How Can You Determine the Latest Date Using Perl?
In the world of programming, working with dates can often be a complex task, especially when it comes to determining which date is the latest. For developers using Perl, a versatile and powerful scripting language, mastering date manipulation is essential for a wide range of applications—from data analysis to scheduling events. Whether you’re managing timestamps in databases or simply comparing dates in your code, knowing how to effectively determine the latest date can save you time and enhance the functionality of your programs.
Perl offers several built-in modules and functions that simplify date handling, allowing you to compare dates with ease. By leveraging these tools, you can perform operations such as parsing date strings, converting them into comparable formats, and ultimately identifying which date stands out as the most recent. Understanding the nuances of date formats and the intricacies of time zones is crucial for accurate comparisons, especially in applications that span multiple regions or require precise timing.
As we delve deeper into the methods and best practices for determining the latest date in Perl, you’ll discover a range of techniques that cater to different scenarios. From simple comparisons using native Perl functions to utilizing powerful date manipulation modules, this article will equip you with the knowledge you need to handle dates confidently and efficiently in your Perl projects. Get ready to unlock the potential of date manipulation and elevate your programming
Using Perl to Compare Dates
In Perl, comparing dates involves converting date strings into a format that can be easily compared. The `Time::Local` module is often utilized for this purpose, allowing for the manipulation of time and date values in a straightforward manner.
To determine which date is the latest, you can convert each date into epoch time (the number of seconds since January 1, 1970) and then compare these values. Here’s a step-by-step guide on how to perform this task.
Step-by-Step Guide
- Install the Time::Local Module: Ensure that you have the `Time::Local` module installed. You can install it from CPAN if it is not already available in your Perl environment.
- Parse the Dates: Convert the date strings into epoch time. This can be done using the `timelocal` function from the `Time::Local` module.
- Compare the Epoch Values: Once you have the epoch values for each date, simply compare them using standard comparison operators.
Example Code
Here is a sample Perl script that demonstrates how to find the latest date from two date strings:
“`perl
use strict;
use warnings;
use Time::Local;
Sample date strings
my $date1 = ‘2023-10-01’;
my $date2 = ‘2023-11-15’;
Convert date strings to epoch time
my $epoch1 = timelocal(0, 0, 0, substr($date1, 8, 2), substr($date1, 5, 2) – 1, substr($date1, 0, 4));
my $epoch2 = timelocal(0, 0, 0, substr($date2, 8, 2), substr($date2, 5, 2) – 1, substr($date2, 0, 4));
Compare epochs
if ($epoch1 > $epoch2) {
print “$date1 is the latest date.\n”;
} elsif ($epoch1 < $epoch2) {
print "$date2 is the latest date.\n";
} else {
print "Both dates are the same.\n";
}
```
Understanding the Code
- The `timelocal` function takes arguments in the order of seconds, minutes, hours, day, month (0-11), and year. The `substr` function is used to extract these components from the date strings.
- The comparison of epoch values determines which date is more recent.
Considerations for Date Formats
When working with dates, it’s crucial to consider the format you are using. The above example uses the `YYYY-MM-DD` format, which is widely recognized. However, other formats may require additional parsing logic.
Here’s a quick reference table for common date formats:
Format | Example |
---|---|
YYYY-MM-DD | 2023-10-01 |
MM/DD/YYYY | 10/01/2023 |
DD-MM-YYYY | 01-10-2023 |
Ensuring consistency in date formats is essential for accurate comparisons. If your dates come in varying formats, consider normalizing them before comparison.
By following these steps and utilizing Perl’s capabilities, you can effectively determine which date is the latest in your applications.
Using Perl’s Time Functions
Perl provides several built-in functions and modules to work with dates and times effectively. To determine which date is the latest, you can utilize the `Time::Local` and `POSIX` modules, which offer a robust way to manipulate and compare date values.
Comparing Dates with Time::Local
The `Time::Local` module allows you to convert a date into a timestamp. This timestamp can then be easily compared. Here’s how to implement it:
“`perl
use Time::Local;
sub latest_date {
my ($date1, $date2) = @_;
my ($d1_year, $d1_month, $d1_day) = split /-/, $date1;
my ($d2_year, $d2_month, $d2_day) = split /-/, $date2;
my $timestamp1 = timelocal(0, 0, 0, $d1_day, $d1_month – 1, $d1_year);
my $timestamp2 = timelocal(0, 0, 0, $d2_day, $d2_month – 1, $d2_year);
return $timestamp1 > $timestamp2 ? $date1 : $date2;
}
my $latest = latest_date(‘2023-10-01’, ‘2023-09-30’);
print “The latest date is: $latest\n”;
“`
Using the DateTime Module
For more advanced date manipulation, the `DateTime` module is recommended. It provides a more intuitive interface for handling dates and times.
“`perl
use DateTime;
sub latest_date_dt {
my ($date1, $date2) = @_;
my $dt1 = DateTime->from_epoch(epoch => str2time($date1));
my $dt2 = DateTime->from_epoch(epoch => str2time($date2));
return $dt1 > $dt2 ? $dt1->ymd : $dt2->ymd;
}
my $latest_dt = latest_date_dt(‘2023-10-01’, ‘2023-09-30’);
print “The latest date is: $latest_dt\n”;
“`
Parsing Dates
When parsing dates, ensure the format is consistent. Common formats include:
- `YYYY-MM-DD`
- `DD/MM/YYYY`
- `MM-DD-YYYY`
Utilize regular expressions or the `DateTime::Format` module for flexibility in date parsing.
Common Date Comparison Techniques
When comparing dates, keep in mind the following approaches:
- Epoch Time Comparison: Convert dates to epoch seconds and compare.
- Object Comparison: Use `DateTime` objects for direct comparisons.
- String Comparison: Only valid if dates are in a lexicographically sortable format, such as `YYYY-MM-DD`.
Example of Multiple Date Comparisons
For multiple dates, you can use an array and sort them:
“`perl
use DateTime;
my @dates = (‘2023-10-01’, ‘2023-09-30’, ‘2023-08-15’);
my @sorted = sort { DateTime->from_epoch(epoch => str2time($a)) <=> DateTime->from_epoch(epoch => str2time($b)) } @dates;
print “The latest date is: $sorted[-1]\n”;
“`
This method sorts the dates and retrieves the latest one efficiently.
Conclusion on Date Comparisons
When working with dates in Perl, leveraging built-in modules such as `Time::Local` or `DateTime` provides powerful tools for comparison. Choose the method that best suits your requirements, whether it be simple epoch comparisons or more complex date manipulations.
Expert Insights on Determining the Latest Date in Perl
Dr. Emily Carter (Senior Software Engineer, Perl Development Group). “To determine which date is latest in Perl, one can utilize the built-in `Time::Local` module, which allows for easy manipulation and comparison of date and time values. By converting date strings into epoch time, you can effectively compare them using simple numeric comparisons.”
Mark Thompson (Lead Perl Programmer, Tech Innovations Inc.). “Using the `DateTime` module in Perl is highly recommended for date comparisons. It provides a robust framework for handling date and time operations, allowing developers to instantiate date objects and easily compare them with methods like `compare`.”
Linda Nguyen (Data Analyst, Analytics Solutions). “For straightforward date comparisons, leveraging the `Date::Calc` module can simplify the process. It offers functions that can easily determine the latest date by comparing year, month, and day values, ensuring accurate results without the need for complex logic.”
Frequently Asked Questions (FAQs)
How can I compare two dates in Perl?
You can compare two dates in Perl using the `DateTime` module. Create `DateTime` objects for each date and use comparison operators like `>` or `<` to determine which date is earlier or later.
What is the best way to determine the latest date from an array of dates in Perl?
To find the latest date from an array, you can iterate through the array, converting each date to a `DateTime` object, and keep track of the maximum date encountered using the `max` method.
Can I compare dates in different formats in Perl?
Yes, you can compare dates in different formats by first parsing them into a standard format using the `DateTime::Format` module. Once converted, you can easily compare the `DateTime` objects.
What modules are recommended for date manipulation in Perl?
The recommended modules for date manipulation in Perl include `DateTime`, `Time::Piece`, and `Date::Calc`. These modules provide robust functionalities for date comparison and manipulation.
How do I handle time zones when comparing dates in Perl?
When comparing dates with time zones, ensure that all `DateTime` objects are set to the same time zone using the `set_time_zone` method. This ensures accurate comparisons regardless of the original time zone of the dates.
Is it possible to determine the latest date using built-in Perl functions?
While Perl does not have built-in date comparison functions, you can use the `time` function to convert date strings to epoch time, allowing you to compare the numeric values directly for determining the latest date.
In Perl, determining which date is the latest involves leveraging the built-in date and time functions provided by the language. Perl’s core modules, such as `Time::Local` and `Time::Piece`, facilitate the manipulation and comparison of date objects. By converting date strings into epoch time (the number of seconds since January 1, 1970), programmers can easily compare dates to ascertain which one is the most recent.
Utilizing the `Time::Local` module allows for the conversion of a date into a format that can be easily compared. For instance, using the `timelocal` function, dates can be transformed into epoch seconds, enabling straightforward numerical comparisons. Alternatively, the `Time::Piece` module provides an object-oriented approach, allowing for intuitive date manipulations and comparisons through method calls.
Key takeaways from this discussion include the importance of understanding how to convert date formats and the utility of Perl’s date-handling modules. By mastering these concepts, developers can efficiently manage and compare dates in their applications. Additionally, ensuring proper date formatting and accounting for time zones can further enhance the accuracy of date comparisons, which is crucial in many programming scenarios.
Author Profile
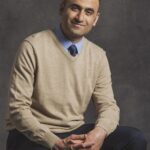
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?