How Can You Easily Check Your Node.js Version?
Node.js has revolutionized the way developers build server-side applications, providing a powerful platform that leverages JavaScript’s asynchronous capabilities. Whether you’re a seasoned developer or just starting out, knowing the version of Node.js you’re working with is crucial for ensuring compatibility with libraries, frameworks, and tools. As the ecosystem evolves, keeping track of your Node.js version can help you harness the latest features and improvements while avoiding potential pitfalls associated with outdated versions.
In this article, we will explore the straightforward methods to check your Node.js version, ensuring you’re equipped with the knowledge to maintain your development environment effectively. Understanding how to identify the version you’re using can aid in troubleshooting issues, managing dependencies, and optimizing your application’s performance. We’ll cover various approaches, from command-line techniques to graphical interfaces, making it easy for anyone to verify their setup.
Whether you’re working on a personal project or collaborating on a large-scale application, being aware of your Node.js version is a fundamental skill that can enhance your development workflow. Join us as we delve into the essential steps to check your Node.js version and empower yourself with the tools to navigate this dynamic programming landscape confidently.
Checking Node.js Version via Command Line
To determine the installed version of Node.js on your system, you can use the command line interface. This is a straightforward process that can be performed in various operating systems, including Windows, macOS, and Linux.
- Open your command line interface:
- Windows: Search for “Command Prompt” or “PowerShell” in the Start menu.
- macOS: Open “Terminal” from Applications or search for it using Spotlight.
- Linux: Access the terminal from the applications menu or use a keyboard shortcut (usually Ctrl + Alt + T).
- Type the following command:
“`bash
node -v
“`
or
“`bash
node –version
“`
- Press Enter: The output will display the currently installed version of Node.js. For example, it might return something like `v18.12.1`.
Checking Node.js Version via Package Manager
If you have installed Node.js using a package manager, you can also check the version through that manager. Below are instructions for some popular package managers.
- Using npm:
- Open your command line interface.
- Run the command:
“`bash
npm -v
“`
- This will return the version of npm, which is often updated in tandem with Node.js.
- Using Homebrew (macOS):
- If you installed Node.js using Homebrew, you can check the version with:
“`bash
brew list node
“`
- This command will show the installed version alongside other information.
- Using Apt (Debian/Ubuntu):
- For systems using the apt package manager, you can check Node.js version by:
“`bash
apt show nodejs
“`
Node.js Versioning Information
Node.js follows semantic versioning, which consists of three numbers: major, minor, and patch. Understanding these components can help you manage updates and compatibility better.
Version Component | Description |
---|---|
Major | Introduces breaking changes and significant updates. |
Minor | Adds functionality in a backward-compatible manner. |
Patch | Includes backward-compatible bug fixes. |
Understanding the versioning of Node.js is crucial when managing dependencies and ensuring compatibility with libraries and frameworks. By keeping your Node.js version updated, you can leverage the latest features and security improvements.
Using the Command Line to Check Node.js Version
To verify the version of Node.js installed on your system, you can utilize the command line interface. This method is straightforward and effective for all operating systems, including Windows, macOS, and Linux.
- Open your command line interface:
- Windows: Press `Win + R`, type `cmd`, and hit `Enter`.
- macOS: Press `Command + Space`, type `Terminal`, and hit `Enter`.
- Linux: Open your terminal emulator from the applications menu.
- Execute the version command:
- Type the following command and press `Enter`:
“`
node -v
“`
- Alternatively, you can use:
“`
node –version
“`
The output will display the installed Node.js version, such as `v16.13.0`. This method ensures you know exactly which version is currently in use.
Checking Node.js Version through npm
If you have npm (Node Package Manager) installed alongside Node.js, you can also check the version of Node.js using npm commands. This is particularly useful if you want to ensure compatibility with specific packages.
- Open your command line interface.
- Execute the following command:
“`
npm version
“`
This command will return an output that includes the current versions of Node.js and npm, formatted as follows:
“`
{ npm: ‘8.1.0’,
ares: ‘1.18.0’,
http_parser: ‘5.0’,
node: ‘16.13.0’,
v8: ‘9.4.146.24-node.14’,
uv: ‘1.41.0’,
zlib: ‘1.2.11’,
brotli: ‘1.0.9’,
cryptography: ‘1.1.1’ }
“`
Look for the `node` entry in the output to determine your Node.js version.
Using Node.js REPL to Check Version
Another method to check the Node.js version is by accessing the Node.js REPL (Read-Eval-Print Loop). This approach allows you to interact directly with Node.js.
- Open your command line interface.
- Launch Node.js REPL:
- Simply type `node` and press `Enter`.
- Check the version:
- Within the REPL, type:
“`javascript
process.version
“`
- Press `Enter`. The output will display the version in a format similar to `v16.13.0`.
To exit the REPL, type `.exit` or press `Ctrl + C` twice.
Verifying Node.js Version in Application Code
In addition to command-line methods, you can also check the Node.js version programmatically within your application. This is useful for logging or conditional logic based on the Node.js version.
Here’s a simple code snippet to include in your JavaScript file:
“`javascript
console.log(`Node.js version: ${process.version}`);
“`
When you run your application, this will output the current Node.js version to the console.
Utilizing Package Managers to Check Node.js Version
If you installed Node.js using a package manager, such as Homebrew on macOS or apt on Ubuntu, you can check the installed version using the package manager commands.
- Homebrew (macOS):
“`
brew list –versions node
“`
- apt (Ubuntu):
“`
apt list –installed | grep nodejs
“`
These commands will list the installed version of Node.js as managed by the respective package manager.
Expert Insights on Checking Node.js Version
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To check the Node.js version, you can simply use the command ‘node -v’ in your terminal. This command provides a quick and reliable way to verify the installed version, ensuring that your development environment is set up correctly.”
Michael Chen (Lead Developer, Open Source Projects). “It’s essential to keep your Node.js version updated for security and performance reasons. Running ‘node –version’ will not only show you the current version but also help you determine if an upgrade is necessary for your projects.”
Sarah Thompson (Technical Writer, Node.js Foundation). “For those new to Node.js, using ‘node -v’ is the most straightforward method to check your version. Additionally, incorporating version checks into your deployment scripts can help maintain consistency across different environments.”
Frequently Asked Questions (FAQs)
How can I check the Node.js version installed on my system?
You can check the Node.js version by opening your terminal or command prompt and executing the command `node -v`. This will display the currently installed version of Node.js.
What command should I use to verify the npm version?
To verify the version of npm (Node Package Manager), use the command `npm -v` in your terminal or command prompt. This will return the version number of npm installed alongside Node.js.
Is there a way to check the Node.js version from within a JavaScript file?
Yes, you can check the Node.js version within a JavaScript file by using `console.log(process.version);`. This will output the version of Node.js when the script is executed.
What if the command ‘node -v’ returns an error?
If the command `node -v` returns an error, it may indicate that Node.js is not installed or not added to your system’s PATH. Ensure that Node.js is installed correctly and that the installation directory is included in your PATH environment variable.
Can I check the Node.js version using a package manager?
Yes, if you installed Node.js using a package manager like Homebrew on macOS or apt on Ubuntu, you can check the version using commands like `brew info node` or `apt show nodejs`, respectively. These commands will provide details about the installed version.
How can I update Node.js to the latest version?
To update Node.js to the latest version, you can use a version manager like nvm (Node Version Manager) by running `nvm install node` or you can download the latest installer from the official Node.js website and run it.
In summary, checking the Node.js version installed on your system is a straightforward process that can be accomplished using the command line interface. The most common method involves executing the command `node -v` or `node –version`, which will return the current version of Node.js. This is essential for developers to ensure compatibility with various libraries and frameworks that may require specific Node.js versions.
Additionally, it is important to recognize that keeping your Node.js version up to date can enhance performance and security. Regularly checking the version allows developers to stay informed about the latest features and improvements, which can lead to more efficient coding practices. Furthermore, understanding the versioning system, including major, minor, and patch updates, can help in making informed decisions regarding upgrades.
being familiar with the methods to check the Node.js version is a crucial skill for any developer working within the JavaScript ecosystem. This knowledge not only aids in maintaining a stable development environment but also contributes to better project management and collaboration among team members. Regularly verifying the Node.js version should be a part of best practices in software development.
Author Profile
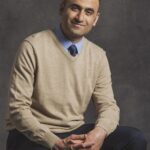
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?