How Can I Effectively Use Nested Queries in a Data Fetcher?
In the world of data management and retrieval, the ability to efficiently access and manipulate information is paramount. As applications grow in complexity, so does the need for sophisticated querying techniques that can handle intricate data structures. Enter the concept of nested queries in data fetchers—a powerful tool that allows developers to dive deeper into their datasets, extracting nuanced insights and relationships that flat queries simply can’t uncover. Whether you’re building a robust API, developing a dynamic web application, or analyzing large datasets, understanding nested queries can significantly enhance your data-fetching capabilities.
Nested queries, often referred to as subqueries, are an advanced querying technique that enables users to execute one query within another. This allows for a more granular approach to data retrieval, where the results of one query can influence or filter the results of another. In practice, this means that developers can create more complex and efficient data-fetching strategies, particularly when dealing with hierarchical or relational data. As we explore the intricacies of nested queries, we will uncover their potential to streamline data access and improve application performance.
Moreover, the implementation of nested queries can vary significantly across different data-fetching frameworks and languages. Understanding the nuances of these variations is crucial for developers looking to optimize their applications. In the following sections, we will delve into the mechanics of nested
Understanding Nested Queries
Nested queries, often referred to as subqueries, are a powerful feature in data fetchers that allow users to retrieve data from multiple related sources in a single query. These queries are executed within another query, enabling users to filter or manipulate data based on results from another data set. The structure of nested queries can enhance the efficiency of data retrieval processes and provide deeper insights.
When implementing nested queries, the inner query executes first, and its results are then utilized by the outer query. This hierarchical approach is particularly useful in scenarios where complex relationships exist between data entities.
Benefits of Using Nested Queries
Utilizing nested queries can bring several advantages to data manipulation and retrieval processes:
- Enhanced Data Relationships: Nested queries allow for the exploration of complex relationships between different datasets.
- Improved Performance: By reducing the need for multiple queries, nested queries can enhance performance, especially when dealing with large datasets.
- Simplified Queries: They can simplify the overall structure of the query, making it easier to read and maintain.
- Dynamic Filtering: Nested queries enable dynamic data filtering based on the results of another query, leading to more tailored results.
Examples of Nested Queries
To illustrate the concept, consider the following SQL examples:
- **Simple Nested Query**:
“`sql
SELECT employee_name
FROM employees
WHERE department_id IN (SELECT department_id FROM departments WHERE location_id = 100);
“`
In this example, the inner query retrieves `department_id` values for departments located at `location_id` 100, which the outer query then uses to fetch employee names from those departments.
- **Correlated Nested Query**:
“`sql
SELECT employee_name
FROM employees e
WHERE salary > (SELECT AVG(salary) FROM employees WHERE department_id = e.department_id);
“`
Here, the inner query calculates the average salary for each department, and the outer query retrieves names of employees who earn more than the average salary in their respective departments.
Considerations When Using Nested Queries
While nested queries are beneficial, there are some considerations to keep in mind:
- Performance Impact: Overusing nested queries can lead to performance degradation, particularly if the inner query returns a large result set.
- Readability: Complex nested queries can become challenging to read and maintain; thus, it’s essential to strike a balance between complexity and clarity.
- Database Support: Not all database systems handle nested queries in the same way, leading to potential compatibility issues.
Table of Nested Query Types
Type | Description | Use Case |
---|---|---|
Single Nested Query | A query within another query where the inner query executes first. | Filtering results based on a specific condition. |
Correlated Nested Query | An inner query that references columns from the outer query. | Calculating aggregates based on related records. |
Multiple Nested Queries | Queries nested within each other at multiple levels. | Complex data retrieval from interrelated tables. |
Incorporating nested queries into data fetching strategies can lead to more efficient and insightful data manipulation, provided that best practices are followed to mitigate performance concerns and maintain readability.
Understanding Nested Queries in Data Fetching
Nested queries allow for more complex data retrieval by embedding one query within another. This technique is particularly useful when dealing with hierarchical or related data structures. By structuring queries in this manner, developers can streamline their data-fetching process and reduce the number of API calls or database queries required.
Benefits of Using Nested Queries
Nested queries provide several advantages, including:
- Efficiency: Reduces the number of separate requests needed to gather related data.
- Simplicity: Encapsulates complex data relationships within a single query structure, enhancing readability.
- Atomicity: Ensures that related data is retrieved in a single transaction, maintaining consistency.
Common Use Cases for Nested Queries
Nested queries are commonly utilized in various scenarios, such as:
- Database Management Systems: To retrieve data from multiple tables in a relational database.
- GraphQL APIs: To fetch related data in one API call, reducing overhead.
- NoSQL Databases: For aggregating and retrieving hierarchical data structures.
Example of a Nested Query in SQL
Consider a scenario where you want to retrieve a list of users along with their associated orders from a relational database. A nested query might look like this:
“`sql
SELECT u.id, u.name,
(SELECT COUNT(*) FROM orders o WHERE o.user_id = u.id) AS order_count
FROM users u;
“`
In this example, the inner query counts the number of orders for each user, providing a more comprehensive view of user data in a single call.
Implementing Nested Queries in GraphQL
In GraphQL, nested queries can be implemented by defining relationships in the schema. For instance, if you have a `User` type that has an associated list of `Orders`, a nested query can be structured as follows:
“`graphql
{
users {
id
name
orders {
id
total
}
}
}
“`
This query retrieves users along with their respective orders in one request, leveraging the relational capabilities of GraphQL.
Performance Considerations
While nested queries can enhance efficiency, there are important performance considerations to keep in mind:
- Data Volume: Large datasets can lead to increased response times; consider pagination strategies.
- Complexity: Deeply nested queries can become difficult to manage and debug, so limit nesting levels when possible.
- Indexing: Ensure proper indexing in databases to optimize query performance.
Tools and Libraries for Nested Queries
Several tools and libraries facilitate the use of nested queries, including:
Tool/Library | Description |
---|---|
SQL | Standard query language for relational databases. |
GraphQL | Query language for APIs, allowing nested data retrieval. |
Prisma | ORM that simplifies database interactions with nested queries. |
MongoDB | NoSQL database that supports nested document structures. |
Utilizing these tools can streamline the implementation and management of nested queries, ensuring optimal performance and maintainability.
Expert Insights on Nested Queries in Data Fetching
Dr. Emily Chen (Data Architect, Tech Innovations Inc.). “Nested queries are essential in optimizing data retrieval processes, especially when dealing with complex datasets. They allow for more efficient filtering and aggregation of data, which can significantly improve application performance.”
Michael Torres (Senior Software Engineer, Data Solutions Group). “Utilizing nested queries in data fetchers provides a powerful mechanism to handle relationships between data entities. This approach not only enhances the clarity of the data retrieval logic but also minimizes the number of calls to the database, thus reducing latency.”
Lisa Patel (Database Consultant, Analytics Experts LLC). “When implemented correctly, nested queries can simplify complex data interactions and improve the maintainability of code. However, developers must be cautious of potential performance drawbacks, especially with deeply nested structures that can lead to inefficiencies.”
Frequently Asked Questions (FAQs)
What is a nested query in a data fetcher?
A nested query in a data fetcher refers to a query that retrieves data from multiple related sources or tables, where one query is embedded within another. This allows for complex data retrieval scenarios, enabling users to obtain comprehensive datasets in a single request.
How do I implement a nested query in a data fetcher?
To implement a nested query, you typically structure your query to include subqueries or joins that specify the relationships between the data sources. This can often be done using SQL syntax or specific query language features provided by the data fetching tool.
What are the benefits of using nested queries?
Nested queries enhance data retrieval efficiency by allowing users to gather related data in one go, reducing the number of requests made to the database. They also enable more complex data relationships to be represented and queried effectively.
Are there performance considerations when using nested queries?
Yes, nested queries can impact performance, particularly if they involve large datasets or complex relationships. It is essential to optimize the queries by indexing relevant fields and minimizing the depth of nesting to ensure efficient data fetching.
Can all data fetchers support nested queries?
Not all data fetchers support nested queries. The capability to execute nested queries depends on the specific data fetching technology or database management system being used. Always consult the documentation for the specific tool to confirm support.
What are common pitfalls when using nested queries?
Common pitfalls include excessive complexity leading to performance degradation, misunderstanding the relationships between data sources, and failing to handle null or missing values properly. Careful planning and testing are crucial to avoid these issues.
In the realm of data fetching, nested queries play a pivotal role in enhancing the efficiency and depth of data retrieval processes. A nested query, often referred to as a subquery, allows for the execution of a query within another query. This structure enables developers to extract complex data relationships and hierarchies from databases, facilitating more sophisticated data analysis and manipulation. By leveraging nested queries, data fetchers can streamline their operations, making it easier to handle multi-layered data requirements.
One of the key insights from the discussion on nested queries is their ability to simplify complex data retrieval tasks. Instead of executing multiple separate queries, a nested query can encapsulate the logic needed to fetch related data in a single operation. This not only improves performance by reducing the number of database calls but also enhances the readability and maintainability of the code. Developers can write more concise and efficient queries that yield the desired results without unnecessary overhead.
Additionally, it is essential to consider the potential performance implications of using nested queries. While they can provide significant advantages in terms of data organization and retrieval, poorly constructed nested queries may lead to inefficiencies and slower response times. Therefore, developers must strike a balance between leveraging the power of nested queries and ensuring that their queries are
Author Profile
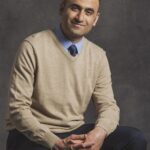
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?