How Can You Use the DD/MM/YYYY Date Format in SQL?
When working with databases, the way we represent dates can significantly impact data manipulation and retrieval. One of the most commonly used formats across various regions is the `dd/mm/yyyy` format, where the day precedes the month and year. This format is particularly prevalent in many countries outside of the United States, making it essential for developers and data analysts to understand how to implement and manipulate this date format within SQL databases. Whether you’re creating queries, inserting records, or formatting output, mastering the `dd/mm/yyyy` date format in SQL can enhance your data management skills and ensure accuracy in your applications.
In SQL, handling date formats can be a nuanced task, especially when different systems and applications may default to varying formats. The `dd/mm/yyyy` format requires specific functions and considerations to ensure that dates are interpreted correctly by the database. Understanding how to convert, compare, and display dates in this format is crucial for effective data analysis and reporting. Moreover, knowing how to handle potential discrepancies in date formats can save time and prevent errors in your SQL queries.
As we delve deeper into the intricacies of using the `dd/mm/yyyy` format in SQL, we will explore various techniques and best practices. From formatting functions to date validation, you’ll gain insights that will empower you to manage date
Date Format in SQL: dd/mm/yyyy
In SQL, the standard date format can vary significantly depending on the database management system (DBMS) in use. However, many systems allow for the use of custom date formats, including `dd/mm/yyyy`. This format, where the day precedes the month and year, is commonly used in many regions, including Europe and Asia.
To work effectively with dates in SQL, it is essential to understand how to manipulate and format date data. Below are some key points regarding date handling in SQL:
- SQL Server: Uses the `CONVERT` function to change the date format. For instance, to convert a date to `dd/mm/yyyy`, the code would look like this:
“`sql
SELECT CONVERT(varchar, GETDATE(), 103) AS FormattedDate;
“`
Here, `103` is the style code for the British/French format.
- MySQL: Utilizes the `DATE_FORMAT` function. To format a date as `dd/mm/yyyy`, you would use:
“`sql
SELECT DATE_FORMAT(NOW(), ‘%d/%m/%Y’) AS FormattedDate;
“`
- PostgreSQL: Supports the `TO_CHAR` function for date formatting:
“`sql
SELECT TO_CHAR(NOW(), ‘DD/MM/YYYY’) AS FormattedDate;
“`
- Oracle: Similar to PostgreSQL, it uses the `TO_CHAR` function:
“`sql
SELECT TO_CHAR(SYSDATE, ‘DD/MM/YYYY’) AS FormattedDate FROM dual;
“`
When working with date formats, it is crucial to consider the implications of regional settings, as incorrect formats can lead to data misinterpretation. Below is a summary of common date format functions across different SQL databases.
DBMS | Function/Method | Example |
---|---|---|
SQL Server | CONVERT | CONVERT(varchar, GETDATE(), 103) |
MySQL | DATE_FORMAT | DATE_FORMAT(NOW(), ‘%d/%m/%Y’) |
PostgreSQL | TO_CHAR | TO_CHAR(NOW(), ‘DD/MM/YYYY’) |
Oracle | TO_CHAR | TO_CHAR(SYSDATE, ‘DD/MM/YYYY’) |
When inserting or updating dates, it is also advisable to ensure the format aligns with the expected input format of the database. This can prevent errors during data manipulation and ensure data integrity across applications.
Additionally, understanding how to handle date comparisons is vital, as different formats can lead to unexpected results in queries. Utilizing functions to convert or format dates appropriately will help maintain consistency and accuracy in your database operations.
Date Format in SQL: dd/mm/yyyy
In SQL, handling date formats can vary significantly between different database management systems (DBMS). The `dd/mm/yyyy` format is commonly used in various regions but may not be natively supported by all SQL databases. Below, we explore how to manage this date format in popular SQL databases.
MySQL
In MySQL, dates are typically stored in the `YYYY-MM-DD` format. To convert a date from `dd/mm/yyyy` to the format MySQL expects, use the `STR_TO_DATE()` function.
“`sql
SELECT STR_TO_DATE(’31/12/2023′, ‘%d/%m/%Y’) AS formatted_date;
“`
This will convert the string ’31/12/2023′ into a date object recognized by MySQL.
SQL Server
SQL Server utilizes the `CONVERT()` function to manage date formats. To convert a date string in the `dd/mm/yyyy` format, specify the appropriate style code.
“`sql
SELECT CONVERT(DATE, ’31/12/2023′, 103) AS formatted_date;
“`
In this case, `103` indicates the British/French style, which corresponds to the `dd/mm/yyyy` format.
PostgreSQL
PostgreSQL uses the `TO_DATE()` function to convert strings into date formats. To parse a date string in the `dd/mm/yyyy` format, the following syntax can be used:
“`sql
SELECT TO_DATE(’31/12/2023′, ‘DD/MM/YYYY’) AS formatted_date;
“`
This command will yield a date object in PostgreSQL.
Oracle
In Oracle databases, the `TO_DATE()` function also serves to convert string representations of dates. To specify the `dd/mm/yyyy` format, use the following syntax:
“`sql
SELECT TO_DATE(’31/12/2023′, ‘DD/MM/YYYY’) AS formatted_date FROM dual;
“`
This effectively transforms the string into an Oracle date.
Best Practices for Date Management
When working with dates in SQL, consider these best practices:
- Always Store Dates in a Standard Format: Use the native date type of the DBMS to avoid confusion.
- Use Functions for Conversion: Make use of the provided date functions to ensure accurate parsing.
- Be Aware of Locale Settings: Different regions may have varying default date formats; always specify the format explicitly.
- Validate Input Dates: Ensure that the date strings conform to the expected format before processing them.
Common Errors and Troubleshooting
When handling date formats, some common issues may arise:
Error Type | Description | Solution |
---|---|---|
Incorrect Format | Input string does not match expected format | Validate the input format before conversion |
Locale Mismatch | Database settings conflict with date format used | Specify the format in the conversion function |
Data Type Confusion | Storing dates as strings instead of date types | Always use date data types for date values |
By adhering to the aforementioned practices and solutions, effective date management in SQL can be achieved, ensuring accuracy and consistency across applications.
Understanding SQL Date Formats: Expert Insights
Dr. Emily Carter (Database Architect, Tech Solutions Inc.). “Using the date format dd/mm/yyyy in SQL can lead to significant confusion, especially in environments where the default format is set to mm/dd/yyyy. It is crucial to explicitly specify the format during data entry and retrieval to avoid misinterpretation of date values.”
Michael Chen (Senior SQL Developer, DataWise Corp.). “When working with SQL databases, understanding the implications of date formats is essential. The dd/mm/yyyy format, while common in many regions, may not be recognized by all SQL dialects. It is advisable to convert dates to a standard format like ISO 8601 (yyyy-mm-dd) for compatibility and to prevent errors.”
Lisa Thompson (Data Analyst, Analytics Pro). “Inserting and querying dates in the dd/mm/yyyy format can lead to unexpected results if the database settings do not align with this format. Always check the database locale settings and consider using functions like STR_TO_DATE() in MySQL to ensure accurate date handling.”
Frequently Asked Questions (FAQs)
What is the standard date format in SQL?
The standard date format in SQL is typically ‘YYYY-MM-DD’. This format is used for storing and manipulating date values in databases.
Can I use the format DD/MM/YYYY in SQL?
Yes, you can use the DD/MM/YYYY format in SQL, but it requires conversion. SQL databases may not recognize this format directly, so you should convert it to the standard format before storing or querying.
How do I convert a date from DD/MM/YYYY to YYYY-MM-DD in SQL?
You can use the `STR_TO_DATE` function in MySQL or `CONVERT` function in SQL Server to convert dates. For example, in MySQL:
`SELECT STR_TO_DATE(’31/12/2023′, ‘%d/%m/%Y’);`
What happens if I insert a date in the wrong format?
Inserting a date in the wrong format may result in an error or the date being stored incorrectly. It is essential to ensure that the date format matches the expected format of the database.
Are there any SQL functions to handle different date formats?
Yes, SQL provides several functions like `FORMAT`, `CAST`, and `CONVERT` to handle different date formats. These functions allow you to format, convert, and manipulate date values as needed.
Is it possible to set a default date format for an SQL session?
Yes, you can set a default date format for an SQL session using session variables or configuration settings, depending on the database system you are using. For example, in MySQL, you can set the `DATE_FORMAT` session variable.
In SQL, handling date formats is crucial for ensuring accurate data manipulation and retrieval. The common date format of dd/mm/yyyy is frequently used in various regions, particularly in Europe and Asia. However, SQL databases often default to the format of yyyy-mm-dd. This discrepancy can lead to confusion and errors if not properly addressed. Understanding how to convert and format dates in SQL is essential for developers and database administrators to maintain data integrity and facilitate effective querying.
To work with the dd/mm/yyyy format in SQL, one must utilize functions such as `STR_TO_DATE()` for MySQL or `TO_DATE()` for Oracle databases. These functions allow users to convert string representations of dates into the appropriate date data types recognized by SQL. Additionally, formatting functions such as `DATE_FORMAT()` in MySQL can be employed to display dates in the desired format when retrieving data. This understanding is vital for ensuring that date comparisons and calculations yield accurate results.
Moreover, it is important to be aware of the database settings and locale configurations that may affect date interpretation. When inserting or querying dates, using the correct format and function can prevent misinterpretation by the database engine. This attention to detail is particularly important in applications where dates play a critical role, such as
Author Profile
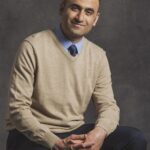
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?