How Can I Effectively Use Temp Tables in SQL Server?
In the realm of database management, SQL Server stands out as a powerful tool for handling complex data operations. One of its most versatile features is the use of temporary tables, which can significantly enhance performance and streamline processes. Whether you’re a seasoned database administrator or a budding developer, understanding how to effectively utilize temporary tables can transform the way you manage data within SQL Server. This article delves into the intricacies of temporary tables, exploring their benefits, use cases, and best practices to empower you to make the most of this essential feature.
Temporary tables in SQL Server serve as a temporary storage solution for data that is only needed for the duration of a session or a specific task. They allow for quick data manipulation and retrieval without the overhead of creating permanent tables. This flexibility is particularly useful in scenarios where complex queries require intermediate results, enabling developers to break down large operations into manageable steps. By leveraging temporary tables, users can optimize their queries, reduce execution time, and maintain a cleaner database environment.
Moreover, SQL Server offers various types of temporary tables, each suited for different scenarios. Understanding the distinctions between local and global temporary tables, as well as their respective lifecycles, is crucial for effective database management. As we explore the practical applications and advantages of using temporary tables,
Understanding Temporary Tables in SQL Server
Temporary tables in SQL Server are special tables that are created to store data temporarily during the session of a user or the execution of a specific procedure. They are particularly useful for storing intermediate results or for handling data that doesn’t need to be permanently stored. There are two types of temporary tables: local temporary tables and global temporary tables.
Local temporary tables are prefixed with a single “ symbol (e.g., `TempTable`), while global temporary tables are prefixed with two “ symbols (e.g., `GlobalTempTable`). Local temporary tables are visible only to the session that created them, whereas global temporary tables can be accessed by any session.
Creating Temporary Tables
Creating a temporary table is similar to creating a standard table in SQL Server, but with some specific syntax. Here is an example of how to create a local temporary table:
“`sql
CREATE TABLE TempTable (
ID INT,
Name VARCHAR(50),
CreatedDate DATETIME
);
“`
You can also define constraints, indexes, and other properties just as you would with a regular table. Once the temporary table is created, you can insert data into it using standard SQL commands.
Inserting Data into Temporary Tables
Data can be inserted into temporary tables using the `INSERT INTO` statement. Here’s an example:
“`sql
INSERT INTO TempTable (ID, Name, CreatedDate)
VALUES (1, ‘John Doe’, GETDATE());
“`
You can also insert data from other tables using a `SELECT` statement:
“`sql
INSERT INTO TempTable (ID, Name, CreatedDate)
SELECT ID, Name, CreatedDate FROM Users WHERE Active = 1;
“`
Using Temporary Tables in Queries
Temporary tables can be utilized in complex queries to simplify data manipulation and enhance performance. For example, you can join temporary tables with other tables to produce complex datasets or aggregate data for reporting purposes.
“`sql
SELECT t.ID, t.Name, COUNT(o.OrderID) AS TotalOrders
FROM TempTable t
LEFT JOIN Orders o ON t.ID = o.UserID
GROUP BY t.ID, t.Name;
“`
Benefits of Using Temporary Tables
The advantages of using temporary tables in SQL Server include:
- Performance: They can improve query performance by storing intermediate results.
- Simplicity: Simplify complex queries by breaking them down into manageable parts.
- Isolation: Data stored in temporary tables is isolated from other sessions, ensuring data integrity.
Limitations of Temporary Tables
While temporary tables offer several benefits, they also come with limitations:
- Scope: Local temporary tables are only accessible within the session that created them.
- Lifetime: Temporary tables are automatically dropped when the session ends or when they are explicitly dropped.
- Resource Usage: They consume resources and can lead to performance issues if overused.
Comparison of Temporary and Permanent Tables
Feature | Temporary Tables | Permanent Tables |
---|---|---|
Scope | Session-specific | Database-wide |
Lifetime | Short-lived | Persistent |
Performance | Optimized for temporary storage | Optimized for long-term data storage |
Usage | Intermediate results | Production data |
temporary tables are a powerful feature in SQL Server that facilitate efficient data management and querying within a session. Understanding their functionality and limitations is crucial for optimizing database performance.
Creating Temporary Tables in SQL Server
Temporary tables in SQL Server are created using the `CREATE TABLE` statement with a prefix of “ for local temporary tables or “ for global temporary tables. Local temporary tables are visible only within the session that created them, while global temporary tables are accessible to all sessions.
Syntax for Creating a Local Temporary Table:
“`sql
CREATE TABLE TempTable (
ID INT,
Name VARCHAR(100),
CreatedDate DATETIME
);
“`
Syntax for Creating a Global Temporary Table:
“`sql
CREATE TABLE GlobalTempTable (
ID INT,
Name VARCHAR(100),
CreatedDate DATETIME
);
“`
Inserting Data into Temporary Tables
Once a temporary table is created, data can be inserted into it using the `INSERT INTO` statement. This is similar to inserting data into a regular table.
Example of Inserting Data:
“`sql
INSERT INTO TempTable (ID, Name, CreatedDate)
VALUES (1, ‘John Doe’, GETDATE());
“`
Multiple rows can also be inserted at once:
“`sql
INSERT INTO TempTable (ID, Name, CreatedDate)
VALUES
(2, ‘Jane Smith’, GETDATE()),
(3, ‘Sam Johnson’, GETDATE());
“`
Querying Temporary Tables
Querying data from temporary tables is done in the same manner as querying from a permanent table. You can use `SELECT`, `JOIN`, and other SQL statements to manipulate and retrieve data.
Example Query:
“`sql
SELECT * FROM TempTable;
“`
You can also perform more complex queries, such as aggregations or joins with other tables:
“`sql
SELECT COUNT(*) AS TotalRecords FROM TempTable;
“`
Dropping Temporary Tables
Temporary tables are automatically dropped when the session that created them ends. However, you can manually drop them using the `DROP TABLE` statement at any time.
Example of Dropping a Temporary Table:
“`sql
DROP TABLE TempTable;
“`
For global temporary tables, the same command applies, but note that they will remain until all sessions referencing them are closed.
Best Practices for Using Temporary Tables
- Scope Awareness: Use local temporary tables when data does not need to be shared across sessions. Opt for global temporary tables when sharing is necessary.
- Naming Conventions: Use descriptive names for temporary tables to make your code more readable while still following the “ or “ convention.
- Performance: Be mindful of the number of rows in temporary tables; excessive data can lead to performance degradation. Consider using indexed temporary tables for large datasets.
- Cleanup: Always drop temporary tables when they are no longer needed to free resources.
Limitations and Considerations
Limitation | Description |
---|---|
Size Limitations | Temporary tables are subject to the same size limitations as regular tables. |
Indexing | Temporary tables can have indexes, but they must be created after the table is created. |
Transactions | Be cautious when using temporary tables in transactions; they may lock resources. |
Nested Temporary Tables | You can create temporary tables within stored procedures, but they will be dropped when the procedure exits. |
Using temporary tables effectively can enhance performance and manageability in SQL Server applications.
Expert Insights on Utilizing Temp Tables in SQL Server
Dr. Emily Chen (Database Architect, Tech Innovations Inc.). “Using temporary tables in SQL Server can significantly enhance query performance, especially when dealing with large datasets. They allow for intermediate results to be stored and manipulated without affecting the main database, providing a streamlined approach to complex queries.”
Michael Thompson (Senior Data Analyst, Analytics Solutions Group). “In my experience, temp tables are invaluable for breaking down complicated SQL processes into manageable steps. They not only improve readability but also facilitate debugging by allowing developers to inspect intermediate results during execution.”
Lisa Patel (SQL Server Consultant, DataWise Consulting). “While temp tables can be beneficial, it is crucial to use them judiciously. Over-reliance on temp tables can lead to increased resource consumption and potential performance bottlenecks. Proper indexing and cleanup after usage are essential to maintain optimal database performance.”
Frequently Asked Questions (FAQs)
What is a temp table in SQL Server?
A temp table in SQL Server is a temporary storage structure that holds data temporarily during a session. It is useful for storing intermediate results and is automatically dropped when the session ends.
How do I create a temp table in SQL Server?
You can create a temp table using the `CREATE TABLE` statement prefixed with a “ symbol for local temp tables (e.g., `CREATE TABLE TempTable (Column1 INT, Column2 VARCHAR(50));`).
What is the difference between a local and global temp table?
A local temp table (prefixed with “) is accessible only within the session that created it, while a global temp table (prefixed with “) is accessible to any session until all sessions referencing it are closed.
Can I use indexes on temp tables?
Yes, you can create indexes on temp tables just like regular tables. This can enhance performance for queries that involve large datasets.
Do temp tables consume resources in SQL Server?
Yes, temp tables consume system resources such as memory and disk space. However, they are generally more efficient for temporary data storage compared to permanent tables.
How do I drop a temp table in SQL Server?
You can drop a temp table using the `DROP TABLE` statement followed by the table name (e.g., `DROP TABLE TempTable;`). Local temp tables are automatically dropped at the end of the session.
In summary, utilizing temporary tables in SQL Server is a powerful technique that enhances data management and query performance. Temporary tables allow developers to store intermediate results, which can be particularly beneficial in complex queries or when dealing with large datasets. By creating a temporary table, users can simplify their SQL statements, improve readability, and optimize execution time by breaking down processes into manageable steps.
Moreover, temporary tables are session-specific, meaning they are automatically dropped when the session ends, which helps in managing resources effectively. This feature ensures that temporary data does not clutter the database, maintaining overall system performance. Additionally, SQL Server provides flexibility in defining temporary tables, allowing users to specify data types, constraints, and indexes, which can further enhance performance and data integrity.
Key takeaways include the importance of understanding the scope and lifespan of temporary tables, as well as their impact on query performance. Developers should consider using temporary tables for complex data manipulations and aggregations, where intermediate results are necessary. Furthermore, leveraging the capabilities of temporary tables can lead to cleaner code and improved maintainability in database applications.
Author Profile
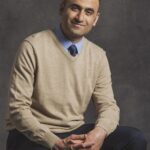
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?