How Can I Use Regular Expressions to Validate Social Security Numbers?
In an increasingly digital world, the need for secure and efficient data handling is more critical than ever. One of the most sensitive pieces of information individuals possess is their Social Security Number (SSN). As a unique identifier in the United States, the SSN plays a vital role in various transactions, from applying for loans to filing taxes. However, with the rise of data breaches and identity theft, ensuring that this information is processed and stored securely is paramount. Enter regular expressions—a powerful tool that can help developers and data analysts validate, search, and manipulate SSNs with precision and ease. In this article, we will explore the intricacies of using regular expressions to handle Social Security Numbers, equipping you with the knowledge to safeguard this crucial data.
Regular expressions, often abbreviated as regex, are sequences of characters that define a search pattern. They are widely used in programming and data processing to match strings of text, making them invaluable for tasks such as validating formats, searching for specific patterns, and extracting information. When it comes to Social Security Numbers, regex provides a systematic way to ensure that the SSNs entered into systems conform to the correct format, thereby reducing errors and enhancing security. Understanding how to construct a regular expression for SSNs can empower developers to create more
Understanding Regular Expressions for Social Security Numbers
Regular expressions (regex) are powerful tools used for pattern matching within strings, which makes them particularly useful for validating formats like Social Security Numbers (SSNs). An SSN is typically structured as three digits, followed by two digits, and then four digits, represented in the format `AAA-GG-SSSS`.
To create a regex pattern for validating SSNs, one must consider the specific format and potential restrictions. The following regex pattern can be utilized:
“`
^\d{3}-\d{2}-\d{4}$
“`
This expression breaks down as follows:
- `^` asserts the start of the string.
- `\d{3}` matches exactly three digits (the first segment).
- `-` matches the hyphen.
- `\d{2}` matches exactly two digits (the second segment).
- `-` matches the second hyphen.
- `\d{4}` matches exactly four digits (the last segment).
- `$` asserts the end of the string.
Examples of Valid and Invalid SSNs
When using the regex pattern, certain strings will match the criteria while others will not. Here are examples for clarity:
Example | Valid/Invalid |
---|---|
123-45-6789 | Valid |
123-456-789 | Invalid |
123-45-67890 | Invalid |
123-45-67A9 | Invalid |
123-45-678 | Invalid |
Additional Considerations
While the regex pattern can effectively validate the basic format of an SSN, additional considerations may be necessary:
- Exclusion of Certain Patterns: Certain SSNs are invalid by default, such as those starting with `666` or `000` or containing `123-45-6789`, which is often used as a placeholder.
- Whitespace Handling: If SSNs may include spaces, the regex can be adapted to account for optional whitespace.
An enhanced regex pattern that considers the exclusion of specific invalid SSNs might look like this:
“`
^(?!666|000)\d{3}-(?!00)\d{2}-(?!0{4})\d{4}$
“`
This pattern ensures that the first segment does not start with `666` or `000`, the second segment is not `00`, and the last segment does not consist of four zeros.
Testing and Implementation
When implementing regex for SSN validation, it is crucial to test the pattern against various input cases. Here are recommended testing scenarios:
- Valid SSNs in correct format.
- Invalid SSNs in incorrect formats.
- Edge cases, such as empty strings or strings with only hyphens.
By applying the regex in programming environments (such as Python, JavaScript, or Java), developers can enforce the correct SSN format in applications where personal identification is essential.
Understanding the Regular Expression for Social Security Numbers
The regular expression (regex) for validating Social Security Numbers (SSNs) is designed to ensure proper formatting and adherence to specific patterns. An SSN consists of nine digits, typically represented in the format “XXX-XX-XXXX”. Here’s a breakdown of the regex pattern used to validate SSNs:
- Pattern: `^\d{3}-\d{2}-\d{4}$`
Explanation of the Regex Components
- `^`: Asserts the start of the line.
- `\d{3}`: Matches exactly three digits (the first part of the SSN).
- `-`: Matches the hyphen separating the first and second parts of the SSN.
- `\d{2}`: Matches exactly two digits (the second part of the SSN).
- `-`: Matches the hyphen separating the second and third parts of the SSN.
- `\d{4}`: Matches exactly four digits (the last part of the SSN).
- `$`: Asserts the end of the line.
Additional Considerations
While the basic regex validates the format, it does not account for invalid SSNs, which can include specific combinations that are not issued. Here are some common invalid patterns to consider:
- All zeros: `000-00-0000`
- Numbers in certain ranges:
- First three digits cannot be `666` or `000`.
- Second two digits cannot be `00`.
- Last four digits cannot be `0000`.
Enhanced Regex for Validation
To incorporate these considerations, a more complex regex can be utilized:
- Enhanced Pattern: `^(?!666|000)\d{3}-(?!00)\d{2}-(?!0000)\d{4}$`
This enhanced pattern includes negative lookaheads to prevent specific invalid formats.
Example Valid and Invalid SSNs
SSN | Validity |
---|---|
123-45-6789 | Valid |
000-12-3456 | Invalid |
123-00-6789 | Invalid |
666-45-6789 | Invalid |
123-45-0000 | Invalid |
Implementation in Programming Languages
In different programming languages, the regex can be implemented similarly. Here are examples in Python and JavaScript:
Python Example
“`python
import re
def is_valid_ssn(ssn):
pattern = r’^(?!666|000)\d{3}-(?!00)\d{2}-(?!0000)\d{4}$’
return bool(re.match(pattern, ssn))
“`
JavaScript Example
“`javascript
function isValidSSN(ssn) {
const pattern = /^(?!666|000)\d{3}-(?!00)\d{2}-(?!0000)\d{4}$/;
return pattern.test(ssn);
}
“`
By utilizing these patterns and functions, developers can effectively validate Social Security Numbers to ensure compliance with standard formatting and avoid common pitfalls associated with invalid entries.
Expert Insights on Regular Expressions for Social Security Numbers
Dr. Emily Carter (Data Privacy Consultant, SecureTech Solutions). “Using regular expressions to validate Social Security Numbers is essential for ensuring data integrity. A well-structured regex can help identify valid formats while preventing the entry of incorrect or malicious data.”
James Thornton (Software Engineer, CodeGuard Technologies). “When implementing regular expressions for Social Security Numbers, it is crucial to consider edge cases. A regex pattern must not only match the standard format but also account for variations that could arise in user input.”
Linda Chen (Compliance Officer, FinSecure Corp). “Incorporating regular expressions for Social Security Number validation is not just a technical requirement; it also plays a significant role in compliance with data protection regulations. Ensuring that only valid SSNs are processed can mitigate risks associated with identity theft.”
Frequently Asked Questions (FAQs)
What is a regular expression for a Social Security Number (SSN)?
A regular expression for a Social Security Number typically follows the pattern `^\d{3}-\d{2}-\d{4}$`, which matches the format of three digits, a hyphen, two digits, another hyphen, and four digits.
Why is it important to validate SSNs using regular expressions?
Validating SSNs using regular expressions ensures that the entered data adheres to the correct format, preventing errors in data collection and enhancing data integrity.
Can a regular expression for SSNs account for invalid numbers?
While a basic regular expression can verify the format, it cannot check for invalid SSNs, such as those that are not issued or are otherwise incorrect. Additional logic is required for comprehensive validation.
Are there variations in the regular expression for SSNs across different programming languages?
Yes, variations may exist in syntax and delimiters depending on the programming language. For example, some languages may require escaping certain characters differently.
How can I implement a regular expression for SSN validation in my application?
You can implement SSN validation by using the regular expression in your programming language’s regex library, applying it to user input, and checking for matches against the defined pattern.
What should I do if the SSN does not match the regular expression?
If the SSN does not match the regular expression, prompt the user to re-enter the number, providing guidance on the correct format to ensure compliance with the expected structure.
In summary, a regular expression (regex) for validating Social Security Numbers (SSNs) is a powerful tool for ensuring that the format of these sensitive identifiers is correct. An SSN typically follows the pattern of three digits, followed by two digits, and then four digits, often represented as “XXX-XX-XXXX”. The regex pattern for this format is usually expressed as `^\d{3}-\d{2}-\d{4}$`, which enforces the structure and helps prevent the entry of invalid SSNs.
It is important to note that while regex can effectively validate the format of an SSN, it does not verify the authenticity of the number itself. For example, it cannot determine if the SSN has been issued or if it belongs to a living individual. Therefore, while regex serves as a preliminary check, additional validation methods may be necessary for comprehensive data integrity.
Furthermore, when implementing regex for SSN validation, developers should consider the context in which SSNs are used. Data privacy laws and regulations, such as the GDPR or HIPAA, impose strict guidelines on the handling of personal information, including SSNs. Therefore, it is crucial to ensure that any system using regex for SSN validation complies
Author Profile
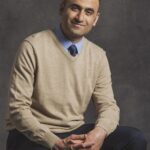
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?