Why Am I Getting a TypeError: String Indices Must Be Integers, Not Strings?
In the world of programming, encountering errors is an inevitable part of the journey. Among the myriad of issues developers face, the `TypeError: string indices must be integers, not str` stands out as a common yet perplexing hurdle, particularly for those navigating the intricacies of Python. This error serves as a reminder of the importance of understanding data types and their interactions within your code. As you delve into the nuances of this error, you’ll discover not only its causes but also the strategies to prevent it from derailing your coding endeavors.
The `TypeError` in question typically arises when a programmer attempts to access elements of a string using a string index rather than an integer. This fundamental misunderstanding can lead to frustration, especially for those new to Python or those who may be transitioning from languages with different indexing systems. Understanding the underlying principles of data types—specifically strings and integers—will empower you to write more robust and error-free code.
As we explore the intricacies of this error, we will uncover the common scenarios that lead to its occurrence, the implications of misusing string indices, and practical solutions to rectify the issue. Whether you’re debugging a small script or working on a larger project, grasping the concept behind this `TypeError` will enhance your
Understanding the Error
A `TypeError` in Python, particularly the message “string indices must be integers, not str,” typically occurs when attempting to access an element of a string using a string key rather than an integer index. Strings in Python are sequences of characters, and each character can be accessed using its position (integer index) in the string.
For example:
“`python
my_string = “hello”
print(my_string[0]) This is valid and outputs ‘h’
print(my_string[‘0’]) This will raise a TypeError
“`
The second line raises the error because it tries to access an index using a string (‘0’) instead of an integer (0).
Common Causes
Several scenarios can lead to this error:
- Accessing String Elements Incorrectly: Attempting to access a string with a non-integer value.
- Confusing Strings with Dictionaries: If a string is mistakenly treated like a dictionary, where keys are used for access.
- JSON Parsing Mistakes: When parsing JSON data, if the expected structure is not followed, strings may be accessed incorrectly.
Examples of the Error
Consider the following examples that demonstrate how this error can occur:
- Wrong Indexing:
“`python
data = “example”
print(data[‘1’]) Raises TypeError
“`
- Improper JSON Handling:
Suppose you receive a JSON response that is improperly accessed:
“`python
import json
response = ‘{“name”: “Alice”, “age”: 30}’
data = json.loads(response)
Incorrect access
print(data[‘name’][0]) This is valid
print(data[‘age’][‘0’]) This will raise TypeError
“`
In the above code, `data[‘age’]` is an integer, and attempting to access it with a string key results in an error.
How to Fix the Error
To resolve the “string indices must be integers, not str” error, consider the following strategies:
- Check Your Indexing: Ensure you are using integers when accessing string elements.
- Validate Data Types: Verify that the data structure you are working with is as expected (string, list, dictionary).
- Use Type Checking: Implement type checks before accessing elements to prevent this error.
Here is a simple way to check types:
“`python
if isinstance(data, str):
print(data[0]) Safe to access as a string
elif isinstance(data, dict):
print(data.get(‘key’)) Accessing dictionary safely
“`
Summary of Best Practices
To prevent encountering this error in your Python code, follow these best practices:
- Always use integer indices for string access.
- Familiarize yourself with the data types you are working with.
- Utilize debugging techniques, such as print statements or logging, to inspect variable types before accessing their elements.
Common Causes | Solution |
---|---|
Using string as index | Use an integer index instead |
Accessing wrong data type | Validate data type before access |
Improper JSON parsing | Check the structure of parsed data |
Understanding the TypeError
The `TypeError: string indices must be integers, not str` occurs in Python when you attempt to access a character in a string using a string index instead of an integer. Python strings are indexed using integers, where each character’s position is represented by a number starting from zero.
Common scenarios that lead to this error include:
- Attempting to access elements of a string with a string key instead of an integer index.
- Misunderstanding the data structure being used, such as confusing a string with a dictionary or list.
Common Causes
- String Access with String Keys: This typically happens when you mistakenly treat a string as a dictionary. For example:
“`python
my_string = “hello”
print(my_string[“h”]) Raises TypeError
“`
- Incorrect Data Structure: If you assume a variable is a dictionary but it’s actually a string:
“`python
my_data = “example”
print(my_data[“key”]) Raises TypeError
“`
- Mixed Data Types: When working with data that can be either a string or a dictionary:
“`python
data = “text”
if isinstance(data, dict):
print(data[“key”]) Raises TypeError if data is a string
“`
Debugging Steps
To resolve this error, follow these debugging steps:
- Check Variable Types: Use the `type()` function to confirm the data type of the variable being accessed.
- Print Statements: Insert print statements before the line causing the error to display the variable’s value and type.
- Review Data Structure: Ensure that you are using the correct data structure for your intended operations.
Example code to illustrate:
“`python
data = “sample”
print(type(data)) Outputs:
if isinstance(data, str):
print(data[0]) Correctly accesses the first character
“`
Best Practices
To prevent encountering this TypeError, adhere to the following best practices:
- Use Descriptive Variable Names: Clear variable names help avoid confusion regarding their types.
- Type Checking: Before performing operations, check if the variable is of the expected type using `isinstance()`.
- Data Structure Awareness: Be familiar with the data structures you are working with, ensuring you understand their properties and methods.
Example Scenarios
Scenario | Code Example | Result |
---|---|---|
Accessing string incorrectly | `my_str = “abc”; print(my_str[“a”])` | Raises TypeError |
Correct integer access | `my_str = “abc”; print(my_str[1])` | Outputs: ‘b’ |
Accessing dictionary | `my_dict = {“key”: “value”}; print(my_dict[“key”])` | Outputs: ‘value’ |
By implementing these practices, you can minimize the risk of encountering the `TypeError: string indices must be integers, not str` in your Python projects.
Understanding the TypeError: String Indices Must Be Integers Not Str
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The TypeError indicating that string indices must be integers typically arises when a programmer attempts to access a character in a string using a string key instead of an integer index. This often occurs in situations where a dictionary is mistakenly treated as a string, leading to confusion in data types.”
Michael Chen (Data Scientist, AI Solutions Group). “In my experience, this error can frequently be found in data manipulation tasks, especially when working with JSON data. It is crucial to ensure that the data structure is correctly understood; for instance, attempting to access a nested dictionary without proper indexing can lead to this error.”
Lisa Patel (Python Developer, CodeCraft Academy). “To effectively debug this TypeError, I recommend using print statements or logging to inspect the types of variables before accessing them. Understanding the data structure you are working with is vital to prevent this common pitfall.”
Frequently Asked Questions (FAQs)
What does the error “TypeError: string indices must be integers, not str” mean?
This error indicates that you are trying to access a character in a string using a string key instead of an integer index. In Python, string indices must be whole numbers representing the position of the character in the string.
When does this error commonly occur?
This error commonly occurs when attempting to access elements of a string as if it were a dictionary or a list. For example, using a string variable with a key like `my_string[‘key’]` will trigger this error.
How can I fix the “TypeError: string indices must be integers, not str” error?
To fix this error, ensure you are using an integer index to access characters in a string. If you intended to access a dictionary, verify that the variable you are using is indeed a dictionary and not a string.
Can this error occur in JSON data handling?
Yes, this error can occur when parsing JSON data if you mistakenly treat a string as a dictionary. Ensure that you are accessing the data correctly, using integer indices for strings and string keys for dictionaries.
What is a common scenario that leads to this error in Python programming?
A common scenario is when iterating over a list of dictionaries and mistakenly accessing a string element. For example, if you loop through a list and try to access a string element using a dictionary key, this error will arise.
Are there any debugging tips to resolve this error?
To debug this error, check the type of the variable you are trying to access. Use the `type()` function to confirm whether it is a string or a dictionary. Additionally, review your code logic to ensure proper data structures are being used.
The error message “TypeError: string indices must be integers, not str” typically arises in Python programming when an attempt is made to access a character in a string using a string index instead of an integer. This error indicates a fundamental misunderstanding of how strings are indexed in Python. Strings are sequences of characters, and each character can only be accessed using its numeric index, which starts from zero. Thus, using a string as an index will lead to this type of error.
Common scenarios that lead to this error include mistakenly treating a string as a dictionary or attempting to access string elements with string keys. For instance, if a developer tries to retrieve a value from a string by using a string key (like in a dictionary), Python will raise this TypeError. It is essential for programmers to differentiate between data types and ensure that the correct indexing methods are applied according to the type of data structure being used.
To resolve this issue, developers should carefully review their code and confirm that they are using integer indices when accessing strings. If the intention is to work with a dictionary, it is crucial to ensure that the variable being accessed is indeed a dictionary and not a string. Understanding the data types and their associated operations is vital for effective programming in
Author Profile
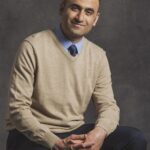
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?