What Is the Return Statement in Python and Why Is It Important?
In the world of programming, the concept of “return” plays a pivotal role in how functions operate, making it a fundamental aspect of Python that every developer should master. Whether you’re a seasoned coder or just beginning your journey, understanding how to effectively use the return statement can significantly enhance the functionality and efficiency of your code. It serves as a bridge between the execution of a function and the output it generates, allowing for greater control over the flow of data and the results of computations.
At its core, the return statement in Python is designed to send a value back to the caller of a function, effectively terminating the function’s execution and passing the result for further use. This simple yet powerful feature not only helps in organizing code but also plays a crucial role in building reusable and modular programs. By using return, developers can encapsulate logic within functions, making their code cleaner and easier to maintain.
Moreover, the versatility of the return statement extends beyond just returning single values; it can also handle complex data types such as lists, dictionaries, or even custom objects. This flexibility allows programmers to create intricate functions that can perform a variety of tasks while still providing meaningful outputs. As we delve deeper into the nuances of the return statement in Python, you’ll discover how to leverage this tool to
Understanding the Return Statement
In Python, the `return` statement is used to exit a function and optionally pass an expression back to the caller. When a function is invoked, it executes its block of code and may return a value to the point where it was called. This is essential for functions that need to provide output or results after performing computations.
The syntax for the `return` statement is straightforward:
python
def function_name(parameters):
# function body
return value
When the `return` statement is executed, the function terminates immediately, and any code following the `return` statement within that function will not be executed. If no value is specified after `return`, the function will return `None` by default.
Function Return Values
Functions can return various types of values, such as integers, strings, lists, or even other functions. The returned value can be stored in a variable for later use. Here are some examples:
- Returning a single value:
python
def add(a, b):
return a + b
result = add(5, 3) # result is 8
- Returning multiple values:
python
def get_coordinates():
return (10, 20)
x, y = get_coordinates() # x is 10, y is 20
- Returning a list:
python
def get_numbers():
return [1, 2, 3, 4, 5]
numbers = get_numbers() # numbers is [1, 2, 3, 4, 5]
Return Statement Characteristics
The `return` statement has several characteristics that are important to understand:
- It can be used to return multiple values, which will be returned as a tuple.
- If a function does not contain a `return` statement, or if it reaches the end of the function body without hitting a `return`, it will return `None`.
- The `return` statement can be placed conditionally within the function, allowing for flexible return behaviors based on input parameters or internal logic.
Characteristics | Description |
---|---|
Single Value Return | Returns one value from the function. |
Tuple Return | Returns multiple values as a tuple. |
Default Return | Returns None if no return statement is present. |
Early Exit | Exits the function immediately when return is executed. |
The `return` statement is a powerful feature that allows for effective control over the flow of data within Python functions. Understanding its use is crucial for writing efficient and maintainable code.
Understanding the `return` Statement
The `return` statement in Python is utilized within functions to send back a value to the caller. This mechanism plays a vital role in function definitions, allowing functions to produce output that can be utilized elsewhere in the code.
Function Structure with Return
A typical function structure that incorporates the `return` statement is illustrated below:
python
def multiply(a, b):
result = a * b
return result
In this example, the `multiply` function takes two arguments, calculates their product, and returns the result to the caller.
Key Characteristics of `return`
– **Early Exit**: The `return` statement terminates the function’s execution immediately. Any code following the `return` statement will not be executed.
– **Multiple Returns**: A function can have multiple `return` statements, often used in conditional statements to return different values based on different conditions.
python
def check_number(num):
if num > 0:
return “Positive”
elif num < 0:
return "Negative"
return "Zero"
- Returning Multiple Values: Functions can return multiple values using tuples.
python
def get_coordinates():
return (10, 20)
x, y = get_coordinates() # x will be 10, y will be 20
Return Value Types
The `return` statement can return various types of values, including:
Return Type | Example |
---|---|
Integer | `return 42` |
Float | `return 3.14` |
String | `return “Hello, World!”` |
List | `return [1, 2, 3]` |
Dictionary | `return {‘key’: ‘value’}` |
Tuple | `return (1, 2)` |
None | `return` (implicitly returns `None`) |
Importance of `return` in Function Design
Using `return` effectively enhances the design and functionality of functions by:
- Encapsulation: It encapsulates logic and data, allowing for modular programming.
- Reusability: Functions that return values can be reused in different parts of the program, reducing redundancy.
- Clarity: Returning values makes it clear what the function is intended to provide, improving code readability.
Best Practices for Using `return`
- Avoid Side Effects: Functions should ideally perform a single task and return a value without causing side effects, such as modifying global variables.
- Use Meaningful Values: Ensure that the values returned are meaningful and relevant to the function’s purpose.
- Explicit Return: If a function does not need to return a value, using `return` explicitly as `return None` can enhance clarity.
Understanding the Role of Return in Python Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, the ‘return’ statement is crucial as it allows a function to send back a value to the caller, effectively enabling the function to produce an output that can be utilized in further calculations or processes.”
Mark Thompson (Lead Python Developer, CodeCraft Solutions). “The ‘return’ statement not only exits a function but also specifies the value that should be returned. This makes it a fundamental aspect of function behavior in Python, allowing for modular and reusable code.”
Linda Garcia (Python Programming Instructor, Future Coders Academy). “Understanding how to use ‘return’ effectively is essential for any Python programmer. It enables functions to communicate results, which is a key principle in functional programming and enhances code clarity.”
Frequently Asked Questions (FAQs)
What is return in Python?
The `return` statement in Python is used to exit a function and send a value back to the caller. It effectively terminates the function’s execution and can return any data type.
How does the return statement affect a function’s execution?
When a `return` statement is executed, the function stops running immediately, and control is passed back to the point where the function was called. Any code following the `return` statement within the function will not be executed.
Can a function return multiple values in Python?
Yes, a function can return multiple values as a tuple. This is done by separating the values with commas in the return statement, allowing the caller to unpack them into separate variables.
What happens if a function does not have a return statement?
If a function does not include a `return` statement, it will return `None` by default upon completion. This indicates that no specific value was returned.
Is it possible to have multiple return statements in a single function?
Yes, a function can contain multiple `return` statements. The first `return` statement that is executed will terminate the function and return its value, while subsequent `return` statements will be ignored.
Can return values be used in expressions in Python?
Yes, the value returned by a function can be used in expressions. This allows for the result of the function to be assigned to a variable, passed as an argument to another function, or used in calculations.
The concept of ‘return’ in Python is fundamental to the functionality of functions. It serves as a mechanism for a function to send back a value to the caller, effectively allowing the function to produce an output based on its internal computations. The ‘return’ statement not only terminates the function but also specifies the value that should be passed back. If no value is specified, the function returns ‘None’ by default, which is an important aspect to consider when designing functions that require specific outputs.
Understanding how to use ‘return’ effectively can significantly enhance the modularity and reusability of code. By returning values, functions can be composed together, allowing for more complex operations to be built from simpler ones. This practice promotes cleaner code and helps in debugging, as it allows developers to isolate functionality and verify outputs at various stages of execution.
Moreover, the use of ‘return’ fosters better control over the flow of a program. It enables conditional outputs and can be strategically placed to exit a function early under certain conditions. This flexibility is crucial in scenarios where functions may need to handle exceptions or special cases without executing the entire block of code. Overall, mastering the ‘return’ statement is essential for any Python programmer aiming to write efficient and
Author Profile
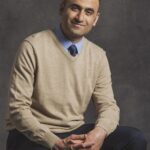
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?