vault comes into play—a powerful tool designed to store and control access to secrets in a centralized manner. For TypeScript developers, integrating Vault into their applications can elevate security practices while maintaining seamless workflows. In this article, we will explore how to effectively access HashiCorp Vault values within a TypeScript application, empowering you to enhance your app’s security posture.
Accessing secrets from HashiCorp Vault in a TypeScript application involves understanding both the Vault’s API and the TypeScript environment. By leveraging Vault’s robust features, developers can retrieve sensitive data securely and efficiently, ensuring that their applications remain compliant with security best practices. The integration process typically includes setting up the Vault client, authenticating against the Vault server, and implementing the necessary logic to fetch and utilize secrets within the application.
As we delve deeper into this topic, we will cover the essential steps and best practices for accessing Vault values in a TypeScript context. Whether you are a seasoned developer looking to enhance your security practices
Setting Up the Environment
To access HashiCorp Vault values in a TypeScript application, you first need to ensure your environment is properly set up. This involves installing necessary packages and configuring your Vault instance.
- Install Dependencies: Use npm or yarn to install the required packages for interacting with Vault.
“`bash
npm install @hashicorp/vault-client
“`
- Configure Vault: Set up your Vault server and ensure it is running. You must also create a policy that allows your application to read the secrets you want to access.
- Environment Variables: To keep your application secure, store your Vault address and token in environment variables.
“`bash
export VAULT_ADDR=’http://127.0.0.1:8200′
export VAULT_TOKEN=’your-vault-token’
“`
Accessing Secrets in TypeScript
Once your environment is set up, you can begin accessing secrets from Vault in your TypeScript application. The following steps outline how to authenticate and retrieve secrets.
- Import Required Modules: Start by importing the necessary modules in your TypeScript file.
“`typescript
import { VaultClient } from ‘@hashicorp/vault-client’;
“`
- Initialize the Vault Client: Create an instance of the Vault client using the environment variables.
“`typescript
const vault = new VaultClient({
endpoint: process.env.VAULT_ADDR,
token: process.env.VAULT_TOKEN,
});
“`
- Read Secrets: Use the Vault client to read secrets from a specified path. For example, if you have a secret stored at `secret/myapp`, you can access it as follows:
“`typescript
async function getSecret() {
try {
const secret = await vault.read(‘secret/myapp’);
console.log(secret.data);
} catch (error) {
console.error(‘Error accessing secrets:’, error);
}
}
“`
Handling Errors and Responses
When interacting with Vault, it is crucial to handle potential errors and understand the structure of the responses. This will ensure your application can gracefully manage any issues that arise during API calls.
- Error Handling: Always wrap your read calls in try-catch blocks to handle errors effectively.
- Response Structure: The response from the Vault typically contains the secret data along with metadata. Here’s a basic structure of the response:
Field | Description |
---|---|
data | The actual secret data retrieved from Vault. |
lease_duration | The duration for which the secret is valid. |
metadata | Information about the secret, such as the creation time. |
This structure allows you to navigate the response effectively and extract necessary information for your application’s needs.
Setting Up HashiCorp Vault
To access values in HashiCorp Vault from a TypeScript application, you first need to ensure that Vault is properly set up and configured. Here are the steps to follow:
- Install Vault: Download and install HashiCorp Vault from the official website.
- Initialize Vault: Run the command `vault operator init` to initialize Vault. This will generate unseal keys and a root token.
- Unseal Vault: Use the unseal keys to unseal Vault by executing `vault operator unseal
`. - Authenticate: Use the root token to authenticate: `vault login
`. - Enable Secrets Engine: Enable a secrets engine, for example, the Key/Value secrets engine: `vault secrets enable -path=secret kv`.
Installing Required Packages
In your TypeScript application, you will need a few npm packages to interact with HashiCorp Vault. Install these packages:
“`bash
npm install @hashicorp/vault-client dotenv
“`
- @hashicorp/vault-client: This package allows you to connect to and interact with HashiCorp Vault.
- dotenv: This package helps in managing environment variables.
Configuring Environment Variables
Set up a `.env` file in your project root to store your Vault configuration details:
“`
VAULT_ADDR=’http://127.0.0.1:8200′
VAULT_TOKEN=’
“`
This file should not be included in version control to keep your credentials secure.
Accessing Vault Values in TypeScript
You can now access Vault values in your TypeScript application. Below is a sample code snippet demonstrating how to retrieve secrets:
“`typescript
import * as dotenv from ‘dotenv’;
import { VaultClient } from ‘@hashicorp/vault-client’;
dotenv.config();
const vault = new VaultClient({
endpoint: process.env.VAULT_ADDR,
token: process.env.VAULT_TOKEN,
});
async function getSecret(secretPath: string) {
try {
const secret = await vault.kv.read(secretPath);
console.log(‘Secret Data:’, secret.data);
} catch (error) {
console.error(‘Error accessing Vault:’, error);
}
}
getSecret(‘secret/my-secret’);
“`
Explanation of the Code
- dotenv.config(): Loads environment variables from the `.env` file.
- VaultClient: Initializes the Vault client with the address and token.
- getSecret function: This function accepts a `secretPath` and retrieves the associated secret.
Handling Errors and Security
When dealing with sensitive data, proper error handling and security measures are essential:
- Error Handling:
- Always catch errors when accessing Vault to prevent application crashes.
- Log errors in a secure manner, avoiding exposure of sensitive information.
- Security Best Practices:
- Use short-lived tokens for applications.
- Implement proper access policies in Vault.
- Regularly rotate secrets and tokens.
By following these guidelines, you can securely access HashiCorp Vault values within your TypeScript application.
Expert Insights on Accessing HashiCorp Vault Values in TypeScript Applications
Dr. Emily Chen (Cloud Security Architect, SecureTech Solutions). “To effectively access HashiCorp Vault values in a TypeScript application, developers should utilize the official Vault API client libraries. This ensures secure communication and simplifies the handling of authentication tokens, which are essential for accessing sensitive data.”
Michael Thompson (Lead Software Engineer, DevOps Innovations). “Integrating HashiCorp Vault with a TypeScript application requires a solid understanding of asynchronous programming. Using Promises or async/await syntax will streamline the process of fetching secrets from Vault, enhancing both performance and readability of your code.”
Sarah Patel (DevSecOps Consultant, CyberGuard Technologies). “When accessing secrets from HashiCorp Vault in a TypeScript app, it is crucial to implement proper error handling and logging mechanisms. This not only aids in debugging but also ensures that sensitive information is managed securely throughout the application lifecycle.”
Frequently Asked Questions (FAQs)
How can I authenticate my TypeScript app with HashiCorp Vault?
To authenticate your TypeScript app with HashiCorp Vault, you can use methods such as AppRole, Token, or LDAP authentication. Implement the chosen method by configuring the Vault client in your app and providing the necessary credentials.
What libraries are available for accessing HashiCorp Vault in TypeScript?
You can use libraries such as `node-vault`, which is a popular client for interacting with HashiCorp Vault. This library provides a simple API to perform operations like reading and writing secrets.
How do I read a secret value from HashiCorp Vault in a TypeScript application?
To read a secret value, initialize the Vault client and call the `read` method with the path of the secret. Ensure that your application has the necessary permissions to access the secret.
What are the best practices for managing Vault secrets in a TypeScript application?
Best practices include using environment variables for sensitive configurations, implementing proper access controls, regularly rotating secrets, and utilizing Vault’s dynamic secrets feature for enhanced security.
How can I handle errors when accessing HashiCorp Vault in TypeScript?
Handle errors by implementing try-catch blocks around your Vault API calls. Log the errors appropriately and provide fallback mechanisms or user notifications to ensure application stability.
Is it possible to use HashiCorp Vault with serverless TypeScript applications?
Yes, HashiCorp Vault can be integrated with serverless TypeScript applications. Use the Vault client within your serverless function to securely access secrets and manage configurations as needed.
Accessing HashiCorp Vault values in a TypeScript application involves several key steps that ensure secure and efficient retrieval of sensitive data. First, it is essential to set up the Vault server and configure the necessary authentication methods, such as token-based or AppRole authentication. This setup allows the TypeScript application to authenticate securely with Vault and request the secrets it needs.
Once the authentication is established, developers can utilize the official HashiCorp Vault client libraries or REST API to interact with the Vault server. In TypeScript, using libraries like `node-vault` can streamline the process of reading secrets. Proper error handling and security practices, such as managing tokens and ensuring they are not hard-coded, are crucial to maintaining the integrity of the application.
Additionally, integrating environment variables to store Vault configurations can enhance security and flexibility. This approach allows the application to adapt to different environments without exposing sensitive information in the codebase. Overall, following best practices for accessing Vault values not only secures sensitive data but also simplifies the management of secrets within a TypeScript application.
Author Profile
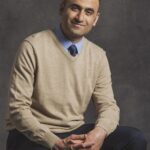
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?