How Can I Create a Form to Connect to an API?
In today’s digital landscape, the ability to seamlessly connect various applications and services is more crucial than ever. Whether you’re a developer looking to enhance user experience or a business owner aiming to streamline operations, creating a form that connects to an API can be a game-changer. APIs, or Application Programming Interfaces, serve as bridges between different software systems, enabling them to communicate and share data effortlessly. By harnessing the power of APIs through well-designed forms, you can unlock a world of possibilities, from automating data collection to integrating real-time information into your applications.
Creating a form to connect to an API involves understanding both the technical aspects of API interactions and the user experience of form design. At its core, this process requires a solid grasp of how to structure requests and handle responses, ensuring that data flows smoothly between the user interface and the backend services. Additionally, it’s essential to consider the various types of data you may need to collect, as well as the security measures necessary to protect sensitive information.
As we delve deeper into this topic, we will explore the fundamental steps involved in designing an effective form that communicates with an API. From selecting the right tools and technologies to implementing best practices for user interaction, you’ll gain insights that will empower you to create robust, functional forms
Designing the Form
Creating a form to connect to an API involves careful consideration of both user experience and technical requirements. The form should be intuitive, allowing users to input data easily. Key components of a well-designed form include:
- Input Fields: Clearly labeled fields for user data entry.
- Validation: Real-time validation to ensure that the input meets the API’s requirements.
- Feedback Messages: Informative messages that guide users on errors or successful submissions.
When designing the form, consider the types of data required by the API, such as text, numbers, dates, or selections. Each input type should have appropriate HTML elements:
Field Type | HTML Element | Description |
---|---|---|
Text | `` | For short text entries |
`` | For email address validation | |
Password | `` | For password inputs |
Number | `` | For numerical values |
Date | `` | For date selection |
Select | ` | For dropdown selections |
Implementing API Connection
To connect the form to an API, you need to capture the user input and send it to the API endpoint. This typically involves using JavaScript to handle the form submission. Here’s a basic example using the Fetch API:
“`javascript
document.getElementById(“myForm”).addEventListener(“submit”, function(event) {
event.preventDefault(); // Prevent the default form submission
const formData = new FormData(this);
const jsonData = Object.fromEntries(formData.entries());
fetch(“https://api.example.com/endpoint”, {
method: “POST”,
headers: {
“Content-Type”: “application/json”
},
body: JSON.stringify(jsonData)
})
.then(response => response.json())
.then(data => {
console.log(“Success:”, data);
})
.catch((error) => {
console.error(“Error:”, error);
});
});
“`
In this snippet, the form data is serialized into JSON format, which is a common requirement for API requests. You must also handle potential errors gracefully, providing feedback to the user if the API call fails.
Testing and Validation
Before deploying the form, thorough testing is essential. Consider the following testing strategies:
- Unit Testing: Validate individual components of the form and API connection.
- Integration Testing: Ensure that the form interacts correctly with the API.
- User Acceptance Testing: Gather feedback from users to improve usability.
Additionally, implement input validation on both the client and server sides. For example:
- Client-side Validation: Use JavaScript to check for empty fields or incorrect formats before submission.
- Server-side Validation: Ensure that the API processes incoming data correctly and responds with appropriate error messages when invalid data is received.
By following these guidelines, you can create a robust form that efficiently connects to an API, providing a seamless experience for users while ensuring data integrity and security.
Understanding API Basics
To create a form that connects to an API, it’s crucial to have a solid grasp of API functionalities and the data formats commonly used. APIs (Application Programming Interfaces) allow applications to communicate with one another, enabling data exchange and functionality integration.
- Types of APIs:
- REST (Representational State Transfer): Uses standard HTTP methods (GET, POST, PUT, DELETE).
- SOAP (Simple Object Access Protocol): Relies on XML and is often used for enterprise-level services.
- GraphQL: Allows clients to request only the data they need.
- Common Data Formats:
- JSON (JavaScript Object Notation): Lightweight and easy to read, widely used in REST APIs.
- XML (eXtensible Markup Language): More verbose, often used in SOAP APIs.
Designing the Form
The design of the form should be user-friendly and intuitive. Consider the following elements when creating your form:
- Input Fields: Determine which data you need from the user. Common types include:
- Text fields (for strings)
- Dropdowns (for selections)
- Radio buttons (for single-choice options)
- Checkboxes (for multiple selections)
- Validation: Implement client-side and server-side validation to ensure data integrity.
- Accessibility: Ensure that the form is accessible to all users, including those with disabilities.
Connecting the Form to the API
The next step involves writing the necessary code to connect the form to the API. Depending on the technology stack you are using, the implementation will vary. Below is a basic example using JavaScript and Fetch API for a RESTful API.
“`html
“`
Handling API Responses
After submitting the form, it’s essential to handle the API responses effectively. Possible outcomes include:
- Success Response:
- Display a success message or redirect the user.
- Error Handling:
- Show appropriate error messages based on the response.
- Implement retry logic for transient errors.
Status Code | Description | Action Required |
---|---|---|
200 | OK | Process the response |
400 | Bad Request | Display an error message |
401 | Unauthorized | Prompt for authentication |
500 | Internal Server Error | Log the error and notify user |
Testing the Integration
Thorough testing is vital to ensure the form and API integration work as intended. Consider the following testing strategies:
- Unit Testing: Test individual components of your code.
- Integration Testing: Verify that the form interacts correctly with the API.
- User Acceptance Testing (UAT): Ensure that the form meets user requirements and expectations.
Utilize tools such as Postman or Insomnia to test API endpoints independently before integrating them with your form.
Expert Insights on Creating Forms to Connect to APIs
Dr. Emily Carter (Lead Software Engineer, Tech Innovations Inc.). “When creating a form to connect to an API, it is crucial to ensure that the form captures all necessary data fields required by the API. This includes understanding the API’s documentation thoroughly to align the form inputs with the expected parameters.”
Michael Chen (API Integration Specialist, Cloud Solutions Group). “Security should be a top priority when designing forms that connect to APIs. Implementing proper validation and sanitization of user inputs helps prevent common vulnerabilities such as SQL injection and cross-site scripting.”
Sarah Thompson (UX/UI Designer, Digital Experience Agency). “User experience is paramount in form design. A well-structured form that provides clear instructions and feedback can significantly enhance user engagement and ensure successful API interactions.”
Frequently Asked Questions (FAQs)
What is the first step in creating a form to connect to an API?
The first step is to define the purpose of the form and the specific data you want to collect from users. This will guide the design and functionality of the form.
How do I choose the right API for my form?
Select an API that aligns with your data requirements and functionality goals. Review the API documentation to ensure it supports the necessary endpoints and data formats.
What technologies can I use to create a form that connects to an API?
You can use various technologies such as HTML for the form structure, JavaScript for client-side scripting, and frameworks like React or Angular for enhanced interactivity. Server-side languages like Python or Node.js can also be utilized for processing API requests.
How do I handle form submission to the API?
Implement an event listener for the form submission that captures the input data, formats it as required by the API, and sends it using an HTTP request method like POST or GET. Use libraries like Axios or Fetch API for easier handling of requests.
What should I do if the API returns an error after form submission?
Implement error handling in your code to capture and display meaningful error messages to users. Review the error response from the API to understand the issue and adjust your form or submission logic accordingly.
How can I ensure data security when connecting a form to an API?
Utilize HTTPS for secure data transmission, validate and sanitize user input to prevent injection attacks, and implement authentication mechanisms like OAuth if required by the API. Always adhere to best practices for data protection and privacy.
Creating a form to connect to an API involves several critical steps that ensure seamless data exchange between the user interface and the backend service. Initially, it is essential to understand the API’s requirements, including authentication methods, endpoint structures, and the expected data formats. This foundational knowledge allows developers to design forms that accurately capture user input and format it correctly for API requests.
Furthermore, implementing client-side validation is crucial to enhance user experience and ensure that only valid data is submitted. This can prevent unnecessary API calls and reduce server load. Additionally, handling API responses effectively is vital. Developers should implement mechanisms to manage success and error responses, providing users with clear feedback based on their interactions with the form.
Lastly, security considerations must not be overlooked. Protecting sensitive data, especially when dealing with user authentication and personal information, is paramount. Utilizing HTTPS for secure communication and adhering to best practices for API key management can significantly mitigate risks. Overall, a well-structured approach to creating forms that connect to APIs can lead to robust applications that provide valuable functionality and enhance user engagement.
Author Profile
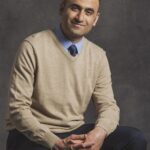
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?